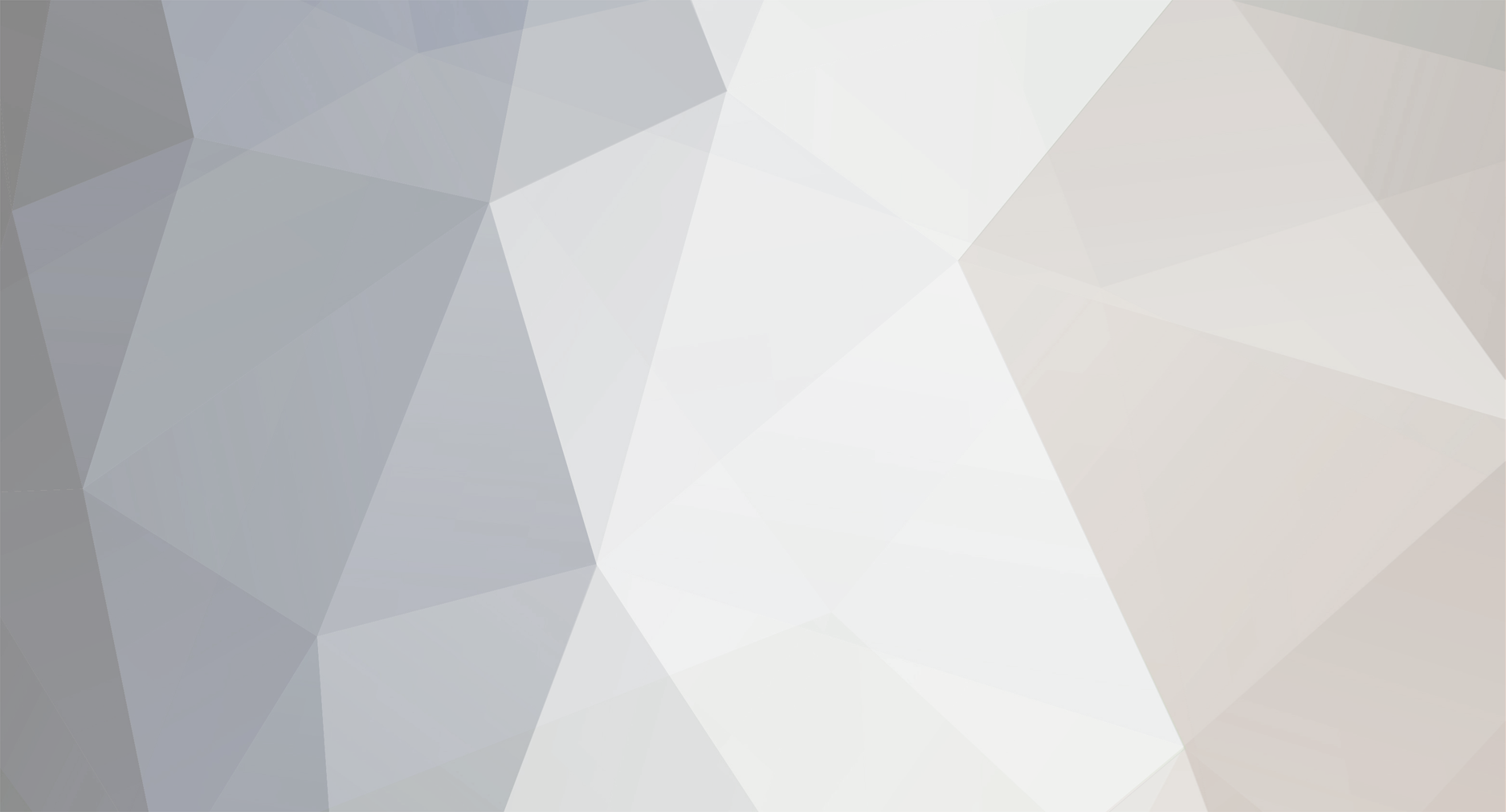
loudog
-
Posts
33 -
Joined
-
Last visited
Never
Posts posted by loudog
-
-
that's a good idea but wouldn't that be cheating?? Im a newbie and i have a list of per set functions that i want to rewrite. Im doing this cuz it will help me better understand and know that tool's i have to my disposal. Is there any other way??
-
hey guy's. Im trying to write my own function for array_udiff_assoc. So i need to compare two arrays in the same function and i was thinking that the best way would be to go element by element in other words compare each individual key to each other. for example compare key1 or array to key one of array2. My question is how can i compare each key of the array to each other?? Does it make sense??
$a1=array(1=>"Dog",2=>"Cat",3=>"Horse");
$a2=array(1=>"Cat",2=>"Horse",3=>"Dog");
-
Its the array_flip is done and Thank you guys for helping me.......I love this site.
