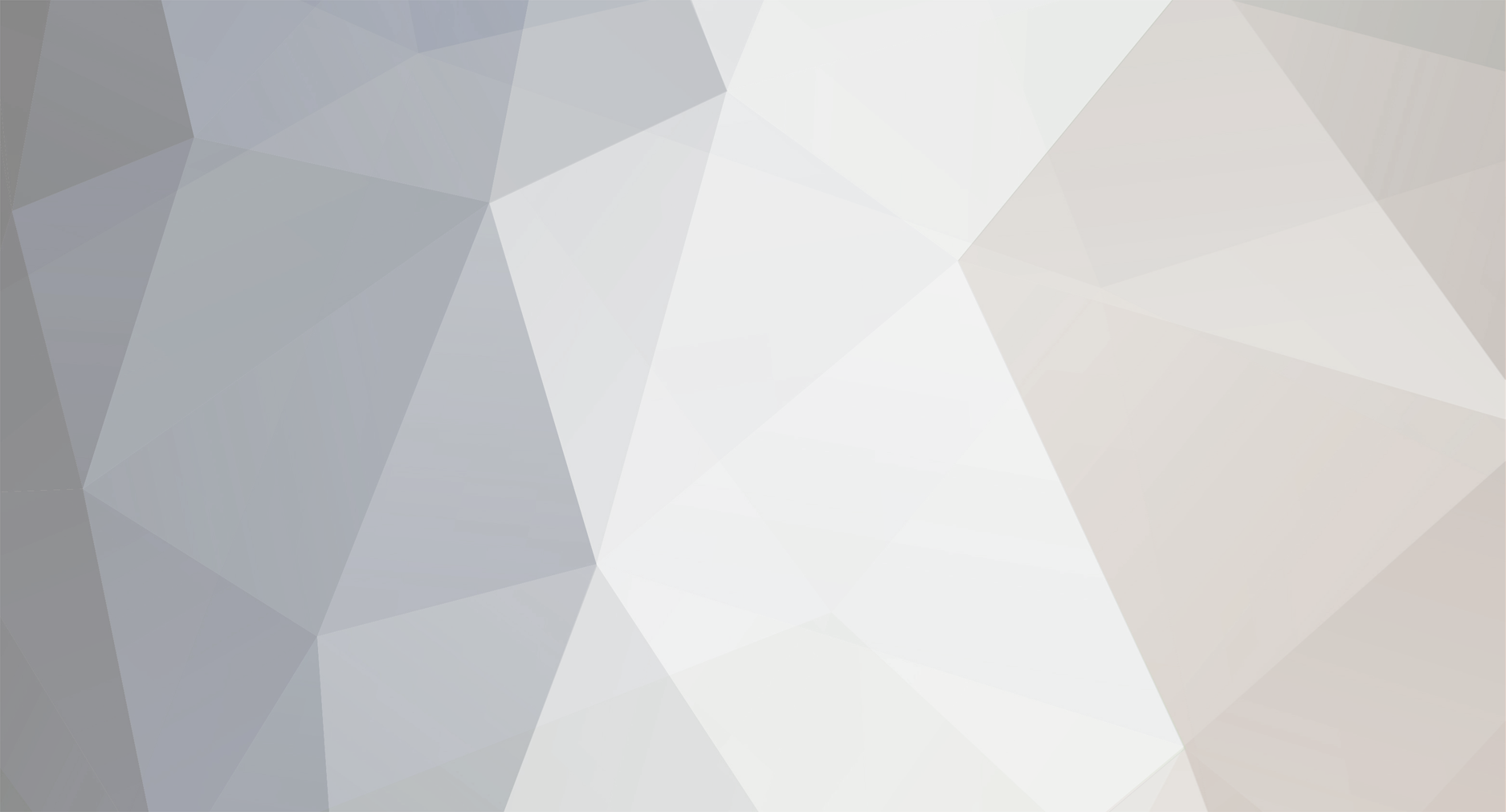
QuickOldCar
Staff Alumni-
Posts
2,972 -
Joined
-
Last visited
-
Days Won
28
Everything posted by QuickOldCar
-
The function you posted creates an array of all the results. I assume after this function you are doing something else to add another set of results to the same array. I don't really know why this function is used to insert data, it has to do a lot just to find the next row. What are you using for a database? Lets say if using mysql, usually everyone uses auto increment as the primary on an id field. You can just insert into next available id. Which is very fast and uses hardly anything. Or can do checks: if it exists...either do nothing or update the exisiting data, if does not exist then insert by next id . Making indexes on any WHERE or AND values in the select statements also speeds up the entire fetching process.
