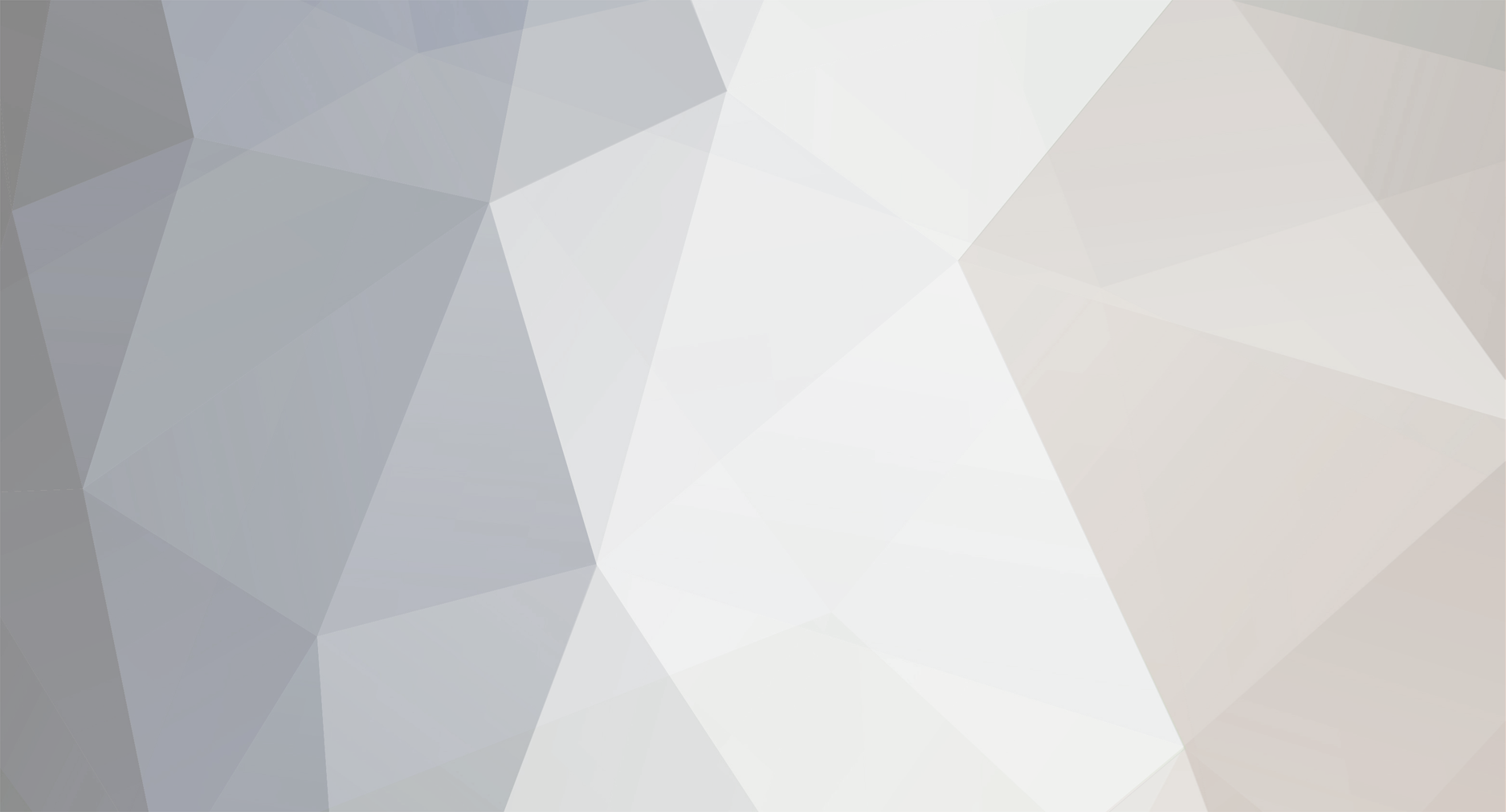
HDFilmMaker2112
Members-
Posts
547 -
Joined
-
Last visited
Never
Everything posted by HDFilmMaker2112
-
Okay, well I got it to the following. It doesn't show any error notices, but it also doesn't display anything. class menu{ public function __construct($array1, $index1, $array2, $index2, $select = null){ if(isset($index2) && $index2!=null){ // logic to determine which menu to use if(isset($_SESSION['username']) && $_SESSION['username']!=null){ $this->menu_array[$this->index1] = $array1; } else{ $this->menu_array[$this->index2] = $array2; } } else{ $this->menu_array[$this->index1] = $array1; } //Get Text for Links from Keys of Array $menu_text = array_keys($this->menu_array); // produce and output the correct menu $i=0; if(isset($select)){ $newmenu="<select>"; } foreach(array_values($this->menu_array) as $link_option){ $newmenu.=$this->menu; $i++; } if(isset($select)){ $newmenu.="</select>"; } } public function generateTopMenu(){ $this->menu='<a href="'.$link_option.'">'; $this->menu.=$menu_text[$i]; $this->menu.='</a> '; } public function generateSearchMenu(){ $this->menu.='<option value="'.$search_text[$i].'" >'.$search_item.'</option>'."\n"; } public function returnMenu(){ return $newmenu; } } //Generate menu array $menu_array = array(); $menu_array['loggedin'] = array("Home" => "/home","News Feed" => "/newsfeed","Notifications" => "/notifications","Requests" => "/requests", "Messages" => "/messages"); $menu_array['loggedout'] = array("Music" => "/music","Movies" => "/movies","Television" => "/television","Games" => "/games", "Videos" => "/videos", "Browse People" => "/people"); $toplinks = new menu($menu_array['loggedin'], "loggedin", $menu_array['loggedout'], "loggedout"); $toplinks->generateTopMenu(); echo $toplinks->returnMenu();
-
I'm trying to make a class for my menu generation. One generates a row of links, the other generates a drop down menu, but their cores are pretty much identical, so I figure I could condense these into a single class with two functions, a construct, and return function. class menu{ public function __construct($array1, $index1, $array2, $index2, $select = null){ if(isset($index2) && $index2!