-
Alright guy's, I think just got me into a situation here. I was able to flip them around with out using the array_flip function but here is the situation. i get both array's the regular and the flip one now i just don't know how to get rid of the regular one and just keep the array that i flip. :-\ Here is the code.
thanks in advance.
<?php
function dz_flip($temp){ /*$arr=''; for($i=0; $i>$temp; $i--){ $arr.=$temp[$i]; }*/ foreach($temp as $key => $value){ $temp[$value]=$key; } return $temp; The output is this Array ( [horse] => 1 [cat] => 2 [dog] => 3 [1] => horse [2] => cat [3] => dog ) } $temp=array("horse"=>1,"cat"=>2,"dog"=>3); print_r (dz_flip($temp)); //print_r(array_flip($temp)); ?>
-
I need to do this cuz is part of assignment. So im going to try my darnest to do it if not i'll be back here begging for help. :'(
-
hey guy's
Im having trouble with writing my own function for array_flip. What im trying to get the same output that the original array_flip does but im not sure if im on the right direction. Here is what i got so far. Plz keep in mind im a newbie.
thanks in advance.
<?php function dz_flip($trans){ $temp=array("a"=>0,"b"=>1,"c"=>2); $arr=''; for($i=-1; $i<$temp; $i--){ $arr.=$temp[$i]; $temp=array_flip($trans); } return $temp; }
-
Hey guy's, I got an assignment that made hit a roadblock in my learning process of PHP. I don't want you to do it for me but instead help me understand it better so i can do it on my own.
Im willing to pay between $12 to $16 the hour maybe a little more(I am a student). This isn't like a big job it more like once a week kind of thing,easy money in your pocket just to tutor me.
-
That is the hardest thing about all this, i am still not very familiar with loops and functions. so what is the best way to learn how to use them and when to use them?? btw that last comment u made gizmola really took some weight off me. I was trying to feel like i was biting more than i could chew and was going to choke to death .thanks bro for reminding me that this is learning process.
-
function my_str_pad($input, $length, $padchar = ' ', $location = STR_PAD_RIGHT) {
// now you need to start coding it.
$inputLen = strlen($input);
$padsize = $length - $inputLen;
if ($padsize > 0) {
switch ($location) {
case STR_PAD_BOTH :
break;
case STR_PAD_LEFT :
break;
default:
// STR_PAD_RIGHT
$repeat = ceil($padsize / strlen($padchar));
$t = $input;
for ($x=0; $x < $repeat; $x++)
$t .= $padchar;
}
return substr($t, 0, $length);
} else {
return $input;
}
function my_str_pad($input, $length, $padchar = ' ', $location = STR_PAD_RIGHT) {// now you need to start coding it.
$inputLen = strlen($input);
$padsize = $length - $inputLen;
if ($padsize > 0) {
switch ($location) {
case STR_PAD_BOTH :
break;
case STR_PAD_LEFT :
break;
default:
// STR_PAD_RIGHT
$repeat = ceil($padsize / strlen($padchar));
$t = $input;
for ($x=0; $x < $repeat; $x++)
$t .= $padchar;
}
return substr($t, 0, $length);
} else {
return $input;
}
}
That should be a good start for you. As you can see i threw in one of the 3 cases, albeit probbly the easiest one. It also occurs to me that there is a better way to handle the padding string -- figure that out seperately and concat it at the very end when you return it, would be better. At any rate, flesh it out, and you have an idea how to approach the rest of these. Fair warning, there could be problems but with this, it's all off the top of my head, but at least it should give you an idea how you should start approaching the process of coding these.
That's what im talking about gizmola. what you just did above in the quote section and how u just wrote that function even tho it was just of the top of your head and you are not sure it works That's what my problem is when i start the coding process. i get stuck i don't know if i should use the if, switch, or foreach statements like you did. how did u decide what statements to use? what process do take to know what kind of statements ur going to to be using when u begin the code or function?
-
that's the problem that idk what statement should i use. should i use the if,while, or foreach. im lost and the teacher won't except a reuse of the php net example even if i change the name or the variable. am i missing the point??
this is the php manual example
<?php
$input = "Alien";
echo str_pad($input, 10); // produces "Alien "
echo str_pad($input, 10, "-=", STR_PAD_LEFT); // produces "-=-=-Alien"
echo str_pad($input, 10, "_", STR_PAD_BOTH); // produces "__Alien___"
echo str_pad($input, 6 , "___"); // produces "Alien_"
?>
-
The funny thing is that in the internet course i took for php that was the project. we did the following things of course u still have to valid it them with php or javascript and inserting the values into my sql. If you don't know what to put for info you could always copy the reg and log in page from facebook.
