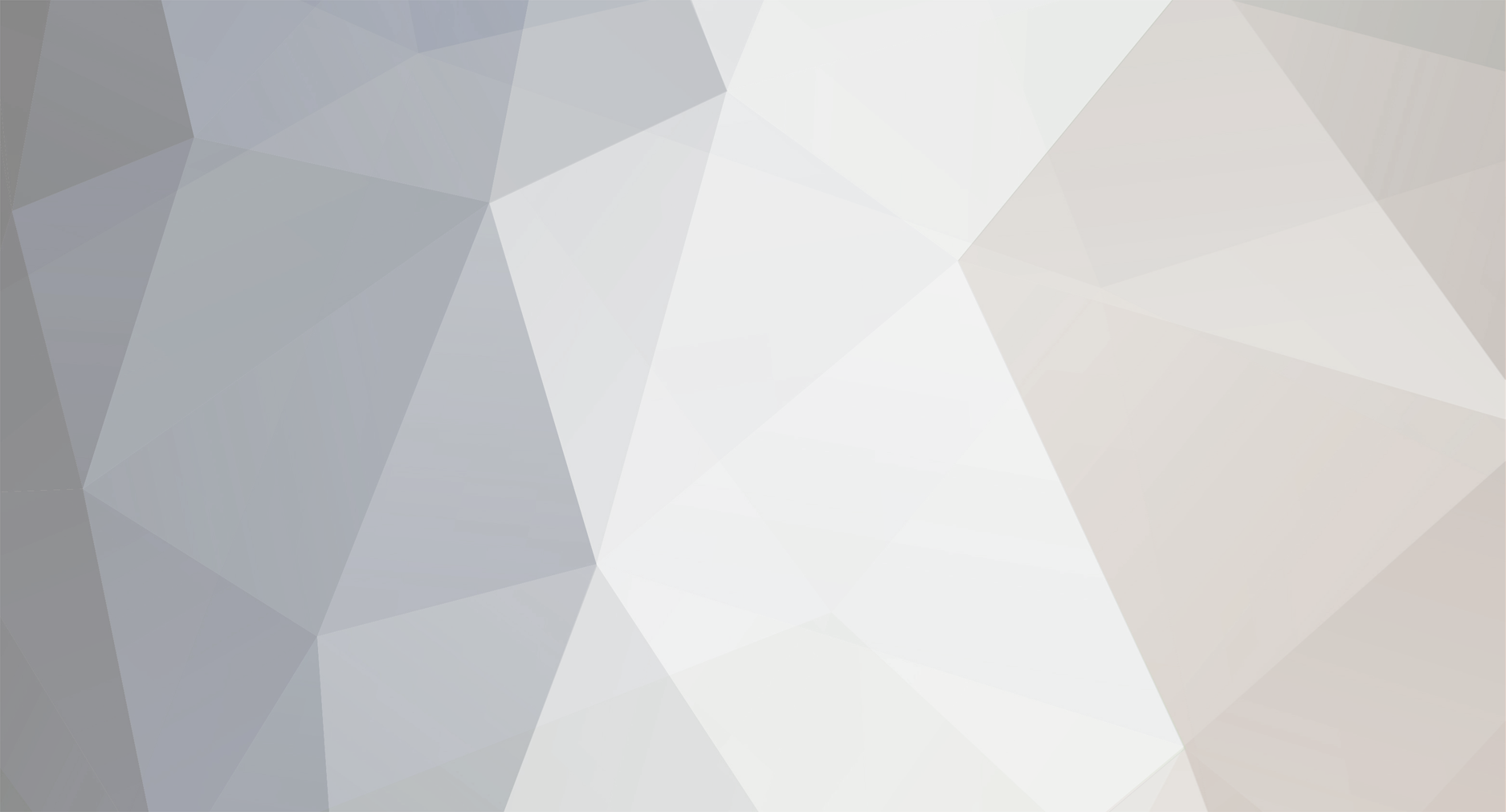
Rabastan
Members-
Posts
33 -
Joined
-
Last visited
Profile Information
-
Gender
Not Telling
Rabastan's Achievements

Member (2/5)
0
Reputation
-
This is my first attempt at a registration script. Where do I need improve it? Thanks in advance Rab // Get values from form $username=$_POST['username']; $password=$_POST['password']; $confirm=$_POST['confirm']; $firstname=$_POST['firstname']; $lastname=$_POST['lastname']; $email=$_POST['email']; $address=$_POST['address']; $address2=$_POST['address2']; $city=$_POST['city']; $state=$_POST['state']; $zip=$_POST['zip']; $phone=$_POST['phone']; $dob=$_POST['dob']; // Strip any escape characters $username = stripslashes($username); $password = stripslashes($password); $confirm = stripslashes($confirm); $firstname = stripslashes($firstname); $lastname = stripslashes($lastname); $email = stripslashes($email); $address = stripslashes($address); $address2 = stripslashes($address2); $city = stripslashes($city); $state = stripslashes($state); $zip = stripslashes($zip); $phone = stripslashes($phone); $dob = stripslashes($dob); //Check for empty fields if(empty($_POST['username'])){ echo (USERNAME_BLANK_ERROR); } if(empty($_POST['password'])){ echo (PASSWORD_BLANK_ERROR); } if(empty($_POST['confirm'])){ echo (CONFIRM_BLANK_ERROR); } if(empty($_POST['firstname'])){ echo (FIRSTNAME_BLANK_ERROR); } if(empty($_POST['lastname'])){ echo (LASTNAME_BLANK_ERROR); } if(empty($_POST['email'])){ echo (EMAIL_BLANK_ERROR); } if(empty($_POST['address'])){ echo (ADDRESS_BLANK_ERROR); } if(empty($_POST['city'])){ echo (CITY_BLANK_ERROR); } if(empty($_POST['state'])){ echo (STATE_BLANK_ERROR); } if(empty($_POST['zip'])){ echo (ZIP_BLANK_ERROR); } if(empty($_POST['phone'])){ echo (PHONE_BLANK_ERROR); } if(empty($_POST['dob'])){ echo (DOB_BLANK_ERROR); } // Verify field lengths if(strlen($username) < 6){ echo (USERNAME_SHORT_ERROR); } if(strlen($username) > 15){ echo (USERNAME_LONG_ERROR); } if(strlen($password) < 6){ echo (PASSWORD_SHORT_ERROR); } if(strlen($password) > 15){ echo (PASSWORD_LONG_ERROR); } if(strlen($firstname) < 2){ echo (FIRSTNAME_SHORT_ERROR); } if(strlen($firstname) > 25){ echo (FIRSTNAME_LONG_ERROR); } if(strlen($lastname) < 2){ echo (LASTNAME_SHORT_ERROR); } if(strlen($laststname) > 25){ echo (LASTNAME_LONG_ERROR); } if(strlen($email) < 10){ echo (EMAIL_SHORT_ERROR); } if(strlen($email) > 100){ echo (EMAIL_LONG_ERROR); } if(strlen($address) < 5){ echo (ADDRESS_SHORT_ERROR); } if(strlen($address) > 200){ echo (ADDRESS_LONG_ERROR); } if(strlen($city) < 3){ echo (CITY_SHORT_ERROR); } if(strlen($city) > 22){ echo (CITY_LONG_ERROR); } if(strlen($zip) < 5){ echo (ZIP_SHORT_ERROR); } if(strlen($zip) > 5){ echo (ZIP_LONG_ERROR); } // Compare Passwords if ($password != $confirm) { echo(PASSWORD_MATCH_ERROR); } // Validate Phone Number if( !preg_match("/^([1]-)?[0-9]{3}-[0-9]{3}-[0-9]{4}$/i", $phone) ) { echo (PHONE_VALIDATE_ERROR); } // Validate Email Address if( !preg_match("/^[_a-z0-9-]+(.[_a-z0-9-]+)*@[a-z0-9-]+(.[a-z0-9-]+)*(.