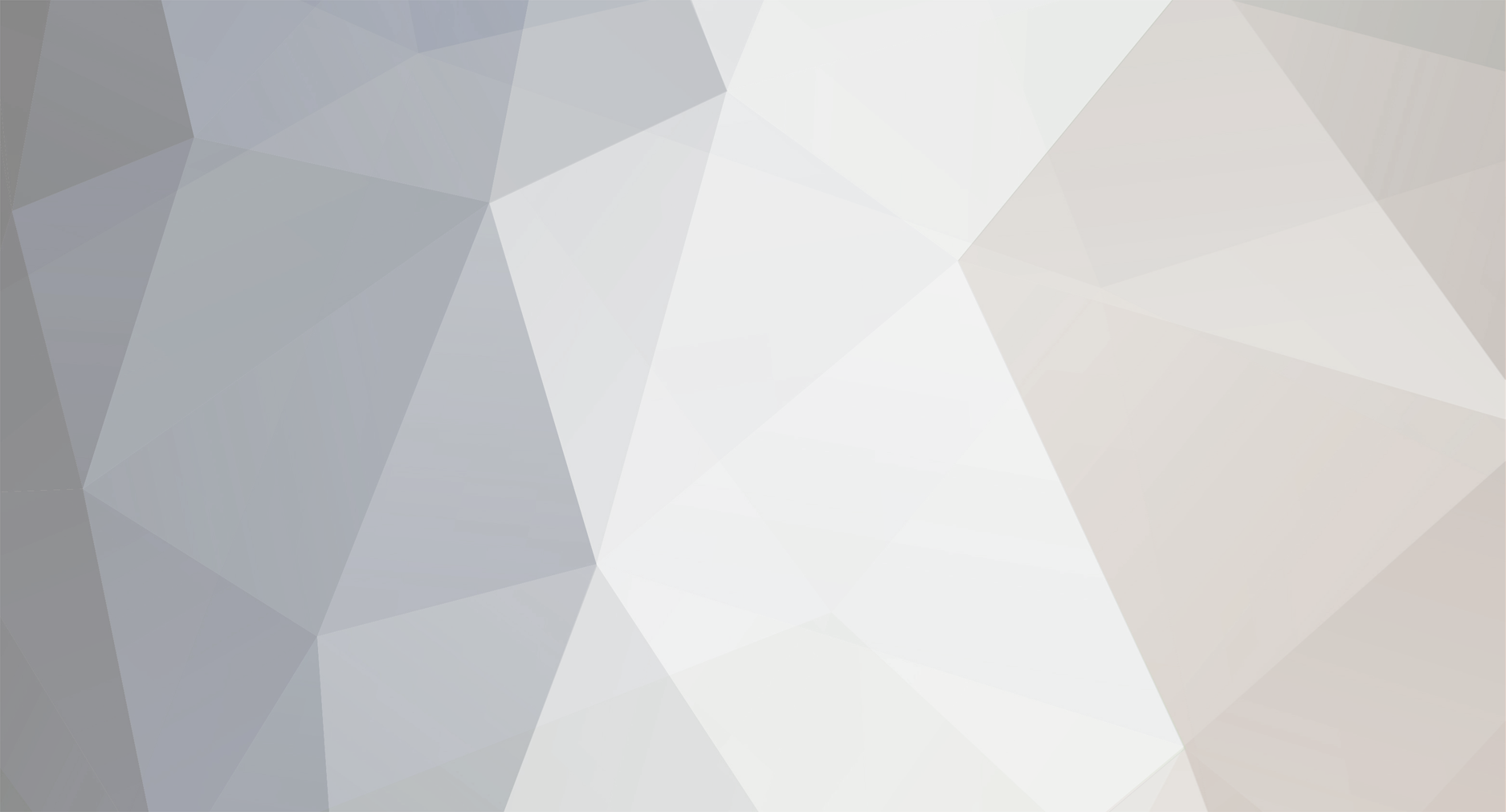
davids_media
-
Posts
58 -
Joined
-
Last visited
Posts posted by davids_media
-
-
I have a problem at the moment. I want to create dynamic hyperlinks, so when new links are eventually added, they will automatically populate rather than create them one by one.
The problem I have is the displaying of them at the moment. Because I have gone down the procedural route, I have done my code like this;
<?php require_once (MYSQL); $query = "SELECT * FROM cat WHERE catID='{$_GET['catID']}' ORDER BY cat ASC"; $r = mysqli_query($dbc, $query); while($row = mysqli_fetch_array($r, MYSQLI_ASSOC)) { echo "<li>" . "<a href='cat.php?catID={$row['catID']}' title='{$row['cat']}'>" . $row['cat'] . "</a>" . "</li>"; } ?>
However I get the error message as displayed as my topic title. How do I resolve this please as I dont think I have much work to do, though its just a real struggle trying to resolve it.
-
Following PFMaBiSmAd's advice, I have applied the mysqli_insert_id() statement after my insert query statement, but whilst it writes perfectly fine to the database, I now would like if is possible to direct to add_image.php and retrieve the ID of the last record inserted. here is my code at present;
<?php ini_set('display_errors',1); error_reporting(-1); require_once ('./includes/config.inc.php'); require_once (MYSQL); $add_cat_errors = array(); if ($_SERVER['REQUEST_METHOD'] == 'POST') { // Check for a name: if (empty($_POST['product'])) { $add_cat_errors['product'] = 'Please enter the name!'; } // Check for a description: if (empty($_POST['prod_descr'])) { $add_cat_errors['prod_descr'] = 'Please enter the description!'; } // Check for a category: if (!isset($_POST['cat']) || !filter_var($_POST['cat'], FILTER_VALIDATE_INT, array('min_range' => 1))) { $add_cat_errors['cat'] = 'Please select a category!'; } // Check for a price: if (empty($_POST['price']) || !filter_var($_POST['price'], FILTER_VALIDATE_FLOAT) || ($_POST['price'] <= 0)) { $add_cat_errors['price'] = 'Please enter a valid price!'; } // Check for a category: if (!isset($_POST['directory']) || !filter_var($_POST['directory'], FILTER_VALIDATE_INT, array('min_range' => 1))) { $add_cat_errors['directory'] = 'Please select a directory!'; } // Check for a stock: if (empty($_POST['stock']) || !filter_var($_POST['stock'], FILTER_VALIDATE_INT, array('min_range' => 1))) { $add_cat_errors['stock'] = 'Please enter the quantity in stock!'; } if (empty($add_cat_errors)) { $query = "INSERT INTO product (product, prod_descr, catID, price, dirID, stock) VALUES (?, ?, ?, ?, ?, ?)"; // Prepare the statement: $stmt = mysqli_prepare($dbc, $query); // $id = mysqli_insert_id($dbc, $query); // For debugging purposes: //if (!$stmt) echo mysqli_stmt_error($stmt); // Bind the variables: mysqli_stmt_bind_param($stmt, 'sssssi', $name, $desc, $_POST['cat'], $_POST['price'], $_POST['directory'], $_POST['stock']); $last_id = mysqli_stmt_insert_id($stmt); $last_id = mysqli_insert_id($dbc); // Make the extra variable associations: $name = strip_tags($_POST['product']); $desc = strip_tags($_POST['prod_descr']); // Execute the query: mysqli_stmt_execute($stmt); if (mysqli_stmt_affected_rows($stmt) == 1) { // If it ran OK. // Print a message: echo '<h4>The product has been added!</h4>'; // Clear $_POST: $_POST = array(); // Clear $_FILES: $_FILES = array(); } else { // If it did not run OK. trigger_error('The product could not be added due to a system error. We apologize for any inconvenience.'); } } // End of $errors IF. } else { // Clear out the session on a GET request: } // End of the submission IF. require_once ('./includes/form_functions.inc.php'); ?> <form enctype="multipart/form-data" action="add_product2.php" method="post" accept-charset="utf-8"> <input type="hidden" name="MAX_FILE_SIZE" value="524288" /> Product<br /><?php create_form_input('product', 'text', $add_cat_errors); ?> Description<br /><?php create_form_input('prod_descr', 'textarea', $add_cat_errors); ?> Category<br /><select name="cat"<?php if (array_key_exists('cat', $add_cat_errors)); ?