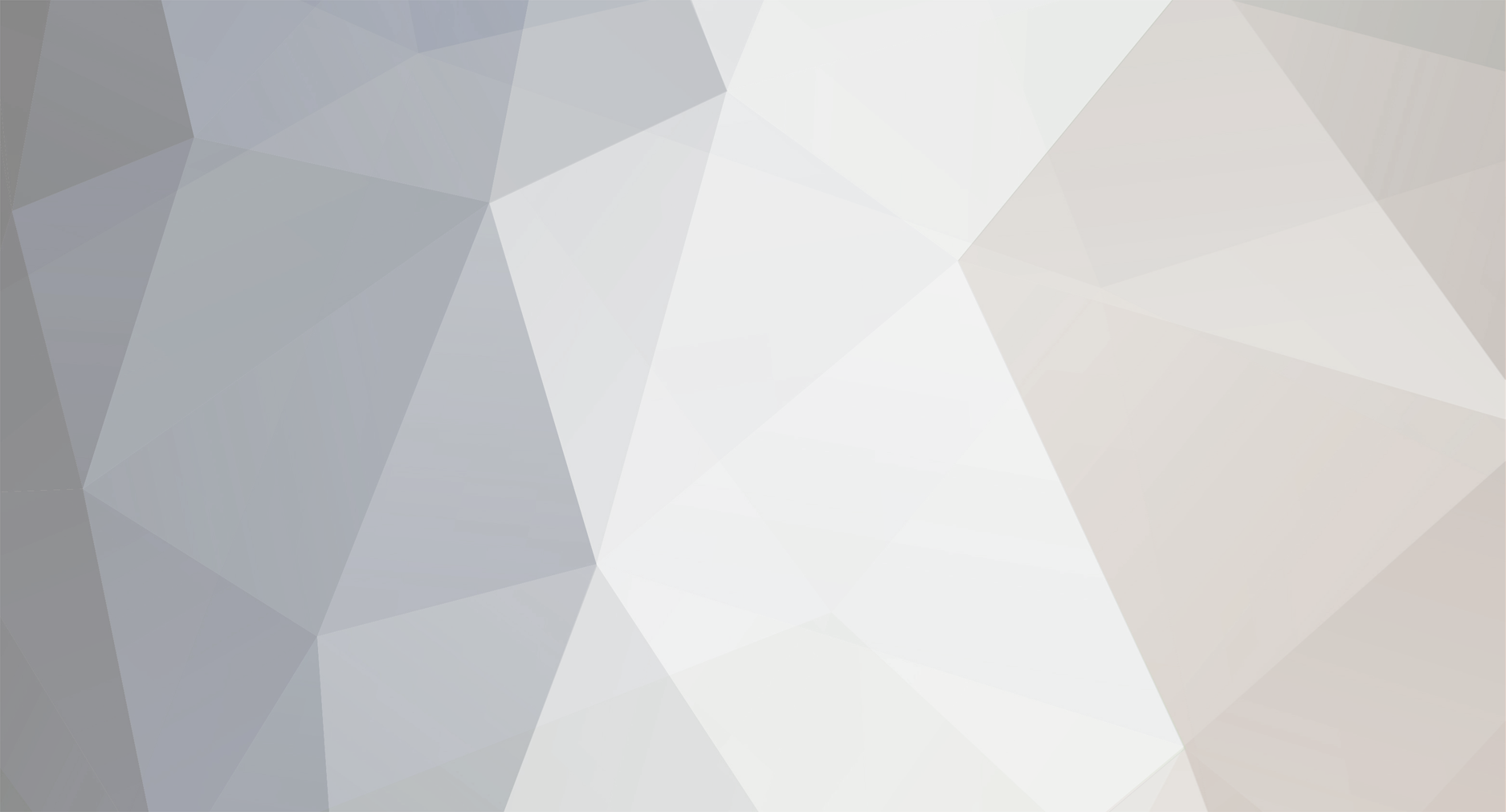
wazza91
-
Posts
12 -
Joined
-
Last visited
Never
Posts posted by wazza91
-
-
thanks but there is nothing showing up in the dropdown menu.
-
hi i have this code here and i was wondering how i could make it print out the values from my database using a drop down menu.
i no this is probably very basic but im a real novice at php and sql.
<?php
mysql_connect('localhost', 'root', '');
mysql_select_db('charity_data');
$result = mysql_query('select * from donations');
?>
<select name="selectname">
<?php
$i = 0;
while ($i < mysql_num_fields($result)){
$fieldname = mysql_field_name($result, $i);
echo '<option value="'.$fieldname.'">'.$fieldname.'</option>';
$i++;
}
?>
</select>
-
okay this is what i got someone plz help
<?php
$output = "";
$total = 0;
for($i = 1; $i <= 7; $i++) {
$people = array(array(21, "John"), array(25, "Bill"), array(23, "Anna"),
array(29, "Aine"), array(19, "Pat"), array(30 , "Leanne") );
print "<table border=1>";
print "<tr><th>Age</th><th>Name</th></tr>";
for($x=0;$x<6;$x=$x+1) {
print "<tr><td>" . $people[$x][0] . "</td>";
print "<td>" . $people[$x][1] . "</td></tr>";
}
?>
-
this is what i got at the minute am i on the right lines?