[a-z]{2,3})$/i", $email) ) { echo (EMAIL_VALIDATE_ERROR); } // Hash password and validate hash $hash = $hasher->HashPassword($password); if(strlen($hash) <= 20){ echo (HASH_ERROR); } // Insert User into database $sql="INSERT INTO $users(user_username, user_password, user_email, user_firstname, user_lastname, user_address, user_address2, user_city, user_state, user_zip, user_phone, user_dob)VALUES('$username', '$hash', '$firstname', '$lastname', '$address', '$address2', '$city', '$state', '$zip', '$phone', '$dob')"; $result=mysql_query($sql); if($result){ echo (REGISTRATION_SUCCESS); echo "<BR>"; echo "<a href='reg_thankyou.php'>Back to main page</a>"; } else { echo (REGISTRATION_ERROR); } ?> <?php // close connection mysql_close(); ?>
-
Ok, I am getting this error: Warning: mysql_num_rows() expects parameter 1 to be resource, boolean given in C:\xampp\htdocs\base_site\admin\app\classes\authentication.php on line 74 From This code: class Login { private $_id; private $_username; private $_password; private $_passmd5; private $_errors; private $_access; private $_login; private $_token; public function __construct() { $this->_errors = array(); $this->_login = isset($_POST['login'])? 1 : 0; $this->_access = 0; $this->_token = $_POST['token']; $this->_id = 0; $this->_username = ($this->_login)? $this->filter($_POST['username']) : $_SESSION['username']; $this->_password = ($this->_login)? $this->filter($_POST['password']) : ''; $this->_passmd5 = ($this->_login)? md5($this->_password) : $_SESSION['password']; } public function isLoggedIn() { ($this->_login)? $this->verifyPost() : $this->verifySession(); return $this->_access; } public function filter($var) { return preg_replace('/[^a-zA-Z0-9]/','',$var); } public function verifyPost() { try { if(!$this->isTokenValid()) throw new Exception('Invalid Form Submission'); if(!$this->isDataValid()) throw new Exception('Invalid Form Data'); if(!$this->verifyDatabase()) throw new Exception('Invalid Username/Password'); $this->_access = 1; $this->registerSession(); } catch(Exception $e) { $this->_errors[] = $e->getMessage(); } } public function verifySession() { if($this->sessionExist() && $this->verifyDatabase()) $this->_access = 1; } public function verifyDatabase() { //Database Connection Data mysql_connect("localhost", "root", "") or die(mysql_error()); mysql_select_db("base_admin") or die(mysql_error()); $data = mysql_query("SELECT ID FROM tblAdmins WHERE adminsUser = '{$this->_username}' AND adminsPassword = '{$this->_passmd5}'"); if(mysql_num_rows($data)) { list($this->_id) = @array_values(mysql_fetch_assoc($data)); return true; } else { return false; } } public function isDataValid() { return (preg_match('/^[a-zA-Z0-9]{5,12}$/',$this->_username) && preg_match('/^[a-zA-Z0-9]{5,12}$/',$this->_password))? 1 : 0; } public function isTokenValid() { return (!isset($_SESSION['token']) || $this->_token != $_SESSION['token'])? 0 : 1; } public function registerSession() { $_SESSION['ID'] = $this->_id; $_SESSION['username'] = $this->_username; $_SESSION['password'] = $this->_passmd5; } public function sessionExist() { return (isset($_SESSION['username']) && isset($_SESSION['password']))? 1 : 0; } public function showErrors() { echo "<h3>Errors</h3>"; foreach($this->_errors as $key=>$value) echo $value."<br>"; } } What in the world am I doing wrong?? Rab
