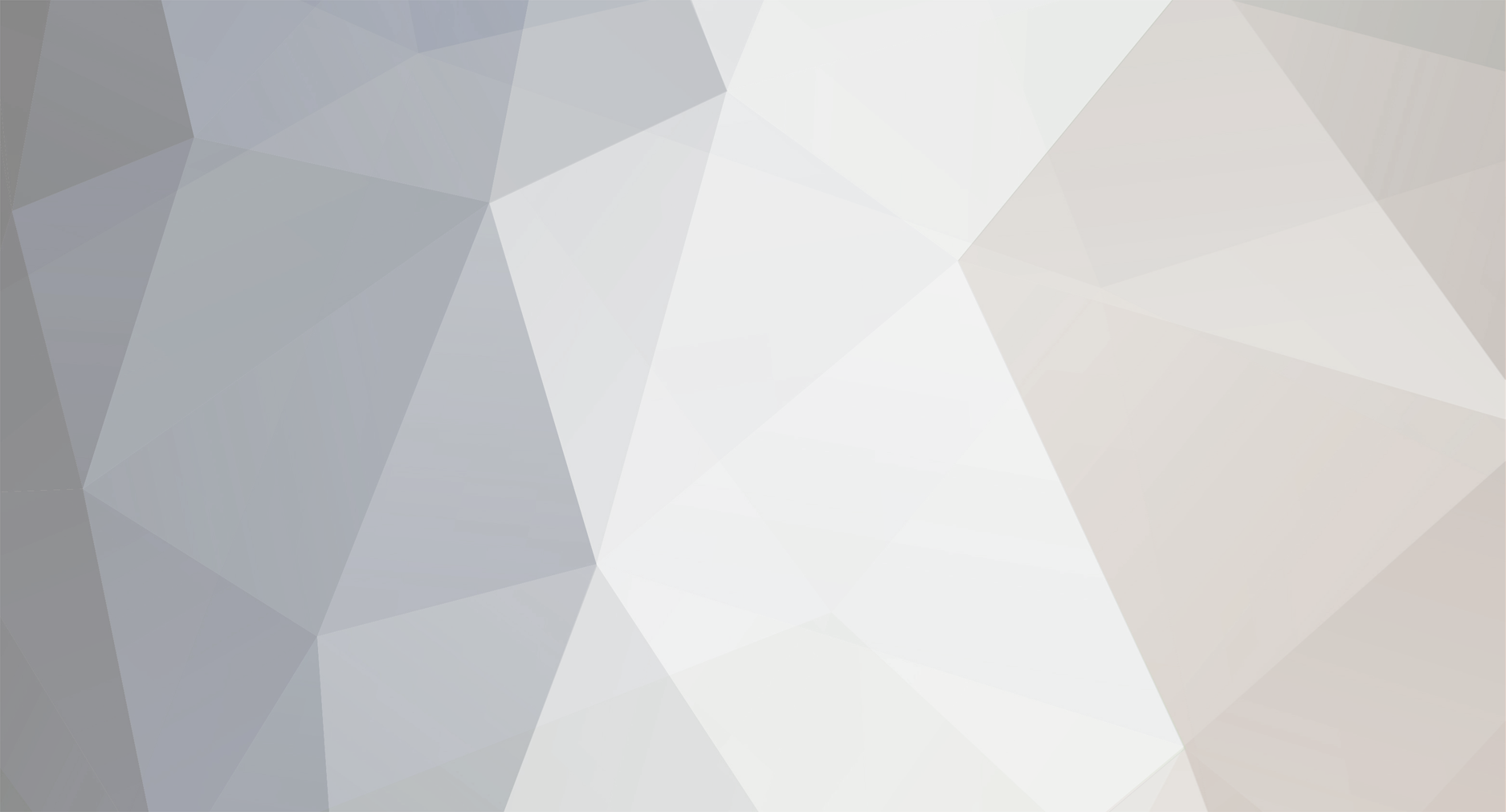
pixeltrace
Members-
Posts
577 -
Joined
-
Last visited
Never
Everything posted by pixeltrace
-
need help on my display page with pagination
pixeltrace replied to pixeltrace's topic in PHP Coding Help
this is the code inside my_pagina_class.php require("db_config.php"); error_reporting(E_ALL); // only for testing class JHpage { var $sql; var $result; var $outstanding_rows = false; var $get_var; var $rows_on_page; var $max_rows; // constructor function JHpage($rows = 0) { $this->connect_db(); $this->max_rows = $rows; $this->get_var = QS_VAR; $this->rows_on_page = NUM_ROWS; } // sets the current page number function set_page() { $page = (isset($_REQUEST[$this->get_var]) && $_REQUEST[$this->get_var] != "") ? $_REQUEST[$this->get_var] : 0; return $page; } // gets the total number of records function get_total_rows() { $tmp_result = mysql_query($this->sql); $all_rows = mysql_num_rows($tmp_result); if (!empty($this->max_rows) && $all_rows > $this->max_rows) { $all_rows = $this->max_rows; $this->outstanding_rows = true; } mysql_free_result($tmp_result); return $all_rows; } // database connection function connect_db() { $conn_str = mysql_connect(DB_SERVER, DB_USER, DB_PASSWORD); mysql_select_db(DB_NAME, $conn_str); } // get the totale number of result pages function get_num_pages() { $number_pages = ceil($this->get_total_rows() / $this->rows_on_page); return $number_pages; } // returns the records for the current page function get_page_result() { $start = $this->set_page() * $this->rows_on_page; $page_sql = sprintf("%s LIMIT %s, %s", $this->sql, $start, $this->rows_on_page); $this->result = mysql_query($page_sql); return $this->result; } // get the number of rows on the current page function get_page_num_rows() { $num_rows = mysql_num_rows($this->result); return $num_rows; } // free the database result function free_page_result() { mysql_free_result($this->result); } // function to handle other querystring than the page variable function rebuild_qs($curr_var) { if (!empty($_SERVER['QUERY_STRING'])) { $parts = explode("&", $_SERVER['QUERY_STRING']); $newParts = array(); foreach ($parts as $val) { if (stristr($val, $curr_var) == false) { array_push($newParts, $val); } } if (count($newParts) != 0) { $qs = "&".implode("&", $newParts); } else { return false; } return $qs; // this is your new created query string } else { return false; } } // this method will return the navigation links for the conplete recordset function navigation($separator = " | ", $css_current = "", $back_forward = false, $use_images = false) { $max_links = NUM_LINKS; $curr_pages = $this->set_page(); $all_pages = $this->get_num_pages() - 1; $var = $this->get_var; $navi_string = ""; if (!$back_forward) { $max_links = ($max_links < 2) ? 2 : $max_links; } if ($curr_pages <= $all_pages && $curr_pages >= 0) { if ($curr_pages > ceil($max_links/2)) { $start = ($curr_pages - ceil($max_links/2) > 0) ? $curr_pages - ceil($max_links/2) : 1; $end = $curr_pages + ceil($max_links/2); if ($end >= $all_pages) { $end = $all_pages + 1; $start = ($all_pages - ($max_links - 1) > 0) ? $all_pages - ($max_links - 1) : 1; } } else { $start = 0; $end = ($all_pages >= $max_links) ? $max_links : $all_pages + 1; } if($all_pages >= 1) { $forward = $curr_pages + 1; $backward = $curr_pages - 1; // the text two labels are new sinds ver 1.02 $lbl_forward = $this->build_back_or_forward("forward", $use_images); $lbl_backward = $this->build_back_or_forward("backward", $use_images); $navi_string = ($curr_pages > 0) ? "<a class='link1' href=\"".