-
I thought you showed your db and it shows stuff like chair,shoe,tv as product. Well you can also do something like this. <?php $product = "chair"; $getcount = mysql_query("SELECT product FROM inventory_file WHERE product='$product'"); $productcount = mysql_num_rows($getcount); echo $productcount; ?>
-
Maybe what you should do is normal mysql select queries and then pagination to split up the data. And forget that function you made. As I said, either the data you are trying to fetch is too large to display, or maybe even your shared host doesn't have the full 128mb available for you any longer. But even if it uses 128mb I would do something different to get the results.
-
Of the 2 you mentioned it would be preg_replace. http://php.net/manual/en/function.preg-replace.php But for this you can use str_replace http://php.net/manual/en/function.str-replace.php $str = str_replace(" ", ".", trim($str)); I added trim just to be sure it doesn't add an extra . if is leading or trailing whitespace.
-
Can you put a youtube link in script to display in a page
QuickOldCar replied to rdkd1970's topic in PHP Coding Help
Try this. http://code.google.com/apis/youtube/youtube_player_demo.html so need the video id <object width="220" height="148"><param name="movie" value="http://www.youtube.com/v/LRAYG7XiFtk?version=3&feature=player_embedded"></param><param name="allowFullScreen" value="true"></param><param name="allowscriptaccess" value="always"></param><embed src="http://www.youtube.com/v/LRAYG7XiFtk?version=3&feature=player_embedded" type="application/x-shockwave-flash" allowscriptaccess="always" allowfullscreen="true" width="220" height="148"></embed></object> -
Mysql server hosting to support massive loads...
QuickOldCar replied to ajlisowski's topic in MySQL Help
There is gonna be limits on how many you can actually do depending on the scripts you run, cpu usage, memory, amount of traffic and then your actual bandwidth. Not to mention most likely tweaking apache to become more efficient. A single dedicated server can do what you want. (shared sucks) I really doubt "about a millions of queries per minute" One of the above I mentioned would most likely bottleneck you. Sure if want to spend lots of $$. With doing so many requests you will have to establish some sort of cache just to take the load off, even if they are unique, if it even saves one fetch to the database it will save you a lot. As a note, myisam locks the table on updates, which means no fetching until that is completed. This article explains some of the good and bads of MyISAM and InnoDB http://tag1consulting.com/MySQL_Engines_MyISAM_vs_InnoDB Silly me... I'm using myisam because I wanted the full text indexing (I most likely will use cassandra in the future). And while on the subject, I'll give a link for cassandra http://cassandra.apache.org/ Cassandra is in use at Digg, Facebook, Twitter, Reddit, Rackspace, Cloudkick, Cisco, SimpleGeo, Ooyala, OpenX, and more companies that have large, active data sets. The largest production cluster has over 100 TB of data in over 150 machines. As can see it's just not that easy to display lots of different data to many people at the same time without having lots of hardware and a proper database designed for server clusters. -
You should post your code. But are you doing a check if action is set or not? if(isset($_GET['action'])) { //execute the query } //or even if(!isset($_GET['action']) || $_GET['action'] == "") { //do not execute the query } else { //execute the query }
-
Try this in the php.ini file Raise limits until see working. memory_limit = 128M post_max_size = 15M upload_max_filesize = 10M I don't believe upload time counts towards execution time when using move_uploaded_file.
