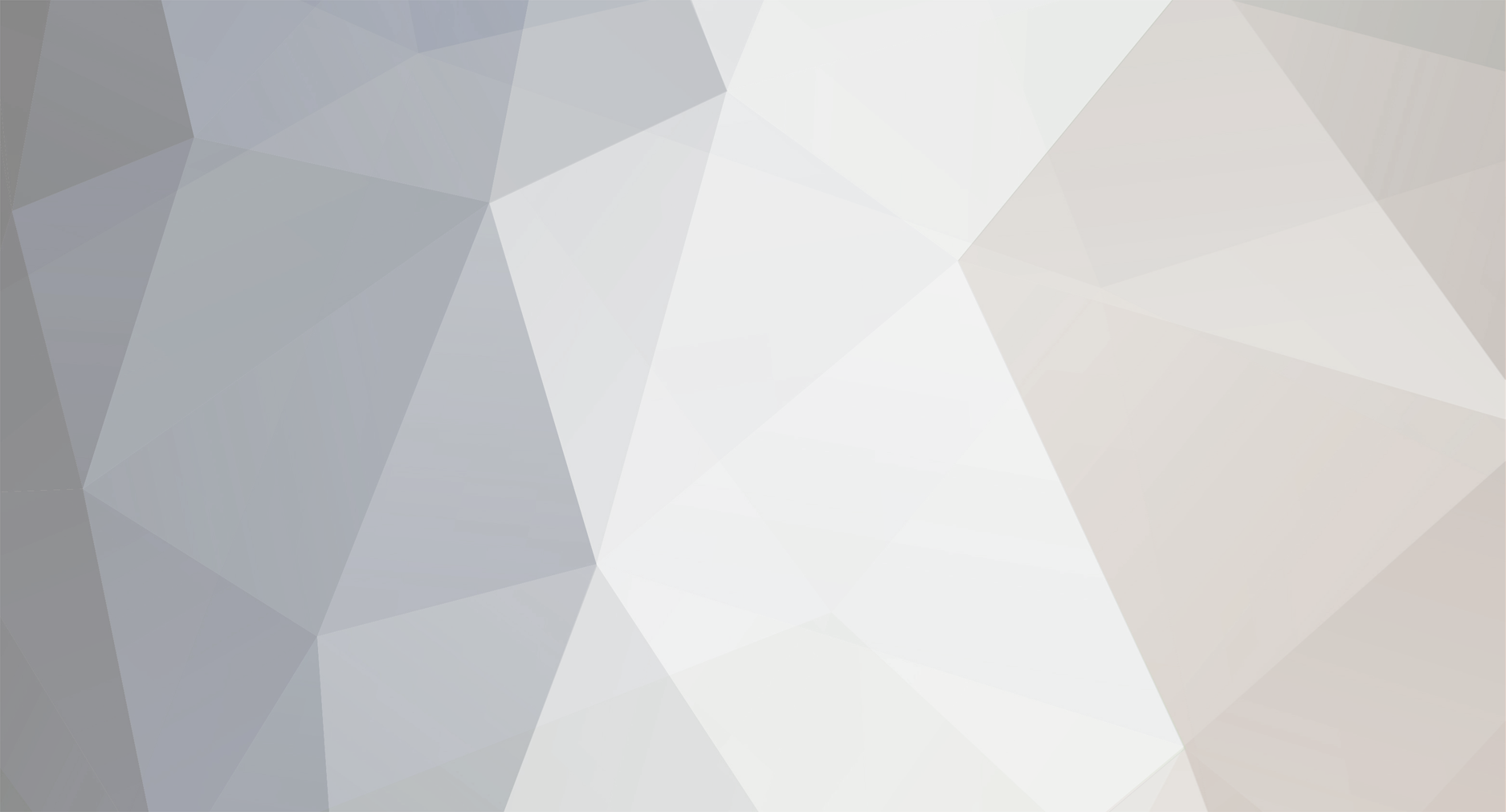
synritical
-
Posts
3 -
Joined
-
Last visited
Never
Posts posted by synritical
-
-
Thanks for your reply!
However, I already tried both of those ways - using double quotes causes it to be interpreted literally as $file, ie not render the variable at all.
Using double quotes throws up a PHP error.
Stuck!
-
Hello!
I'm very new to PHP, and I'm sure this is a noob question - still, its got me stuck!
How would I best escape this properly?
$resizeObj = new resize('C:\xampp\htdocs\images_test\$file');
so that $file is parsed?
the whole script is as follows:
<?php // *** Include Nathan's class include("resize-class.php"); // Define the full path to your folder from root $path = 'C:\xampp\htdocs\images_test'; // Open the folder $dir_handle = @opendir($path) or die("Unable to open $path"); // Loop through the files while ($file = readdir($dir_handle)) { if($file == "." || $file == ".." || $file == "index.php" ) continue; // *** 1) Initialise / load image $resizeObj = new resize('C:\xampp\htdocs\images_test\$file'); // *** 2) Resize image $resizeObj -> resizeImage(100, 100, 'auto'); // *** 3) Save image $resizeObj -> saveImage('C:\xampp\htdocs\images_test\$file', 90); echo "<a href=\"$file\">$file</a><br />"; echo $path; } // Close closedir($dir_handle); ?>
Escaping a PHP variable
in PHP Coding Help
Posted
Error is:
Warning: imagesx() expects parameter 1 to be resource, boolean given in C:\xampp\htdocs\resize-class.php on line 30
Warning: imagesy() expects parameter 1 to be resource, boolean given in C:\xampp\htdocs\resize-class.php on line 31
Warning: imagecopyresampled() expects parameter 2 to be resource, boolean given in C:\xampp\htdocs\resize-class.php on line 73
I'm guessing it has something to do with the class, which is