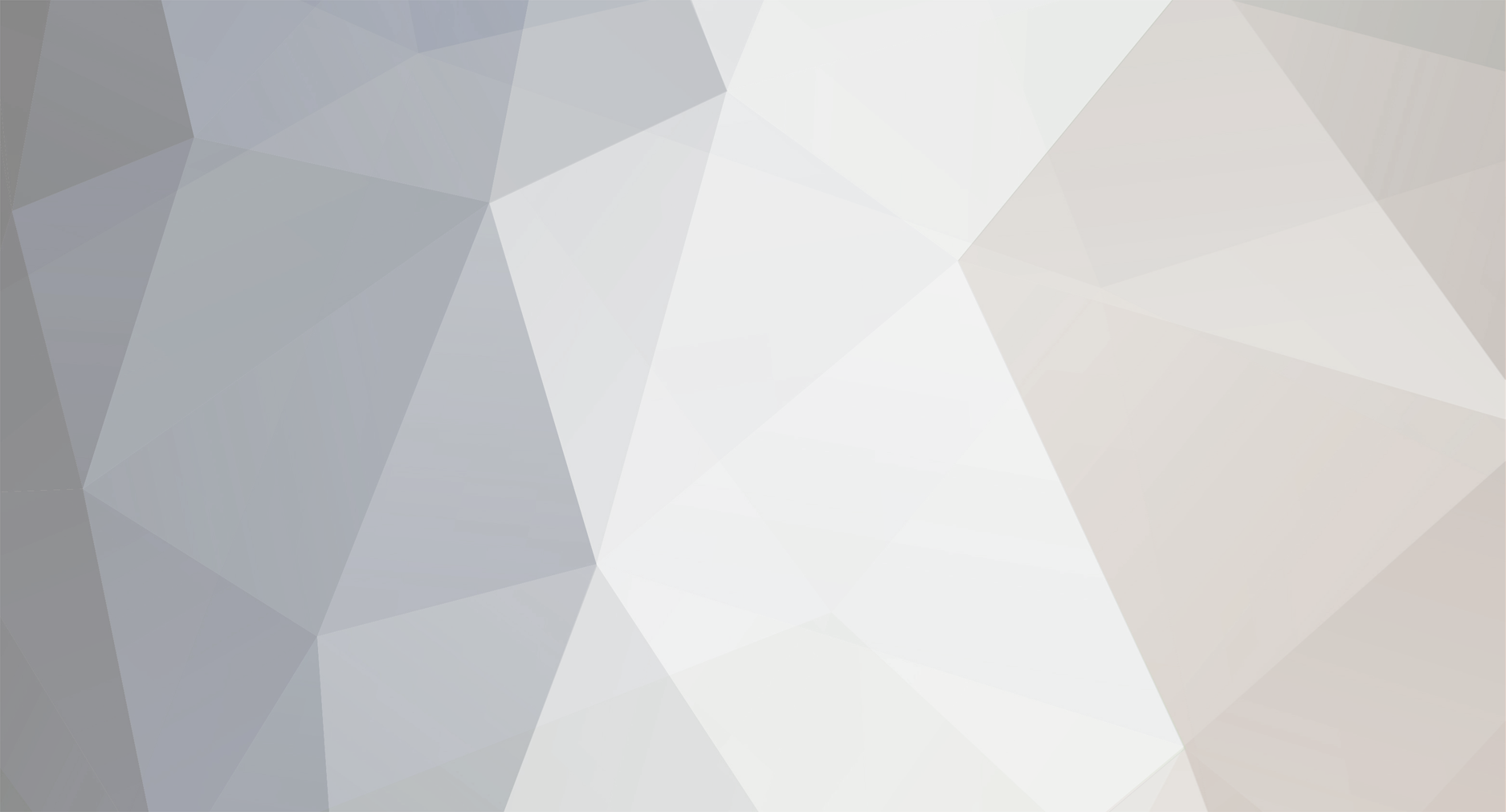
Diether
Members-
Posts
41 -
Joined
-
Last visited
Everything posted by Diether
-
hi ChristianF, can you help me build the code for that so that i can study the logic behind?