=null){ // logic to determine which menu to use if(isset($_SESSION['username']) && $_SESSION['username']!=null){ $this->type = $index1; $this->menu_array = $array1; } else{ $this->type = $index2; $this->menu_array = $array2; } } else{ $this->type = $index1; $this->menu_array = $array1; } //Get Text for Links from Keys of Array $menu_text = array_keys($this->menu_array[$this->type]); // produce and output the correct menu $i=0; if(isset($select)){ $newmenu="<select>"; } foreach(array_values($menu_array[$type]) as $link_option){ $newmenu.=$this->menu; $i++; } if(isset($select)){ $newmenu.="</select>"; } } public function generateTopMenu(){ $this->menu='<a href="'.$link_option.'">'; $this->menu.=$menu_text[$i]; $this->menu.='</a> '; } public function generateSearchMenu(){ $this->menu.='<option value="'.$search_text[$i].'" >'.$search_item.'</option>'."\n"; } public function returnMenu(){ return $newmenu; } //Generate menu array $menu_array = array(); $menu_array['loggedin'] = array("Home" => "/home","News Feed" => "/newsfeed","Notifications" => "/notifications","Requests" => "/requests", "Messages" => "/messages"); $menu_array['loggedout'] = array("Music" => "/music","Movies" => "/movies","Television" => "/television","Games" => "/games", "Videos" => "/videos", "Browse People" => "/people"); $toplinks = new menu($menu_array['loggedin'], "loggedin", $menu_array['loggedout'], "loggedout"); $toplinks->generateTopMenu(); echo $toplinks->returnMenu(); I'm sure there's a way to make this class simpler, I just don't know it. This is currently throwing the following error notices: Warning: Illegal offset type in /homepages/27/d418565624/htdocs/class.php on line 118 Warning: array_keys() expects parameter 1 to be array, string given in /homepages/27/d418565624/htdocs/class.php on line 118 Warning: array_values() expects parameter 1 to be array, null given in /homepages/27/d418565624/htdocs/class.php on line 125 Warning: Invalid argument supplied for foreach() in /homepages/27/d418565624/htdocs/class.php on line 125 Ad
-
Shouldn't be a issue, but try placing the function before it's called. Generally you should be able to place a function anywhere and have it work, but worth a try.