$_SERVER['PHP_SELF']."?".$var."=".$backward.$this->rebuild_qs($var)."\">".$lbl_backward."</a> " : $lbl_backward." "; if (!$back_forward) { for($a = $start + 1; $a <= $end; $a++){ $theNext = $a - 1; // because a array start with 0 if ($theNext != $curr_pages) { $navi_string .= "<a class='link1' href=\"".$_SERVER['PHP_SELF']."?".$var."=".$theNext.$this->rebuild_qs($var)."\">"; $navi_string .= $a."</a>"; $navi_string .= ($theNext < ($end - 1)) ? $separator : ""; } else { $navi_string .= ($css_current != "") ? "<span class='text6'\"".$css_current."\">".$a."</span>" : $a; $navi_string .= ($theNext < ($end - 1)) ? $separator : ""; } } } $navi_string .= ($curr_pages < $all_pages) ? " <a class='link1' href=\"".$_SERVER['PHP_SELF']."?".$var."=".$forward.$this->rebuild_qs($var)."\">".$lbl_forward."</a>" : " ".$lbl_forward; } } return $navi_string; } // function to create the back/forward elements; $what = forward or backward // type = text or img function build_back_or_forward($what, $img = false) { $label['text']['forward'] = STR_FWD; $label['text']['backward'] = STR_BWD; $label['img']['forward'] = IMG_FWD; $label['img']['backward'] = IMG_BWD; if ($img) { // $img_info = getimagesize($label['img'][$what]); //$label = "<img src=\"".$label['img'][$what]."\" ".$img_info[3]." border=\"0\">"; } else { $label = $label['text'][$what]; } return $label; } // this info will tell the visitor which number of records are shown on the current page function page_info($str = "Result: %d - %d of %d") { $first_rec_no = ($this->set_page() * $this->rows_on_page) + 1; $last_rec_no = $first_rec_no + $this->rows_on_page - 1; $last_rec_no = ($last_rec_no > $this->get_total_rows()) ? $this->get_total_rows() : $last_rec_no; $info = sprintf($str, $first_rec_no, $last_rec_no, $this->get_total_rows()); return $info; } // simple method to show only the page back and forward link. function back_forward_link($images = true) { $simple_links = $this->navigation(" ", "", false, $images); return $simple_links; } } ?> -
guys, i need help, i usually uploads csv to our database and currently this is my code LOAD DATA INFILE 'test_users.csv' INTO TABLE test FIELDS TERMINATED BY ',' OPTIONALLY ENCLOSED BY '"' ESCAPED BY '\\' LINES TERMINATED BY '\n' (id, name, username, email, md5(password), usertype, block, sendEmail, gid, registerDate, lastvisitDate, activation, params); this one is working if i replaced md5(password) with just password my problem now, i need the password to be in md5 format. what is the correct code for this? thanks!
-
need help on my display page with pagination
pixeltrace replied to pixeltrace's topic in PHP Coding Help
this is the error Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in c:\hosting\webhost4life\member\diorgrace\admean\pagina\my_pagina_class.php on line 90 Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in c:\hosting\webhost4life\member\diorgrace\admean\pagina\my_pagina_class.php on line 63 Warning: mysql_free_result(): supplied argument is not a valid MySQL result resource in c:\hosting\webhost4life\member\diorgrace\admean\pagina\my_pagina_class.php on line 68 Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in c:\hosting\webhost4life\member\diorgrace\admean\pagina\my_pagina_class.php on line 63 Warning: mysql_free_result(): supplied argument is not a valid MySQL result resource in c:\hosting\webhost4life\member\diorgrace\admean\pagina\my_pagina_class.php on line 68 Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in c:\hosting\webhost4life\member\diorgrace\admean\pagina\my_pagina_class.php on line 63 