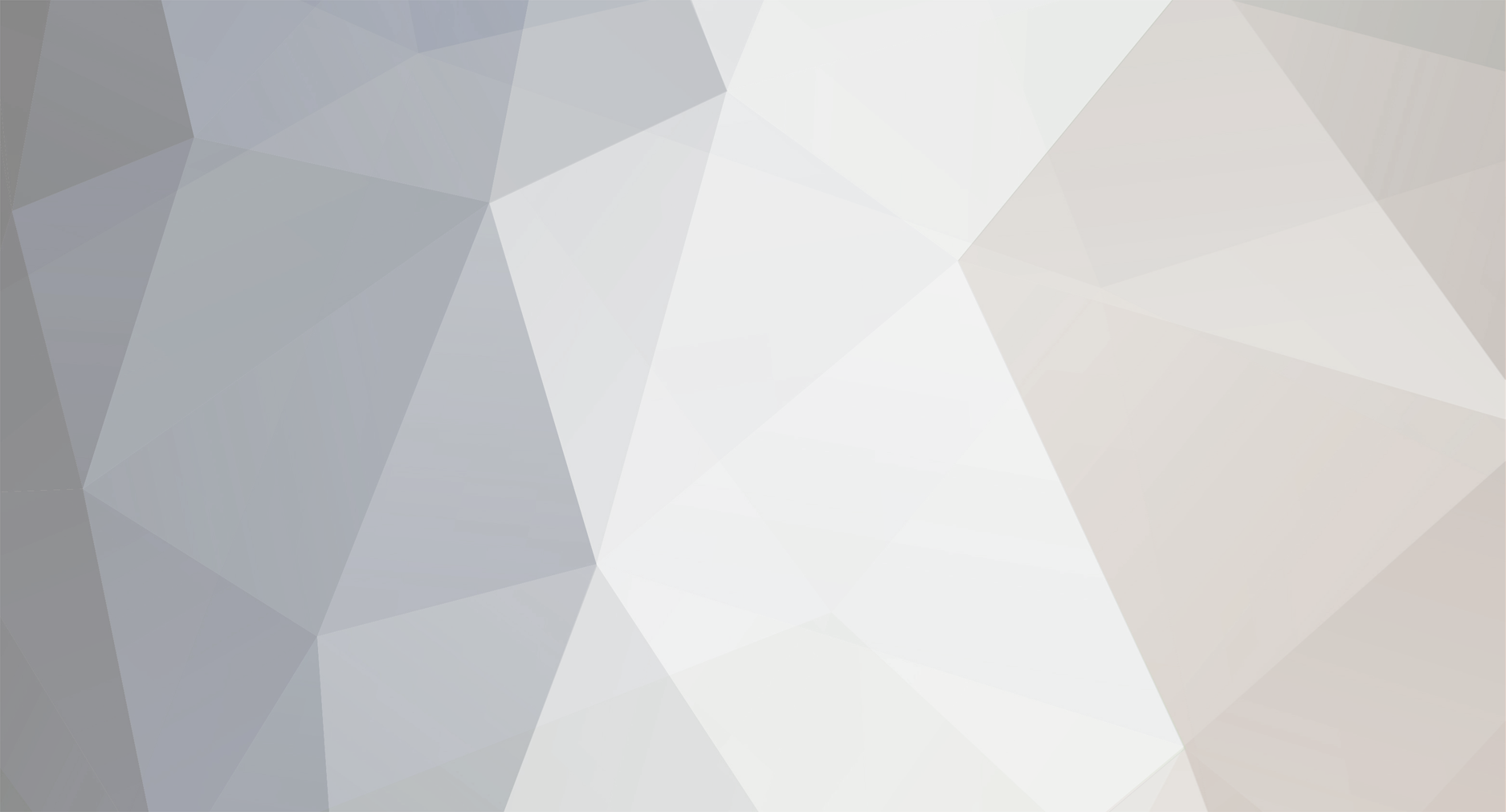
TheMiggyDgz
-
Posts
6 -
Joined
-
Last visited
Never
Posts posted by TheMiggyDgz
-
-
Have you heard of AJAX?