>> <option>Select One</option> <?php // Retrieve all the categories and add to the pull-down menu: $q = 'SELECT catID, cat FROM category ORDER BY cat ASC'; $r = mysqli_query ($dbc, $q); while ($row = mysqli_fetch_array ($r, MYSQLI_NUM)) { echo "<option value=\"$row[0]\""; // Check for stickyness: if (isset($_POST['cat']) && ($_POST['cat'] == $row[0]) ) echo ' selected="selected"'; echo ">$row[1]</option>\n"; } ?> </select><?php if (array_key_exists('cat', $add_cat_errors)) echo $add_cat_errors['cat']; ?> Price<br /><?php create_form_input('price', 'text', $add_cat_errors); ?> Directory<br /><select name="directory"<?php if (array_key_exists('directory', $add_cat_errors)); ?>> <option>Select One</option> <?php // Retrieve all the categories and add to the pull-down menu: $q = 'SELECT dirID, directory FROM directory ORDER BY directory ASC'; $r = mysqli_query ($dbc, $q); while ($row = mysqli_fetch_array ($r, MYSQLI_NUM)) { echo "<option value=\"$row[0]\""; // Check for stickyness: if (isset($_POST['directory']) && ($_POST['directory'] == $row[0]) ) echo ' selected="selected"'; echo ">$row[1]</option>\n"; } ?> </select><?php if (array_key_exists('directory', $add_cat_errors)) echo $add_cat_errors['directory']; ?> </select> <br /> Stock<br /><?php create_form_input('stock', 'text', $add_cat_errors); ?> <input type="submit" value="Add This Product" class="button" /> </fieldset> </form>
however, I have tried using this querystring in my form
<form action="add_image.php?prodID=$lastid method="post">
but it does not output the product ID. also I did contemplate using headers (to redirect) butI thought it was not a good idea especially since I am writing and posting data from a HTML form.
also, here is my add_image.php code at present (the page which should then be redirected to upon submission)
<?php ini_set('display_errors',1); error_reporting(-1); require_once ('./includes/config.inc.php'); require_once (MYSQL); $add_cat_errors = array(); if($id = isset($_GET['prodID'])) { $q = "SELECT `prodID`, `product`, `prod_descr`, `catID`, `dirID`, `price`, `stock` FROM product WHERE `prodID`='{$_GET['prodID']}'"; $r = mysqli_query($dbc, $q); $id = mysqli_insert_id($dbc); if (!$r) { echo "Error with MySQL query:<br /><i>{$sql}</i><br />MySQL returned: " . mysql_error() . "<P />"; } while($row = mysqli_fetch_array($r)) { echo $row['product']; echo "<br />"; echo $row['prod_descr']; echo "<br />"; echo $row['catID']; echo "<br />"; echo $row ['dirID']; echo "<br />"; echo $row['price']; echo "<br />"; echo $row['stock']; } }
-
I have followed everything that ManiacDan has suggested, the implementation of the mysqli_error() and the mysqli_insert_id() after the $r variable but to no avail, I get no error message but it does not write to the database.
-
1) Add.php doesn't contain the text "show.php," so I don't see where it's forwarding at all. All I see is add_image.php
2) The error on show.php means your query is wrong, echo mysql_error() to get the actual mysql error message.
3) Josh was right about your IF statement being wrong.
4) This line refers to $row but $row is not available at that time. Again, answering Josh's question would have fixed this:
<form enctype="multipart/form-data" action="add_image.php?prodID={$row['prodID']}" method="post" accept-charset="utf-8">
You are most likely not getting the variable that was pointed out in your first reply.
1. My own mistake, the name of the file being redirected is actually add_image.php not show.php which I soon realised. But they both have the same code regardless.
2. Believe or not, I have often chosen not to use mysqli_error() on much of my code, so should I implement a or die(mysqli_error()) after line 16 on add_image.php (what I originally stated as show.php).
3. Should I just remove the IF statement, as I tried that (I think I misunderstood what he meant in his post)?
4. My querystring, I guess I would have to remove the $row from it but if I plan to use mysql_insert_id(), what two parameters should I use inside it and then, how would I be able to retrieve that using the querystring from the form action URL?