-
Thank you all so much!! Rab
-
Where do I place: return (mysql_connect($strHostName, $strUserName, $strPassword)) ? true : false; in the code Thanx so much Rab
-
OK, I got this now Parse error: syntax error, unexpected 'return' (T_RETURN) in C:\xampp\htdocs\base_site\admin\app\functions\database.php on line 23 Rab
-
I am new to PHP/MySQL and I am experimenting with separating things to different pages and Include/Requiring them when needed. I am trying to make a database connection, but keep getting my "die" error. OK Here is what I have: At the top of the page I am trying to load I have: require('app/application.php'); I have a page called "app.php" // set the level of error reporting error_reporting(E_ALL & ~E_NOTICE); // load file, folder and database parameters include('app/config.php'); // include the list of project filenames require(DIR_APPLICATION . 'filenames.php'); // include the list of project database tables require(DIR_APPLICATION . 'db_tables.php'); // include the database functions require(DIR_FUNCTIONS . 'database.php'); // make a connection to the database... now dbconn($strHostName, $strDbName, $strUserName, $strPassword) or die('Unable to connect to database server!'); Here is "config.php" // Define the webserver and path parameters define('DIR_APPLICATION', 'app/'); define('DIR_INCLUDES', 'inc/'); define('DIR_FUNCTIONS', DIR_APPLICATION . 'functions/'); define('DIR_CLASSES', DIR_APPLICATION . 'classes/'); define('DIR_MODULES', DIR_APPLICATION . 'modules/'); define('DIR_LANGUAGES', DIR_APPLICATION . 'languages/'); // Define image directories define('DIR_IMAGES', 'images/'); define('DIR_ICONS', DIR_IMAGES . 'icons/'); Here is "database.php" $strHostName = "localhost"; //e.g., "localhost". $strDbName = "base_admin"; //Check if yours have prefixes. $strUserName = "root"; //For the database, not your hosting account. $strPassword = ""; function dbconn($strHostName, $strDbName, $strUserName, $strPassword) { } I just keep getting "Unable to connect to database server!" What am I missing for petes sake. Rab
-
Pikachu, My apologies then, you came off to me as slamming me for using code from another site. If that was not the case I truly apologize. Rab
-
Yah I am pretty sure that there pretty much explains what I am trying to do!! Rab
-
What does it matter where I got the code from, this forum is entitled "PHP Coding HELP"!!! Which would lead one to assume its a place to go when you need help with PHP, NOT somewhere to go and get ridiculed about my php. If that is what I was looking for I would have gone to "Tease you because your not as good as I am" forum. Come on people grow the fuck up!! If your not interested in helping don't post, does it really make you feel better to put people down?? Rab
-
