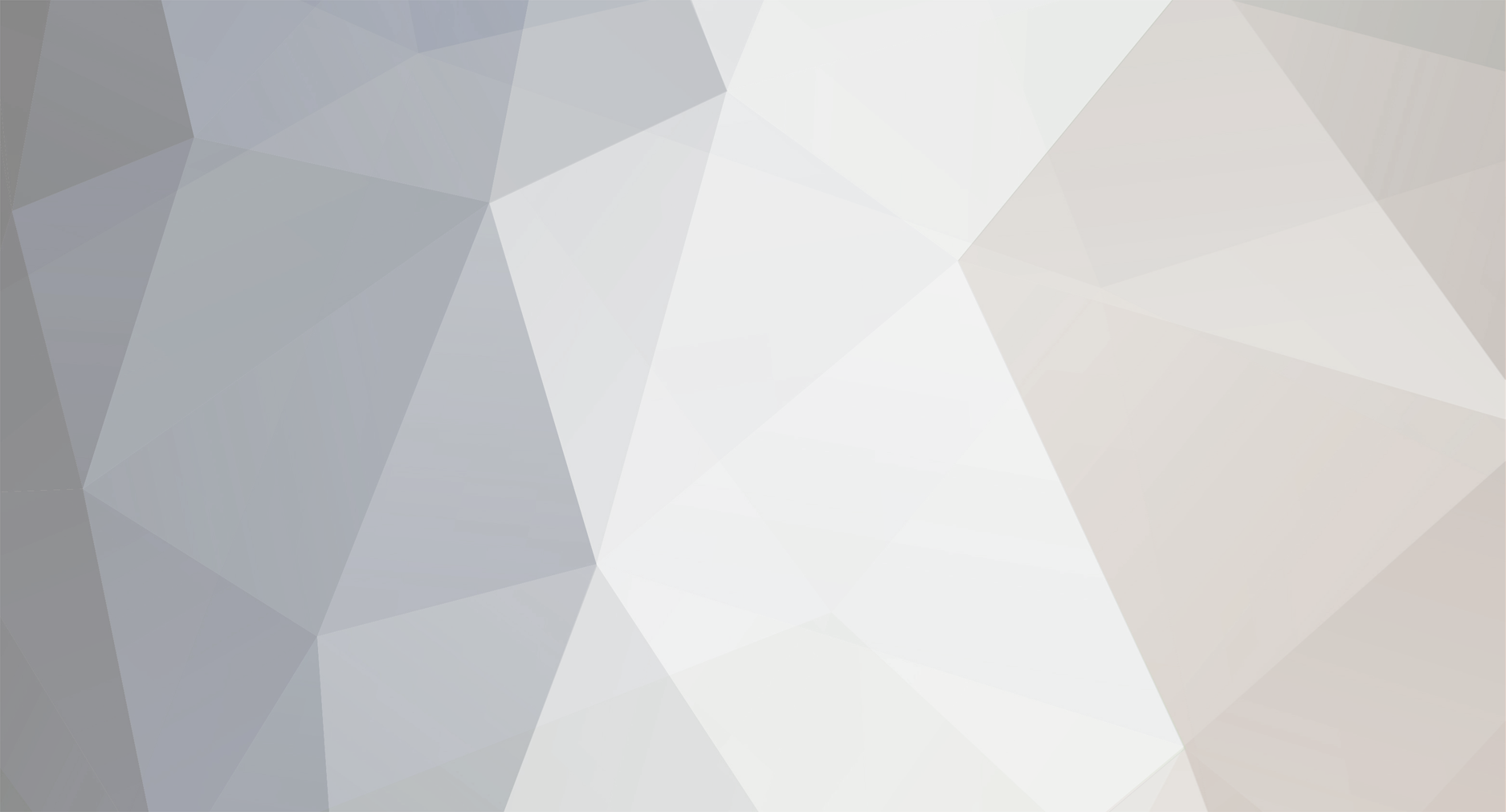
dontpanic
Members-
Posts
11 -
Joined
-
Last visited
Never
Everything posted by dontpanic
-
I'm leaving work right now so just a quick response: it seems like you're doing things right from a distance but, again, the answer is in your code. I just recently finished coding up something similar in function to a chat room using asynchronous techniques (I refuse to use the marketing term, ajax) and my script is making hundreds of queries every iteration without any noticeable latency. I used one table for raw data and one table for metadata, and initiated my script when the metadata was in the right state. Anyway, hopefully someone else can provide more insight than I or you find the reason soon! Now its time to leave work and become inebriated. TGIF.