-
That should really be phrased as no style sheets, javascript or HTML should be before your HTML tag. PHP is frequently, and most usually SHOULD be before any HTML. CSS, and Javascript (unless its absolutely necessary to place it somewhere else), should be inside the head tag. And it is of course better to get all CSS, and as much Javascript and PHP as possible out of the actual documents that contain HTML. Where PHP is necessary in HTML is to format the display with conditionals.
-
How to order MySQL data from multiple queries?
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
This is a basic process when dealing with retrieving associated records in a one-to-many configuration. The solution is to simply create a "flag" variable to detect a change in the root record within the while loop. The flag variable needs to track a value that will be unique for that root record. In this case you could possibly rely upon the status message. But, that's a poor solution since you probably don't require the status updates to be unique. The possibility of two users having the same status update back to back is small, but why rely upon that when you can use a better value - the status update ID? So, you would need to add the status ID to the result set in the query. NOTE: I just realized you will need a LEFT JOIN on the comment table since there will not always be a comment. Example: $current_status_id = false; while($row = mysql_fetch_assoc($result)) { //Detect if this status is different than the last if($current_status_id != $row['status_id']) { //Set the new flag value to suppress output for //subsequent comments on the same status $current_status_id = $row['status_id']; //Display the status info for 1st record assoc. w/ the status echo "<br><b>{$row['status_name']} {$row['status_time']}</b><br>\n"; echo "{$row['message']}<br>\n"; } //Display the comments //Need a condition to cover scenario where no comment exists if($row['comment'] != '') { echo "<br><b>{$row['comment_name']} {$row['comment_time']}</b><br>\n"; echo "{$row['comment']}<br>\n"; } } That's pretty damn clever. Your not based in or around New York are you? Cause I'd definitely hire you as one of the lead programmers once we get investment backing. This is definitely the most complicated thing I've done to date. Anyway, thank you for the help. -
How to order MySQL data from multiple queries?
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
So, if I am reading that correctly, I would build out the query starting with the status updates. I don't know if this is more efficient or not, but give it a try if you want. I think it is more organized. FYI: Your query wasn't pulling the comments - which I thought you wanted. SELECT status.message, status.time_posted AS status_time, status_details.name AS status_name comment.comment, comment.time_posted AS comment_time commenter_details.name AS commenter_name FROM status_updates AS status -- Pull data from status table JOIN user_details AS status_details -- Join user table to status records ON status_details.user_id = status.user_id -- to get user info of poster JOIN comment AS comment -- Join comment table to status records ON comment.status_id = comment.id -- to get associated comments JOIN user_details AS commenter_details -- Join user table to comment records ON commenter_details.user_id = status.user_id -- to get user info of commenter LEFT JOIN subscribers AS subscribe -- Conditionally JOIN subscriber table ON subscribe.user_id = status.user_id -- to status table ONLY where the AND subscribe.subscriber_id = 000000000003 -- the subscriber is the current user WHERE status.user_id = 000000000003 -- Filter out all records except those where OR subscribe.subscriber_id = 000000000003 -- the status poster is the current user or -- where the current user is a subscriber ORDER BY status.time_posted DESC That does look like a good option too. Now one thing I'm curious about. Since I'm pulling all the comments together with the status_update. The rows returned all have the status update message attached to them. So how would I increment through the comments to show with the status update and only show the status update once. Doing a simple while loop would cause me to have repeated status updates with no more than one comment each. Should I just append [$i] to the specific $row variable and do the comments separately in a for loop? How would I prevent the duplicate status updates though? -
How to order MySQL data from multiple queries?
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
I've seemed to have gotten something that works. SELECT status.message , status.time_posted AS status_time , status_details.name AS status_name , commenter_details.name AS commenter_name FROM subscribers AS subscribe INNER JOIN status_updates AS status ON status.user_id = subscribe.subscriber_id INNER JOIN user_details AS status_details ON status_details.user_id = status.user_id INNER JOIN comments ON comments.status_id = status.id INNER JOIN user_details AS commenter_details ON commenter_details.user_id = comments.user_id WHERE subscribe.subscriber_id=000000000003 ORDER BY status.time_posted DESC -
How to order MySQL data from multiple queries?
