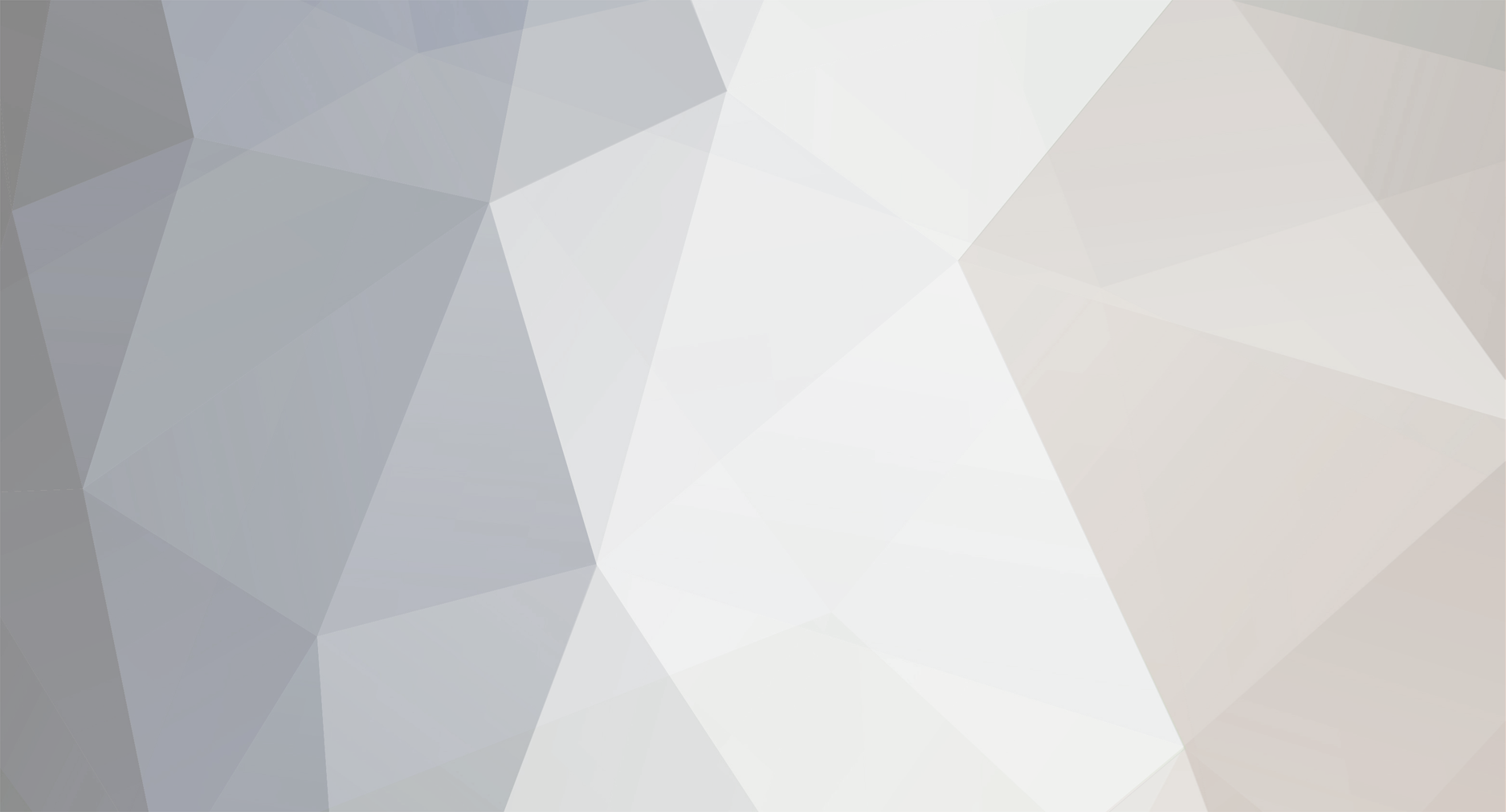
EdwinPaul
-
Posts
137 -
Joined
-
Last visited
Posts posted by EdwinPaul
-
-
Maybe batstanggt should also google 'sqlinjection' because this isn't safe. A username of 1' or '1=1 might give funny results...