-
Well, unfortunately its impossible to provide a solution without seeing all of the code and database, but I can say that using a flat file will definitely be slower and prone to corruption. Lets try to get some more info and hammer this out... Are you hosting your own server or are you going through a hosting company? Are your HTTP and MySQL servers running on the same machine? If not, what is their latency (ping)? Does your php code that accesses the database get executed more frequently then needed? Is it really necessary to post every new message as it arrives? Why not buffer some sql in a local text file that only gets executed after a set time? (This isn't the same as using text files as a database, appending is very fast) Those are just a few things I could think of randomly, and I'm sure theres plenty more effecting your script's execution time. My suggestion is to really study your code and see where the most work is being done. Litter it with time() calculations to see how long your SQL queries take, and stuff like that. Plenty of chat rooms out there use php and MySQL and can handle huge loads; know this, and know that your code just needs optimization. One last thing is the structure of your database, which can greatly effect the speed of your queries. I'm terrible at database design so don't ask me, but if you find that it may be the problem, hop on over to the MySQL forums and ask around.
-
// Change the fopen mode from 'w' to 'a+' $fh = fopen($myFile, 'a+') or die("can't open file"); and for future reference, see the manual. You don't. Thats why God invented databases. In all honesty MySQL will always be faster and safer than a flat file, and can handle tons of requests in a short amount of time. Try changing the way you access the database if it really is slowing you down.
-
OOP linking parent/child classes (and if to instantiate or not)
dontpanic replied to johnsmith153's topic in PHP Coding Help
Either make another class, called something like "Logger", and pass an object of that type into your User or Database class's constructor and manage a log from there, or you could parse your class's return values and report in the procedural part of your script. for example, $logger = new Logger(); $myObj = new User($logger); $myOtherObj = new User($logger); // Uses the same logger! $myObj->do_something_bad(); // This function fails for whatever reason and reports to $logger or $myObj = new User(); $ret = $myObj->do_something_bad(); if ($ret == false) { echo 'An error occurred.'; } Could be possible solutions, but I'm sure you could think up any number of alternatives. This is one of those things that has lots of solutions, some good and some bad, but it all comes down to preference and context. If your class methods are atomic enough (as in, they do exactly one complete operation), it is very easy to check return values and report in the global scope. If your methods are long-winded it may be neccessary to report warnings or other non-critical sorts of things before you want to return. In that case I would go with a logger object that is either global (easy, not safe) or instanced (difficult, but safe). My first example uses a global logger object. -
OOP linking parent/child classes (and if to instantiate or not)
dontpanic replied to johnsmith153's topic in PHP Coding Help
class User extends DatabaseControl You are missing a fundamental concept of object orientation (OO). Unlike procedural programming, OO is all about separating your data into small, logical groups called classes. The way your rolling all of your database functionality into your User objects tells us that you are thinking of objects incorrectly. Don't inherit Database with your User class, simply pass an object of type Database into your User constructor like our example code has shown. From inside the User class you can use that previously created database object to manipulate your database while ensuring only one connection is ever made. Honestly you should start with with exactly two classes, and expand to many classes with inheritance later on. Use a single Database class to encapsulate all database functionality, and a single User class to define a generic user. Have a look at php.net's object orientation tutorial. Its not the best introduction to OO, but I'm certain it will turn on a lot of lights for you. -
OOP linking parent/child classes (and if to instantiate or not)
dontpanic replied to johnsmith153's topic in PHP Coding Help