1 registration page.
2 log in page
3 profile page.(In that page is where you upload you're pics)
4 add and delete friends page
5 changing ur password page.
-
its not so much the the function but what type of argument should i use that gets me confuse in this assignment. i don't know if i use and if statement,while loop,or , foreach. I look at the examples the they have in php manaul and it just makes me feel even more lost
:-\
-
hey guys, I just got an assignment which im having alot of trouble with and i was hoping that you guy's could help me.
The assignment is that i must write my own functions. I've read the php manual and yes i do understand how to write a function but im not very good at writing my own this is my first time and what makes it worst is that i can't copy someone else function i have to write my own.
In this assignment you will have to
rewrite some of the built-in functions. For example: If I ask you
rewrite the function strrev() (strrev reverses a given string),you can
do something like this:
function dan_strrev($input) {
$i = 0;
$myLen = 0;
while (true) {
if (isset($input[$i])) {
$myLen = $myLen + 1;
$i = $i + 1;
}
else {
break;
}
}
$temp = '';
for ($i = $myLen - 1; $i >= 0; $i--) {
$temp = $temp.$input[$i];
}
return $temp;
}
echo dan_strrev('Hello');
echo strrev('Hello');
So, you can see that both the built-in function strrev() as well as
the one we wrote (dan_strrev()) gives the same output.
Please do the same for the following built-in functions:
1).str_pad — Pad a string to a certain length with another string
2).str_split — Convert a string to an array
3).str_repeat — Repeat a string
4).array_combine — Creates an array by using one array for keys and
another for its values
5).array_flip — Exchanges all keys with their associated values in an array
6).array_pop — Pop the element off the end of array
7).array_udiff_assoc — Computes the difference of arrays with
additional index check, compares data by a callback function
.array_walk — Apply a user function to every member of an array
9).array_uintersect — Computes the intersection of arrays, compares
data by a callback function
10).usort — Sort an array by values using a user-defined comparison function
11).array_flip — Exchanges all keys with their associated values in an array
12).ksort — Sort an array by key
13).in_array — Checks if a value exists in an array
14).array_search — Searches the array for a given value and returns
the corresponding key if successful
15).array_merge — Merge one or more arrays
If you want to know how these functions,please take a look at the php
manual/documentation on php.net.
Email me if you have not understood anything that I have explained so
far. I am not setting a deadline but it will be good if you can
complete this in the next 2 weeks
-
wow.. simple answers like that make me feel so dumb for asking questions like that .lol I guess that's the process you go thru to learn valuable skill in life. Like they once told me your not nosy you will never learn.lol
ps....thanks to everyone in advance for having such patience with noob like me.
-
Hey guy's I want you to write custom php functions which behaves like the
built-in ones. What is the best way to learn how to write you're own functions??
-
hey guys, I wanted to learn php, so i took a php course over the internet that lasted about a month. In that course i learned basic html,basic javascript, php, and basic ajax. I still consider myself very new to all this stuff, although been that the internet course was my first ever dive into programming of any kind. Not to mention that its been about 2 months since the class ended. i did learn how create forms and valid it them using javascript and php. I also learned how to insert data and retrieve data from mysql's database. All that i mentioned above is something that i now know how to do. Although there is alot more that he taught me and i still have the class files that i constantly keep learning it and going over to try remember stuff .
I was wondering how long before i can start applying for entry level jobs or interships?? :shy
-
Hey gristoi, i really appreciate ur patience and the fact that your taking the time out of your schedule to help me with this issue. But like you said, i think i was missing the point by trying to go around the fact that i needed an isp address something i don't have. so i think im just gonna purchse some hosting form go daddy and be done
ps.... the internet stuff your right. Im moving out in a week so there is no point in changing my actual internet to wireless.
-
i use wamp and is under localhost.
-
i don't have a host like godaddy or anything like that. all i have been trying is to configure the php.ini file tosend mail. Im starting to get to the point where i feel like unless i know the isp address there is no use to even configure that file. In other words i have to get a server from go daddy or somthing. RIght?
-
I don't have internet i use wireless thru someone else in my apartment complex cuz its an unlocked connection. honestly i don't even know who it belongs to i just know that his internet provider is qwest. so i did the following and i still got the error.
[mail function]
; For Win32 only.
SMTP = qwest
smtp_port = 25
; For Win32 only.
; http://php.net/sendmail-from
sendmail_from = dan@qwest.com
I have change the settings in the php,.ini file and i still get the damn error:Warning: mail() [function.mail]: Failed to connect to mailserver at "71.210.196.246" port 25, verify your "SMTP" and "smtp_port" setting in php.ini or use ini_set() in C:\wamp\www\GuestBook\mail.php on line 7
New settings
[mail function]
; For Win32 only.