Warning: mysql_free_result(): supplied argument is not a valid MySQL result resource in c:\hosting\webhost4life\member\diorgrace\admean\pagina\my_pagina_class.php on line 68 Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in c:\hosting\webhost4life\member\diorgrace\admean\pagina\my_pagina_class.php on line 63 Warning: mysql_free_result(): supplied argument is not a valid MySQL result resource in c:\hosting\webhost4life\member\diorgrace\admean\pagina\my_pagina_class.php on line 68 Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in c:\hosting\webhost4life\member\diorgrace\admean\pagina\my_pagina_class.php on line 63 Warning: mysql_free_result(): supplied argument is not a valid MySQL result resource in c:\hosting\webhost4life\member\diorgrace\admean\pagina\my_pagina_class.php on line 68 Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in c:\hosting\webhost4life\member\diorgrace\admean\pagina\my_pagina_class.php on line 63 Warning: mysql_free_result(): supplied argument is not a valid MySQL result resource in c:\hosting\webhost4life\member\diorgrace\admean\pagina\my_pagina_class.php on line 68 actually i noticed, since i print the $test so i will see whats happening on my query. i noticed that on my first page the query result is this jhpage Object ( [sql] => SELECT * FROM applicant WHERE country = 'Singapore' [result] => [outstanding_rows] => [get_var] => page [rows_on_page] => 20 [max_rows] => 0 ) but when i click on page 2 the query result is this jhpage Object ( [sql] => SELECT * FROM applicant WHERE [result] => [outstanding_rows] => [get_var] => page [rows_on_page] => 20 [max_rows] => 0 ) the value of the country ='Singapore' is gone i think it has something to do with the POST and GET function but i dont know how to fix it. hope you could help me with this. thanks! -
guys, i need help, i have a display page with pagination and gets an error whenever i go to the other page (let say page2) i have 2 page first page is the search page, wherein i search an item and then goes to the second page which is the display page. on my display page, i have a search tool there also, and that one works fine and whenever i used it, i dont get the error on the pagination. below is the code for my first page <table width="562" border="0" cellspacing="2" cellpadding="3"> <tr> <td colspan="3" valign="top"><img src="images/spacer.gif" width="10" height="1" /></td> </tr> <tr> <td colspan="3" bgcolor="#999999" class="text2">Keyword Search </td> </tr> <tr> <td colspan="3" valign="top"><img src="images/spacer.gif" width="10" height="10" /></td> </tr> <form action="searchcnddate.php?id=2" method="post"><tr> <td width="83" align="right" valign="top" class="text6">keywords : </td> <td width="1" rowspan="4"> </td> <td width="452" align="left" class="text6"><input name="keywords" type="text" size="35" /></td> </tr> <tr> <td align="right" valign="top" class="text6">country : </td> <td align="left" class="text6"><select name="country"> <option value="Afghanistan">Afghanistan</option> <option value="Albania">Albania</option> <option value="Algeria">Algeria</option> <option value="American Samoa">American Samoa</option> <option value="Andorra">Andorra</option> </select></td> </tr> <tr> <td align="right" valign="top" class="text6">gender : </td> <td align="left" class="text7"><input name="gender" type="radio" value="Male" /> male <input name="gender" type="radio" value="Female" /> female</td> </tr> <tr> <td align="right" valign="top" class="text6">last employed position : </td> <td align="left" valign="top" class="text6"><input