-
@ PFMaBismAd , your codes works perfectly. thank you so much. i take down notes also so that i can study the logic. Can you also help me to simplify this line. <?php ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// // Section 3 (if user chooses to adjust item quantity) ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// if (isset($_POST['item_to_adjust']) && $_POST['item_to_adjust'] != "") { // execute some code $item_to_adjust = $_POST['item_to_adjust']; $quantity = $_POST['quantity']; $quantity = preg_replace('#[^0-9]#i', '', $quantity); // filter everything but numbers if ($quantity >= 100) { $quantity = 99; } if ($quantity < 1) { $quantity = 1; } if ($quantity == "") { $quantity = 1; } $i = 0; foreach ($_SESSION["cart_array"] as $each_item) { $i++; while (list($key, $value) = each($each_item)) { if ($key == "item_id" && $value == $item_to_adjust) { // That item is in cart already so let's adjust its quantity using array_splice() array_splice($_SESSION["cart_array"], $i-1, 1, array(array("item_id" => $item_to_adjust, "quantity" => $quantity))); } // close if condition } // close while loop } // close foreach loop } what i did is this.. but it does not adjust my items in the cart. if (isset($_POST['item_to_adjust']) && $_POST['item_to_adjust'] != ""){ $item_to_adjust =(int) $_POST['item_to_adjust']; if($item_to_adjust > 0){ if(!isset($_SESSION['cart_array'][$pid])){ //item is in tht cart so add it with 1 $_SESSION['cart_array'][$item_to_adjust] = array("item_id" => $pid, "quantity" =>1); }else{ //item is in the cart, increment quantity $_SESSION['cart_array'][$item_to_adjust]['quantity']++; } } header("location : cart.php"); exit(); }
-
thanks PFMaBismad ,, i will try this.. i think this is more easier to understand..i will leave comment here if it works. thanks also to Christian F for your comment. have a nice day to everyone
-
// RUN IF THE CART HAS AT LEAST ONE ITEM IN IT foreach ($_SESSION["cart_array"] as $each_item) { $i++; while (list($key, $value) = each($each_item)) { if ($key == "item_id" && $value == $pid) { // That item is in cart already so let's adjust its quantity using array_splice() array_splice($_SESSION["cart_array"], $i-1, 1, array(array("item_id" => $pid, "quantity" => $each_item['quantity'] + 1))); $wasFound = true; } // close if condition } // close while loop } // close foreach loop i mean this one? can it be simplified? thanks
-
thanks trq , it really helps. can i simplify this line of codes?
-
thank you muddy_funster, in addition ,Do you know how to grab the values of that array for testing to make it more readable? just like this.
-
hi guys..This codes really works fine to me but as a student i want to know how it works. PLease see my problem below. <?php ob_start(); session_start(); // Start session first thing in script // Script Error Reporting error_reporting(E_ALL); ini_set('display_errors', '1'); ?> <?php // Connect to the MySQL database require_once("includes/connection.php"); ?> <?php include("includes/header.php"); ?> <?php // // Section 1 (if user attempts to add something to the cart from the product page) // if (isset($_POST['pid'])) { $pid = $_POST['pid']; $wasFound = false; $i = 0; // If the cart session variable is not set or cart array is empty if (!isset($_SESSION["cart_array"]) || count($_SESSION["cart_array"]) < 1) { // RUN IF THE CART IS EMPTY OR NOT SET $_SESSION["cart_array"] = array(0 => array("item_id" => $pid, "quantity" => 1)); } else { // RUN IF THE CART HAS AT LEAST ONE ITEM IN IT foreach ($_SESSION["cart_array"] as $each_item) { $i++; while (list($key, $value) = each($each_item)) { if ($key == "item_id" && $value == $pid) { // That item is in cart already so let's adjust its quantity using array_splice() array_splice($_SESSION["cart_array"], $i-1, 1, array(array("item_id" => $pid, "quantity" => $each_item['quantity'] + 1))); $wasFound = true; } // close if condition } // close while loop } // close foreach loop if ($wasFound == false) { array_push($_SESSION["cart_array"], array("item_id" => $pid, "quantity" => 1)); } } header("location: cart.php"); exit(); } ?