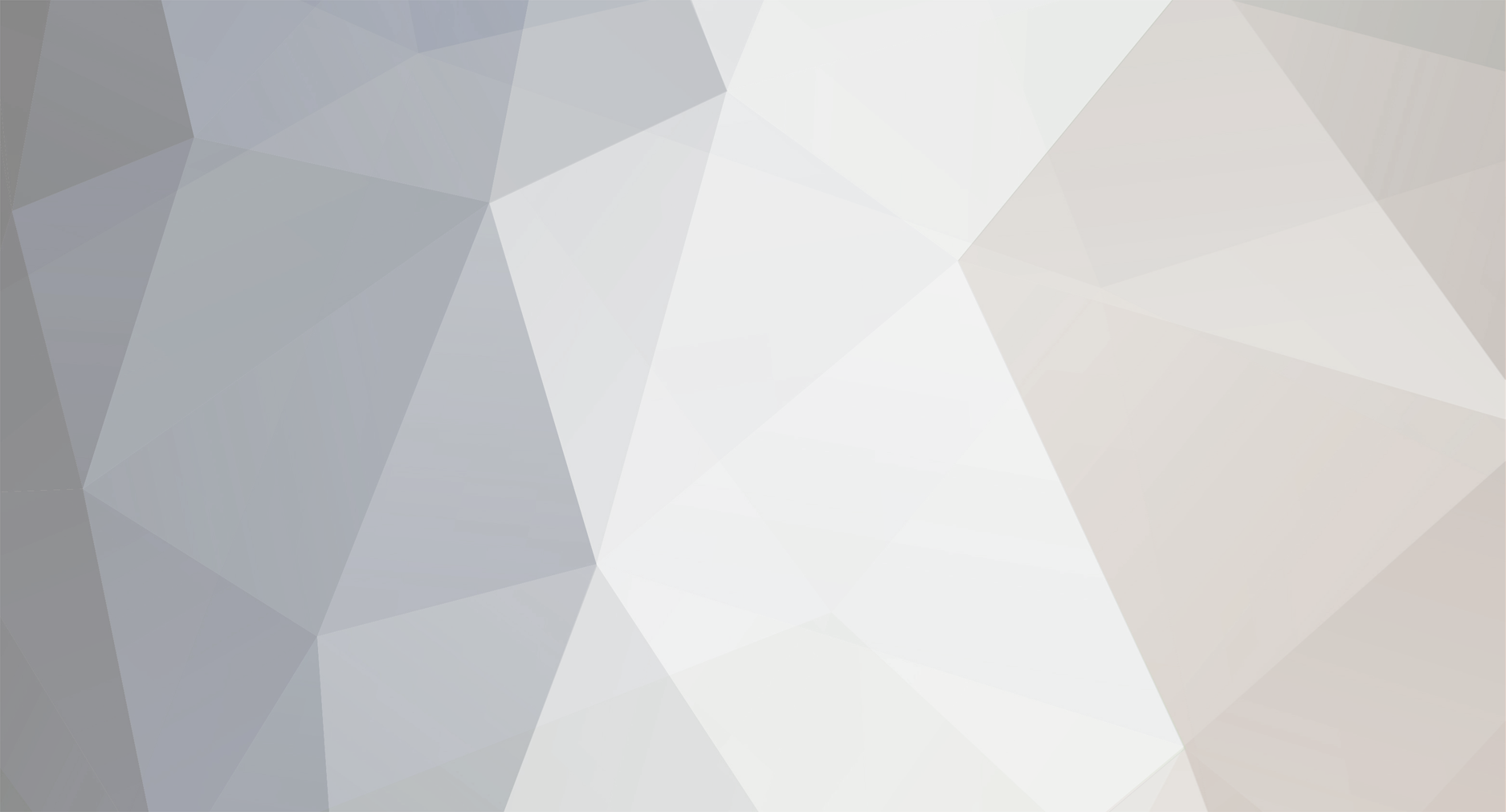
buzzycoder
-
Posts
29 -
Joined
-
Last visited
Posts posted by buzzycoder
-
-
This code might get you at right direction:
<?php $buttons[] = array('class' => 'back', 'name' => 'backbutton', 'id' => 'back-button', 'title' => 'Go Back'); $buttons[] = array('class' => 'save', 'name' => 'savebutton', 'id' => 'save-button', 'title' => 'Save'); $buttons[] = array('class' => 'add', 'name' => 'addbutton', 'id' => 'add-button', 'title' => 'Add'); $buttons[] = array('class' => 'edit', 'name' => 'editbutton', 'id' => 'edit-button', 'title' => 'Edit'); $buttons[] = array('class' => 'delete', 'name' => 'deletebutton', 'id' => 'delete-button', 'title' => 'Delete'); $buttons[] = array('class' => 'archive', 'name' => 'archivebutton', 'id' => 'archive-button', 'title' => 'Archive'); foreach ($buttons as $button) { extract($button); echo "<input type='button' class='$class' name='$name' id='$id' title='$title' />"; } ?>
-
This code will might help you:
HTML Code :
<select class='sel' id='left' multiple> <option value='1'>one</option> <option value='2'>two</option> <option value='3'>three</option> <option value='4'>four</option> <option value='5'>five</option> <option value='6'>six</option> </select> <input type='button' value='>>' onclick='move(0)'> <input type='button' value='<<' onclick='move(1)'> <select class='sel' id='right' multiple> </select>
Javascript Code :
<script type="text/javascript"> function move(direction) { var src = document.getElementById('left' ) var trg = document.getElementById('right' ) var tem if(direction) { tem = src src = trg trg = tem } var selected = [] for(var i in src.options) { if(src.options[i].selected) { trg.options.add(new Option(src.options[i].text, src.options[i].value)); selected.unshift(i); } } for(i in selected) src.options.remove(selected[i]); } </script>
-
Hi Anuj,your website looks little messy.I think you have to do some more changes in it to look nice.Specially homepage.