name="lastposition" type="text" size="35" /></td> </tr> <tr> <td align="right" valign="top" class="text6"> </td> <td> </td> <td><span class="text6"> <input type="submit" name="Submit" value="Search by Keywords" class="textfield1" /> <span class="text7"> <input type="hidden" name="id4" value="2" /> </span></span></td> </tr></form> <tr> <td colspan="3" bgcolor="#999999" class="text2">Search by Email </td> </tr> <tr> <td colspan="3" align="right" valign="top" class="text6"><img src="images/spacer.gif" width="10" height="10" /></td> </tr> <tr> <td align="right"><span class="text6">email : </span></td> <td align="right"> </td> <form action="searchcnddate.php?id=3" method="post"> <td align="left" class="text7"> <input name="email" type="text" size="35" /> <input type="submit" name="Submit2" value="Search Email" class="textfield1"></td> </form></tr> <tr> <td colspan="3" align="right" valign="top" class="text6"> </td> </tr> <tr> <td colspan="3" bgcolor="#999999" class="text2">Search by Candidate Name </td> </tr> <tr> <td colspan="3" align="right" valign="top" class="text6"><img src="images/spacer.gif" width="10" height="10" /></td> </tr> <tr> <td align="right" valign="top" class="text6">name : </td> <td align="right" valign="top" class="text6"> </td> <form action="searchcnddate.php?id=4" method="post"> <td align="left" valign="top" class="text6"> <input name="name" type="text" size="35" /> <input type="submit" name="Submit22" value="Search Name" class="textfield1"></td> </form></tr> <tr> <td colspan="3" align="right" class="text6"> </td> </tr> <tr> <td colspan="3" bgcolor="#999999" class="text2">Keyword by Job Specialization </td> </tr> <tr> <td colspan="3" align="right" valign="top" class="text6"><img src="images/spacer.gif" width="10" height="10" /></td> </tr> <tr> <td align="right" valign="top" class="text6">specialization : </td> <td align="right" valign="top" class="text6"> </td> <form action="searchcnddate.php?id=5" method="post"> <td align="left" valign="top" class="text6"><select name="specialization"> <option value="" selected>-- select specialization here --</option> <? $uSql = "SELECT specialization FROM specialization"; $uResult = mysql_query($uSql, $connection); if(!$uResult){ echo 'no data found'; }else{ while($uRow = mysql_fetch_array($uResult)){ ?> <option value="<?= $uRow[specialization]?>"> <?= $uRow[specialization]?> </option> <? } } ?> </select> <input type="submit" name="Submit222" value="Search Specialization" class="textfield1" /></td> </form></tr> <tr> <td colspan="3" align="right" valign="top" class="text6"> </td> </tr> </table> and this is the code for my display page <?php session_start(); if (session_is_registered("username")){ $id = $_POST['id']; include("pagina/my_pagina_class.php"); include 'db_connect.php'; //establish query array $query_array = array(); //append each input field into a new index //in the query_array if the user selected //it or entered information in the field if(!empty($_POST['keywords'])){ $query_array[] = "resume LIKE '%". $_POST['keywords'] ."%'"; } if(!empty($_POST['country'])){ $query_array[] = "country = '". $_POST['country'] ."'"; } if(!empty($_POST['gender'])){ $query_array[] = "gender = '". $_POST['gender'] ."'"; } if(!empty($_POST['lastposition'])){ $query_array[] = "currentposition LIKE '%". $_POST['lastposition'] ."%'"; } //-- if(!empty($_POST['keywords2'])){ $query_array[] = "lname LIKE '%". $_POST['keywords2'] ."%' OR fname LIKE '%". $_POST['keywords2'] ."%' OR resume LIKE '%". $_POST['keywords2'] ."%' OR email LIKE '%". $_POST['keywords2'] ."