-
i've tried that getting absolutely nowhere whatsoever
-
I have two pages - add_product.php and show.php
here is the code for add_product.php
<?php ini_set('display_errors',1); error_reporting(-1); require_once ('./includes/config.inc.php'); require_once (MYSQL); $add_cat_errors = array(); if ($_SERVER['REQUEST_METHOD'] == 'POST') { // Check for a name: if (empty($_POST['product'])) { $add_cat_errors['product'] = 'Please enter the name!'; } // Check for a description: if (empty($_POST['prod_descr'])) { $add_cat_errors['prod_descr'] = 'Please enter the description!'; } // Check for a category: if (!isset($_POST['cat']) || !filter_var($_POST['cat'], FILTER_VALIDATE_INT, array('min_range' => 1))) { $add_cat_errors['cat'] = 'Please select a category!'; } // Check for a price: if (empty($_POST['price']) || !filter_var($_POST['price'], FILTER_VALIDATE_FLOAT) || ($_POST['price'] <= 0)) { $add_cat_errors['price'] = 'Please enter a valid price!'; } // Check for a category: if (!isset($_POST['directory']) || !filter_var($_POST['directory'], FILTER_VALIDATE_INT, array('min_range' => 1))) { $add_cat_errors['directory'] = 'Please select a directory!'; } // Check for a stock: if (empty($_POST['stock']) || !filter_var($_POST['stock'], FILTER_VALIDATE_INT, array('min_range' => 1))) { $add_cat_errors['stock'] = 'Please enter the quantity in stock!'; } if (empty($add_cat_errors)) { $query = "INSERT INTO product (product, prod_descr, catID, price, dirID, stock) VALUES (?, ?, ?, ?, ?, ?)"; // Prepare the statement: $stmt = mysqli_prepare($dbc, $query); // For debugging purposes: // if (!$stmt) echo mysqli_stmt_error($stmt); // Bind the variables: mysqli_stmt_bind_param($stmt, 'sssssi', $name, $desc, $_POST['cat'], $_POST['price'], $_POST['directory'], $_POST['stock']); // Make the extra variable associations: $name = strip_tags($_POST['product']); $desc = strip_tags($_POST['prod_descr']); // Execute the query: mysqli_stmt_execute($stmt); if (mysqli_stmt_affected_rows($stmt) == 1) { // If it ran OK. // Print a message: echo '<h4>The product has been added!</h4>'; // Clear $_POST: $_POST = array(); // Clear $_FILES: $_FILES = array(); } else { // If it did not run OK. trigger_error('The product could not be added due to a system error. We apologize for any inconvenience.'); } } // End of $errors IF. } else { // Clear out the session on a GET request: } // End of the submission IF. require_once ('./includes/form_functions.inc.php'); ?> <form enctype="multipart/form-data" action="add_image.php?prodID={$row['prodID']}" method="post" accept-charset="utf-8"> <input type="hidden" name="MAX_FILE_SIZE" value="524288" /> Product<br /><?php create_form_input('product', 'text', $add_cat_errors); ?> Description<br /><?php create_form_input('prod_descr', 'textarea', $add_cat_errors); ?> Category<br /><select name="cat"<?php if (array_key_exists('cat', $add_cat_errors)); ?>> <option>Select One</option> <?php // Retrieve all the categories and add to the pull-down menu: $q = 'SELECT catID, cat FROM category ORDER BY cat ASC'; $r = mysqli_query ($dbc, $q); while ($row = mysqli_fetch_array ($r, MYSQLI_NUM)) { echo "<option value=\"$row[0]\""; // Check for stickyness: if (isset($_POST['cat']) && ($_POST['cat'] == $row[0]) ) echo ' selected="selected"'; echo ">$row[1]</option>\n"; } ?> </select><?php if (array_key_exists('cat', $add_cat_errors)) echo $add_cat_errors['cat']; ?> Price<br /><?php create_form_input('price', 'text', $add_cat_errors); ?> Directory<br /><select name="directory"<?php if (array_key_exists('directory', $add_cat_errors)); ?>> <option>Select One</option> <?php // Retrieve all the categories and add to the pull-down menu: $q = 'SELECT dirID, directory FROM directory ORDER BY directory ASC'; $r = mysqli_query ($dbc, $q); while ($row = mysqli_fetch_array ($r, MYSQLI_NUM)) { echo "<option value=\"$row[0]\""; // Check for stickyness: if (isset($_POST['directory']) && ($_POST['directory'] == $row[0]) ) echo ' selected="selected"'; echo ">$row[1]</option>\n"; } ?> </select><?php if (array_key_exists('directory', $add_cat_errors)) echo $add_cat_errors['directory']; ?> </select> <br /> Stock<br /><?php create_form_input('stock', 'text', $add_cat_errors); ?> <input type="submit" value="Add This Product" class="button" /> </fieldset> </form>
and here is the code for show.php
<?php ini_set('display_errors',1); error_reporting(-1); require_once ('./includes/config.inc.php'); require_once (MYSQL); $add_cat_errors = array(); if($id = isset($_GET['prodID'])) { $q = "SELECT `prodID`, `product`, `prod_descr`, `catID`, `dirID`, `price`, `stock` FROM product WHERE `prodID`='{$_GET['prodID']}'"; $r = mysqli_query($dbc, $q); while($row = mysqli_fetch_array($r)) { echo $row['product']; echo "<br />"; echo $row['prod_descr']; echo "<br />"; echo $row['catID']; echo "<br />"; echo $row ['dirID']; echo "<br />"; echo $row['price']; echo "<br />"; echo $row['stock']; } }
What I want is to try and retrieve the id of the last record inserted in add_product and pass it into show.php, but I get this error message.
An error occurred in script 'C:\Users\David Morgan\Desktop\WEBSITES\hairz_&_graces\site\admin\add_image.php' on line 16:mysqli_fetch_array() expects parameter 1 to be mysqli_result, boolean given
I am struggling to find out where I am going wrong at the moment, if anyone could please help me that would be really appreciated.
-
I have this code where I add records:
<?php ini_set('display_errors',1); error_reporting(-1); require_once ('./includes/config.inc.php'); require_once (MYSQL); $add_cat_errors = array(); if ($_SERVER['REQUEST_METHOD'] == 'POST') { // Check for a name: if (empty($_POST['product'])) { $add_cat_errors['product'] = 'Please enter the name!'; } // Check for a description: if (empty($_POST['prod_descr'])) { $add_cat_errors['prod_descr'] = 'Please enter the description!'; } // Check for a category: if (!isset($_POST['cat']) || !filter_var($_POST['cat'], FILTER_VALIDATE_INT, array('min_range' => 1))) { $add_cat_errors['cat'] = 'Please select a category!'; } // Check for a price: if (empty($_POST['price']) || !filter_var($_POST['price'], FILTER_VALIDATE_FLOAT) || ($_POST['price'] <= 0)) { $add_cat_errors['price'] = 'Please enter a valid price!'; } // Check for a category: if (!isset($_POST['directory']) || !filter_var($_POST['directory'], FILTER_VALIDATE_INT, array('min_range' => 1))) { $add_cat_errors['directory'] = 'Please select a directory!'; } // Check for an image: if (is_uploaded_file ($_FILES['image']['tmp_name']) && ($_FILES['image']['error'] == UPLOAD_ERR_OK)) { $file = $_FILES['image']; $size = ROUND($file['size']/1024); // Validate the file size: if ($size > 512) { $add_cat_errors['image'] = 'The uploaded file was too large.'; } // Validate the file type: $allowed_mime = array ('image/jpeg', 'image/JPG', 'image/jpg'); $allowed_extensions = array ('.jpg', 'jpeg'); $image_info = getimagesize($file['tmp_name']); $ext = substr($file['name'], -4); if ( (!in_array($file['type'], $allowed_mime)) || (!in_array($image_info['mime'], $allowed_mime) ) || (!in_array($ext, $allowed_extensions) ) ) { $add_cat_errors['image'] = 'The uploaded file was not of the proper type.'; } // Move the file over, if no problems: if (!array_key_exists('image', $add_cat_errors)) { // Create a new name for the file: $new_name = (string) sha1($file['name'] . uniqid('',true)); // Add the extension: $new_name .