The global keyword is pretty tricky. You cannot have global member variables (it doesn't make sense, really), but you can use global variables within functions: class Foo { public function do_something() { global $var; echo "In class Foo, var=$var\n"; } } $obj = new Foo(); $var = 'bar'; $obj->do_something(); $var = 'changed'; $obj->do_something(); C:\Scripts>php .\test2.php In class Foo, var=bar In class Foo, var=changed -
OOP linking parent/child classes (and if to instantiate or not)
dontpanic replied to johnsmith153's topic in PHP Coding Help
I was working on a reply but AbraCadaver hit all the points I needed to make. Here's the example I had going just for kicks: // Database class from http://snipplr.com/view/12697.16543/ class Database { private $host; private $user; private $pwd; // etc ... } // Your home-brewed User class class User { private $db; private $id; public $name; public function __construct($database, $id) { $this->db = $database; $this->id = $id } public function update_record() { $this->db->query("UPDATE employee_table SET name='$this->name' WHERE id='$this->id'"); } // etc... } // Now using these classes is very simple. Every object of type 'User' will // need to know what database to work on. // Instantiate our employee database $employee_db = new Database(); $employee_db->init('localhost', 'mysql_user', 'mysql_password', 'employee_db_name'); // Instantiate our customer database $customer_db = new Database(); $customer_db->init('localhost', 'mysql_user', 'mysql_password', 'customer_db_name'); // Now on to the users, lets work with an employee-style user $some_employee = new User($employee_db); $some_employee->name = "John Doe"; // Change some data $some_employee->update_record(); // Update database record // And using the second database... $some_customer = new User($customer_db); // etc... -
That did it! I can't believe how much I was over thinking that ":<password>-" part. Thanks a ton, sasa!
-
That got me closer, but not quite there... $pattern = '/(?P<name>^[^-]+)-(?P<username>[^:]+)?P<password>[^@]+)@(?P<server>.*$)/'; $subject = 'name-username:p@ssword@server'; preg_match($pattern, $subject, $matches); print_r($matches); yields Array ( [0] => name-username:p@ssword@server [name] => name [1] => name [username] => username [2] => username [password] => p [3] => p [server] => ssword@server [4] => ssword@server ) I do feel like we're really close though...
-
OOP linking parent/child classes (and if to instantiate or not)
dontpanic replied to johnsmith153's topic in PHP Coding Help
If ClassB inherits from ClassA (using the extend keyword), instantiating an object of ClassB automatically instantiates the ClassA-inherited members. You could instantiate an object of CassA, but it would only have the members and methods of ClassA and would have NO knowledge of ClassB (ClassA can't access ClassB's members, inheritance is a one-way street). Here's an example: <?php // test.php class Widget { public $foo = 'hello'; public $bar = 'world'; function widgetize() { echo $this->foo . ' ' . $this->bar . "\n"; } } class WidgetConfabilator extends Widget { function confabilate($new_bar) { $this->bar = $new_bar; } } $myObj = new WidgetConfabilator(); $myObj->widgetize(); $myObj->confabilate("php land"); $myObj->widgetize(); // Reusing same variable--I'm lazy $myObj = new Widget(); $myObj->widgetize(); $myObj->confabilate("php land"); // Error $myObj->widgetize(); // This code never gets executed ?> C:\Scripts>php .\test.php hello world hello php land hello world Fatal error: Call to undefined method Widget::confabilate() in C:\Scripts\test.php on line 31 -
I'm trying to match something along the lines of: "name-username:password@server," and I can do that with this regex: $pattern = '/(?P<name>\w+)-(?P<username>\w+)?P<password>\w+)@(?P<server>\w+)/' But things start going wrong with a password that contains one of the delimiter characters. I could change the password \w+ to something more specific ([a-zA-Z0-9!@#$^&*()_-=+]) but something in my gut tells me thats not right. I don't have enough experience with regular expressions to tackle this and was wondering if anyone here has any ideas. This format (other than the first '-') is commonly used when logging into websites, so I imagine its been parsed before. I think I need a sort of greedy negative look-behind, but I just can't seem to grasp how to go about it. Thanks in advance for your help! Update: here is a list of non-alphanumeric characters that passwords may contain : ~!@#$%^&*()_+{}|:"<>?/.,';\][=-`)