> <?php // // Section 2 (if user chooses to empty their shopping cart) // if (isset($_GET['cmd']) && $_GET['cmd'] == "emptycart") { unset($_SESSION["cart_array"]); } ?> <?php // // Section 3 (if user chooses to adjust item quantity) // if (isset($_POST['item_to_adjust']) && $_POST['item_to_adjust'] != "") { // execute some code $item_to_adjust = $_POST['item_to_adjust']; $quantity = $_POST['quantity']; $quantity = preg_replace('#[^0-9]#i', '', $quantity); // filter everything but numbers if ($quantity >= 100) { $quantity = 99; } if ($quantity < 1) { $quantity = 1; } if ($quantity == "") { $quantity = 1; } $i = 0; foreach ($_SESSION["cart_array"] as $each_item) { $i++; while (list($key, $value) = each($each_item)) { if ($key == "item_id" && $value == $item_to_adjust) { // That item is in cart already so let's adjust its quantity using array_splice() array_splice($_SESSION["cart_array"], $i-1, 1, array(array("item_id" => $item_to_adjust, "quantity" => $quantity))); } // close if condition } // close while loop } // close foreach loop } ?> <?php // // Section 4 (if user wants to remove an item from cart) // if (isset($_POST['index_to_remove']) && $_POST['index_to_remove'] != "") { // Access the array and run code to remove that array index $key_to_remove = $_POST['index_to_remove']; if (count($_SESSION["cart_array"]) <= 1) { unset($_SESSION["cart_array"]); } else { unset($_SESSION["cart_array"]["$key_to_remove"]); sort($_SESSION["cart_array"]); } } ?> <?php // // Section 5 (render the cart for the user to view on the page) // $cartOutput = ""; $cartTotal = ""; $pp_checkout_btn = ''; $product_id_array = ''; if (!isset($_SESSION["cart_array"]) || count($_SESSION["cart_array"]) < 1) { $cartOutput = "<h2 align='center'>Your shopping cart is empty</h2>"; } else { // Start PayPal Checkout Button $pp_checkout_btn .= '<form action="https://www.paypal.com/cgi-bin/webscr" method="post"> <input type="hidden" name="cmd" value="_cart"> <input type="hidden" name="upload" value="1"> <input type="hidden" name="business" value="you@youremail.com">'; // Start the For Each loop $i = 0; foreach ($_SESSION["cart_array"] as $each_item) { $item_id = $each_item['item_id']; $sql = mysql_query("SELECT * FROM product WHERE id='$item_id' LIMIT 1"); while ($row = mysql_fetch_array($sql)) { $product_name = $row["name"]; $price = $row["price"]; $details = $row["details"]; } $pricetotal = $price * $each_item['quantity']; $cartTotal = $pricetotal + $cartTotal; setlocale(LC_MONETARY, "en_US"); $pricetotal = number_format($pricetotal,2); // Dynamic Checkout Btn Assembly $x = $i + 1; $pp_checkout_btn .= '<input type="hidden" name="item_name_' . $x . '" value="' . $product_name . '"> <input type="hidden" name="amount_' . $x . '" value="' . $price . '"> <input type="hidden" name="quantity_' . $x . '" value="' . $each_item['quantity'] . '"> '; // Create the product array variable $product_id_array .= "$item_id-".$each_item['quantity'].","; // Dynamic table row assembly $cartOutput .= "<tr>"; $cartOutput .= '<td><a href="product.php?id=' . $item_id . '">' . $product_name . '</a><br /><img src="inventory_images/' . $item_id . '.jpg" alt="' . $product_name. '" width="40" height="52" border="1" /></td>'; $cartOutput .= '<td>' . $details . '</td>'; $cartOutput .= '<td>$' . $price . '</td>'; $cartOutput .= '<td><form action="cart.php" method="post"> <input name="quantity" type="text" value="' . $each_item['quantity'] . '" size="1" maxlength="2" /> <input name="adjustBtn' . $item_id . '" type="submit" value="change" /> <input name="item_to_adjust" type="hidden" value="' . $item_id . '" /> </form></td>'; //$cartOutput .= '<td>' . $each_item['quantity'] . '</td>'; $cartOutput .= '<td>' . $pricetotal . '</td>'; $cartOutput .= '<td><form action="cart.php" method="post"><input name="deleteBtn' . $item_id . '" type="submit" value="X" /><input name="index_to_remove" type="hidden" value="' . $i . '" /></form></td>'; $cartOutput .= '</tr>'; $i++; } setlocale(LC_MONETARY, "en_US"); $cartTotal = number_format($cartTotal,2); $cartTotal = "<div style='font-size:18px; margin-top:12px;' align='right'>Cart Total : ".$cartTotal." Php</div>"; // Finish the Paypal Checkout Btn $pp_checkout_btn .