= ((substr($ext, 0, 1) != '.') ? ".{$ext}" : $ext); //$new_name = $dir . '/' . $new_name; $dest = "../db/images/$new_name"; // Move the file to its proper folder but add _tmp, just in case: //$dest = "../db/images/$new_name"; $dirs = array('full_heads', 'human_hair', 'lip_tattoos', 'ponytails', 'synthetic_hair'); if (move_uploaded_file($file['tmp_name'], $dest)) { // Store the data in the session for later use: $_SESSION['image']['new_name'] = $new_name; $_SESSION['image']['file_name'] = $file['name']; // Print a message: echo '<h4>The file has been uploaded!</h4>'; } else { trigger_error('The file could not be moved.'); unlink ($file['tmp_name']); } } // End of array_key_exists() IF. } elseif (!isset($_SESSION['image'])) { // No current or previous uploaded file. switch ($_FILES['image']['error']) { case 1: case 2: $add_cat_errors['image'] = 'The uploaded file was too large.'; break; case 3: $add_cat_errors['image'] = 'The file was only partially uploaded.'; break; case 6: case 7: case 8: $add_cat_errors['image'] = 'The file could not be uploaded due to a system error.'; break; case 4: default: $add_cat_errors['image'] = 'No file was uploaded.'; break; } // End of SWITCH. } // End of $_FILES IF-ELSEIF-ELSE. // Check for a stock: if (empty($_POST['stock']) || !filter_var($_POST['stock'], FILTER_VALIDATE_INT, array('min_range' => 1))) { $add_cat_errors['stock'] = 'Please enter the quantity in stock!'; } if (empty($add_cat_errors)) { $query = "INSERT INTO product (product, prod_descr, catID, price, dirID, image, stock) VALUES (?, ?, ?, ?, ?, ?, ?)"; // Prepare the statement: $stmt = mysqli_prepare($dbc, $query); // For debugging purposes: // if (!$stmt) echo mysqli_stmt_error($stmt); // Bind the variables: mysqli_stmt_bind_param($stmt, 'ssssssi', $name, $desc, $_POST['cat'], $_POST['price'], $_POST['directory'], $_SESSION['image']['new_name'], $_POST['stock']); // Make the extra variable associations: $name = strip_tags($_POST['product']); $desc = strip_tags($_POST['prod_descr']); // Execute the query: mysqli_stmt_execute($stmt); if (mysqli_stmt_affected_rows($stmt) == 1) { // If it ran OK. // Print a message: echo '<h4>The product has been added!</h4>'; // Clear $_POST: $_POST = array(); // Clear $_FILES: $_FILES = array(); // Clear $file and $_SESSION['image']: unset($file, $_SESSION['image']); } else { // If it did not run OK. trigger_error('The product could not be added due to a system error. We apologize for any inconvenience.'); unlink ($dest); } } // End of $errors IF. } else { // Clear out the session on a GET request: unset($_SESSION['image']); } // End of the submission IF. require_once ('./includes/form_functions.inc.php'); ?> <form enctype="multipart/form-data" action="add_product.php" method="post" accept-charset="utf-8"> <input type="hidden" name="MAX_FILE_SIZE" value="524288" /> Product<br /><?php create_form_input('product', 'text', $add_cat_errors); ?> Description<br /><?php create_form_input('prod_descr', 'textarea', $add_cat_errors); ?> Category<br /><select name="cat"<?php if (array_key_exists('cat', $add_cat_errors)); ?>> <option>Select One</option> <?php // Retrieve all the categories and add to the pull-down menu: $q = 'SELECT catID, cat FROM category ORDER BY cat ASC'; $r = mysqli_query ($dbc, $q); while ($row = mysqli_fetch_array ($r, MYSQLI_NUM)) { echo "<option value=\"$row[0]\""; // Check for stickyness: if (isset($_POST['cat']) && ($_POST['cat'] == $row[0]) ) echo ' selected="selected"'; echo ">$row[1]</option>\n"; } ?> </select><?php if (array_key_exists('cat', $add_cat_errors)) echo $add_cat_errors['cat']; ?> Price<br /><?php create_form_input('price', 'text', $add_cat_errors); ?> Directory<br /><select name="directory"<?php if (array_key_exists('directory', $add_cat_errors)); ?>> <option>Select One</option> <?php // Retrieve all the categories and add to the pull-down menu: $q = 'SELECT dirID, directory FROM directory ORDER BY directory ASC'; $r = mysqli_query ($dbc, $q); while ($row = mysqli_fetch_array ($r, MYSQLI_NUM)) { echo "<option value=\"$row[0]\""; // Check for stickyness: if (isset($_POST['directory']) && ($_POST['directory'] == $row[0]) ) echo ' selected="selected"'; echo ">$row[1]</option>\n"; } ?> </select><?php if (array_key_exists('directory', $add_cat_errors)) echo $add_cat_errors['directory']; ?> </select> Image<br /><?php // Check for an error: if (array_key_exists('image', $add_cat_errors)) { echo $add_cat_errors['image'] . '<br /><input type="file" name="image"/>'; } else { // No error. echo '<input type="file" name="image" />'; // If the file exists (from a previous form submission but there were other errors), // store the file info in a session and note its existence: if (isset($_SESSION['image'])) { echo "<br />Currently '{$_SESSION['image']['file_name']}'"; } } // end of errors IF-ELSE. ?> <br /> Stock<br /><?php create_form_input('stock', 'text', $add_cat_errors); ?> <input type="submit" value="Add This Product" class="button" /> </fieldset> </form>
What I want to achieve is this;
When a record is being inserted, a user will make a selection from the dropdown box with a list of directories. Then, a user will upload an image. When a image is uploaded and a directory is chosen, I want the image to be assigned to a specific directory from the list so when the record is inserted, that image will be placed in the specific directory. How do I achieve this please?
-
I have created a table called directories. Now basically I have two fields;
dirID (INT)
directory (VARCHAR)
now I am fully aware this might sound like more of an SQL question but I have three physical directories:
db/images/web1
db/images/web2
db/images/web3
I have inputted them into the database but I was just wondering, how could I created a page which links those records to a directory. I want to have each row hyperlinked, with the output page displaying list of contents for each directory. How can I possibly achieve this please?
-
thanks for that, i soon realised that it was only inputting six fields rather than seven.