Basically, it's Javascript that calls a php file, and uses the output.
You would have the javascript call the ajax function when text is typed into the textbar, and when @ is found in the text.
Isolate the text directly adjacent to the @, send it to the function. PHP would then search the database for similar results, and return them. Javascript would update the screen with the suggestions.
I had some scripts with AJAX and jQuery and another with AJAX and JavaScript but I couldn't figure out how to get the PHP to get the information from the db.
-
Post the relevant code that you have, and some data about the relevant database tables.
Table Relationships. It's an advanced concept, but you'll benefit learning it early.
I had separate pages for it.
edit_groups.php
<?php include_once "scripts/checkuserlog.php"; $id = $logOptions_id; if (isset($_POST['parse_var']) && $_POST['parse_var'] == "add_to_group"){ $toGroup = $_POST['toGroup']; $addFriend = $_POST['addFriend']; $toGroup = preg_replace('#[^0-9]#i', '', $toGroup); $addFriend = preg_replace('#[^0-9]#i', '', $addFriend); $id = preg_replace('#[^0-9]#i', '', $id); if (($toGroup != "")&&($addFriend !== "")&&($id != "")){ $sql = mysql_query("INSERT INTO user_groups (group_oid, group_mid, group_id) VALUES('$id','$addFriend','$toGroup')"); header ("Location: edit_groups.php"); } } if (isset($_POST['parse_var']) && $_POST['parse_var'] == "remove_from_group"){ $removeFriend = $_POST['removeFriend']; $removeFriend = preg_replace('#[^0-9]#i', '', $removeFriend); $id = preg_replace('#[^0-9]#i', '', $id); if (($removeFriend !== "")&&($id !== "")){ $sql = mysql_query("DELETE FROM user_groups WHERE group_oid-'$id' AND group_mid='$removeFriend' LIMIT 1"); header ("Location: edit_groups.php"); } } $sql = mysql_query("SELECT friend_array FROM myMembers WHERE id='$id'"); while($row = mysql_fetch_array($sql)){ $friendArray = $row['friend_array']; } $in_group = array(); $sql2 = mysql_query("SELECT group_mid FROM user_groups WHERE group_oid='$id'"); while($row2 = mysql_fetch_array($sql2)){ $in_group[] = $row2['group_mid']; } $in_group2 = implode(", ", $in_group); $addList = ""; if ($friendArray != ""){ $addList .= '<select name="addFriend">'; $addList .= '<option value=""></option>'; if ($in_group2 != ""){ $sql = mysql_query("SELECT id, username FROM myMembers WHERE id IN($friendArray) AND id NOT IN($in_group2)"); } else { $sql = mysql_query("SELECT id, username FROM myMembers WHERE id IN($friendArray)"); } while($row = mysql_fetch_array($sql)){ $addList .= '<option value="'.$row["id"].'">'.$row["username"].'</option>'; } $addList .= '</select>'; } $remList = ""; if (($friendArray != "") && ($in_group2 !== "")) { $remList .= '<select name="removeFriend">'; $remList .= '<option value=""></option>'; $remsql = mysql_query("SELECT id, username FROM myMembers WHERE id IN($friendArray) AND id IN($in_group2)"); while($remrow = mysql_fetch_array($remsql)){ $remList .= '<option value="'.$remrow["id"].'">'.$remrow["username"].'</option>'; } $remList .= '</select>'; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Manage Your Groups</title> <link href="style/main.css" rel="stylesheet" type="text/css" /> <style type="text/css"> <!-- #groupsWrap { width: 900px; margin-right:auto; margin-left:auto; background-color: #f2f2f2; } #groupsTitle { height: 50px; width: 880px; padding-top: 20px; padding-left: 20px; } #groupsContent { float:left; width: 700px; margin-left: 20px; } #groupsAd { float:right; height: 600px; width: 160px; } .groupsClear { clear:both; } .glists { float:left; width: 200px; margin-right: 30px; } --> </style> </head> <body> <?php include_once "header_template.php"; ?> <div id="groupsWrap"> <div id="groupsTitle"><h1>Group Manager</h1></div><!