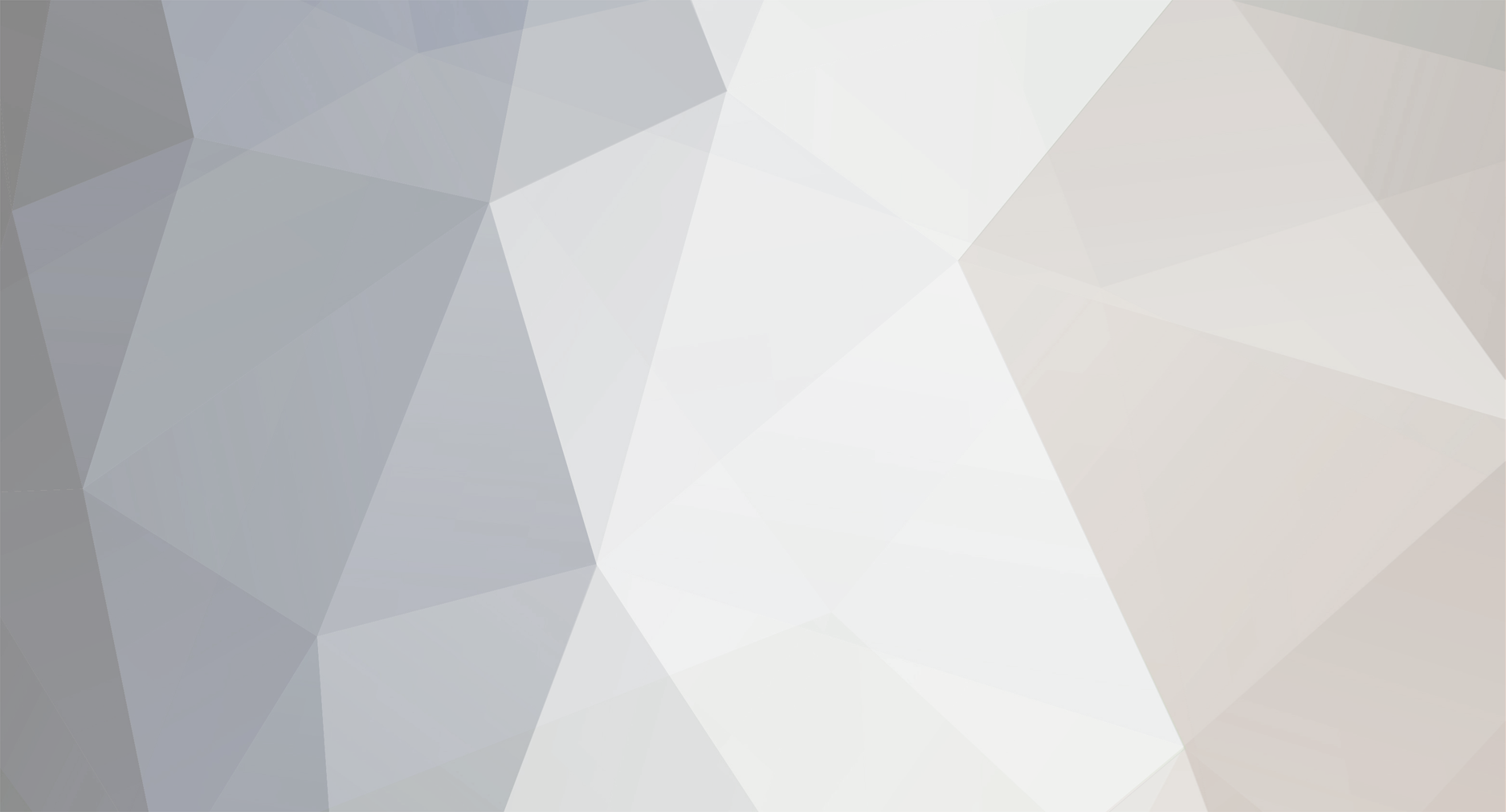
Unknown98
-
Posts
38 -
Joined
-
Last visited
Unknown98's Achievements

Member (2/5)
0
Reputation
Unknown98 has no recent activity to show
0
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.