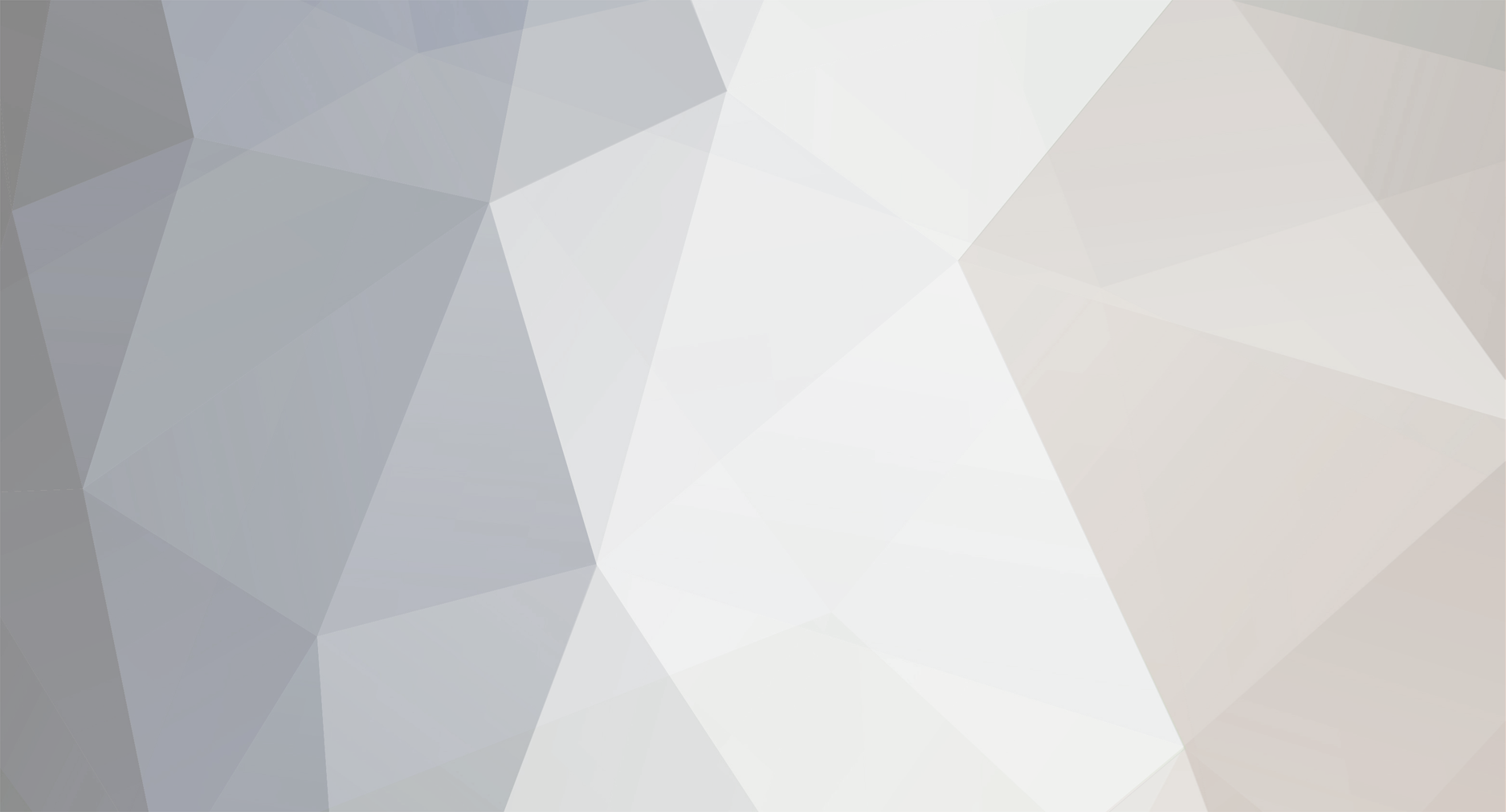
antmeeks
-
Posts
21 -
Joined
-
Last visited
Posts posted by antmeeks
-
-
Have you looked at Doctrine's DBAL?