-
Well since the data appears to be just 32bytes, maybe it's stuck in an infinite loop. So continues to run until your memory limit is reached. do you even need this in your foreach? $i++; especially since it is not a for or a while and no limit is set. foreach itself should return all the results
-
Here at phpfreaks is a pagination tutorial. http://www.phpfreaks.com/tutorial/basic-pagination I made a pagination myself, each link below is a bit different. The demo and codes are at each. This one is hard set to 10 results per page, the more calculations isn't worth the effort to me. http://get.blogdns.com/dynaindex/paginate.php Then this one can set any amount per page you want. http://get.blogdns.com/paginate/ You will see the idea of pagination is to specify what row to start on, how many results from that point, then for each new page that many more results are displayed, until there is no more results. The mysql start row begins at zero.
-
Searchbar with multiple select options
QuickOldCar replied to fernando1980's topic in PHP Coding Help
You should also post your showroom.php here. Remember to hide from the code for your user login information to your database. What you need to do is use multiple queries, or a multiple variable value in your select queries. -
how to use select box for displaying a database value
QuickOldCar replied to rabesh's topic in PHP Coding Help
http://www.tizag.com/mysqlTutorial/mysqlquery.php -
You most likely are running out of memory. Either raise the limit in php.ini, or better yet make a more efficient function. Judging by your code you are getting all the results from a table and displaying it, the table is getting large.
-
Need a Pro advice on learning PHP for automation
QuickOldCar replied to ars.magna88's topic in PHP Coding Help
The better you know the programming language the more efficient and faster you will get tasks completed. Since I feel everyone doesn't know everything, and even if they know more than others....mistakes or bad judgement still occurs. It would be wise to try to learn the basics as much as can, then try to learn the more advanced as you progress. Coming to this forum was a very good start for you, I myself learned all by myself with no guidance...and it was a hard long road. With help I'm sure it makes learning a lot easier. -
here's one way make a text file named numbers.txt same location as this script, or rename it to your text file <?php $my_file = "numbers.txt"; if (file_exists($my_file)) { $data = file($my_file); $number = $data[rand(0, count($data) - 1)]; echo $number; } else { echo "$my_file does not exist"; } ?>
-
I think the first thing to get do is to set up each of your select queries in if/elseif/else depending what ad type need to display. http://php.net/manual/en/control-structures.elseif.php Then you can move onto reading about pagination for that one select result. http://www.phpfreaks.com/tutorial/basic-pagination
-
I sat here for a bit, and I think I came up with something that can work for you. When you visit the page it will show all results, and you can then select any options to see the different values for each. The query will build from the selects, and place the WHERE,AND in the proper places. The POST empty or ALL gets the same results <form name="input" action="" method="post"> Make: <Select name="make"> <option "Input" value="<?php echo $_POST['make']; ?>"><?php echo $_POST['make']; ?></option> <option value="ALL">ALL</option> <option value="Ford">ford</option> <option value="BMW">BMW</option> <option value="Honda">Honda</option> <option value="Lexus">Lexus</option> </select> Model: <Select name="model"> <option "Input" value="<?php echo $_POST['model']; ?>"><?php echo $_POST['model']; ?></option> <option value="ALL">ALL</option> <option value="Civic">Civic</option> <option value="3 Series">3 Series</option> <option value="Fiesta">Fiesta</option> <option value="IS200">IS200</option> </select> Fuel: <Select name="fuel"> <option "Input" value="<?php echo $_POST['fuel']; ?>"><?php echo $_POST['fuel']; ?></option> <option value="ALL">ALL</option> <option value="Petrol">Petrol</option> <option value="Diesel">Diesel</option> </select> <input type="submit" value="Search Cars" /> </form> <?php //connect to mysql $con = mysql_connect("server","a5525005_cars","password"); if (!$con) { die('Could not connect: ' . mysql_error()); } //select database mysql_select_db("a5525005_cars", $con); //variables from selection form $make = mysql_real_escape_string($_POST['make']); $model = mysql_real_escape_string($_POST['model']); $fuel = mysql_real_escape_string($_POST['fuel']); //default query $query .