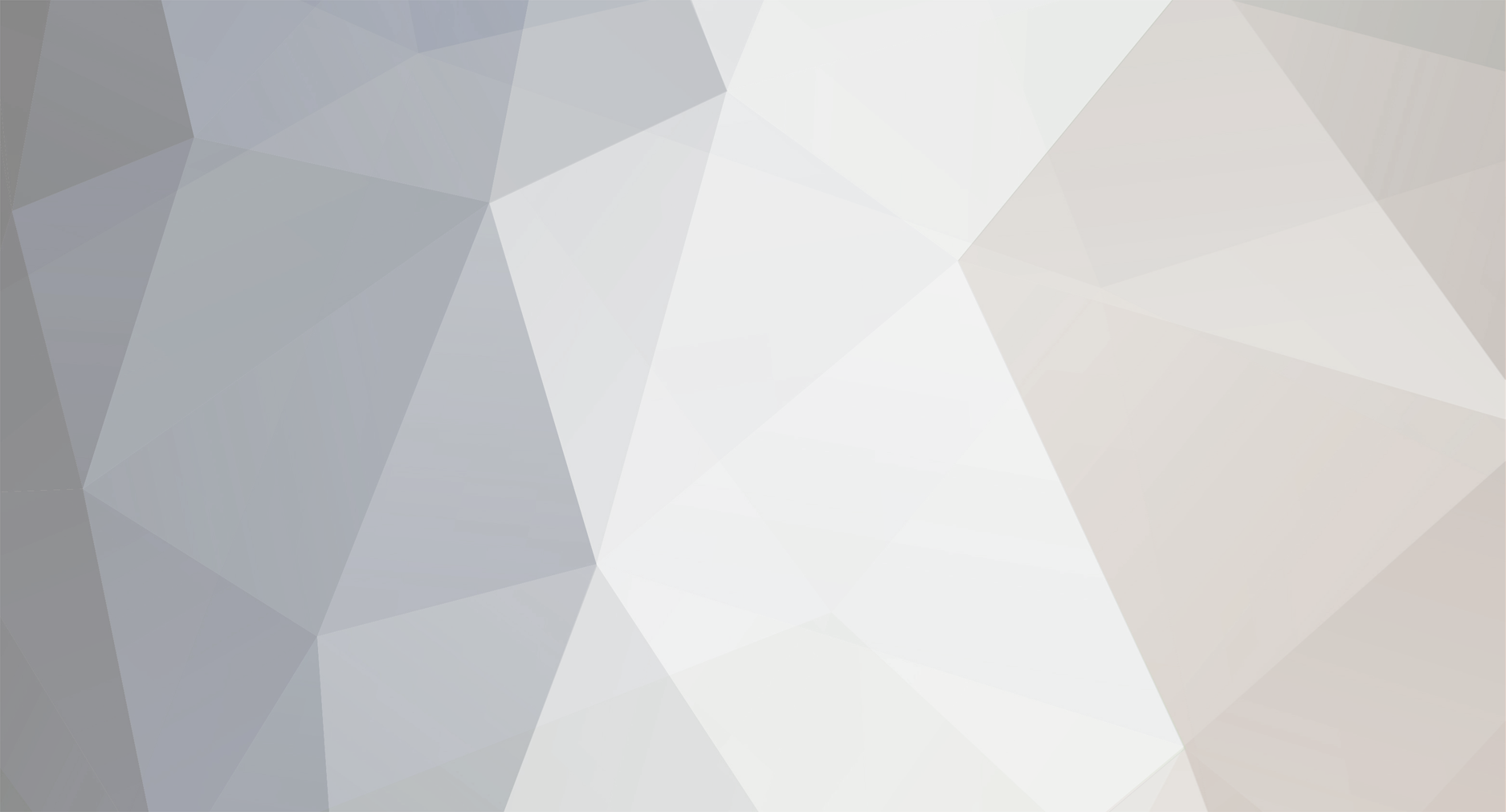
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
You have many wrong things going on in that. 1. $sql_query = mysqli_query(mysqli_connect($this->host,$this->username,$this->host_password),$this->query); $row = mysqli_fetch_assoc($this->sql_query); $num_row = mysqli_num_rows($this->sql_query);You are creating a local variable $sql_query, but then trying to use a class property $this->sql_query. Pick one or the other. 2. That class should not be responsible for connecting to the database, and shouldn't be connecting multiple times. You should pass in an already established connection that happens only once in your application. class my_login_class { private $db; public function __construct($db) { $this->db = $db; } } $db = new mysqli('localhost', 'root', '', 'cndesk'); // notice we have no mysqli_select_db - that's because // the database name is the 4th argument in the constructor $login = new my_login_class($db); 3.Your method names are a bit weird and inconsistent. In set_sql_query(), you are setting a class var but then in get_sql_query() you are executing, and getting results for, the query that you set previously. With this naming convention one would assume that get_sql_query() simply returns the query that you gave to set_sql_query(). 4. What is user_signup() supposed to be doing? 5. Make sure to always include visibility keywords on properties and methods, for clarity. Instead of function get_sql_query(){, it should be public function get_sql_query(){, like you do elsewhere. 6. Using var on class properties has been frowned upon for a long time now. You should use public, protected, or private accordingly. 7. The get_sql_query() method should not know what to do when the query has no results. It should simply tell the calling code such, and let the calling code figure it out. This method should not have that responsibility. Hope that helps.