%'"; } //turn array into string $query_string = implode(" AND ", $query_array); //-- $test = new JHpage; $test->sql = "SELECT * FROM applicant WHERE ". $query_string .""; //$test->sql = "SELECT * FROM applicant WHERE specialization LIKE '%". $sort3 ."%' ORDER BY ".$sort1 ." ".$sort2.""; // the (basic) sql statement (use the SQL whatever you like) $result = $test->get_page_result(); // result set $num_rows = $test->get_page_num_rows(); // number of records in result set $nav_links = $test->navigation(" | ", "currentStyle"); // the navigation links (define a CSS class selector for the current link) $nav_info = $test->page_info(); // information about the number of records on page ("to" is the text between the number) $simple_nav_links = $test->back_forward_link(true); // the navigation with only the back and forward links, use true to use images $total_recs = $test->get_total_rows(); // the total number of records ?> <script type="text/JavaScript"> <!-- function MM_openBrWindow(theURL,winName,features) { //v2.0 window.open(theURL,winName,features); } //--> </script> <table width="100" border="0" cellspacing="0" cellpadding="0"> <tr> <td><img src="../images/spacer.gif" width="6" height="10"></td> <td><table width="216" border="0" cellspacing="0" cellpadding="0"> <tr> <td colspan="3" valign="top" bgcolor="#3261BB"><img src="../resume/images/spacer.gif" width="1" height="1"></td> </tr> <tr> <td align="left" bgcolor="#3261BB" width="1"><img src="../resume/images/spacer.gif" width="1" height="1"></td> <td width="214" valign="top"> <table width="897" border="0" cellspacing="2" cellpadding="0"> <tr> <td colspan="6" align="right"><form method="post" action="searchcnddate.php?id=2"> <table width="100%" border="0" cellspacing="0" cellpadding="0"> <tr> <td width="407"><span class="text6"> <?php echo " total record found: " .$total_recs; ?> </span></td> <td width="486" align="right" class="text6">new search : <input type="text" name="keywords2" value="enter keywords"> <input type="submit" name="Submit" value="search" /> </td> </tr> </table> </form></td> </tr> <tr> <td colspan="6"><table width="100%" border="0" cellspacing="0" cellpadding="0"> <tr> <td width="60%" align="left" class="text8"> </td> <td width="39%" align="right"><!-- nav top --> <? echo "<span class='link1'>".$nav_links."</span>"; ?> </td> <td width="1%" align="right"><img src="../images/spacer.gif" width="1" height="1" /></td> </tr> </table></td> </tr> <tr> <td width="68" align="center" bgcolor="#83C2ED" class="text5">ID</td> <td width="320" align="center" valign="top" bgcolor="#83C2ED" class="text5">name</td> <td width="220" align="center" bgcolor="#83C2ED" class="text5">email</td> <td width="163" align="center" bgcolor="#83C2ED" class="text5">last employed position </td> <td width="125" align="center" bgcolor="#83C2ED" class="text5">date registered </td> </tr> <?php for ($i = 0; $i < $num_rows; $i++) { $appid = mysql_result($result, $i, "appid"); $fname = mysql_result($result, $i, "fname"); $lname = mysql_result($result, $i, "lname"); $email = mysql_result($result, $i, "email"); $currentposition = mysql_result($result, $i, "currentposition"); $dateregistered = mysql_result($result, $i, "dateregistered"); ?> <tr> <td align="center" bgcolor="#FFFFCC" class="text8"><span class="text5"> </span> A<? echo ($appid <= 9) ? $appid : $appid;?> </td> <td bgcolor="#FFFFCC"> <a href="javascript:;" class="link1" onClick="MM_openBrWindow('resume/preview.php?appid=<? echo "$appid"; ?>','','scrollbars=yes,width=760')"> <? echo " ".$fname." ".$lname." "; ?