Rather new to PHP but I am learning I have a simple login system for a website, and a table for users. user table has these fields; id firstname lastname email username password What I would like to do is store the first and last name in the session also in order to display "Welcome FIRSTNAME LASTNAME" on the site Code is as follows; main_login.php <table width="300" border="0" align="center" cellpadding="0" cellspacing="1" bgcolor="#CCCCCC"> <tr> <form name="form1" method="post" action="checklogin.php"> <td> <table width="100%" border="0" cellpadding="3" cellspacing="1" bgcolor="#FFFFFF"> <tr> <td colspan="3"><strong>Member Login </strong></td> </tr> <tr> <td width="78">Username</td> <td width="6">:</td> <td width="294"><input name="myusername" type="text" id="myusername"></td> </tr> <tr> <td>Password</td> <td>:</td> <td><input name="mypassword" type="text" id="mypassword"></td> </tr> <tr> <td> </td> <td> </td> <td><input type="submit" name="Submit" value="Login"></td> </tr> </table> checklogin.php $host="localhost"; // Host name $username="******"; // Mysql username $password="*****"; // Mysql password $db_name="*****"; // Database name $tbl_name="members"; // Table name // Connect to server and select databse. mysql_connect("$host", "$username", "$password")or die("cannot connect"); mysql_select_db("$db_name")or die("cannot select DB"); // username and password sent from form $myusername=$_POST['myusername']; $mypassword=$_POST['mypassword']; // To protect MySQL injection (more detail about MySQL injection) $myusername = stripslashes($myusername); $mypassword = stripslashes($mypassword); $myusername = mysql_real_escape_string($myusername); $mypassword = mysql_real_escape_string($mypassword); $sql="SELECT * FROM $tbl_name WHERE username='$myusername' and password='$mypassword'"; $result=mysql_query($sql); // Mysql_num_row is counting table row $count=mysql_num_rows($result); // If result matched $myusername and $mypassword, table row must be 1 row if($count==1){ // Register $myusername, $mypassword and redirect to file "login_success.php" session_register("myusername"); session_register("mypassword"); header("location:index.php"); } else { echo "Wrong Username or Password"; } ?> Any help is appreciated Thank You Ran
-
In the following code, when I load the page into my browser everything works fine. However when I include it in its div I get an error. I dont get it?? The error i get is "Query was empty" Here is the code, Like I said I ohnly get the error when I include the page in the site. If I load it on its own it works fine. <?php if (!function_exists("GetSQLValueString")) { function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "") { if (PHP_VERSION < 6) { $theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue; } $theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue); switch ($theType) { case "text": $theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL"; break; case "long": case "int": $theValue = ($theValue != "") ? intval($theValue) : "NULL"; break; case "double": $theValue = ($theValue != "") ? doubleval($theValue) : "NULL"; break; case "date": $theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL"; break; case "defined": $theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue; break; } return $theValue; } } if (!function_exists("GetSQLValueString")) { function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "") { if (PHP_VERSION < 6) { $theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue; } $theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue); switch ($theType) { case "text": $theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL"; break; case "long": case "int": $theValue = ($theValue != "") ? intval($theValue) : "NULL"; break; case "double": $theValue = ($theValue != "") ? doubleval($theValue) : "NULL"; break; case "date": $theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL"; break; case "defined": $theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue; break; } return $theValue; } } $editFormAction = $_SERVER['PHP_SELF']; if (isset($_SERVER['QUERY_STRING'])) { $editFormAction .= "?" . htmlentities($_SERVER['QUERY_STRING']); } if ((isset($_POST["MM_update"])) && ($_POST["MM_update"] == "form1")) { $updateSQL = sprintf("UPDATE todo SET updated=NOW(), completed=%s WHERE id=%s", GetSQLValueString(isset($_POST['completed']) ? "true" : "", "defined","'Y'","'N'"), GetSQLValueString($_POST['id'], "int")); mysql_select_db($database_sitterlink, $sitterlink); $Result1 = mysql_query($updateSQL, $sitterlink) or die(mysql_error()); $updateGoTo = "../../index.php"; if (isset($_SERVER['QUERY_STRING'])) { $updateGoTo .= (strpos($updateGoTo, '?')) ? "&" : "?"; $updateGoTo .= $_SERVER['QUERY_STRING']; } header(sprintf("Location: %s", $updateGoTo)); } $editFormAction = $_SERVER['PHP_SELF']; if (isset($_SERVER['QUERY_STRING'])) { $editFormAction .= "?" . htmlentities($_SERVER['QUERY_STRING']); } if ((isset($_POST["MM_update"])) && ($_POST["MM_update"] == "form1")) { $updateSQL = sprintf("UPDATE todo SET completed=%s WHERE id=%s,", GetSQLValueString(isset($_POST['completed']) ? "true" : "", "defined","'Y'","'N'"), GetSQLValueString($_POST['id'], "int")); mysql_select_db($database_sitterlink, $sitterlink); $Result1 = mysql_query($updateSQL, $sitterlink) or die(mysql_error()); $updateGoTo = "../../index.php"; if (isset($_SERVER['QUERY_STRING'])) { $updateGoTo .= (strpos($updateGoTo, '?')) ? "&" : "?"; $updateGoTo .= $_SERVER['QUERY_STRING']; } header(sprintf("Location: %s", $updateGoTo)); } ?> <?php do { ?> <table width="100%" border="0"> <tr> <td><?php echo $row_todopend['id']; ?></td> </tr> <tr> <td><?php echo $row_todopend['created']; ?></td> </tr> <tr> <td><?php echo $row_todopend['name']; ?></td> </tr> <tr> <td><?php echo $row_todopend['desc']; ?></td> </tr> <tr> <td> </td> </tr> <tr> <td> <form action="<?php echo $editFormAction; ?>" method="post" name="form1" id="form1"> <table align="center"> <tr valign="baseline"> <td nowrap="nowrap" align="right">Completed:</td> <td><input type="checkbox" name="completed" value="" <?php if (!(strcmp(htmlentities($row_todopend['completed'], ENT_COMPAT, ''),"Y"))) {echo "checked=\"checked\"";} ?