-
Use syntax highlighter in wordpress plug-in options
scootstah replied to silvercover's topic in Applications
Something like one of these perhaps: http://ace.c9.io/#nav=about http://codemirror.net/ -
Post your code please.
-
You can use strpos() to see if a string exists within another string.
-
You can get the HTML with CURL and then use something like the DOM module (or one of the many other third party libraries) to parse it.
-
http://php.net/manual/en/function.glob.php
-
money_format() or number_format(). EDIT: I should read better. Those might not do what you want. Probably easiest to just use regex or what you're already doing.
-
80-90C for a modern CPU is a bit on the warm side, in my opinion. It's probably not going to do any immediate harm, and it should shut itself down if it gets too hot, but I definitely wouldn't want to run at those temps for long. Idling at 60C is definitely not okay with me. Make sure that your heatsink is mounted properly, with a good quality thermal paste. Don't try to spread it like peanut butter like some people try to tell you, just put a pea sized dot in the center and you're good. It could just be that your tower doesn't have very good airflow. Make sure all of the fans are working properly and running at the correct RPM, and make sure all of the fan grills are free of dust/debris. Make sure there is not a whole jungle of wiring directly in the flow path. Could also be that ambient temperatures are too high. What is the temperature in that room?
-
I would say it probably has to do with the blocking, then. The blocking says that PHP has to wait for output, so it seems to be stuck there.
-
Have a look at the manual. http://php.net/manual/en/function.curl-exec.php
-
Something I just thought of: you can try stream_get_contents instead of all the fread stuff. $stream = ssh2_exec($connection, $runCMD); echo stream_get_contents($stream);See if that works.
-
PHP and HTML are not interchangeable languages. They do very different things. You cannot just convert PHP code to HTML. It's possible to generate static HTML from PHP, but then your site will not be dynamic, and you cannot do things like select data based on a query parameter, which is what it looks like you want. Pay a couple dollars a month and get some hosting.
-
No, feof() returns true once it reaches the end of the file.
-
When I create a test stream, everything works perfectly. $stream = fopen('data://text/plain,This is a sample stream of text', 'r'); $data = ''; while(feof($stream) === false) { $data .= fread($stream, 4096); } echo $data;I get "This is a sample stream of text" output. I would suggest inspecting $stream to make sure that it is in fact what you think it is, and also to make sure error reporting is turned on.
-
I really see no reason to use ? placeholders. It makes it hard to read and on more complicated queries it just becomes a headache very fast.
-
Yes that makes sense that it would refresh and never return anything, because you had an infinite loop going.
-
Are you sure that $stream is what you think it is? And do you have error reporting on?
-
Oops, my bad. Updated: while(feof($stream) === false) { $data .= fread($stream, 4096); }
-
You have an infinite loop. It should be like this: while(feof($stream) === false) { $data .= fread($buffer, 4096); }
-
rawurldecode() not decoding %20 as white space
scootstah replied to jbonnett's topic in PHP Coding Help
When you call $_GET['url'], PHP automatically decodes it. So when you run it through filter_var(), the string has a space in it which is an illegal character, and is thus removed. I've played with it for a bit and I can't see a real clean way to do what you want. I came up with this: (warning - I'm very tired right now. This could be terrible) $parts = explode("/", trim($_GET['url'], "/")); $parts = array_map(function($part){ return urldecode(filter_var(urlencode($part), FILTER_SANITIZE_URL)); }, $parts);This will break up your parameter without sanitizing it, and then it will iterate over each part and do the following: urlencode (to convert the decoded spaces back to +), filter_var (to remove illegal characters), urldecode (to convert any encoded characters back again). Pretty hacky. The way I would do it though, is to just not use filter_var. I would just use regex to replace illegal characters, since there is a whole bunch of characters allowed in FILTER_SANITIZE_URL that really don't need to be in a URL. -
rawurldecode() not decoding %20 as white space
scootstah replied to jbonnett's topic in PHP Coding Help
How are you retrieving that URI? Can we see some actual code? echo rawurldecode("On%20the%20go"); running this produces "On the go" as expected, for me anyway. -
imagerotate() function sometimes work and sometimes not
scootstah replied to CocoDu43's topic in PHP Coding Help
Perhaps it is neither 90 nor 270 degrees? -
You could either use file_get_contents() with the allow_url_fopen config setting turned on, or you could just use CURL and save the response.
-
Yes but it's using the INSERT syntax, not UPDATE syntax. Look at his example compared to your code.