-
At the moment I am getting this error message when I am trying to add a record:
mysqli_stmt_bind_param() [function.mysqli-stmt-bind-param]: Number of elements in type definition string doesn't match number of bind variablesBelow is my code
<?php ini_set('display_errors',1); error_reporting(-1); require_once ('./includes/config.inc.php'); require_once (MYSQL); $add_cat_errors = array(); if ($_SERVER['REQUEST_METHOD'] == 'POST') { // Check for a name: if (empty($_POST['product'])) { $add_cat_errors['product'] = 'Please enter the name!'; } // Check for a description: if (empty($_POST['prod_descr'])) { $add_cat_errors['prod_descr'] = 'Please enter the description!'; } // Check for a category: if (!isset($_POST['cat']) || !filter_var($_POST['cat'], FILTER_VALIDATE_INT, array('min_range' => 1))) { $add_cat_errors['cat'] = 'Please select a category!'; } // Check for a price: if (empty($_POST['price']) || !filter_var($_POST['price'], FILTER_VALIDATE_FLOAT) || ($_POST['price'] <= 0)) { $add_cat_errors['price'] = 'Please enter a valid price!'; } // Check for a category: if (!isset($_POST['directory']) || !filter_var($_POST['directory'], FILTER_VALIDATE_INT, array('min_range' => 1))) { $add_cat_errors['directory'] = 'Please select a directory!'; } // Check for an image: if (is_uploaded_file ($_FILES['image']['tmp_name']) && ($_FILES['image']['error'] == UPLOAD_ERR_OK)) { $file = $_FILES['image']; $size = ROUND($file['size']/1024); // Validate the file size: if ($size > 512) { $add_cat_errors['image'] = 'The uploaded file was too large.'; } // Validate the file type: $allowed_mime = array ('image/jpeg', 'image/JPG', 'image/jpg'); $allowed_extensions = array ('.jpg', 'jpeg'); $image_info = getimagesize($file['tmp_name']); $ext = substr($file['name'], -4); if ( (!in_array($file['type'], $allowed_mime)) || (!in_array($image_info['mime'], $allowed_mime) ) || (!in_array($ext, $allowed_extensions) ) ) { $add_cat_errors['image'] = 'The uploaded file was not of the proper type.'; } // Move the file over, if no problems: if (!array_key_exists('image', $add_cat_errors)) { // Create a new name for the file: $new_name = (string) sha1($file['name'] . uniqid('',true)); // Add the extension: $new_name .= ((substr($ext, 0, 1) != '.') ? ".{$ext}" : $ext); //$new_name = $dir . '/' . $new_name; $dest = "../db/images/$new_name"; // Move the file to its proper folder but add _tmp, just in case: //$dest = "../db/images/$new_name"; $dirs = array('full_heads', 'human_hair', 'lip_tattoos', 'ponytails', 'synthetic_hair'); if (move_uploaded_file($file['tmp_name'], $dest)) { // Store the data in the session for later use: $_SESSION['image']['new_name'] = $new_name; $_SESSION['image']['file_name'] = $file['name']; // Print a message: echo '<h4>The file has been uploaded!</h4>'; } else { trigger_error('The file could not be moved.'); unlink ($file['tmp_name']); } } // End of array_key_exists() IF. } elseif (!isset($_SESSION['image'])) { // No current or previous uploaded file. switch ($_FILES['image']['error']) { case 1: case 2: $add_cat_errors['image'] = 'The uploaded file was too large.'; break; case 3: $add_cat_errors['image'] = 'The file was only partially uploaded.'; break; case 6: case 7: case 8: $add_cat_errors['image'] = 'The file could not be uploaded due to a system error.'; break; case 4: default: $add_cat_errors['image'] = 'No file was uploaded.'; break; } // End of SWITCH. } // End of $_FILES IF-ELSEIF-ELSE. // Check for a stock: if (empty($_POST['stock']) || !filter_var($_POST['stock'], FILTER_VALIDATE_INT, array('min_range' => 1))) { $add_cat_errors['stock'] = 'Please enter the quantity in stock!'; } if (empty($add_cat_errors)) { $query = "INSERT INTO product (product, prod_descr, catID, price, dirID, image, stock) VALUES (?, ?, ?, ?, ?, ?, ?)"; // Prepare the statement: $stmt = mysqli_prepare($dbc, $query); // For debugging purposes: // if (!$stmt) echo mysqli_stmt_error($stmt); // Bind the variables: mysqli_stmt_bind_param($stmt, 'sssssi', $name, $desc, $_POST['cat'], $_POST['price'], $_POST['directory'], $_SESSION['image']['new_name'], $_POST['stock']); // Make the extra variable associations: $name = strip_tags($_POST['product']); $desc = strip_tags($_POST['prod_descr']); // Execute the query: mysqli_stmt_execute($stmt); if (mysqli_stmt_affected_rows($stmt) == 1) { // If it ran OK. // Print a message: echo '<h4>The product has been added!</h4>'; // Clear $_POST: $_POST = array(); // Clear $_FILES: $_FILES = array(); // Clear $file and $_SESSION['image']: unset($file, $_SESSION['image']); } else { // If it did not run OK. trigger_error('The product could not be added due to a system error. We apologize for any inconvenience.'); unlink ($dest); } } // End of $errors IF. } else { // Clear out the session on a GET request: unset($_SESSION['image']); } // End of the submission IF. require_once ('./includes/form_functions.inc.php'); ?> <form enctype="multipart/form-data" action="add_product.php" method="post" accept-charset="utf-8"> <input type="hidden" name="MAX_FILE_SIZE" value="524288" /> Product<br /><?php create_form_input('product', 'text', $add_cat_errors); ?> Description<br /><?php create_form_input('prod_descr', 'textarea', $add_cat_errors); ?> Category<br /><select name="cat"<?php if (array_key_exists('cat', $add_cat_errors)); ?>> <option>Select One</option> <?php // Retrieve all the categories and add to the pull-down menu: $q = 'SELECT catID, cat FROM category ORDER BY cat ASC'; $r = mysqli_query ($dbc, $q); while ($row = mysqli_fetch_array ($r, MYSQLI_NUM)) { echo "<option value=\"$row[0]\""; // Check for stickyness: if (isset($_POST['cat']) && ($_POST['cat'] == $row[0]) ) echo ' selected="selected"'; echo ">$row[1]</option>\n"; } ?> </select><?php if (array_key_exists('cat', $add_cat_errors)) echo $add_cat_errors['cat']; ?> Price<br /><?php create_form_input('price', 'text', $add_cat_errors); ?> Directory<br /><select name="directory"<?php if (array_key_exists('directory', $add_cat_errors)); ?>> <option>Select One</option> <?php // Retrieve all the categories and add to the pull-down menu: $q = 'SELECT dirID, directory FROM directory ORDER BY directory ASC'; $r = mysqli_query ($dbc, $q); while ($row = mysqli_fetch_array ($r, MYSQLI_NUM)) { echo "<option value=\"$row[0]\""; // Check for stickyness: if (isset($_POST['directory']) && ($_POST['directory'] == $row[0]) ) echo ' selected="selected"'; echo ">$row[1]</option>\n"; } ?> </select><?php if (array_key_exists('directory', $add_cat_errors)) echo $add_cat_errors['directory']; ?> </select> Image<br /><?php // Check for an error: if (array_key_exists('image', $add_cat_errors)) { echo $add_cat_errors['image'] . '<br /><input type="file" name="image"/>'; } else { // No error. echo '<input type="file" name="image" />'; // If the file exists (from a previous form submission but there were other errors), // store the file info in a session and note its existence: if (isset($_SESSION['image'])) { echo "<br />Currently '{$_SESSION['image']['file_name']}'"; } } // end of errors IF-ELSE. ?> <br /> Stock<br /><?php create_form_input('stock', 'text', $add_cat_errors); ?> <input type="submit" value="Add This Product" class="button" /> </fieldset> </form>
How can I please solve this? Because I have checked my code and I have no idea where I am going wrong
-
yes, I am just using it to sell products ONLY. that being said, a lot of the techniques I have used are derived from Larry Ullman's 2010 book "Effortless E-Commerce".
however, the IPN discussed in that book relates to subscription and renewal of content rather than buying physical products online so I may have to take a different approach. are they're any tutorials in relation to PayPal IPN's specifically for buying/selling physical products?
-
if you do not mind me asking, but where within my code should i use this statement please? reason being is that item.php primarily displays data with the aforementioned Buy Now and Add To Cart buttons.
