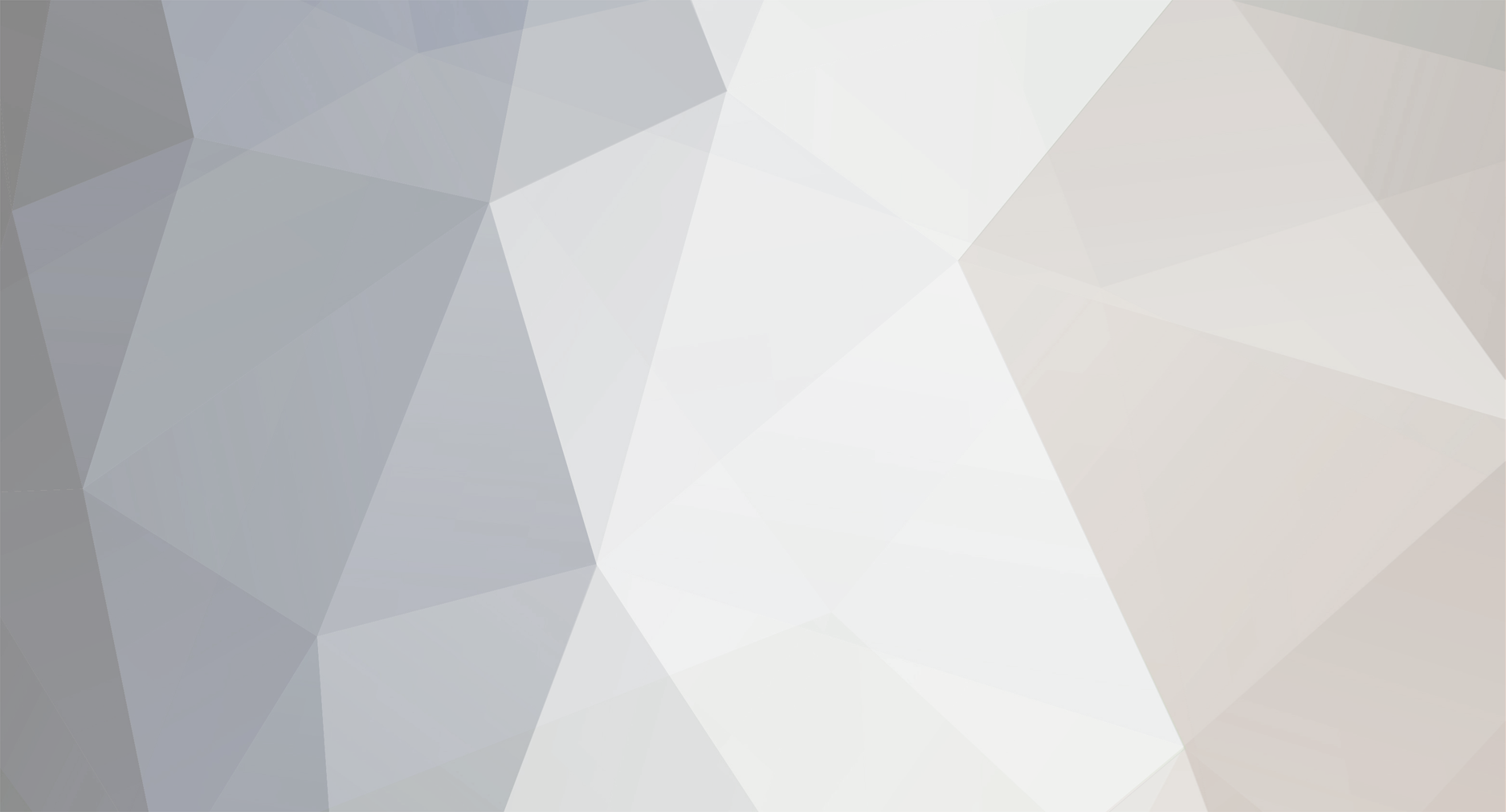
iRush
-
Posts
39 -
Joined
-
Last visited
Never
Posts posted by iRush
-
-
anyone have any ideas on how i would incorporate the table into my code
-
This is my code
<?PHP // please add login and pass here// $host = "localhost"; $login = "root" ; $pass = ""; mysql_connect("$host","$login","$pass") OR DIE ("There is a problem with the system. Please notify your system administrator." .mysql_error()); //Seems in this case we can use a general call $connection = mysql_connect("$host","$login","$pass") or die(mysql_error()); $dbs = @mysql_list_dbs($connection)or die(mysql_error()); $db_list="<ul>"; $i =0; while ($i < mysql_num_rows($dbs)){ $db_names[$i] = mysql_tablename($dbs,$i); $db_list .="<li>$db_names[$i]"; $i++; } //Start Create DB// IF (isset($_POST['result'])){ $database=$_POST['database']; $sql="CREATE DATABASE $database "; $result = mysql_query($sql,$connection) or die(mysql_error()); echo "Database $database has been added"; } IF (isset($_POST['delete'])){ $db=$_POST['db']; $query=mysql_query("DROP DATABASE $db"); echo "Database $db has been deleted"; } ?> <html> <head> <title>MySQL Databases</title> </head> <body> <p><strong>Databases on localhost</strong>:</p> <? echo "$db_list"; ?> <?PHP //print_r($_POST); ?> <form action="pretask.php" method="post"> <select name="db"> <?PHP $db_list = mysql_list_dbs($connection); while ($row = mysql_fetch_object($db_list)) { //Here you are listing anything that should not be included if ($row->Database!="information_schema" && $row->Database!="mysql" && $row->Database!="phpmyadmin"){ echo "<option value=\"".$row->Database."\">".$row->Database."</option>"; } } ?> </select> <input type="submit" name="delete" value="Delete"/> </form> <form action="pretask.php" method="post"> Create Database <input type="text" name="database" /> <input type="submit" name="result" value="Create" /> </form> </body>
-
So if i post the code i already have that has a list of databases and which also contains me being able to add and remove databases from a list can you help me out as to where i should implant the code so i can do it with a table instead?