></a></td> <td bgcolor="#FFFFCC" class="text8"> <? echo "$email";?> </td> <td bgcolor="#FFFFCC" class="text8"> <? echo "$currentposition";?><br></td> <td bgcolor="#FFFFCC" class="text8"><? echo "$dateregistered";?></td> <? echo "</td></tr>"; ?> <? } ?> <tr> <td colspan="6" bgcolor="#83C2ED"><img src="../images/spacer.gif" width="10" height="2" /></td> </tr> <tr> <td colspan="6"><table width="100%" border="0" cellspacing="0" cellpadding="0"> <tr> <td width="60%" align="left" class="text8"> </td> <td width="39%" align="right"><!-- nav top --> <? echo "<span class='link1'>".$nav_links."</span>"; ?> </td> <td width="1%" align="right"><img src="../images/spacer.gif" width="1" height="1" /></td> </tr> </table></td> </tr> <tr> <td colspan="6"> </td> </tr> </table> </td> <td align="right" bgcolor="#3261BB" width="1"><img src="../resume/images/spacer.gif" width="1" height="1"></td> </tr> <tr> <td colspan="3" valign="top" bgcolor="#3261BB"><img src="../resume/images/spacer.gif" width="1" height="1"></td> </tr> </table></td> <td><img src="../images/spacer.gif" width="6" height="10"></td> </tr> </table> <? }else{ echo "<font face=\"Arial\">You are not authorized to access this page ... Please <a href='../index.php'>Login</a></font>"; } ?> hope you could help me with this. thanks!
-
hi, i dont know if this is already a form of hacking. but is there any php or any scripts that grabs a specfic information in a specific website and send it to you via email? or is there any scripts that can inform you via email whenever there are new items being posted on a specific website? aside from rss feeds? hope you could help me with this. thanks so much!
-
hi, i need help, i have a table that got lots of duplicates. i need a query that will display all items with duplicates on the username order by username where in the display will be something like: ========= | id | username | email 1 username1 | email1@email.com 2 username1 | email2@email.com 3 username2 | email3@email.com 4. username2 | email4@email.com hope you could help me with this. thanks!
-
Thanks! I'll try this out.
-
hi, i need help, i want to upload a csv file let say on table 1 and later on compare table 1 with table 2 for some duplicates. what is the sql query that will check fields in table 1 with table2 for any duplicate items? let say select * from table1 where email=email of table2 what is the correct code for this? also, what query to use to check fields in table1 with table2 for items that are not yet existing in table 2? let say select * from table1 where email not equal to email of table2 what is the correct code for this? hope you could help me with this. thanks so much!
-
hi, i accidentally loaded a csv file and forgot to set the password fields to md5 what is the sql query that will change my password to md5? like UPDATE users SET password =md(password) WHERE password='123456'"; hope you could help me with this. thanks!
-
hi, is there any sql code that can import csv file into your mysql db and at the same time checked or send a report of the duplicate items? currently this is the query that i use for uploading csv file LOAD DATA INFILE 'jos_muse_users1.csv' INTO TABLE jos_muse_users FIELDS TERMINATED BY ',' OPTIONALLY ENCLOSED BY '"' ESCAPED BY '\\' LINES TERMINATED BY '\n' (MemberID, ContactID, GivenName, FamilyName, Email, AddressedAsEmail, HomePhone, BusinessPhone, Mobile, Gender, DateofBirth, Address1, Address2, PostalCode, SourceFrom, NRIC, Race, Nationality, ChildName, ChildDOB, ChildGender, City, State, Country, SourcePortal) hope you could help me with this. thanks!