<?php $output = ""; $total = 0; for($i = 1; $i <= 7; $i++) { $employee = array(array(21, "1employee"), array(25, "2employee"), array(23, "3employee"), array(29, "4employee"), array(19, "5employee"), array(30 , "6employee") array(32 ,"7employee") ); print "Whole Table<br>"; ); ?>
-
any one have any ideas on what functions, i need to use and how i would write the code!!!!
-
how would i go about the task?
Create a php script to
- receive the chosen option from the select box & textbox in the html file & check it is within range and is a number (using the is_numeric( ) function)
- Assign 7 employee records (Employee Number, Employee Name, Age, Department Name) into a 2 dimensional sequential array (using employee numbers 1 to 7) and your own made up values for the other record details – remember that text values are enclosed within inverted commas whereas numeric values are not:
- See PHP worksheet 3 for how a 2D array is created in a program
- If the chosen select option is to print the whole table
o – then print all 7 records in an html table with a header row
• You will need a for loop to print a record
• You will need to manipulate array values
(You should use a variable for the row number to
generalise row printing a row using the for loop)
- If the chosen option is to print a selected record, use the record number from the textbox to
o Print that particular record including the header details row
• E.g. for employee number 1 by transposing it to the array row index number
• This row number should be generalised using a variable for the row index number
• Sample record
-
sorry if the seems a bit confusing heres a more detail description of the task.
Q1
a. Create an html file with a textbox to enter numbers 0 to 6 to be posted to a php file. The number represents a guess for how many times a particular sequence of letters (ABC) will be randomly created
b. Create a php file to generate a random 3 letter word whose letters are made up of A, B or C 50 times and check if the guess received from the html file is correct
1. receive the guess value and check that
- The value lies between 0 & 6 and is a number –(using the is_numeric( ) function)
2. create 3 random numbers (each either 1, 2 or 3) using a for loop and rand function
- check the value of each number as they are created (using an if statement) to assign the
letter A for 1, B for 2, C for 3 to a letterstring variable
- add the letter to a wordstring variable each time (using string concatenation with .
[e.g. $wholename = $firstname . “ “ . $surname] - analagous to the way of
creating a running total of numbers within a for loop using $total = $total + $number; )
ending up with a wordstring variable made up of 3 letters in random order
c. Now create 50 different word strings using a for loop around the code for b2.
- check the wordstring to see if “ABC” has been generated
[ since it is random there are 27 different combinations of 3 letters that
can be created: AAA, AAB, AAC,ABA, ABB, ABC, BAC,BBA,BBB,BBC,BCA,BCB,
ACA,ACB,ACC,BAA,BAB, BCC,CAA,CAB,CAC,CBA,CBB,CBC,CCA,CCB,CCC ]
- if “ABC” is created print “Found ABC string” & increment a count for ABC strings
- print the guess value and the number of times the “ABC” sequence occurs
- compare the guess with the actual number of times the “ABC” sequence occurs
- print either “You have guessed correctly” or “Incorrect guess – try again!”
depending on whether the guess matches the ABC count or not
-
ok fair enough, but what is the function i need to use for the ABC count
-
thanks very much Nightslyr, there a second part you couldn't help me with.
Now generate 50 words
For loop (50)
For loop (3)
Generate a number between 1 & 3
If 1 then assign A, If 2 then assign B, If 3 then assign
C
Add to a wordstring
End loop
Check if word is ABC
If so increment ABC count
End Loop
Print ABC Count
-
i have been given this task in in uni. i was wondering it some one would be so kind to help me with it. all i have so far is this
<?php
for($x=1; $x<=3; $x=$x+1) {
print "$x <br>";
}
if ($x== "A") {
}
?>
the task is below
Generate a number between 1 & 3
If 1 then assign A, If 2 then assign B, If 3 then assign C
Add to a wordstring (using string concatenation with .)
We need 3 letters generated to give a 3 letter word
For loop (3)
Generate a number between 1 & 3
If 1 then assign A, If 2 then assign B, If 3 then assign
C
Add to a wordstring (string concatenation with .)
End loop
Print 3 letter word
insert query-help
in PHP Coding Help
Posted
hi im trying to do a insert query but i keep getting the error messeage ? mysql_error() expects parameter 1 to be resource, object given in /Users/garykane/Sites/update2.php on line 6
success in database entry.-1 row inserted?
here is my code
<?php
$con = mysqli_connect("localhost", "root", "") or die ("No connection"); /*connect to server*/ mysqli_select_db($con ,"Declan") or die ("db will not open"); /*connect to database*/ $dno = $_POST["dno"];
$dname = $_POST["dname"];
$location = $_POST["location"];
$query="Update INTO AccountNumber VALUES (" .$dno . ", '" . $dname . "','" . $location . "')"; if(!mysqli_query($con, $query)) echo mysql_error($con);
echo "success in database entry.";
$numrows = mysqli_affected_rows($con);
echo $numrows . " row inserted<br>";
mysqli_close($con);
?>
here is the front end texbox code
<html>
<head>
<title>Question 2, Accounts</title>
</head>
<h1>Enter Details</h1>
<body>
<form action="update2.php" method="post">
Account Number : <input type="text" name="dno" /><br />
Balance : <input type="text" name="dname" /><br />
Customer Name : <input type="text" name="location" /><br />
<input type="submit" name="submit" value="submit" />
</form>
<?php
$con = mysqli_connect("localhost", "root", "") or die ("No connection"); /*connect to server*/
mysqli_select_db($con ,"Declan") or die ("db will not open"); /*connect to database*/
?>
</body>
</html>