-
I need some help on just making a plain and simple php table with rows and columns..then i need to beable to add in data from a text box form and submit button. So say i type in the text box test1 then it should put it in the table at row 1 column 1. Any ideas or help would be great to get me started
-
$member=$_POST['Name'];
That should be member inside instead of name im guessing
-
I just want to put this list into a table a simple list from my localhost into a table
-
Anyone have any ideas
-
Now that i have my code that shows my list on the localhost I want to try and put this into a table and beable to add a delete from the table. Here's my code any help would be much appreciated.
<?PHP // please add login and pass here// $host = "localhost"; $login = "root" ; $pass = ""; mysql_connect("$host","$login","$pass") OR DIE ("There is a problem with the system. Please notify your system administrator." .mysql_error()); //Seems in this case we can use a general call $connection = mysql_connect("$host","$login","$pass") or die(mysql_error()); $dbs = @mysql_list_dbs($connection)or die(mysql_error()); $db_list="<ul>"; $i =0; while ($i < mysql_num_rows($dbs)){ $db_names[$i] = mysql_tablename($dbs,$i); $db_list .="<li>$db_names[$i]"; $i++; } //Start Create DB// IF (isset($_POST['result'])){ $database=$_POST['database']; $sql="CREATE DATABASE $database "; $result = mysql_query($sql,$connection) or die(mysql_error()); echo "Database $database has been added"; } IF (isset($_POST['delete'])){ $db=$_POST['db']; $query=mysql_query("DROP DATABASE $db"); echo "Database $db has been deleted"; } ?> <html> <head> <title>MySQL Databases</title> </head> <body> <p><strong>Databases on localhost</strong>:</p> <? echo "$db_list"; ?> <?PHP //print_r($_POST); ?> <form action="pretask.php" method="post"> <select name="db"> <?PHP $db_list = mysql_list_dbs($connection); while ($row = mysql_fetch_object($db_list)) { //Here you are listing anything that should not be included if ($row->Database!="information_schema" && $row->Database!="mysql" && $row->Database!="phpmyadmin"){ echo "<option value=\"".$row->Database."\">".$row->Database."</option>"; } } ?> </select> <input type="submit" name="delete" value="Delete"/> </form> <form action="pretask.php" method="post"> Create Database <input type="text" name="database" /> <input type="submit" name="result" value="Create" /> </form> </body>
-
I'll try it tomorrow..will the database follow over to the next page as well? Thanks bud for all your help so far..you've been great!
-
Didnt change anything..still have to refresh. This is a tricky one to figure out
-
Ok the code you gave me works mint..but when i create and delete databases i have to refresh the page once or more to see the results. Any ideas?
-
Do I need this in the code
while ($i < mysql_num_rows($dbs)){ $db_names[$i] = mysql_tablename($dbs,$i); $db_list .="<li>$db_names[$i]"; $i++; $db_list .="</ul>"
or this
<? echo "$db_list"; ?>
-
I need my original list to show up somehow but no databases are showing up. Also the delete dropdown list isnt showing databases either probably because none are being show
-
^^^^^^^^^ anyone?
-
Did you scroll down through the whole code...i added what you told my to..to my code that i already had started
-
Yeah here is my full code and i have a create script right above that im not sure is 100% right or not..