= '<input type="hidden" name="custom" value="' . $product_id_array . '"> <input type="hidden" name="notify_url" value="https://www.yoursite.com/storescripts/my_ipn.php"> <input type="hidden" name="return" value="https://www.yoursite.com/checkout_complete.php"> <input type="hidden" name="rm" value="2"> <input type="hidden" name="cbt" value="Return to The Store"> <input type="hidden" name="cancel_return" value="https://www.yoursite.com/paypal_cancel.php"> <input type="hidden" name="lc" value="US"> <input type="hidden" name="currency_code" value="USD"> <input type="image" src="http://www.paypal.com/en_US/i/btn/x-click-but01.gif" name="submit" alt="Make payments with PayPal - its fast, free and secure!"> </form>'; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Your Cart</title> <link rel="stylesheet" href="style/style.css" type="text/css" media="screen" /> </head> <body> <a href = "index.php">Continue shopping </a> <div align="center" id="mainWrapper"> <div id="pageContent"> <div style="margin:24px; text-align:left;"> <br /> <table width="100%" border="1" cellspacing="0" cellpadding="6"> <tr> <td width="18%" bgcolor="#C5DFFA"><strong>Product</strong></td> <td width="45%" bgcolor="#C5DFFA"><strong>Product Description</strong></td> <td width="10%" bgcolor="#C5DFFA"><strong>Unit Price</strong></td> <td width="9%" bgcolor="#C5DFFA"><strong>Quantity</strong></td> <td width="9%" bgcolor="#C5DFFA"><strong>Total</strong></td> <td width="9%" bgcolor="#C5DFFA"><strong>Remove</strong></td> </tr> <?php echo $cartOutput; ?> <!-- <tr> <td> </td> <td> </td> <td> </td> <td> </td> <td> </td> <td> </td> </tr> --> </table> <?php echo $cartTotal; ?> <br /> <br /> <?php //echo $pp_checkout_btn; ?> <br /> <br /> <a href="cart.php?cmd=emptycart">Click Here to Empty Your Shopping Cart</a> </div> <br /> </div> </div> </body> <?php include("includes/footer.php"); ob_flush(); ?> </html> This is the part that i can't understand clearly. Can someone help me to explain about this line of codes? and how can i grab the value of this array to be more readable so that i can clearly understand what is happening with this process. This is would be a big help for my study. Thanks in advance. // RUN IF THE CART HAS AT LEAST ONE ITEM IN IT foreach ($_SESSION["cart_array"] as $each_item) { $i++; while (list($key, $value) = each($each_item)) { if ($key == "item_id" && $value == $pid) { // That item is in cart already so let's adjust its quantity using array_splice() array_splice($_SESSION["cart_array"], $i-1, 1, array(array("item_id" => $pid, "quantity" => $each_item['quantity'] + 1))); $wasFound = true; } // close if condition } // close while loop } // close foreach loop
-
at pikachu2000,, can you tell me what will be the best solution to my problem. i cant move on,, almost an hour
-
Good day Guys, Im a beginner in php and currently making simple add, edit and delete. i have this error message when i click the edit button. Please help me to solve this. thanks in advance Warning: mysql_fetch_array() expects parameter 1 to be resource, string given in C:\xampp\htdocs\simpleProgram\edit.php on line 13 i use this code : index.php <?php include_once ("dbcon.php")?> <html> <head><h1>simple add and edit</h1></head> <title>My simple program</title> <body> <form method = "post" action = "add.php"> <table > <tr><td>Title:</td> <td><input type = "text" name = "title" ></td></tr> <tr><td>Author:</td><td><input type = "text" name = "author" ><td/></tr> <tr><td>Publisher Name:</td> <td><input type = "text" name = "publisherName" ></td></tr> <tr><td>Copyright Year:</td> <td><input type = "text" name = "copyrightYear" ></td></tr> <tr><td></td><td><input type = "submit" name = "submit" value = "add"></td></tr> </table> </form> </br> </br> <table border = "1"> <tr><td>id</td> <td>title</td> <td>author</td> <td>Publisher Name</td> <td>Copyright Year</td></tr> <?php $query = mysql_query("SELECT * FROM books") or die (mysql_error()); while($myBookRows = mysql_fetch_array($query)){ ?> <tr> <td><?php echo $myBookRows['BookID']; ?></td> <td><?php echo $myBookRows['Title'] ; ?> </td> <td><?php echo $myBookRows['Author']; ?> </td> <td><?php echo $myBookRows['PublisherName'] ;?></td> <td><?php echo $myBookRows['CopyrightYear'] ;?></td> <td><a href="delete.php<?php echo '?id='.$myBookRows['BookID']; ?>">delete</a></td> <td><a href="edit.php<?php echo '?id='.$myBookRows['BookID']; ?>">Edit</a></td> </tr> <?php } ?> </table> </body> </html> edit.php <?php include_once("dbcon.php") ; $id = $_GET['id']; ?> <head><h1>simple add and edit</h1></head> <title>My simple program</title> <body> <form method = "post" > <table> <?php $query = ("SELECT * FROM books WHERE BOOKID = '$id' ") or die (mysql_error()); $myBookRows= mysql_fetch_array($query); ?> <html> <tr><td>Title:</td> <td><input type = "text" name = "title" value = "<?php echo $myBookRows['Title'] ?>"></td></tr> <tr><td>Author:</td> <td><input type = "text" name = "author" value = "<?php echo $myBookRows['Author']?>"></td></tr> <tr><td>Publisher Name:</td> <td><input type = "text" name = "publisherName" value "<?php echo $myBookRows['PublisherName'] ?> "> </td> </tr> <tr><td>Copyright Year:</td> <td><input type = "text" name ="copyrightYear" value "<?php echo $myBookRows['CopyrightYear'] ?> "> </td></tr> <tr><td></td> <td> <input type = "submit" name = "submit" value = "save" > </td></tr> </table> </form> </html> <?php if (isset($_POST['submit'])){ $title = $_POST['title']; $author = $_POST['author']; $publisherName = $_POST['publisherName']; $copyrightYear = $_POST['copyrightYear']; mysql_query("UPDATE books SET Title = '$title', Author = '$author', PublisherName = '$publisher',copyrightYear = '$copyrightYear' WHERE BOOKID = '$id'"); header('location : index.php'); } ?>
-
Hi guys Good day, my problem is this. everytime i click the "add this item now" button. the data is not insert in my database. is there anyway to isolate my problem in codes? there is also no error appears. thats why i cant figured out whats the problem. please help me guys. thanks in advance here is my form screenshot: my codes: <?php // This block grabs the whole list for viewing $product_list = ""; $sql = mysql_query("SELECT * FROM product ORDER BY date_added DESC"); $productCount = mysql_num_rows($sql); // count the output amount if ($productCount > 0) { while($row = mysql_fetch_array($sql)){ $id = $row["id"]; $product_name = $row["name"]; $price = $row["price"]; $date_added = strftime("%b %d, %Y", strtotime($row["date_added"])); $product_list .= "Product ID: $id - <strong>$product_name</strong> - $$price - <em>Added $date_added</em> <a href='inventory_edit.php?pid=$id'>edit</a> • <a href='inventory_list.php?deleteid=$id'>delete</a><br />"; } } else { $product_list = "You have no products listed in your store yet"; } // Parse the form data and add inventory item to the system if (isset($_POST['name'])) { $product_name = mysql_real_escape_string($_POST['name']); $price = mysql_real_escape_string($_POST['price']); //$category = mysql_real_escape_string($_POST['category']); //$subcategory = mysql_real_escape_string($_POST['subcategory']); $details = mysql_real_escape_string($_POST['details']); // See if that product name is an identical match to another product in the system $sql = mysql_query("SELECT id FROM product WHERE name='$product_name' LIMIT 1"); $productMatch = mysql_num_rows($sql); // count the output amount if ($productMatch > 0) { echo 'Sorry you tried to place a duplicate "Product Name" into the system, <a href="inventory_list.php">click here</a>'; exit(); } // Add this product into the database now $sql = mysql_query("INSERT INTO product (name, price, details,date_added) VALUES('$product_name','$price',now())") or die (mysql_error()); $pid = mysql_insert_id(); // Place image in the folder $newname = $pid. '.jpg'; move_uploaded_file($_FILES['fileField']['tmp_name'], "../inventory_images/".$newname); header("location: inventory_list.php"); exit(); } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <head><title>Inventory</title></head> <style type="text/css"> <!