-
I have somewhat of a dilemma.
When someone clicks on a Buy Now button and subsequently follows necessary steps to complete process of purchase, when that transaction is completed, in my product table, I want to subtract 1 from whatever value is in the field.
E.g. say product one is being purchased, prior to purchase in product table, there are 5 product one's in stock, when purchase is complete, subtract 1 from 5 (to get 4).
Here is my code
<?php $title = "Like This Product, Buy It NOW!!!"; require ('includes/config.inc.php'); include ('./includes/header.html'); require (MYSQL); include ('./includes/main.html'); if($id = isset($_GET['prodID'])) { $query = "SELECT `prodID`, `product`, `prod_descr`, `image`, `price` FROM product WHERE `prodID`='{$_GET['prodID']}'"; $r = mysqli_query($dbc, $query); $showHeader = true; echo "<div id='right'>"; while($row = mysqli_fetch_array($r)) { if($showHeader) { //Display category header echo "<h1>" . "<span>" . "# " . "</span>" . $row['product'] . "<span>" . " #" . "</span>" . "</h1>"; echo "<div id='item'>"; // div class 'item' echo "<div class='item_left'>"; echo "<p id='p_desc'>"; echo $row['prod_descr']; echo "</p>"; echo "<p>" . "<span>" . "£" . $row['price'] . "</span>" . "</p>"; echo "</div>"; echo "<div class='item_right'>"; echo "<img src='db/images/".$row['image']."' />"; $showHeader = false; echo "</div>"; ?> <p> <form target="paypal" action="https://www.sandbox.paypal.com/cgi-bin/webscr" method="post"> <input type="hidden" name="cmd" value="_s-xclick"> <input type="hidden" name="hosted_button_id" value="7UCL9YCYYXL3J"> <input type="hidden" name="item_name" value="<?php echo $row['product']; ?>"> <input type="hidden" name="item_number" value="<?php echo $row['prodID']; ?>"> <input type="hidden" name="amount" value="<?php echo $row['price']; ?>"> <input type="hidden" name="currency_code" value="GBP"> <input type="image" src="https://www.sandbox.paypal.com/en_US/i/btn/btn_cart_LG.gif" border="0" name="submit" alt="PayPal - The safer, easier way to pay online!"> <img alt="" border="0" src="https://www.sandbox.paypal.com/en_US/i/scr/pixel.gif" width="1" height="1"> </form> </p> <p> <form name="_xclick" action="https://www.paypal.com/cgi-bin/webscr" method="post"> <input type="hidden" name="cmd" value="_xclick"> <input type="hidden" name="business" value="me@mybusiness.com"> <input type="hidden" name="currency_code" value="GBP"> <input type="hidden" name="item_name" value="<?php echo $row['product']; ?>"> <input type="hidden" name="amount" value="<?php echo $row['price']; ?>"> <input type="image" src="http://www.paypal.com/en_US/i/btn/btn_buynow_LG.gif" border="0" name="submit" alt="Make payments with PayPal - it's fast, free and secure!"> </form> </p> <?php echo "</div>"; // End of div class 'item' $strSQL = "SELECT prodID, product, price, image FROM product ORDER BY RAND() LIMIT 1"; $objQuery = mysqli_query($dbc, $strSQL) or die ("Error Query [".$strSQL."]"); while($objResult = mysqli_fetch_array($objQuery)) { echo "<div class='love'>"; echo "<h6>Like this......you'll love this!!!</h6>"; echo "<ul>"; echo "<li>" . "<img src='db/images/" . $objResult['image'] . "' width='50' height='50' />" . "</li>"; echo "<br />"; echo "<li>" . "<a href='item.php?prodID={$objResult['prodID']}' title='{$objResult['product']}'>" . $objResult['product'] . "</a>" . " - " . "£" . $objResult['price'] . "</li>"; echo "</ul>"; echo "</div>"; } } } ?> <?php echo "</div>"; } include ('./includes/footer.html'); ?>
How is this achievable please?
-
its called catID and acts as a foreign key, derived from another table i created called category with fields catID(pk) and cat
-
Yesterday, I created a topic about how I could update records and I managed to achieve that successfully.
Now I have another dilemma.
When I have a specific record I want to update, I want to change a category ID of an product (e.g. change it from 1 to 2) but how do I go about doing this?
Here is my code thus far:
<?php require_once ('./includes/config.inc.php'); require_once (MYSQL); $id=$_GET['prodID']; $results = mysqli_query($dbc, "SELECT * FROM product WHERE prodID=".$_GET['prodID'].""); $row = mysqli_fetch_assoc($results); ?> <form action="" method='POST'> Product ID: <input type="text" value="<?php echo $row['prodID'];?>" name="prodID" /> <br /> Product: <input type="text" value="<?php echo $row['product'] ;?>" name="product" /> <br /> Product Description: <input type="text" value="<?php echo $row['prod_descr'] ;?>" name="prod_descr" /> <br /> Category: <select name="category"> <option value="<?php echo $row['catID'];?>"></option> </select> Price: <input type="text" value="<?php echo $row['price'] ;?>" name="price" /> <br /> In Stock: <input type="text" value="<?php echo $row['stock'] ;?>" name="stock" /> <br /> <br /><input type="submit" value="save" name="save"> </form> <?php if(isset($_POST['save'])) { $id = $_POST['prodID']; $product = $_POST['product']; $descr = $_POST['prod_descr']; $price = $_POST['price']; $stock = $_POST['stock']; // Update data $update = mysqli_query($dbc, "UPDATE product SET product='$product', prod_descr='$descr', price='$price', stock='$stock' WHERE prodID=".$_GET['prodID'].""); header( 'Location: update_save.php' ) ; } ?>
-
i now get an error message: undefined index id
i have tried using $id = ['prodID'] (prodID being the unique ID of the table)
and
$id = ['id']
but to no avail
-
I have three pages;
edit_records.php (list of records, user picks one ready to edit, works fine)
update.php (by in large works fine, heres the code for it anyway)
<?php require_once ('./includes/config.inc.php'); require_once (MYSQL); if($id = isset($_GET['prodID'])) { $query = "SELECT * FROM product WHERE prodID='{$_GET['prodID']}'"; $r = mysqli_query($dbc, $query); while ($row = mysqli_fetch_array($r)) { $id = $row['prodID']; $product = $row ['product']; ?> <form action="update_save.php" method="post"> ID: <input type="text" value="<?php echo $id;?>" name="id" disabled="disabled" /> Product: <input type="text" value="<?php echo $product;?>" name="product" /> <br /> <input type="submit" value="submit changes" /> </form> <?php } }
and finally
update_save.php (this is where the actual updating takes place)
<?php require_once ('./includes/config.inc.php'); require_once (MYSQL); $product = $_POST['product']; $query = "UPDATE product SET product = $product WHERE prodID = '$id'"; $r = mysqli_query($dbc, $query); echo 'Database Updated!!'; ?>
however, on line 19 of update save, i get this error;
An error occurred in script 'C:\Users\David Morgan\Desktop\WEBSITES\hairz_&_graces\site\admin\update_save.php' on line 9:Undefined variable: id
now I am aware i should really create a variable but since i already stored it in update.php, i had hoped it would work but it hasnt.