<? $connection = @mysql_connect("localhost", "root", "") or die(mysql_error());; $dbs = @mysql_list_dbs($connection)or die(mysql_error()); $db_list="<ul>"; $i =0; while ($i < mysql_num_rows($dbs)){ $db_names[$i] = mysql_tablename($dbs,$i); $db_list .="<li>$db_names[$i]"; $i++; } $db_list .="</ul>"; ?> <HTML> <HEAD> <TITLE>MySQL Databases</TITLE> </HEAD> <P><strong>Databases on localhost</strong>:</p> <? echo "$db_list"; ?> </BODY> </HTML> <form action="pretask.php" method="post"> Create Database <input type="text" name="database" /> <input type="submit" name="result" value="Create" /> </form> </body> </html> <?php $database=$_POST['database']; $connection = @mysql_connect("localhost","root","") or die(mysql_error()); $sql="CREATE DATABASE $database "; $result = @mysql_query($sql,$connection) or die(mysql_error()); if (isset($_POST['result'])) { Results: echo "Database $database has been added"; } mysql_close($connection) ?> <?PHP print_r($_POST); ?> <form action="pretask.php" method="post"> <select name="db"> </html> <?PHP $connection = mysql_connect("localhost","root","") or die(mysql_error());; $db_list = mysql_list_dbs($connection); while ($row = mysql_fetch_object($db_list)) { if ($row->Database!="information_schema" && $row->Database!="mysql" && $row->Database!="phpmyadmin"){ echo "<option value=\"".$row->Database."\">".$row->Database."</option>"; } } ?> </select> <input type="submit" name="delete" value="delete"/> </form>
-
It says this now
Database testdb6 has been added Array ( [database] => testdb6 [result] => Create )
And it still doesn't delete anything from the list. Thank you so much for helping out this far though I appreciate it alot! I just want to figure this out
-
Do i have to change the "echo option value at the bottom?
-
Where would i add print_r($POST) if you dont mind me asking
-
Yeah i did and when i select one and hit delete nothing happens it doesnt delete it from the list of databases. It comes up with that error though in the above post.
-
Ok this helped me out alot this is my updated code with what you told me to add.. but this error came up..i tried choosing one of the databases i hit the delete button but it did not delete it. Here is the error:
Notice: Undefined index: database in C:\xampp\htdocs\pretask.php on line 42
<? $connection = @mysql_connect("localhost", "root", "") or die(mysql_error());; $dbs = @mysql_list_dbs($connection)or die(mysql_error()); $db_list="<ul>"; $i =0; while ($i < mysql_num_rows($dbs)){ $db_names[$i] = mysql_tablename($dbs,$i); $db_list .="<li>$db_names[$i]"; $i++; } $db_list .="</ul>"; ?> <HTML> <HEAD> <TITLE>MySQL Databases</TITLE> </HEAD> <P><strong>Databases on localhost</strong>:</p> <? echo "$db_list"; ?> </BODY> </HTML> <form action="pretask.php" method="post"> Create Database <input type="text" name="database" /> <input type="submit" name="result" value="Create" /> </form> </body> </html> <?php $database=$_POST['database']; $connection = @mysql_connect("localhost","root","") or die(mysql_error()); $sql="CREATE DATABASE $database "; $result = @mysql_query($sql,$connection) or die(mysql_error()); if (isset($_POST['result'])) { Results: echo "Database $database has been added"; } mysql_close($connection) ?> <html> <body> <form action="pretask.php" method="post"> <select> <?PHP $connection = mysql_connect("localhost","root","") or die(mysql_error());; $db_list = mysql_list_dbs($connection); while ($row = mysql_fetch_object($db_list)) { if ($row->Database!="information_schema" && $row->Database!="mysql" && $row->Database!="phpmyadmin"){ echo "<option>".$row->Database."</option>"; } } ?> </select> <input type="submit" name="delete" value="delete"/> </form>
-
Is there a shortcut instead of writing out all the databases in my if statement? Would it be easier to make another text field and use a delete "submit button" to delete the databases?
-
I have this for my drop down list but how do i actually show all the databases i have to use the variable $db_list somewhere not too sure though. It just shows $db_list and not the actual list is there another way to make a drop down list instead of this way?
<html> <body> <form action="pretask.php" method="post"> <select> <option>$db_list</option> </select> <input type="submit" name="delete" value="delete"/> </form> </body> </html>
-
Where would i put this in my code and i would have to make another submit button and add a value to it just like i did for the create button.
Help with login page
in PHP Coding Help
Posted
Hey guys i have a few question with this login script im trying to make. Ok so first im using php myadmin and i've created a simple login here that works fine but i want to tweak it so when i login i can login to a specific site where i've created tables and stuff. Is there anyone out there that can help me im a little stumped on how to do this. Thanks in regards. Here are my two scripts im using
show_login.html
login.php