= "SELECT * FROM `cars`"; //count the selections for queries $query_count = 0; //set queries and associate WHERE,AND if(isset($_POST['make']) && $_POST['make'] != "ALL" && $_POST['make'] != ""){ $query_count = $query_count +1; if($query_count == 1) { $where_and = "WHERE"; } else { $where_and = "AND"; } $query .= " $where_and Make='$make'"; } if(isset($_POST['model']) && $_POST['model'] != "ALL" && $_POST['model'] != ""){ $query_count = $query_count +1; if($query_count == 1) { $where_and = "WHERE"; } else { $where_and = "AND"; } $query .= " $where_and Model='$model'"; } if(isset($_POST['fuel']) && $_POST['fuel'] != "ALL" && $_POST['fuel'] != ""){ $query_count = $query_count +1; if($query_count == 1) { $where_and = "WHERE"; } else { $where_and = "AND"; } $query .= " $where_and Fuel='$fuel'"; } //echo $query;//query built from values above depending on selections $result = mysql_query("$query"); //check if results if(!$result){ echo "No Results <br />"; } else { echo "<table class='ex1' border='0' width='120%' style=text-align:center; cellpadding='6' cellspacing='0'> </tr>"; while($row = mysql_fetch_array($result)) { echo "<tr style=font-family:verdana;font-size:80%;>"; echo "<td width=13%>" . $row[""] . "<img src=\"" . $row["Photo"] . "\"></a>"; echo '<td width="14%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Make'] . '</a></td>'; echo '<td width="5%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Model'] . '</a></td>'; echo '<td width="4%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Fuel'] . '</a></td>'; echo "</tr>"; } echo "</table>"; } mysql_close($con); ?> Next you need to work on pagination.
-
Give this a whirl ///Combo for Makes <form name="input" action="" method="post"> Make: <Select name="make"> <option "Input" value="<?php echo $_POST['make']; ?>"><?php echo $_POST['make']; ?></option> <option value="Ford">ford</option> <option value="BMW">BMW</option> <option value="Honda">Honda</option> <option value="Lexus">Lexus</option> </select> ///combo for Models Model: <Select name="model"> <option "Input" value="<?php echo $_POST['model']; ?>"><?php echo $_POST['model']; ?></option> <option value="ALL">ALL</option> <option value="Civic">Civic</option> <option value="3 Series">3 Series</option> <option value="Fiesta">Fiesta</option> <option value="IS200">IS200</option> </select> ///Combo for Fuel Fuel: <Select name="fuel"> <option "Input" value="<?php echo $_POST['fuel']; ?>"><?php echo $_POST['fuel']; ?></option> <option value="ALL">ALL</option> <option value="Petrol">Petrol</option> <option value="Diesel">Diesel</option> </select> <input type="submit" value="Search Cars" /> </form> <?php if(!isset($_POST) || $_POST['make'] == "") { echo "<h2>Please check all values.</h2>"; } else { $con = mysql_connect("server","a5525005_cars","password"); $make = mysql_real_escape_string($_POST['make']); $model = mysql_real_escape_string($_POST['model']); $fuel = mysql_real_escape_string($_POST['fuel']); //set to ALL if empty if($model == ""){ $model == "ALL"; } if($fuel == ""){ $fuel == "ALL"; } //set queries if($model == "ALL"){ $model_query = ""; } else { $model_query = " AND Model='$model'"; } if($fuel == "ALL"){ $fuel_query = ""; } else { $fuel_query = " AND Fuel='$fuel'"; } if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("a5525005_cars", $con); $result = mysql_query("SELECT * FROM `cars` WHERE Make='$make' $model_query $fuel_query"); if(!$result){ echo "No Results <br />"; } else { echo "<table class='ex1' border='0' width='120%' style=text-align:center; cellpadding='6' cellspacing='0'> </tr>"; while($row = mysql_fetch_array($result)) { echo "<tr style=font-family:verdana;font-size:80%;>"; echo "<td width=13%>" . $row[""] . "<img src=\"" . $row["Photo"] . "\"></a>"; echo '<td width="14%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Make'] . '</a></td>'; echo '<td width="5%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Model'] . '</a></td>'; echo '<td width="4%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Fuel'] . '</a></td>'; echo "</tr>"; } echo "</table>"; } mysql_close($con); } ?>
-
There was a quote missing george. $date1 = date("Y/m", strtotime("-6 months", time()));
-
<?php $date = date('Y/m'); echo $date; echo "<br/>"; $date1 = date("Y/m", strtotime("-6 month")); echo $date1; ?> Results: 2011/05 2010/12
-
Could you post your current code so can see what are trying to accomplish?