-
First of all, the sequence of all the actions in a program (or php-file) is important. For instance: you can't use a session-variable if you didn't fill it. And you can't use a variable in a SELECT if the contents isn't filled. Besides that, there are a few rules ordered by php and html. For instance: if you put something to the screen ( echo 'something') and after that you code your header of the html, you get an error-message: 'headers allready sent'.
After you execute a query, the result comes in.. $result in your example. With this result you can fill an array of table-values with mysql_fetch_array($result). If you code:
$row=mysql_fetch_array($result) then the array with the name $row is filled with keys (the names of your database-fields) an values (from the corresponding fields). You can acces them with $row['fieldname'] and do with it whatever you want, including putting it in a session-variable.
-
I have an original price column and the new price column and wish to know how to ADD 3.4% to the new column.
can anyone suggest the formular for this ?
$a=150;
$b=$a*1.034;
-
is arithmetic ?
^^^ Inside a string it is not.
$date = "$year-$month"; and $date = $year.'-'.$month; produce exactly the same result.
Yes, I noticed. I removed it. :-\
-
why does my query return 0 if i do have events for this month.
// get the date from the URL or get the current date $month = isset($_GET['month']) ? sprintf('%02d',(int)$_GET['month']) : date('m'); $year = isset($_GET['year']) ? (int)$_GET['year'] : date('Y'); $date = "$year-$month"; // determine previous/next month (valid from 1970 (or 1901, depending on php version and operating system) through 2038 only) $previous = explode('-',date('Y-m',strtotime($date . '-1 -1 month'))); $next = explode('-',date('Y-m',strtotime($date . '-1 +1 month'))); // produce previous/next link $query = (empty($_GET)) ? array() : $_GET; // get an empty array if no existing $_GET parameters or get the current $_GET parameters $query['month'] = $previous[1]; // set or replace the month parameter $query['year'] = $previous[0]; // set or replace the year parameter $prev_link = "<a href='?" . http_build_query($query, '', '&') . "'><img src='prev_month.png' alt=''>Prev</a>"; $query['month'] = $next[1]; // set or replace the month parameter $query['year'] = $next[0]; // set or replace the year parameter $next_link = "<a href='?" . http_build_query($query, '', '&') . "'><img src='next_month.png' alt=''>Next</a>"; // output the links echo $prev_link . "" . $next_link; ?> <?php if(!($db = @ mysql_connect('localhost', 'username', '98098089'))) { echo 'Error: Could not connect to our database sorry for any inconvience.<br /> Please try at a later time.'; exit; } mysql_select_db("edinburg_site"); $actID= $_GET["actID"]; if(!isset($actID) && !isset($typeview)) { // $today = date('l, F jS, Y'); // $today2 = date('Y-m-j'); $actDate=$_GET["actDate"]; // $query="SELECT actID, actTitle, actBody, date_format(actDate, '%W, %M, %Y') actDate, file FROM activities WHERE actDate='$today2'"; $query="SELECT actID, actTitle, actTime, actPlace, actBody, date_format(actDate, '%Y %m') FROM activities WHERE actDate = '$date'"; print $query; $result=mysql_query($query);
Why don't you echo some intermediate results?
<?php $date = "$year-$month"; echo $date."<br/>"; echo date('Y-m',strtotime($date . '-1 -1 month'))."<br/>"; echo date('Y-m',strtotime($date . '-1 +1 month'))."<br/>"; ?>
-
First of all: you cannot use if($post_username && $post_password) as if it were 2 booleans. They are NOT !
Second:
<?php if (strlen($post_username) >25) || strlen($post_password) < 15)) ?>
<?php if ( (strlen($post_username) >25) || (strlen($post_password) < 15) ) ?>
-
Okay, too bad for your time spent.
I copied your script to my server, changed the to-address, and now I think I can see what's wrong. Attachments are added to you message, and separated by boundaries. Those boundaries should be unique for each attachment. Therefor you added a variable in your script, called $num, and you -correctly- integrated this in you boundary. But you don't use and/or increment this, so al your boundaries have the same name.
Furthermore: if you want to compose your own headers, each line you want to make should end with "\r\n" which means: 'go to the beginning of the next line'. http://php.net/manual/en/function.mail.php
All-in-all I would strongly recommend to switch to using one of the mentioned EASY
mailing-packages.
-
Where did you get this script?
