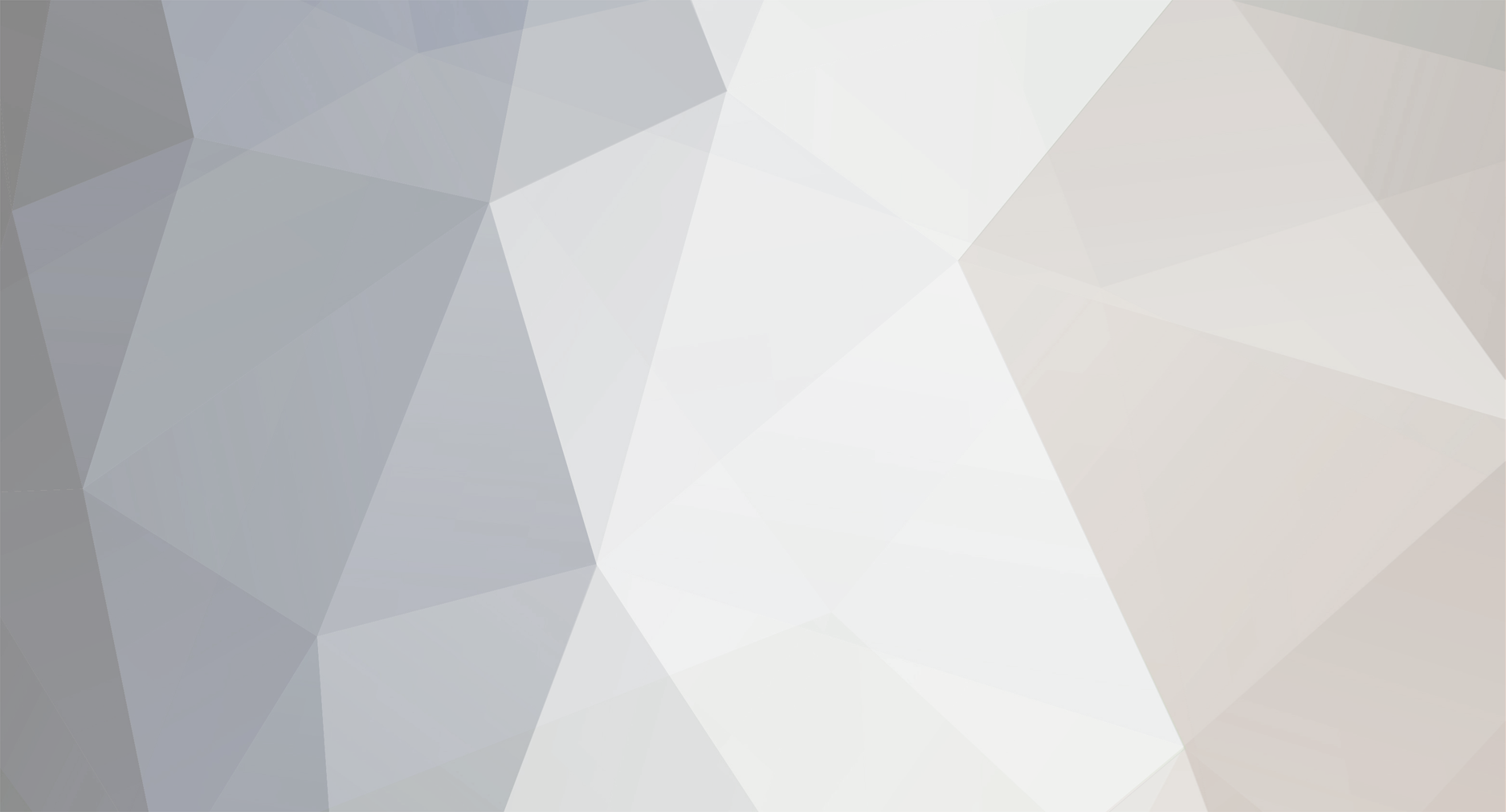
fporter
-
Posts
1 -
Joined
-
Last visited
Never
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.
HTML Form that pulls MYSQL DB with PHP
in PHP Coding Help
Posted
Trying to figure out how to approach this. I have a form that uses php to pull from a mysql database. I can get the text submission form to work but I am having trouble with a drop down menu that pulls directly from the DB and submitting what is selected as the basis of the result query. I am including the two sets of code. one for my search.php and the other for my search_result.php.
search.php
search_result.php