-
Nope, not sick of you, am glad to help and is why I come here. Here's a trick I do and use a multiple query. Either one should work. <form name="input" action="" method="post"> Car Make: <Select name="make"> <option "Input" value="<?php echo $_POST['make']; ?>"><?php echo $_POST['make']; ?></option> <option value="ford">ford</option> <option value="chevrolet">chevrolet</option> <option value="honda">honda</option> <option value="lexus">lexus</option> </select> Car Model: <Select name="model"> <option "Input" value="<?php echo $_POST['model']; ?>"><?php echo $_POST['model']; ?></option> <option value="ALL">ALL</option> <option value="civic">civic</option> <option value="corsica">corsica</option> <option value="lumina">lumina</option> <option value="delsol">delsol</option> </select> <input type="submit" value="Get The Results" /> </form> <?php if(!isset($_POST) || $_POST['make'] == "" || $_POST['model'] == "") { echo "<h2>Please check both values.</h2>"; } else { $con = mysql_connect("server","database","password"); $make = mysql_real_escape_string($_POST['make']); $model = mysql_real_escape_string($_POST['model']); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("a5525005_cars", $con); if($model == "ALL") { $model_query = ""; } else { $model_query = "AND Model='$model'"; } $result = mysql_query("SELECT * FROM `cars` WHERE Makel='$make' $model_query");// added the variables in the query echo "<table class='ex1' border='0' width='113%' style=text-align:center; cellpadding='6' cellspacing='0'> </tr>"; while($row = mysql_fetch_array($result)) { echo "<tr style=font-family:verdana;font-size:80%;>"; echo "<td width=13%>" . $row[""] . "<img src=\"" . $row["Photo"] . "\"></a>"; echo '<td width="14%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Model'] . '</a></td>'; echo '<td width="5%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Year'] . '</a></td>'; echo '<td width="4%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Fuel'] . '</a></td>'; echo "</tr>"; } echo "</table>"; mysql_close($con); } ?> or you can also do it with different select queries <form name="input" action="" method="post"> Car Make: <Select name="make"> <option "Input" value="<?php echo $_POST['make']; ?>"><?php echo $_POST['make']; ?></option> <option value="ford">ford</option> <option value="chevrolet">chevrolet</option> <option value="honda">honda</option> <option value="lexus">lexus</option> </select> Car Model: <Select name="model"> <option "Input" value="<?php echo $_POST['model']; ?>"><?php echo $_POST['model']; ?></option> <option value="ALL">ALL</option> <option value="civic">civic</option> <option value="corsica">corsica</option> <option value="lumina">lumina</option> <option value="delsol">delsol</option> </select> <input type="submit" value="Get The Results" /> </form> <?php if(!isset($_POST) || $_POST['make'] == "" || $_POST['model'] == "") { echo "<h2>Please check both values.</h2>"; } else { $con = mysql_connect("server","database","password"); $make = mysql_real_escape_string($_POST['make']); $model = mysql_real_escape_string($_POST['model']); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("a5525005_cars", $con); if($model == "ALL") { $result = mysql_query("SELECT * FROM `cars` WHERE Makel='$make'"); } else { $result = mysql_query("SELECT * FROM `cars` WHERE Makel='$make' AND Model='$model'"); } echo "<table class='ex1' border='0' width='113%' style=text-align:center; cellpadding='6' cellspacing='0'> </tr>"; while($row = mysql_fetch_array($result)) { echo "<tr style=font-family:verdana;font-size:80%;>"; echo "<td width=13%>" . $row[""] . "<img src=\"" . $row["Photo"] . "\"></a>"; echo '<td width="14%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Model'] . '</a></td>'; echo '<td width="5%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Year'] . '</a></td>'; echo '<td width="4%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Fuel'] . '</a></td>'; echo "</tr>"; } echo "</table>"; mysql_close($con); } ?