-
sorry, this is the current code $database->setQuery(" SELECT DISTINCT(benefits.`provider`) $query ORDER BY benefits.`id` DESC LIMIT $pageNav->limitstart, $pageNav->limit "); $providers = $database->loadObjectList(); foreach ($providers as $provider) { $query = "SELECT providers.name AS `providername`, benefits.* " . " FROM `#__classifieds_providers_benefits` benefits " . " LEFT JOIN `#__classifieds_providers` providers " . " ON benefits.provider = providers.id " . " WHERE `provider` = " . $provider->provider . " AND benefits.`published` = 1" . " ORDER BY `dateadded` DESC " . " LIMIT 0,1"; $database->setQuery($query); if (!$benefit = $database->query()) { echo $database->stderr(); return; } $benefit = $database->loadObjectList(); if($benefit) $benefits[] = $benefit[0];
-
Hi, i need help, i have a page that should display all the list of benefits. whats happening with my current code is that it only displays the latest benefit item. how can i fix this? this is the site http://www.mumcentre.com.my/index.php?option=com_classifieds&act=benefits try to click the "click here to see the complete list of benefits...." let say for bebehaus. notice at the bottom part that there are 2 benfits for this one. hope you could help me fix my query in such a way that i will display all the benefits from the listing page below is my current query $database->setQuery(" SELECT (benefits.`provider`) $query ORDER BY benefits.`id` DESC LIMIT $pageNav->limitstart, $pageNav->limit "); $providers = $database->loadObjectList(); foreach ($providers as $provider) { $query = "SELECT providers.name AS `providername`, benefits.* " . " FROM `#__classifieds_providers_benefits` benefits " . " LEFT JOIN `#__classifieds_providers` providers " . " ON benefits.provider = providers.id " . " WHERE `provider` = " . $provider->provider . " AND benefits.`published` = 1" . " ORDER BY `dateadded` DESC " . " LIMIT 0,1"; $database->setQuery($query); if (!$benefit = $database->query()) { echo $database->stderr(); return; } $benefit = $database->loadObjectList(); if($benefit) $benefits[] = $benefit[0]; hope you could help me with this. thanks so much!
-
thanks! its working! another question how about i need to get it from 2 diff tables? is that possible? let say table 1 will get the data from table 2 and table3 thanks!
-
hi, i need help, what is the sql query that can copy items from one table to another? let say table 1 id | name | address | postalcode | telno and i want to copy all records of fields id | name | address to table 2 id | name | address what is the sql code for this? hope you could help me with this Thanks!
-
Hi, Thanks! i have installed the soap 1.2 already and i already saw soap.ini in my ppp.d directory with the codes soap.wsdl_cache_enabled "1" soap.wsdl_cache_dir "/tmp" soap.wsdl_cache_ttl "86400" my problem now, i dont know if its already enabled. i typed > urpmi soap this is the message that i got The package(s) are already installed The following package names were assumed: php-soap however, i still dont know if its already enabled because i am still getting this error Fatal error: Class 'SoapClient' not found in /var/www/html/myoochi/findit.php on line 2 on one of the test page that i did. is there anything that i need to add in my php.ini file? need help on this please thanks!
-
hi, i need help on my flash banner which is using php. my boss wants that everytime i click on that specific banner, it will add 1 to the clicks field in the table. anyone familiar with flash, because i have just started using flash and barely know actionscripting. hope you could help me with this. db.table id | name | clicks Thanks so much!
-
hi, i actually went to the php.ini already and search for the word "soap" but i cannot find it there. assuming the codes for soap is not present, what is/are the codes that i need to add? the php info page is here http://mango.resonance.com.sg/phpinfo.php hope you could help me with this. thanks!
-
Hi, i need help. how do i enable soap in my php? i have installed soap 1.2 in my linux server already but i dont know how to enable it. i was informed that i need to enable soap in my php.ini file but i dont know what the codes are. this is the error i am currently getting from my site http://mango.resonance.com.sg/myoochi/findit.php Fatal error: Class 'SoapClient' not found in /var/www/html/myoochi/findit.php on line 2 hope you could help me fix this. thanks so much!