SMTP = 71.210.196.246
smtp_port = 25
; For Win32 only.
; http://php.net/sendmail-from
sendmail_from = pea6n@yahoo.com
I still get the error
-
I don't have internet i use wireless thru someone else in my apartment complex cuz its an unlocked connection. honestly i don't even know who it belongs to i just know that his internet provider is qwest. so i did the following and i still got the error.
[mail function]
; For Win32 only.
SMTP = qwest
smtp_port = 25
; For Win32 only.
; http://php.net/sendmail-from
sendmail_from = dan@qwest.com
-
i have changed the mail function and i still get the error why??????
this is the new setting below.
[mail function]
; For Win32 only.
SMTP = localhost
smtp_port = 25
; For Win32 only.
; http://php.net/sendmail-from
sendmail_from = you@yourdomain
-
well that sound easy and i bet it is.
but would you mind telling me how do i find SMTP in the php.ini file and amend it as well. in other words a step by step guide on how to set up a mail server would be appreciated.
thanks in advance
loudog
-
Hey guy's, Im working on how to verify an email address. i dont have my own web site so i keep getting the following errors:Warning: mail() [function.mail]: Failed to connect to mailserver at "localhost" port 25, verify your "SMTP" and "smtp_port" setting in php.ini or use ini_set() in C:\wamp\www\GuestBook\signup_ac.php on line 40 and Undefined variable: sentmail in C:\wamp\www\GuestBook\signup_ac.php on line 49 . I've read that you can actually can don it without having you're on site. If anyone can show me how to do it or tell me what im doing wrong that would be awesome.
thanks in advance
loudog
HERE is the code.
page1
sginup.php
<html>
<head>
</head>
<body>
<table width="350" border="0" align="center" cellpadding="0" cellspacing="0">
<tr>
<td><form name="form1" method="post" action="signup_ac.php">
<table width="100%" border="0" cellspacing="4" cellpadding="0>
<tr>
<td colspan="3"><strong>Sign up</strong></td>
</tr>
<tr>
<td width="76">Name</td>
<td width="3">:</td>
<td width ="305"><input type="text" name="name" id="name" size="30"></td>
</tr>
<tr>
<td>E-mail</td>
<td>:</td>
<td><input type="text" name="email" id="email" size="30"></td>
</tr>
<tr>
<td>Password</td>
<td>:</td>
<td><input type="password" name="password" id="password" size="30"></td>
</tr>
<tr>
<td>Country</td>
<td>:</td>
<td><input type="country" name="country" id="country" size="30"></td>
</tr>
<tr>
<td> </td>
<td> </td>
<td><input type="submit" name="sub" id="sub" value="Submit">
<input type="reset" name="reset" id="reset" value="Reset"></td>
</tr>
</table>
</form></td>
</tr>
</table>
</body>
</html>
page 2
signup_ac.php
<?php
include('config.php');
//table name
$tbl_name='temp_members';
//Random confirmation code
$confirm_code=md5(uniqid(rand()));
//values sent from form
$name=$_POST['name'];
$email=$_POST['email'];
$country=$_POST['country'];
$password=$_POST['password'];
//Insert Data into database
//$sql="INSERT INTO $tbl_name(confrim_code,name,email,password,country)VALUES('$confirm_code','$name','$emailid','$pwd','$country')";
$sql="INSERT INTO $tbl_name(confirm_code, name, email, password, country)VALUES('$confirm_code', '$name', '$email', '$password', '$country')";
$result=mysql_query($sql);
//If suceesfully inserted data into database, send confirmation link to email
if($result)
{
//Send e-mail to..
$to=$email;
//Your subject
$subject="Your confirmation link here";
//From
$header="from:your name<your email>";
//Your message
$message="Your confirmation link \r\n";
$message="Click on this link to activate your account \r\n";
$message="http://www.yourweb.com/confirmation.php?passkey=$confirm_code";
//send email
this line 40->$sentemail=mail($to,$subject,$message,$header);
}
//If not found
else{
echo "Not found your email in our database";
}
//If your email succesfully sent
this is line 49->if($sentmail){
echo "Your Confirmation link Has Been Sent To Your Email Address.";
}
else{
echo"Cannot send Confirmation link to your email Address.";
}
?>
noob needs help......
in PHP Coding Help
Posted
hey guy's, Im trying to compare two array's to see it they have any common variables(i think that's what they are called) if they do have the function print out the common variable if they don't have the function print something saying they don't. Anyway, Im having trouble comparing the actual arrays this is what i have so far.
thanks in advance.