> I also have another idea, we can set the default for model as ALL, this way will always get a result. <form name="input" action="" method="post"> Car Make: <Select name="make"> <option "Input" value="<?php echo $_POST['make']; ?>"><?php echo $_POST['make']; ?></option> <option value="ford">ford</option> <option value="chevrolet">chevrolet</option> <option value="honda">honda</option> <option value="lexus">lexus</option> </select> Car Model: <Select name="model"> <option "Input" value="<?php echo $_POST['model']; ?>"><?php echo $_POST['model']; ?></option> <option value="ALL">ALL</option> <option value="civic">civic</option> <option value="corsica">corsica</option> <option value="lumina">lumina</option> <option value="delsol">delsol</option> </select> <input type="submit" value="Get The Results" /> </form> <?php if(!isset($_POST) || $_POST['make'] == "") { echo "<h2>Please check both values.</h2>"; } else { $con = mysql_connect("server","database","password"); $make = mysql_real_escape_string($_POST['make']); $model = mysql_real_escape_string($_POST['model']); if($_POST['model'] == ""){ $model = "ALL"; } if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("a5525005_cars", $con); if($model == "ALL") { $result = mysql_query("SELECT * FROM `cars` WHERE Makel='$make'"); } else { $result = mysql_query("SELECT * FROM `cars` WHERE Makel='$make' AND Model='$model'"); } echo "<table class='ex1' border='0' width='113%' style=text-align:center; cellpadding='6' cellspacing='0'> </tr>"; while($row = mysql_fetch_array($result)) { echo "<tr style=font-family:verdana;font-size:80%;>"; echo "<td width=13%>" . $row[""] . "<img src=\"" . $row["Photo"] . "\"></a>"; echo '<td width="14%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Model'] . '</a></td>'; echo '<td width="5%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Year'] . '</a></td>'; echo '<td width="4%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Fuel'] . '</a></td>'; echo "</tr>"; } echo "</table>"; mysql_close($con); } ?>
-
A blog is only a blog if you use it as a blog. I feel if you use it as a cms for information and latest news, then it's now that. If anything I'd just rename the blog to tips, current events, latest news, information, so on
-
I "meant" to move it below the connection, obviously I didn't, ha ha.
-
try this <form name="input" action="" method="post"> Car Make: <Select name="make"> <option "Input" value="<?php echo $_POST['make']; ?>"><?php echo $_POST['make']; ?></option> <option value="ford">ford</option> <option value="chevrolet">chevrolet</option> <option value="honda">honda</option> <option value="lexus">lexus</option> </select> Car Model: <Select name="model"> <option "Input" value="<?php echo $_POST['model']; ?>"><?php echo $_POST['model']; ?></option> <option value="civic">civic</option> <option value="corsica">corsica</option> <option value="lumina">lumina</option> <option value="delsol">delsol</option> </select> <input type="submit" value="Get The Results" /> </form> <?php if(!isset($_POST) || $_POST['make'] == "" || $_POST['model'] == "") { echo "<h2>Please check both values.</h2>"; } else { $make = mysql_real_escape_string($_POST['make']); $model = mysql_real_escape_string($_POST['model']); $con = mysql_connect("server","database","password"); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("a5525005_cars", $con); $result = mysql_query("SELECT * FROM `cars` WHERE Makel='$make' AND Model='$model'");// added the variables in the query echo "<table class='ex1' border='0' width='113%' style=text-align:center; cellpadding='6' cellspacing='0'> </tr>"; while($row = mysql_fetch_array($result)) { echo "<tr style=font-family:verdana;font-size:80%;>"; echo "<td width=13%>" . $row[""] . "<img src=\"" . $row["Photo"] . "\"></a>"; echo '<td width="14%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Model'] . '</a></td>'; echo '<td width="5%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Year'] . '</a></td>'; echo '<td width="4%"><a class="mylink" href="' . $row['URL'] . '">' . $row['Fuel'] . '</a></td>'; echo "</tr>"; } echo "</table>"; mysql_close($con); } ?>