-
According to the documentation, the syntax should be:
The AddAttachment function has four arguments:
AddAttachment(PATH_TO_FILE, FILENAME, ENCODING, HEADER_TYPE)
The PATH_TO_FILE is naturally the full path of the header you want to send. Application/octet-stream is default.
What is the contents of your variables $file1 and $file2 ?
-
I used PHPMailer now, but my attachments (.jpg pictures) get converted into .bin files and I don't understand why.
Can you show the code where that happens?
-
Lennart, to speed things up for yourself, consider using a package for sending mail: Libmail, PHPMailer, Swiftmail. And look at http://www.pfz.nl/forum/topic/455-s?do=findComment&comment=3247
-
if ($insert_program) { if ($insert_audience) {
are no booleans.
If $_POST['audience'] is your checkbox, I think it would be wise to check it with isset($_POST['audience']) and with is_array($_POST['audience'])
-
Another thing: some browsers do not send the contents of the submit-button whent the user hits enter, so it might be dangerous to test for the contents of that button. Also: $_REQUEST is an array which consists of everything in the $_GET, $_POST and $_COOKIE -arrays. You'd better check:
if ($_SERVER['REQUEST_METHOD'] == "POST"){
in stead of
if(isset($_REQUEST['submit']))
Also: try to find something on the internet about sql-injection. Your script is vulnerable!
If I enter a user like 1' OR '1=1 with the same pasword, I can enter your site.
-
If you remove the <div> </div> <p> and </p> from within your while-loop, things should go better. But really re-calculate the space !
-
On your site I can see that it is not working. I can also see in the source that you did not copy the part I was giving you.
Another consideration: Is the space where you want the picture + text wide enough for 2? I don't think so.
-
I checked it again, but I don't see what can be wrong. What is (not) happening?
-
$message = "This is a multi-part message in MIME format.\n\n" .
should be:
$message .= "This is a multi-part message in MIME format.\n\n" . // mark the extra dot before the = !!
-
From http://www.php.net/manual/en/features.file-upload.post-method.php :
You could use the $_FILES['userfile']['type'] variable to throw away any files that didn't match a certain type criteria, but use this only as first of a series of checks, because this value is completely under the control of the client and not checked on the PHP side.
-
It means you have to change
if ((($_FILES["file"]["type"] == "text/csv"))
to:
if ((($_FILES["file"]["type"] == "application/vnd.ms-excel"))
-
hey i tried putting those two lines at the top of the register.php file and now when I click register on my index.php file in the browser (which links to my register page). The register page wont load with that at the top. Anything else? I seem to be the only person online who can't figure out a login/register system.
Oh wait!
I just looked where the url usually is in the browser and it says that there is an undefined variable.
Here is the direct quote:
notice: Undefined variable: HTTP_SERVER_VARS in /var/www/register.php on line 126
Thanks guys for your help so far.
Change the next line:
<form action="<? echo $HTTP_SERVER_VARS['PHP_SELF']; ?>" method="post">
To:
<form action="" method="post">
-
Try this:
$showroom_query = mysql_query('SELECT * FROM `showroom` ORDER BY `showroom` ASC;'); if(mysql_num_rows($showroom_query) > 0) { $columns=2; $counter=1; while($showroom = mysql_fetch_array($showroom_query)) { echo '<a href="advanced_search.php?showroom='.$showroom['showroom_id'].'"><img width="243" height="42" src="images/showrooms/showroom_'.$showroom['showroom_id']'._1.gif" alt="">'.$showroom['showroom'].'</a>'; $counter++; if($counter>$columns) { echo '<br/>'; $counter=1; } } }else{ echo 'No results.'; } include_once "includes/footer.php";
-
I mean i want to display only non empty fields.
<tr> <?php if($row['accounts'] != ''){ echo "<td>".$row['accounts']."</td>"; } // but you will have a problem with the table-heading... ?> </tr> [code]
-
The correct way to check if a form is submitted is NOT:
// Check if form is submitted if(isset($_POST['login'])) {
Some browsers do not sent the submit-button if you hit enter. You can check this by displaying the array $_POST before the line mentioned above.
Better is:
if ($_SERVER['REQUEST_METHOD'] == 'POST' ){
-
If you put those two lines on top of your script, you may see more (error-)messages:
ini_set('display_errors',1); error_reporting(E_ALL | E_STRICT);
Login page just refreshes.
in PHP Coding Help
Posted
This code:
should be: