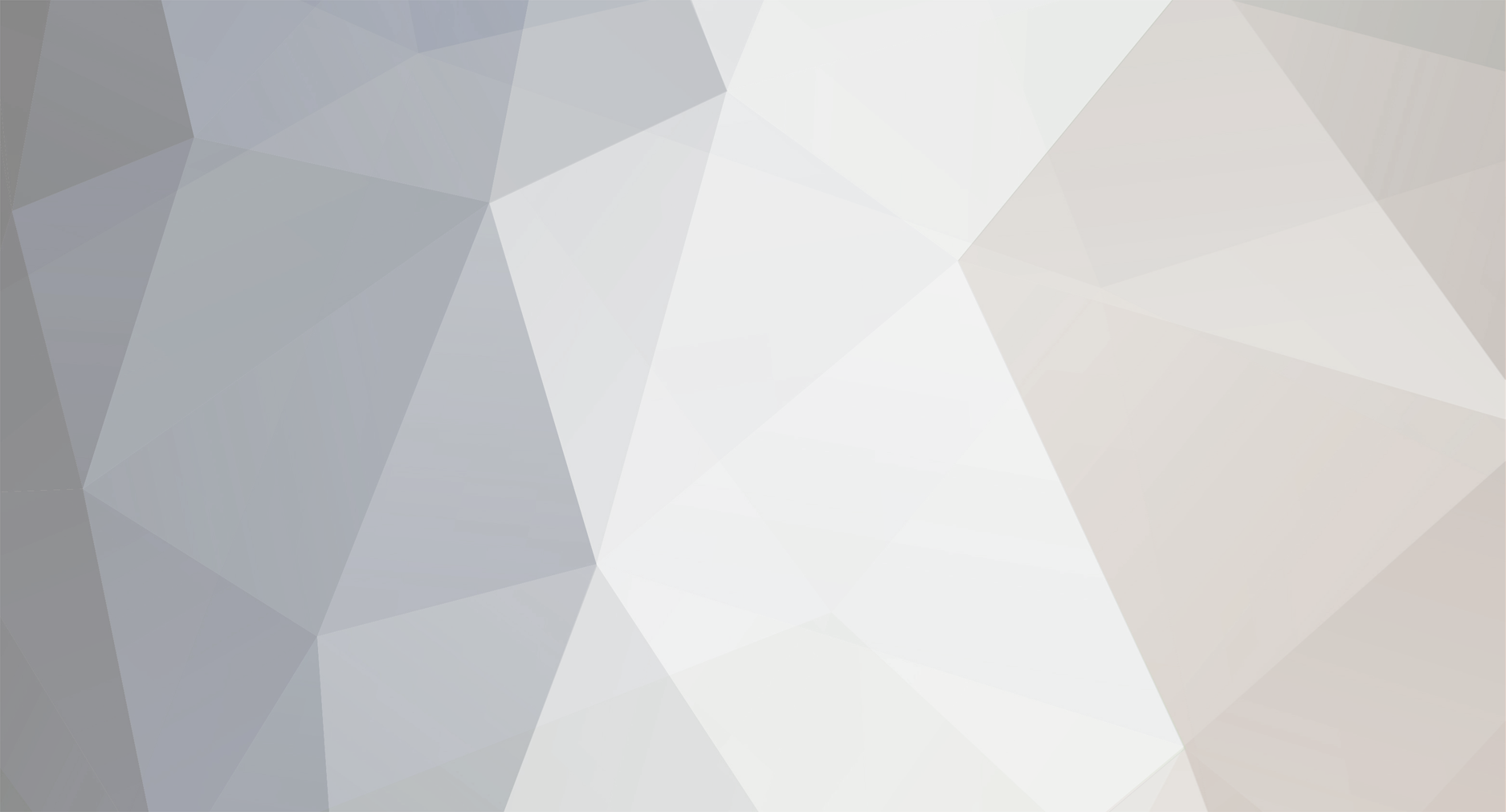
ejay
-
Posts
21 -
Joined
-
Last visited
Never
Posts posted by ejay
-
-
No that's not what I meant. It seems that you're not applying PRG (Post-Redirect-Get) pattern, if I understand correctly. Please post the form handling code.
-
you were misreading the array structure. The first line in var_dump output in reply # 3 is "array(1) {" which indicates that the outer array has only one element in it, so you can't do $matches[1]
Please mark solved if it is solved
-
headers don't have to do anything with it. You will not be using the div's ID but a named anchor just above the target div instead. Say you want to "redirect (as you say)" the user to a specific div element with id=line1, you do something like this
//page test.php //... <a name="line1" /> <div id="line1>hello</div>
and then from the form submit handling script, redirect user to
test.php#line1
instead of just
test.php
The div's id is not bound to be same as named anchor's name.
-
If you are using
header("Location: .....");
after
setCookie(...)
, cookie might not be set in all cases
-
This might work
-------------------------------------- ATSnotes.php class ATSnotes extends ATSdb //... function getNotes($stationID = 0) { $col = $this->getColumnNames(); if (count($col) > 0) { //... $yada = $this->query("SELECT * FROM " . $this->getTableName() . ($stationID > 0? " WHERE fldStationID = $stationID " : '') . " ORDER BY $col[2] DESC, $col[2] DESC"); //...
_________________________________________________ ____________________________________ 3. stationNotesEditDel.php //... //... unset ($dada); $dada = $db->getNotes($StationID); //... //...