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
Alright I modified what I had prior because I also need to pull the users name and url from the user_details table. This query runs, but returns no results: SELECT details.name, subscribe.subscriber_id, status.message, status.time_posted AS status_time FROM status_updates AS status JOIN subscribers AS subscribe ON status.user_id=subscribe.subscriber_id INNER JOIN comments ON status.id=comments.status_id INNER JOIN user_details AS details ON details.user_id=status.user_id AND details.user_id=comments.user_id WHERE subscribe.user_id=000000000002 ORDER BY status.time_posted DESC -
So the page looks for a submission via that input type="image" right? Why not have the form post to self (with the $_SERVER['php_self']) and place the redirect if statement prior to the HTML? Maybe I'm understanding the the issue here though. <?php $email = $_GET['paypal_email']; if(@$_GET['continue'] == "Submit") { $email = $_POST['paypal_email']; header('Location: http://mysite.com/start.php?offer_pgid=071691&email=$email'); } ?> <form action="<? echo $_SERVER['PHP_SELF']; ?>" method="get"> <p><input type="text" style=" width:230px; height:60px; border:2px dashed #D1C7AC; font-size:18px;" name="paypal_email"></p> <p> </p> <input type="image" name="continue" src="images/arw_submit.png" alt="submit" value="Submit"></form></div>
-
How to order MySQL data from multiple queries?
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
Alright, here's what I came up with. It works, but I'm not sure if it works right. SELECT comments.comment, comments.user_id, subscribe.subscriber_id, subscribe.user_id, status.message, status.time_posted FROM status_updates AS status JOIN subscribers AS subscribe ON status.user_id=subscribe.subscriber_id INNER JOIN comments ON status.id=comments.status_id WHERE subscribe.user_id=000000000002 ORDER BY status.time_posted DESC It works, but I can't tell if it's actually pulling the right data. It should be seeing the logged in user (in this case user # 000000000002), status updates for the people their subscribed to in the subscribed table, and then ALL comments that are related to those status updates (including people they're not subscribed to). The tables are currently as follows: subscribers id | user_id | subscriber_id | subscribe_date 1 | 000000000001 | 0000000000002 | 2 | 000000000001 | 0000000000003 | 3 | 000000000001 | 0000000000001 | 4 | 000000000002 | 0000000000003 | 1 | 000000000004 | 0000000000001 | status_updates id | user_id | message | time_posted 1 | 000000000002 | Test Message One | 2012-06-13 00:22:37 2 | 000000000003 | Test Message Two | 2012-06-13 00:22:37 comment id | user_id | comment | time_posted | status_id 1 | 000000000001 | Comment 1 | 2012-06-13 00:23:43 | 1 2 | 000000000003 | Comment Two | 2012-06-13 00:24:33 | 1 3 | 000000000001 | Comment Three | 2012-06-13 00:24:33 | 2 4 | 000000000001 | Comment Four | 2012-06-13 00:24:57 | 2 5 | 000000000001 | Comment Five | 2012-06-13 00:59:23 | 2 When I run the above query I end up with this as the result: comment | user_id | subscriber_id | user_id | message | time_posted Comment Three | 000000000002 | 000000000003 | 000000000002 | Test Message Two | 2012-06-13 00:22:37 Comment Four | 000000000001 | 000000000003 | 000000000002 | Test Message Two | 2012-06-13 00:22:37 Comment 5 | 000000000004 | 000000000003 | 000000000002 | Test Message Two | 2012-06-13 00:22:37 I think what's messing me up, is; should the logged in user viewing the page be queried as the subscriber or the user in the subscriber table. Because technically the user logged in would be the one subscribed to the other user; so they should be the subscriber_id correct? So right now, it's backwards. -
How to order MySQL data from multiple queries?
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
If I go with the subscriber, would I even need to query the friends table? The subscriber table should contain all the information I need on which users status updates to pull. -
That just seems like it adds so much overhead to something that should be so simple. You have to add another file in, additional processing, etc. I just don't see what advantage that has over the regular mail() function.
-
Crap, thank you. Can't believe I missed that.