what should i do? help would be much appreciated please
-
not at the moment all i have is the home page where users will search from:
<?php $title = 'Home'; error_reporting(E_ALL ^ E_NOTICE); ini_set("display_errors", 1); require_once ('includes/config.inc.php'); require_once (MYSQL); include ('./includes/header.html'); include ('./includes/main.html'); ?> <div id="right"> <form id="frmSearch" method="post" action="result.php"> <input type="text" name="search" value="Search for a product..." onfocus="if(this.value==this.defaultValue)this.value='';" onblur="if(this.value=='')this.value=this.defaultValue;" id="txtSearch" /> <input type="submit" name="submit" value="Search" id="btnSearch" /> </form> <?php ?> <br /> <div id="shop"> <div class="shoprow"> <div class="shopcell"> <img src="includes/inc_pics/SCRUNCHIES.jpg" width="100" height="100" /> <div class="shopsubcell"> <li><a href="cat.php?catID=1">Human Hair</a></li> </div> </div> <div class="shopcell"> <img src="includes/inc_pics/SCRUNCHIES.jpg" width="100" height="100" /> <div class="shopsubcell"> <li><a href="cat.php?catID=2">Pony Tails</a></li> </div> </div> <div class="shopcell"> <img src="includes/inc_pics/SCRUNCHIES.jpg" width="100" height="100" /> <div class="shopsubcell"> <li><a href="cat.php?catID=3">Scrunchies</a></li> </div> </div> </div> <br /> <div class="shoprow"> <div class="shopcell"> <img src="includes/inc_pics/SCRUNCHIES.jpg" width="100" height="100" /> <div class="shopsubcell"> <li><a href="cat.php?catID=4">Full Heads</a></li> </div> </div> <div class="shopcell"> <img src="includes/inc_pics/SCRUNCHIES.jpg" width="100" height="100" /> <div class="shopsubcell"> <li><a href="cat.php?catID=5">Synthetic Hair</a></li> </div> </div> <div class="shopcell"> <img src="includes/inc_pics/SCRUNCHIES.jpg" width="100" height="100" /> <div class="shopsubcell"> <li><a href="cat.php?catID=6">Accessories</a></li> </div> </div> </div> <br /> <div class="shoprow"> <div class="shopcell"> <img src="includes/inc_pics/SCRUNCHIES.jpg" width="100" height="100" /> <div class="shopsubcell"> <li><a href="cat.php?catID=7">Contact Lenses</a></li> </div> </div> <div class="shopcell"> <img src="includes/inc_pics/SCRUNCHIES.jpg" width="100" height="100" /> <div class="shopsubcell"> <li><a href="cat.php?catID=8">Lip Tattoos</a></li> </div> </div> </div> </div> </div> <?php include ('./includes/footer.html'); ?>
and the result.php (which is almost redundant at the moment:
<?php $title = "Pick your Product"; //Set number of columns to use $maxCols = 3; error_reporting(E_ALL ^ E_NOTICE); ini_set("display_errors", 1); require_once ('./includes/config.inc.php'); require_once (MYSQL); include ('./includes/header.html'); include ('./includes/main.html'); { //Create and run query to get product category names for the selected cat ID $query = "SELECT `product`.`prodID`, `product`.`product`, `category`.`cat`, `product`.`prod_descr`, `category`.`cat_descr`, `product`.`price`, `product`.`image`, `product`.`stock` FROM `product` LEFT JOIN `category` ON `product`.`catID` = `category`.`catID` WHERE `product`.`product` LIKE 's%' ORDER BY `product`.`product`"; $r = mysqli_query($dbc, $query); $showHeader = true; echo "<div id='right'>"; while($row = mysqli_fetch_array($r)) { if($showHeader) { echo "<table class='table-container'>"; $showHeader = false; //Set index var to track record count $recIdx = 0; $recIdx++; //Open new row if needed if($recIdx % $maxCols == 1) { echo "<tr>"; } } //Display product echo "<td>"; echo "<img src='db/images/".$row['image']."' height=150px width=150px /><br>"; echo "<li>" . "<a href='item.php?prodID={$row['prodID']}' title='{$row['product']}'>" . $row['product'] . "</a>" . "</li>"; echo "<span>" . "£". $row['price'] . "</span>"; echo "<li>" . $row['cat'] . "</li>"; if ($row['stock'] < 2) // If there less than two items in stock!! { echo " (<span> Low in Stock! </span>) "; } elseif ($row ['stock'] = 0) { echo "Sorry, currently not available but we will try hard to get more in!"; } echo "</td>"; //Close row if needed if($recIdx % $maxCols == 1) { echo "</tr>"; } } //Close last row if needed if($recIdx % $maxCols == 0) { echo "</tr>"; } //Close table & div } echo "</table>"; echo "</div>"; include ('./includes/footer.html'); ?>
note in the query code for the SQL I have made use of the LIKE operator (WHERE product LIKE '$').
-
I am currently looking to have two pages, one with a search form (textbox and button) and output the results (from database table) based on the strings input to another page.
how do i achieve this? i just want something very simple nothing too complex and advanced please
-
I am still confused, should I just have the $_POST("myDirs") with an IF statement applied at the beginning and where should my array list of directories go?
Also
On the script that receives the form to be processed, you'll need to prepare the collection of the dir_name.1) you'll want to verify the upload directory submitted by the form is a folder that's actually permitted.
2) you'll want to add that name into the database so all your calls are congruent, and
when should I verify the upload directory?
-
i'm really confused as to where to embed the $_POST['myDirs'], I did try and include it in some form to this bit of the code but to no avail
$dest = "../db/images/$new_name";
-
I have applied this glob_onlydir facility into my code, inside my form
<select name="myDirs"> <option value="" selected="selected">Select a folder</option> <?php $dirs = glob("../db/images/*", GLOB_ONLYDIR); foreach($dirs as $val){ echo '<option value="'.$val.'">'.$val."</option>\n"; } ?> </select>
it reads all of the directories, based on those I have chosen inside the images directory, however, is it possible to workaround this with my code to insert it alongside when I upload image or should i go down the route of using an array and looping through there, if so, how can I achieve this please?
-
I have some code where i input data and upload images to a database (working 100% correctly)
However I want to expand on it - what I want is when a user uploads an image of their choice, to assign it to a directory of their choosing.
Then, when input is successful, write the directory of where the image is stored (not just the image) to the database.
Here is the code;
<?php ini_set('display_errors',1); error_reporting(-1); require_once ('./includes/config.inc.php'); require_once (MYSQL); $add_cat_errors = array(); if ($_SERVER['REQUEST_METHOD'] == 'POST') { // Check for a name: if (empty($_POST['product'])) { $add_cat_errors['product'] = 'Please enter the name!'; } // Check for a description: if (empty($_POST['prod_descr'])) { $add_cat_errors['prod_descr'] = 'Please enter the description!'; } // Check for a category: if (!isset($_POST['cat']) || !filter_var($_POST['cat'], FILTER_VALIDATE_INT, array('min_range' => 1))) { $add_product_errors['cat'] = 'Please select a category!'; } // Check for a price: if (empty($_POST['price']) || !filter_var($_POST['price'], FILTER_VALIDATE_FLOAT) || ($_POST['price'] <= 0)) { $add_cat_errors['price'] = 'Please enter a valid price!'; } // Check for an image: if (is_uploaded_file ($_FILES['image']['tmp_name']) && ($_FILES['image']['error'] == UPLOAD_ERR_OK)) { $file = $_FILES['image']; $size = ROUND($file['size']/1024); // Validate the file size: if ($size > 512) { $add_cat_errors['image'] = 'The uploaded file was too large.'; } // Validate the file type: $allowed_mime = array ('image/jpeg', 'image/JPG', 'image/jpg'); $allowed_extensions = array ('.jpg', 'jpeg'); $image_info = getimagesize($file['tmp_name']); $ext = substr($file['name'], -4); if ( (!in_array($file['type'], $allowed_mime)) || (!in_array($image_info['mime'], $allowed_mime) ) || (!in_array($ext, $allowed_extensions) ) ) { $add_cat_errors['image'] = 'The uploaded file was not of the proper type.'; } // Move the file over, if no problems: if (!array_key_exists('image', $add_cat_errors)) { // Create a new name for the file: $new_name = (string) sha1($file['name'] . uniqid('',true)); // Add the extension: $new_name .= ((substr($ext, 0, 1) != '.') ? ".{$ext}" : $ext); // Move the file to its proper folder but add _tmp, just in case: $dest = "../db/images/$new_name"; if (move_uploaded_file($file['tmp_name'], $dest)) { // Store the data in the session for later use: $_SESSION['image']['new_name'] = $new_name; $_SESSION['image']['file_name'] = $file['name']; // Print a message: echo '<h4>The file has been uploaded!</h4>'; } else { trigger_error('The file could not be moved.'); unlink ($file['tmp_name']); } } // End of array_key_exists() IF. } elseif (!isset($_SESSION['image'])) { // No current or previous uploaded file. switch ($_FILES['image']['error']) { case 1: case 2: $add_cat_errors['image'] = 'The uploaded file was too large.'; break; case 3: $add_cat_errors['image'] = 'The file was only partially uploaded.'; break; case 6: case 7: case 8: $add_cat_errors['image'] = 'The file could not be uploaded due to a system error.'; break; case 4: default: $add_cat_errors['image'] = 'No file was uploaded.'; break; } // End of SWITCH. } // End of $_FILES IF-ELSEIF-ELSE. // Check for a stock: if (empty($_POST['stock']) || !filter_var($_POST['stock'], FILTER_VALIDATE_INT, array('min_range' => 1))) { $add_cat_errors['stock'] = 'Please enter the quantity in stock!'; } if (empty($add_cat_errors)) { $query = "INSERT INTO product (product, prod_descr, catID, price, image, stock) VALUES (?, ?, ?, ?, ?, ?)"; // Prepare the statement: $stmt = mysqli_prepare($dbc, $query); // For debugging purposes: // if (!$stmt) echo mysqli_stmt_error($stmt); // Bind the variables: mysqli_stmt_bind_param($stmt, 'sssssi', $name, $desc, $_POST['cat'], $_POST['price'], $_SESSION['image']['new_name'], $_POST['stock']); // Make the extra variable associations: $name = strip_tags($_POST['product']); $desc = strip_tags($_POST['prod_descr']); // Execute the query: mysqli_stmt_execute($stmt); if (mysqli_stmt_affected_rows($stmt) == 1) { // If it ran OK. // Print a message: echo '<h4>The product has been added!</h4>'; // Clear $_POST: $_POST = array(); // Clear $_FILES: $_FILES = array(); // Clear $file and $_SESSION['image']: unset($file, $_SESSION['image']); } else { // If it did not run OK. trigger_error('The product could not be added due to a system error. We apologize for any inconvenience.'); unlink ($dest); } } // End of $errors IF. } else { // Clear out the session on a GET request: unset($_SESSION['image']); } // End of the submission IF. require_once ('./includes/form_functions.inc.php'); ?> <form enctype="multipart/form-data" action="add_product.php" method="post" accept-charset="utf-8"> <input type="hidden" name="MAX_FILE_SIZE" value="524288" /> Product<br /><?php create_form_input('product', 'text', $add_cat_errors); ?> Description<br /><?php create_form_input('prod_descr', 'textarea', $add_cat_errors); ?> Category<br /><select name="cat"<?php if (array_key_exists('cat', $add_cat_errors)); ?>> <option>Select One</option> <?php // Retrieve all the categories and add to the pull-down menu: $q = 'SELECT catID, cat FROM category ORDER BY cat ASC'; $r = mysqli_query ($dbc, $q); while ($row = mysqli_fetch_array ($r, MYSQLI_NUM)) { echo "<option value=\"$row[0]\""; // Check for stickyness: if (isset($_POST['cat']) && ($_POST['cat'] == $row[0]) ) echo ' selected="selected"'; echo ">$row[1]</option>\n"; } ?> </select><?php if (array_key_exists('cat', $add_cat_errors)) echo $add_product_errors['cat']; ?> Price<br /><?php create_form_input('price', 'text', $add_cat_errors); ?> Image<br /><?php // Check for an error: if (array_key_exists('image', $add_cat_errors)) { echo $add_cat_errors['image'] . '<br /><input type="file" name="image"/>'; } else { // No error. echo '<input type="file" name="image" />'; // If the file exists (from a previous form submission but there were other errors), // store the file info in a session and note its existence: if (isset($_SESSION['image'])) { echo "<br />Currently '{$_SESSION['image']['file_name']}'"; } } // end of errors IF-ELSE. ?> <br /> <select name="select"> <option value="full_heads">db/images/full_heads</option> <option value="human_hair">db/images/human_hair</option> </select> Stock<br /><?php create_form_input('stock', 'text', $add_cat_errors); ?> <input type="submit" value="Add This Product" class="button" /> </fieldset> </form>
How do I achieve this?
-
that last trick (with the sssi) worked brilliantly, as i said before i am still quite new to php and i am trying my hardest to pick things up as i go along.
big thank you to everyone for their help, i also had another minor problem (trying to apply an extension to end of image when uploaded) but i got that working too at the same time which is a bonus so big thanks all round!!!!!
need help on how to utilise paypal with my website
in PHP Coding Help
Posted
I have spent the last few months working on an e-commerce project. However, I am a complete newbie in regards to setting up paypal completely with an e-commerce site.
I have set up a Paypal developer account and I know how to create test accounts in Sandbox. But other than that, I am clueless as to what to do.
I have my orders table set up, here is my create table code for that (I know its NOT php);
So thats my table "orders". I have an item page which users view an item based on a search they make. A friend of mine also gave me some IPN code and a file called toolbox.php but I am struggling to get my head around it.
Since I am selling physical products and NOT digital items, how do I go about doing this please? I have attached the essential files to this topic.
18489_.php
18490_.php
18491_.php