Yes, I have... And for my needs, that's like bringing a bulldozer to dig a flower bed...
I'm really kinda looking for something more compact & straightforward... more along the lines of ezSQL (http://justinvincent.com/ezsql).
-
I'm making the switch from years of using plain-jane 'deprecated' MySQL functions to PDO in a lot of my scripts.
Consequently, I'm looking for a good, well-known & well-liked PDO wrapper / extension class.
Something that automates some common db access functions (CRUD), handles try/catch/exceptions, and keeps track of metrics (query count, memory usage, etc.)...
If anyone can point me in a direction, I'd really appreciate it!!
-
nothing should be before your html tag, except your doctype
+100
I do all of my processing before the output. Only echo'ing inside of HTML.
+1000
-
#1 can be easily solved with sticking the functions in a file in a well-known place, but #2 is actually one of the few decent reasons.
I did have them organized in a separate files, but wanted to eliminate as many manual includes/requires as possible.
Use static methods, not instance methods. Make the class a mere container of functions, not an object of sorts.
Sigh... Yes, you are probably right - that's the best approach... I guess I was just trying to be too clever... I suspected it was dumb, which is why I posted it, lol.
-
Lol, I knew that question would come up...
I have two reasons for doing this:
1. To keep the functions organized in a meaningful way.
2. To load the files dynamically with spl_autoload_register().
-
So I've decided to put several disparate but related functions into helper classes. Here's an example of what such a class would look like (with obviously more involved methods), and I'd like a critique on it's structure.
class MyHelpers { public $str; public $Array; private $Params; public function __construct($method, $Params) { $method = '_' . $method; $this->Params = (is_array($Params)) ? $Params : NULL; $result = (method_exists($this, $method)) ? $this->$method() : NULL; (is_array($result)) ? $this->Array = $result : $this->str = $result; } private function _outputString() { if(is_array($this->Params)) : return (isset($this->Params['str'])) ? $this->Params['str'] : NULL; endif; } private function _makeArray() { if(is_array($this->Params)) : $str = (isset($this->Params['array'])) ? $this->Params['array'] : NULL; return explode(',', $str); endif; } }
And here's how you would instantiate and use it:
$GetResult = new MyHelpers('outputString', array('str'=>'This is a string.')); $str = $GetResult->str; $GetResult = new MyHelpers('makeArray', array('array'=>'1,2,3')); $Array = $GetResult->Array;
Thoughts?
-
You just need to write a recursive file finder/includer to traverse the subdirectories.
There's a ton of them out there already written... just google it.
-
Doh! I just figured that out right before your post....
You know it's getting late when you post in a forum before even trying it out first...
What I wanted to do was nest the arrays into one var - which, as you say, my original post was a completely valid example of:
$d=$Array3[$Array2[$Array1[$a]]];
Time for bed.
-
Sorry, I guess I wasn't clear...
I'm not trying to make a multidimensional array out of 3 other arrays, I'm trying to consolidate the compounding of multiple array key values into one variable.
Literal from my example:
variable '$b' = the value of array1's key that equals whatever is assigned to '$a':
$b=$Array1[$a]
variable '$c' = the value of array2's key named the value of variable '$b':
$c=$Array2[$b]
variable '$d' = the value of array3's key named the value of variable '$c':
$d=$Array3[$c]
So... is it possible to build up the value of var $d with one statement instead of 3?
-
The most aesthetically pleasing thing is to keep all your scripting out of your html.
You could do something like this:
<script language="php">include('my_fantastic_script.php');</script> <html> <head> <title><?=$title?></title> <link rel="stylesheet" href="<?=$stylesheet?>" type="text/css" /> <script type="text/javascript" src="<?=$javascript?>"></script> </head> <body> <div id="wrapper"> <div id="left_col"> <h1><?=$h1_title?></h1> <?=$content?> </div> <div id="right_col"> <?=col_content?> </div> </div> </body> </html>
-
These statements...
$b=$Array1[$a]; $c=$Array2[$b]; $d=$Array3[$c];
combined into one statement like:
$d=$Array3[$Array2[$Array1[$a]]]
???
I know my example isn't valid, but is it possible to do this kind of consolidation?