-
How would I go about sending HTML through the mail() function? I'm currently using <br/> tags <a> tags and when I run the script and have the email sent to me, all the tags are visible. /*Generate activation email*/ $to=$register_email; $subject='Activate Your Kynxin Membership'; $headers='From: support@kynxin.com'; $message='Thank you for registering for Kynxin.<br/> <br/> Please visit the following link to activate your account. <a href="http://www.kynxin.com/signup/activate.knxn?activate='.$activation_number.'"> http://www.kynxin.com/signup/activate.knxn?activate='.$activation_number.'</a> <br/> <br/> Thank You,<br/> The Kynxin Support Team '; /*Send registration activation email*/ mail($to, $subject, $message, $headers);
-
Bizarre Issue with mod_rewrite or something else
HDFilmMaker2112 replied to HDFilmMaker2112's topic in Apache HTTP Server
I unfortunately only want to rewrite specific URLs so something that's does everything won't work. Which I'm not mistaken that does? -
I'm having a bizarre issue with Mod_Rewrite. When I type mydomain.com/signup into my browser it's going to signup.php not index.php?signup as expected. It seems my server is first looking for a file that has file name of signup. I noticed this earlier too because I have a file called signup.css, and it was loading that when I went to /signup; but didn't think much of it at the time. RewriteEngine on RewriteBase / RewriteRule ^signup$ index.php?signup RewriteRule ^signup/(?:$|\?(?:.+)) index.php?signup$1 [QSA] RewriteRule ^activation/(?:$|\?(?:.+)) activate.php?$1 [QSA] AddType x-mapp-php6 .php Any ideas on what's happening here?
-
How to order MySQL data from multiple queries?
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
The reason for duplicate entries would be the subscribed column. Users could be friends but not necessarily be subscribed to their status updates. I guess I could make another table to check whether the user is subscribed or not; but then I'd have another table to JOIN. a subscribed table would look like this: id | user_id | subscribed_id With the subscribed_id being the id of the user their subscribed to. This table would be a one way relationship. So I'd have to JOIN the new table, and then add something to the WHERE clause (probably an AND?) to make sure only the users status updates the person is subscribed to are shown. -
How to order MySQL data from multiple queries?
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
Never mind my last post; since I'm adding other features I'm going to have to redundant entries. id | user_id | friend_id 1 | 0001 | 0002 2 | 0001 | 0003 3 | 0002 | 0003 Will actually need to be: id | user_id | friend_id | is_friend | subscribed 1 | 0001 | 0002 | yes | yes 2 | 0002 | 0001 | yes | yes 3 | 0001 | 0003 | yes | no 4 | 0003 | 0001 | yes | yes 5 | 0002 | 0003 | no | yes 6 | 0003 | 0002 | no | no -
How to order MySQL data from multiple queries?
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
Bit of an extension on my last question. If I structure the friends table as follows: id | user_id | friend_id 1 | 0001 | 0002 2 | 0001 | 0003 3 | 0002 | 0003 How would I alter the above query to pull friendships from both columns. As you can see if I only query the user_id column and user 0002 is logged in, they'll only see the status updates of user 0003, but they should see 0003 and 0001. And user 0003 won't see updates from anybody. -
True story. Listen to this man. I tried using this but it sent the browser into a indefinite loop and eventually ended in a browser alert saying something along the lines of "This page is trying to redirect in a way that will never load.". It would work fine for URLs that have the question mark in them, but I'd end up with the alert on pages that didn't. So index.php?signup would redirect to /signup, but /signup would never actually load because it lacked the question mark. Or atleast that's what it seemed like was going. This is what I was using: if(isset($_SERVER['QUERY_STRING'])){ $key=$_SERVER['QUERY_STRING']; } Using the method you posted I have no issues what so ever.
-
How to order MySQL data from multiple queries?
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
Never, EVER run queries in loops. Do some research on how to properly query using JOINs. I know how to use joins, I just couldn't get my head around how to format a single query that would pull every single status update for every single friend_id that is tied to the logged in user. Thought I didn't really have any other choice. -
Works perfect.
-
I was thinking a locked table on the database. Are you using MyISAM or InnoDB for the DB Engine?
-
okay, I'll have to live with it then. It's because I'm using Mod_Rewrite on a few on my URLs. For example domain.com/index.php?signup is accessible as domain.com/signup, but if you type index.php?signup in the address bar it still works. So I'm catching stuff after the "?" as $key and then comparing it to see if $key is equal to the values that I've rewritten with mod_rewrite, and if it is redirecting to the rewritten url. It's included in function.php which I call on some pages that don't have a ? in the URL so it throws a notice on those pages apparently.