-
[SOLVED] how to check duplicate entries in mysql
pixeltrace replied to pixeltrace's topic in PHP Coding Help
Hi my problem is solved already and i found a solution which i wanted to share also ------------------ STEP 1 - Create new table to have zero duplicates mySQL Code -------------- CREATE TABLE new_table AS SELECT * FROM old_table GROUP BY email HAVING ( COUNT(email) = 1 ) STEP 2 - Rename old_table to another name and rename new_table to old_table mySQL Code -------------- (for backup purposes) RENAME TABLE old_table TO old_table_old; RENAME TABLE new_table TO old_table; Thanks again! -
[SOLVED] how to check duplicate entries in mysql
pixeltrace replied to pixeltrace's topic in PHP Coding Help
Hi, i am still getting an error Warning: mysql_fetch_assoc(): supplied argument is not a valid MySQL result resource in /var/www/html/mumcentre/qry.php on line 17 this is my current code for the page <?php $dbhost = 'localhost'; $dbusername = 'username'; $dbpasswd = 'password'; $database_name = 'db'; $connection = mysql_pconnect("$dbhost","$dbusername","$dbpasswd") or die ("Couldn't connect to server."); $db = mysql_select_db("$database_name", $connection) or die("Couldn't select database."); $qry = "SELECT jos_muse_users.* FROM jos_muse_users AS t1, jos_muse_users AS t2 WHERE t1.username != t2.username AND t1.email != t2.email"; $qry = mysql_query($qry); while ($row = mysql_fetch_assoc($qry)) { print_r($row); echo "<br />"; } ?> hope you could help me with this. also, let say there are duplicate entries, how can i delete the duplicates? what is the code for this? i found a Mysql Delete Duplicate entries software but its not working. need help on this. thanks! -
Hi, I will be developing one site wherein it will need a soap client to make the mapfinder work. the map will be similar to the mapfinder of www.can.com.sg http://www.can.com.sg/neocan/en/mapfinder.html any suggestions as to what soap client version or type i can use for this kind of application? thanks!
-
sorry please check the site again http://mango.resonance.com.sg/mumcentre/qry.php thanks!
-
hi, i need help on fixing my query i am getting errors on my page http://mango.resonance.com.sg/mumcentre/qry.php this is the current code that i have for this <?php $dbhost = 'localhost'; $dbusername = 'username'; $dbpasswd = 'password'; $database_name = 'db'; $connection = mysql_pconnect("$dbhost","$dbusername","$dbpasswd") or die ("Couldn't connect to server."); $db = mysql_select_db("$database_name", $connection) or die("Couldn't select database."); /*$qry = "SELECT `jos_muse_users`.* FROM `jos_muse_users` AS `t1`, `jos_muse_users` AS `t2`WHERE `t1`.`username` != `t2`.`email`"; $qry = mysql_query($qry); while($row = mysql_fetch_assoc($qry) { print_r($row); echo "<br />"; }*/ ?> <? $uSql = "SELECT * FROM jos_muse_users ORDER by MemberID"; $uResult = mysql_query($uSql, $connection); if(!$uResult){ echo 'no data found'; }else{ while($uRow = mysql_fetch_array($uResult)){ ?> <?= $uRow[MemberID]?>|<?= $uRow[Email]?> <? } } ?> hope you could help me with this. thanks!
-
[SOLVED] how to check duplicate entries in mysql
pixeltrace replied to pixeltrace's topic in PHP Coding Help
hi, i tried this codes, im not sure if this is correct. <?php $dbhost = 'localhost'; $dbusername = 'username'; $dbpasswd = 'password'; $database_name = 'db'; $connection = mysql_pconnect("$dbhost","$dbusername","$dbpasswd") or die ("Couldn't connect to server."); $db = mysql_select_db("$database_name", $connection) or die("Couldn't select database."); $qry = "SELECT `jos_muse_users`.* FROM `jos_muse_users` AS `t1`, `jos_muse_users` AS `t2`WHERE `t1`.`username` != `t2`.`email`"; $qry = mysql_query($qry); while($row = mysql_fetch_assoc($qry) { print_r($row); echo "<br />"; } ?> currently i am getting a parsing unexpected '}' in line 18. hope you could help me solve this. i wanted to know if i have duplicated entries for the username, and email. thanks so much! -
[SOLVED] how to check duplicate entries in mysql
pixeltrace replied to pixeltrace's topic in PHP Coding Help
hi! any help on this please. thanks!