-- .style1 {font-size: 10px} --> </style></head> <link rel="stylesheet" href="../stylesheet/style.css" type="text/css" media="screen" /> <body> <div align = "center"id="wrapper"> <div id="content"><br/> <div align = "right" style="margin-right:140px;"> <a href="inventory_list.php#inventory_form">+ Add new inventory item</a> </div> <div align= "left" style = "margin-left:140px;"> <h2>Inventory List</h2> <?php echo $product_list; ?> </div> <div align="left"> <blockquote> <blockquote> <blockquote> <blockquote> <p id="inventory_form" name="inventory_form"><a name = "inventory_form" id="inventory_form"></a> </p> </blockquote> </blockquote> </blockquote> </blockquote> </div> <h3>Add New Inventory Item Form</h3> <form id="my_form" name="my_form" method="post" action="inventory_list.php" enctype="multipart/form-data"> <table width="633" height="248" border="1"> <tr> <td width="156"><label>Product Name:</label> </td> <td width="425"><input type="text" name="product_name" id="product_name" /></td> </tr> <tr> <td><label>Product Price:</label></td> <td>$ <input type="text" name="price" id="price" /></td> </tr> <tr> <td height="45">Product Details:</td> <td> <textarea name="details" id="details"></textarea></td> </tr> <tr> <td>Product Image:</td> <td> <input type="file" name="fileField" id="fileField" /> </td> </tr> <tr> <td> </td> <td> <input type="submit" name="submit" id="submit" value="Add this item now" /></td> </tr> </table> </form> <p> </p> <p> </p> <p> </p> <p><br/> </p> </div> </div> </body> </html> <?php include("../includes/footer.php"); ?>
-
ah i see.. i know now the error came from: localhost/online/admin_login.php which is not existed but my url location is to be like this: localhost/online/storeadmin/admin_login.php my question is where to put that in my code? how to be in that directory when i submit?
-
Good day ,, can someone help me with this?.. i encounter this upon submission of username and password. it says that my object was not found. although i have it in my directory. thanks in advance. error: Object not found! The requested URL was not found on this server. The link on the referring page seems to be wrong or outdated. Please inform the author of that page about the error. If you think this is a server error, please contact the webmaster. Error 404 localhost Apache/2.4.3 (Win32) OpenSSL/1.0.1c PHP/5.4.7 the code use: //home.php <?php include("storeAdmin/admin_login.php"); doLogin() ?> //admin_login.php <?php function doLogin() { // if we found an error save the error message in this variable $errorMessage = ''; $userName = mysql_real_escape_string(!empty( $_POST['txtUsername'])) ? $_POST['txtUsername'] : '' ; $passWord = mysql_real_escape_string(!empty( $_POST['txtPassword'])) ? $_POST['txtUsername'] : '' ; // first, make sure the username & password are not empty if ($userName == '') { $errorMessage = 'You must enter your username'; } else if ($password == '') { $errorMessage = 'You must enter the password'; } else { // check the database and see if the username and password combo do match $sql = "SELECT id FROM user WHERE username = '$userName' AND password = '$password'"; $result = dbQuery($sql); if (dbNumRows($result) == 1) { $row = dbFetchAssoc($result); $_SESSION['plaincart_user_id'] = $row['id']; // log the time when the user last login $sql = "UPDATE user SET user_last_login = NOW() WHERE id = '{$row['id']}'"; dbQuery($sql); // now that the user is verified we move on to the next page // if the user had been in the admin pages before we move to // the last page visited if (isset($_SESSION['login_return_url'])) { header('Location: ' . $_SESSION['login_return_url']); exit; } else { header('Location:index.php'); exit; } } else { $errorMessage = 'Wrong username or password'; } } return $errorMessage; } ?> <html> <form id="form1" name="form1" method="post" action="admin_login.php"> <p align="center">username:<input type="text" name="txtUsername" id="username" /> </p> <p align="center">password:<input type="password" name="txtPassword" id="password" /> </p> <p align="center"> </p> </blockquote> <p align="center"> <input type="submit" name="log_in" id="log_in" value="Log in" /> </p> </form> </html> My folder structure:
-
the form wasn't submitted yet when i encounter the error, how can i debug this? any tips?