-
If all your trying to do is pass a value from one page to the next, you should do that in a SESSION var, not POST and definitely not GET.
-
This is why I hate Wordpress... it's promotion of spaghetti-style coding. Ugh, it hurts my eyes to see all that php inline with html.
-
@ thorpe: I'm curious about Git... Can it integrate with Eclipse like Subversion?
-
Subversion. http://subversion.apache.org/features.html
Quoted from their site:
"File locking.
Subversion supports (but does not require) locking files so that users can be warned when multiple people try to edit the same file. A file can be marked as requiring a lock before being edited, in which case Subversion will present the file in read-only mode until a lock is acquired."
-
How do you keep overwriting each other's work? Are you not using a check out/in system... versioning... CVS... a repository...?
-
Ok, took a nap & solved my own problem...
Forget about all the other stuff about turning a string into a var, etc.
Wrote this function to run on my original array and it works exactly as I want:
function arrayGetNestedByInt($int, $Array) { foreach ($Array as $key => $val) { if(array_key_exists('children', $Array[$key])) { if($key == $int) return $Array[$key]['children']; else return arrayGetNestedByInt($int, $Array[$key]['children']); } } }
It's so stupidly obvious I can't believe it took me this long to get there. Sheesh, I need to go to bed earlier at night...
What's even worse is that no one here even bothered with it... I hope I joined the right forum.
-
No input at all from anyone on this?
Is it because I'm a newb on this forum?
-
Ok so with the "NewArray" above, I'm running this loop:
$str = NULL; foreach ($NewArray as $key => $var) { if(is_integer($var)) $var = "[".$var."]"; else $var = "['".$var."']"; $var = str_ireplace('id', 'children', $var); $str .= $var; } $strfinal = '$'."Array$str";
Which returns this string:
"$Array[5]['children'][7]['children']"
So that string is exactly the nested array I want to access, but how do I convert it to a var?
I tried a variable variable
$FinalArray = $$strfinal;
But that doesn't work.
Also for S&G, I tried eval, but no luck there either.
So can I get any pointers on how to convert the string above to a var?
-
So I'm trying to write a function that returns a nested array from a nested index in a multidimensional array & I'm having problems...
The array:
$Array = Array ( [1] => Array ( [id] => 1 [parent] => 0 ) [5] => Array ( [id] => 5 [parent] => 0 [children] => Array ( [7] => Array ( [id] => 7 [parent] => 5 [children] => Array ( [9] => Array ( [id] => 9 [parent] => 7 ) [12] => Array ( [id] => 12 [parent] => 7 ) ) ) ) ) [2] => Array ( [id] => 2 [parent] => 0 [children] => Array ( [3] => Array ( [id] => 3 [parent] => 2 ) ) ) )
What I want to do is create a function that takes an int and returns the children for that index.
For example, I could pass 7 and get back
$Array[5]['children'][7]['children']
or pass 5 and get just
$Array[5]['children']
i've gotten as far as this function:
function searchArray($int, $Array, $strict=false, $path=array()) { if( !is_array($Array) ) { return false; } foreach( $Array as $key => $val ) { if(is_array($val) && $subPath = searchArray($int, $val, $strict, $path) ) { $path = array_merge($path, array($key), $subPath); return $path; } elseif ((!$strict && $val == $int) || ($strict && $val === $int) ) { $path[] = $key; return $path; } } return false; } $NewArray = searchArray(7, $Array);
But it just returns this, which may be helpful, I'm just not sure how to iterate it into the result I'm looking for as stated above:
$NewArray = Array ( [0] => 5 [1] => children [2] => 7 [3] => id )
Any ideas? Thanks!
Help With 1 To Many Join
in MySQL Help
Posted
I've been stuck on this for a couple of hours now & I'd really appreciate some help(!)
I have 2 tables:
What I'm trying to do is some sort of join statement like:
select * from entries where status = 1 and type = term
join name,value from entry_text where entry_id = entries.id and language = en
order by entries.order, entries.date ASC
where I end up with an associative result, indexed by entries.id with the result from entry_text being a name/val pair nested array:
Doable...?
(sorry for the wonky formatting - I can't get the editor to preserve my spacing)