-
try adding
return true;
as the first line of
validateForm()
or just try removing
onsubmit = \"return validateForm()\"
from your string
-
you need to do
echo $match[0][1];
instead of
echo $match[1];
-
-
you may need to do
<?php $protocol = (!empty($_SERVER['HTTPS']) && $_SERVER['HTTPS'] !== 'off' || $_SERVER['SERVER_PORT'] == 443) ? "https://" : "http://"; $domain_name = $_SERVER['HTTP_HOST']; $site_url = $protocol.$domain_name; ?> <link rel="stylesheet" href="<?php echo $site_url ?>/css/style.css">
-
//code to divide array into chunks of size 10 $group_size = 10; $input_array = array_chunk($input_array, $group_size, true)
-
Pls post your table HTML (not php code that generates the table) and the PHP code that does database update.
-
it happens sometimes
-
replace
if(mysql_num_rows($blog_sql==0)){
with
if(mysql_num_rows($blog_sql)==0){
-
<?php if ($_SERVER['REQUEST_METHOD'] == 'POST') { if (isset($_POST["pokemon_id"])) { $result = mysql_query("SELECT * FROM firemap WHERE id = " . intval($_POST["pokemon_id"])); $row = mysql_fetch_assoc($result); // or whatever you do with plain mysql functions if (isset($row['id'])) { echo "<div class=\"pokemonalign\">"; echo $row['image']; echo "</br>"; echo 'You have captured Wild'; echo $row['name'] . ""; echo "</div>"; $add = mysql_query("INSERT INTO pokemon (pokedex_id, owner_id, type, nickname) VALUES ('$row[id]', '$id', '1', '$row[name]')"); } } } else { $map = rand(1, 10); $result = mysql_query("SELECT * FROM firemap ORDER BY RAND() LIMIT 1"); while ($row = mysql_fetch_array($result)) { if ($map == 1) { echo "<br />"; echo '<img src="' . $row['image'] . '" alt="" />'; echo "<br />"; echo "A wild "; echo $row['name'] . " Appeared. <br /> Level: " . $row['level']; echo "<br />"; echo "<form action='map1.php' method='post'>"; echo "<input type='hidden' name='pokemon_id' value='$row[id]' />"; echo "<input type='submit' class='catchpokemon' value='Catch $row[name]' />"; echo "</form>"; } else { echo "<div class=\"pokemontext\">"; echo "<b>No more wild Pokémon appeared.</b>"; echo "<br />"; echo "<i>Keep moving around to find one.</i>"; echo "</div>"; } } } ?>
-
Following jesirose's suggestion, here's what you want (I'm bored to hell)
<?php if (isset($_POST["pokemon_id"])) { $result = mysql_query("SELECT * FROM firemap WHERE id = " . intval($_POST["pokemon_id"])); $row = mysql_fetch_assoc($result); // or whatever you do with plain mysql functions if (isset($row['id'])) { echo "<div class=\"pokemonalign\">"; echo $row['image']; echo "</br>"; echo 'You have captured Wild'; echo $row['name'] . ""; echo "</div>"; $add = mysql_query("INSERT INTO pokemon (pokedex_id, owner_id, type, nickname) VALUES ('$row[id]', '$id', '1', '$row[name]')"); } } $map = rand(1, 10); $result = mysql_query("SELECT * FROM firemap ORDER BY RAND() LIMIT 1"); while ($row = mysql_fetch_array($result)) { if ($map == 1) { echo "<br />"; echo '<img src="' . $row['image'] . '" alt="" />'; echo "<br />"; echo "A wild "; echo $row['name'] . " Appeared. <br /> Level: " . $row['level']; echo "<br />"; echo "<form action='map1.php' method='post'>"; echo "<input type='hidden' name='pokemon_id' value='$row[id]' />"; echo "<input type='submit' class='catchpokemon' value='Catch $row[name]' />"; echo "</form>"; } else { echo "<div class=\"pokemontext\">"; echo "<b>No more wild Pokémon appeared.</b>"; echo "<br />"; echo "<i>Keep moving around to find one.</i>"; echo "</div>"; } } ?>
-
Ok I got it resolved. It had nothing to do with ffmpeg.
Originally the "$thumb" and "$source" strings were different than my original post. The string were in following form (notice the quotes)
//original dev's code format $source = '"/home/test1/abc.mov"'; $thumb = '"/home/test1/thumb.jpg"';
Something on the server got updated (by admins) and the code stopped working :|
I hope it helps somebody somehow.
thanks.
-
I have been under the impression that it's the other way around
-
thanks for the quick reply.
here's the code (I'm debugging atm)
if (file_exists($thumb)) { echo "<pre>thumb exists</pre>"; } else { echo "<pre>thumb can not be accessed</pre>"; }
and I always get "thumb can not be accessed"
-
I think you want
if(isset($_POST["$row[name]"]))
instead of
if(isset($_POST['$row[name]']))
if this forum is not replacing your quotes.
-
Hello,
I'm having a very strange issue which i'm unable to identify/resolve.
I'm extracting an image using ffmpeg using following command
$source = '/home/test1/abc.mov'; $thumb = '/home/test1/thumb.jpg'; system('ffmpeg -i "' . $source . '" -ss 6 -vcodec mjpeg -f image2 -s 180x100 ' . $thumb);
This piece of code works fine and I can see the file, thumb.jpg, in the FTP fine. But if I run a
file_exists($thumb);
right after the above piece of code, PHP can't find the file. The image is created as is a valid jpg file (displays fine if downloaded via FTP)
I'm able to access all other files in '/home/test1/' directory using file_get_contents(); and file_exists() without any issues.
Any help would be appreciated.
thanks
automatically moving to a div
in PHP Coding Help
Posted
It is. After having a look at your code (which is not complete, so the solution will be a guess), I think you'll need to do following 2 steps
I hope this works.