> /></td> </tr> <tr valign="baseline"> <td nowrap="nowrap" align="right"> </td> <td><input type="submit" value="Update record" /></td> </tr> </table> <input type="hidden" name="id" value="<?php echo $row_todopend['id']; ?>" /> <input type="hidden" name="MM_update" value="form1" /> <input type="hidden" name="id" value="<?php echo $row_todopend['id']; ?>" /> </form> <p> </p></td> </tr> <tr> <td> </td> </tr> <tr> <td> </td> </tr> </table> <?php } while ($row_todopend = mysql_fetch_assoc($todopend)); ?> <?php mysql_free_result($todopend); ?>
-
I have this code produced by dreamweaver. That allows me to click a checkbox and change a Enum Value from no to yes. <?php if (!function_exists("GetSQLValueString")) { function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "") { if (PHP_VERSION < 6) { $theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue; } $theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue); switch ($theType) { case "text": $theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL"; break; case "long": case "int": $theValue = ($theValue != "") ? intval($theValue) : "NULL"; break; case "double": $theValue = ($theValue != "") ? doubleval($theValue) : "NULL"; break; case "date": $theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL"; break; case "defined": $theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue; break; } return $theValue; } } $editFormAction = $_SERVER['PHP_SELF']; if (isset($_SERVER['QUERY_STRING'])) { $editFormAction .= "?" . htmlentities($_SERVER['QUERY_STRING']); } if ((isset($_POST["MM_update"])) && ($_POST["MM_update"] == "form1")) { $updateSQL = sprintf("UPDATE todo SET completed=%s WHERE id=%s", GetSQLValueString(isset($_POST['updatetodo']) ? "true" : "", "defined","'Y'","'N'"), GetSQLValueString($_POST['hiddenField'], "int")); mysql_select_db($database_sitterlink, $sitterlink); $Result1 = mysql_query($updateSQL, $sitterlink) or die(mysql_error()); $updateGoTo = "../../index.php"; if (isset($_SERVER['QUERY_STRING'])) { $updateGoTo .= (strpos($updateGoTo, '?')) ? "&" : "?"; $updateGoTo .= $_SERVER['QUERY_STRING']; } header(sprintf("Location: %s", $updateGoTo)); } mysql_select_db($database_sitterlink, $sitterlink); $query_todopending = "SELECT * FROM todo"; $todopending = mysql_query($query_todopending, $sitterlink) or die(mysql_error()); $row_todopending = mysql_fetch_assoc($todopending); $totalRows_todopending = mysql_num_rows($todopending); ?> How would I eliminate the checkbox so I could just hit submit and have it update. Rab
-
I have a site with a todo list, in the list I have a row called "Complete" with a value of no / yes. I would like to display a list with all unfinished entries and in each entry have a button that say "Complete", when clicked the Enum value will be changed to "yes" and the list will be refreshed. How would I do this?? Rab
-
Ok if i use the insert query you wrote it works, it sets both dates the same on insert, and changes updated after update. But if I use PHPmyadmin's insert function it doesnt work Rab
-
Darn, didnt work. I was hoping It Created the original date in the Updated area and Didnt update either. Rab