-
Hi guys, can someone help me to solve my problem in creating a login system? thanks in advance i get this error Notice: Undefined index: txtUsername in C:\xampp\htdocs\online\storeAdmin\admin_login.php on line 6 Notice: Undefined index: txtPassword in C:\xampp\htdocs\online\storeAdmin\admin_login.php on line 7 This are the two lines : $userName = $_POST['txtUsername']; $password = $_POST['txtPassword']; The code i use: function doLogin() { // if we found an error save the error message in this variable $errorMessage = ''; $userName = $_POST['txtUsername']; $password = $_POST['txtPassword']; // first, make sure the username & password are not empty if ($userName == '') { $errorMessage = 'You must enter your username'; } else if ($password == '') { $errorMessage = 'You must enter the password'; } else { // check the database and see if the username and password combo do match $sql = "SELECT user_id FROM tbl_user WHERE user_name = '$userName' AND user_password = PASSWORD('$password')"; $result = dbQuery($sql); if (dbNumRows($result) == 1) { $row = dbFetchAssoc($result); $_SESSION['plaincart_user_id'] = $row['user_id']; // log the time when the user last login $sql = "UPDATE tbl_user SET user_last_login = NOW() WHERE user_id = '{$row['user_id']}'"; dbQuery($sql); // now that the user is verified we move on to the next page // if the user had been in the admin pages before we move to // the last page visited if (isset($_SESSION['login_return_url'])) { header('Location: ' . $_SESSION['login_return_url']); exit; } else { header('Location: index.php'); exit; } } else { $errorMessage = 'Wrong username or password'; } } return $errorMessage; } <html> <form id="form1" name="form1" method="post" action="admin_login.php"> <p align="center">username:<input type="text" name="txtUsername" id="username" /> </p> <p align="center">password:<input type="password" name="txtPassword" id="password" /> </p> <p align="center"> </p> </blockquote> <p align="center"> <input type="submit" name="log_in" id="log_in" value="Log in" /> </p> </form>
-
thanks Christian F. i will try your suggestion. i think thats a very good idea for a beginner like me..
-
Good day, just new in php and really confuse. i have a dynamic Grid below in my screenshot,that is only straight. the codes are working well but i want to put it in 3 columns. so i research about dynamic grid output, My problem is after trying to insert this in my codes,,, it gives me different error.. sa mga mabubuti po ang loob dyan ,, pls guide me to make my images in 3 columns. thanks in advance current image: my desired output: Here the codes in my index.php <?php // Run a select query to get my letest 6 items // Connect to the MySQL database include "includes/connect_to_mysql.php"; $dynamicList = ""; $sql = mysql_query("SELECT * FROM tbl_products ORDER BY date_added DESC LIMIT 6"); $productCount = mysql_num_rows($sql); // count the output amount if ($productCount > 0) { while($row = mysql_fetch_array($sql)){ $id = $row["id"]; $product_name = $row["product_name"]; $price = $row["price"]; $date_added = strftime("%b %d, %Y", strtotime($row["date_added"])); $dynamicList .= '<table width="418" border="2" cellpadding="6"> <tr> <td width="124" valign="top"><a href="product.php?id=' . $id . '"><img src="inventory_images/' . $id . '.jpg" alt="' . $product_name . '" width="120" height="121" /></a></td> <td width="256" valign="top">' . $product_name . '<br /> $' . $price . '<br /> <a href="product.php?id=' . $id . '">View Product Details</a></td> </tr> </table>'; } } else { $dynamicList = "We have no products listed in our store yet"; } mysql_close(); ?> code that i want to insert: <?php // Include database connection include_once 'connect_to_mysql.php'; // SQL query to interact with info from our database $sql = mysql_query("SELECT id, member_name FROM member_table ORDER BY id DESC LIMIT 15"); $i = 0; // Establish the output variable $dyn_table = '<table border="1" cellpadding="10">'; while($row = mysql_fetch_array($sql)){ $id = $row["id"]; $member_name = $row["member_name"]; if ($i % 3 == 0) { // if $i is divisible by our target number (in this case "3") $dyn_table .= '<tr><td>' . $member_name . '</td>'; } else { $dyn_table .= '<td>' . $member_name . '</td>'; } $i++; } $dyn_table .= '</tr></table>'; ?> <html> <body> <h3>Dynamic PHP Grid Layout From a MySQL Result Set</h3> <?php echo $dyn_table; ?> </body> </html>