-- end groupsTitle --> <div id="groupsContent"> <p> </p> <p>This top section is for adding someone from your friends to an assigned group <br /> Each person may only be in 1 group. </p> <?php if($friendArray != ""){ ?> <form method="post" action="edit_groups.php"> Add <?php echo $addList; ?> To <select name="toGroup"> <option value=""></option> <option value="Friends">Friends</option> <option value="Musicians">Musicians</option> <option value="Dancers">Dancers</option> <option value="Painters">Painters</option> <option value="Singers">Singers</option> </select> <input name="parse_var" type="hidden" value="add_to_group" /> <input name="submit" type="submit" id="add" value="Add To Group" /> </form> <?php } ?> <br /><br /> <p>This bottom section is for removing someone from a group.<br /> This does not delete that person as a friend, it only removes them from that group. </p> <?php if(($friendArray != "")&&($in_group2 !== "")){ ?> <form method="post" action="edit_groups.php"> Remove from group <?php echo $remList; ?> <input name="parse_var" type="hidden" value="remove_from_group" /> <input type="submit" name="add" id="add" value="Remove From Group" /> </form> <?php } ?> <br /> <div class="glists"><b>Friends</b><br /> <?php $sql_friends = mysql_query(" SELECT username FROM myMembers WHERE id IN (SELECT group_mid FROM user_groups WHERE group_oid ='$id' AND group_id= 1) "); while($fr_row = mysql_fetch_array($sql_friends)){ echo $fr_row['username']; echo '<br />'; }?> </div><!-- end friends glist --> <div class="glists"> <b>Musicians</b><br /> <?php $sql_musicians = mysql_query(" SELECT username FROM myMembers WHERE id IN (SELECT group_mid FROM user_groups WHERE group_oid ='$id' AND group_id= 2) "); while($ms_row = mysql_fetch_array($sql_musicians)){ echo $ms_row['username']; echo '<br />'; }?> </div><!-- end musician glist --> <div class="glists"> <b>Dancers</b><br /> <?php $sql_dancers = mysql_query(" SELECT username FROM myMembers WHERE id IN (SELECT group_mid FROM user_groups WHERE group_oid ='$id' AND group_id= 3) "); while($dan_row = mysql_fetch_array($sql_dancers)){ echo $dan_row['username']; echo '<br />'; }?> </div><!-- end dancer glist --> <div class="glists"> <b>Painters</b><br /> <?php $sql_painters = mysql_query(" SELECT username FROM myMembers WHERE id IN (SELECT group_mid FROM user_groups WHERE group_oid ='$id' AND group_id= 4) "); while($pn_row = mysql_fetch_array($sql_painters)){ echo $pn_row['username']; echo '<br />'; }?> </div><!-- end painter glist --> <div class="glists"> <b>Singers</b><br /> <?php $sql_singers = mysql_query(" SELECT username FROM myMembers WHERE id IN (SELECT group_mid FROM user_groups WHERE group_oid ='$id' AND group_id= 5) "); while($sng_row = mysql_fetch_array($sql_singers)){ echo $sng_row['username']; echo '<br />'; }?> </div><!-- end singers glist --> </div><!-- end groupsContent --> <div class="groupsClear"></div><!-- end groupsClear --> </div><!-- end groupsWrap --> </body> </html>
That example you gave is like what I have in my database.
-
Does anyone know how to possibly do a tagging type of thing with @?
I'm having a status update thing but I want the users to also be able to tag other users. Anything?
-
You'll want to use a many to many relationship with a database.
That aside, we cannot tell you anything else without more information.
What do you mean a many to many relationship?
What information would you need?
-
Hey there everyone, I'm having a problem with my code. What I had wanted to do was have my users to create groups and then choose which people in the groups to see their post. I had some radio buttons with the options of the groups and that was supposed to have only the group they chose see their post.
I removed the buttons for now because I couldn't get it to work. Please help, thank you.
Making a group with certain talents using PHP?
in PHP Coding Help
Posted
Thanks! That really helps, I'll test it out.