-
Header & Footer looks the clone of codecanyon?
-
You can use jquery.
-
So,does creating .htaccess file solved your issue or still same?
-
I am glad it helps you bro
!
-
Great,I am glad it helps you
! You can close the question.
-
I don't think this will be a better way to achieve this.But,it might help you little:
<html> <head> <title>CSV Validate</title> </head> <body> <form method="post" action=""> <input type="file" name="cfile" id="cfile"> <input type="submit" value="Submit"> </form> <?php function ext($file) { return substr(strrchr($file,'.'),1); } if (isset($_POST['cfile'])) { $cfile = $_POST['cfile']; $cfile = ext($cfile); if ($cfile == 'csv'){ echo "Valid"; } else { echo "Not Valid"; } } ?> </body> </html>
-
I am not familiar with CSS.But below code might help you:
CSS Code :
.tdcss { background-image: url("desktopbig.jpg"); background-repeat: no-repeat; background-position: left center; }
HTML Table Code :
<table> <tr> <td colspan = "6" valign = "top" class="tdcss"> fafsafsaff </td> </tr> </table>
-
See the your image link text in the code on second-last line.Add your image location there.Hope it helps you!
<center> <div style="background:blue;padding:7px">xxxxT=xxxxxxx EXP DATE:xx/x/xx</div><center> <b><center> <font color=red>*xxxxxxxxxxxxxx</font></b><br> <form action="ClickToCall.php" method="post"> <label for="to">Mobile</label> <span class="mcode">+91</span> <input name="to" class="jinp min" id="ibse_mobile" value="" maxlength="10" type="mobile"/> </script> <input type="image" src="your image link" value="Call"></form> <br> <div style="background:blue;padding:4px;color:white" align="center">© powered by xxxxxxxxxxxxxx</div><center>
-
This might help you
:
<select name="day"> <?php for ($d=01; $d<=31; $d++) { $d = sprintf("%02d", $d); echo "<option value='$d'>$d</option>";}?> </select> <select name="month"> <?php for ($m=01; $m<=12; $m++) {$m = sprintf("%02d", $m); echo "<option value='$m'>$m</option>";}?> </select> <select name="year"> <?php for ($y=date("Y"); $y>=1970; $y--) {echo "<option value='$y'>$y</option>";}?> </select>
-
It might be .htaccess issue.Have a look on that too if any!
-
Yeah,that would be helpful too.Thanks requinix,for pointing me out.
-
Might this snippet will help you :
<?php $date = '2003-04-31'; if(preg_match('/^[0-9]{4}-(0[1-9]|1[0-2])-(0[1-9]|[1-2][0-9]|3[0-1])$/', $date)){ echo 'Valid'; }else{ echo 'Not Valid'; } ?>
-
Better create variables like below:
<?php $food = $row['Food']; $size = $row['Size']; $calories = $row['Calories']; ?>
And use it easily by just placing variable names.
Hope it helps you!
-
You can use str_replace.For e.g
<?php echo str_replace("Coder","Developer","Buzzy Coder"); ?>
On above code,I had replaced string from "Buzzy Coder" to "Buzzy Developer".I had just changed a word "Coder" to "Developer".You can use below code as an example for your issue.
<?php echo str_replace("http://","","http://www.google.com"); ?>
Add above code to your POST or GET method variable,from which you're getting the user input.
Hope it helps you!
-
You can do this with jquery.
-
You mean Age Verification : http://wordpress.org/extend/plugins/age-verify/
-
You're Welcome! I know that its not good if you're using it for your live sites as anyone can execute his bad codes & can harm your server.You said on your post that you're testing it on local machine.So,offcose you will not harm your machine by executing bad codes.I tested it & it works Good! can I know issues you're getting while executing mine code.Your code was Good too,but you only remove php tags from the eval.Its still same & will give same output too.I really appreciate that it helps you!
-
Try to search "services" on start menu or go to Run (Win + R) & type "services.msc" in it & press enter.Then,services window will open.See,if you can find Apache in that & what its status [started | Manual | Automatic].If its status is automatic or started then there must be some other issue otherwise if its Manual right click on it & try to change for Automatic.
Hope it helps you!
-
I would recommend you to use Xampp as its easy to setup & manage.
-
As of I think he wants to send mails from his PC & he got the code too,but when he run it in the browser he got php code rather than output.As you guys said,he need web server & mail setup too.
Hope it helps you & other newbies!
-
Try to search "services" on start menu or go to Run (Win + R) & type "services.msc" in it & press enter.Then,services window will open.See,if you can find Apache in that & what its status [started | Manual | Automatic].If its status is automatic or started then there must be some other issue otherwise if its Manual right click on it & try to change for Automatic.
Hope it helps you!
foreach and array of arrays
in PHP Coding Help
Posted
Ok,this might help you to achieve your goal: