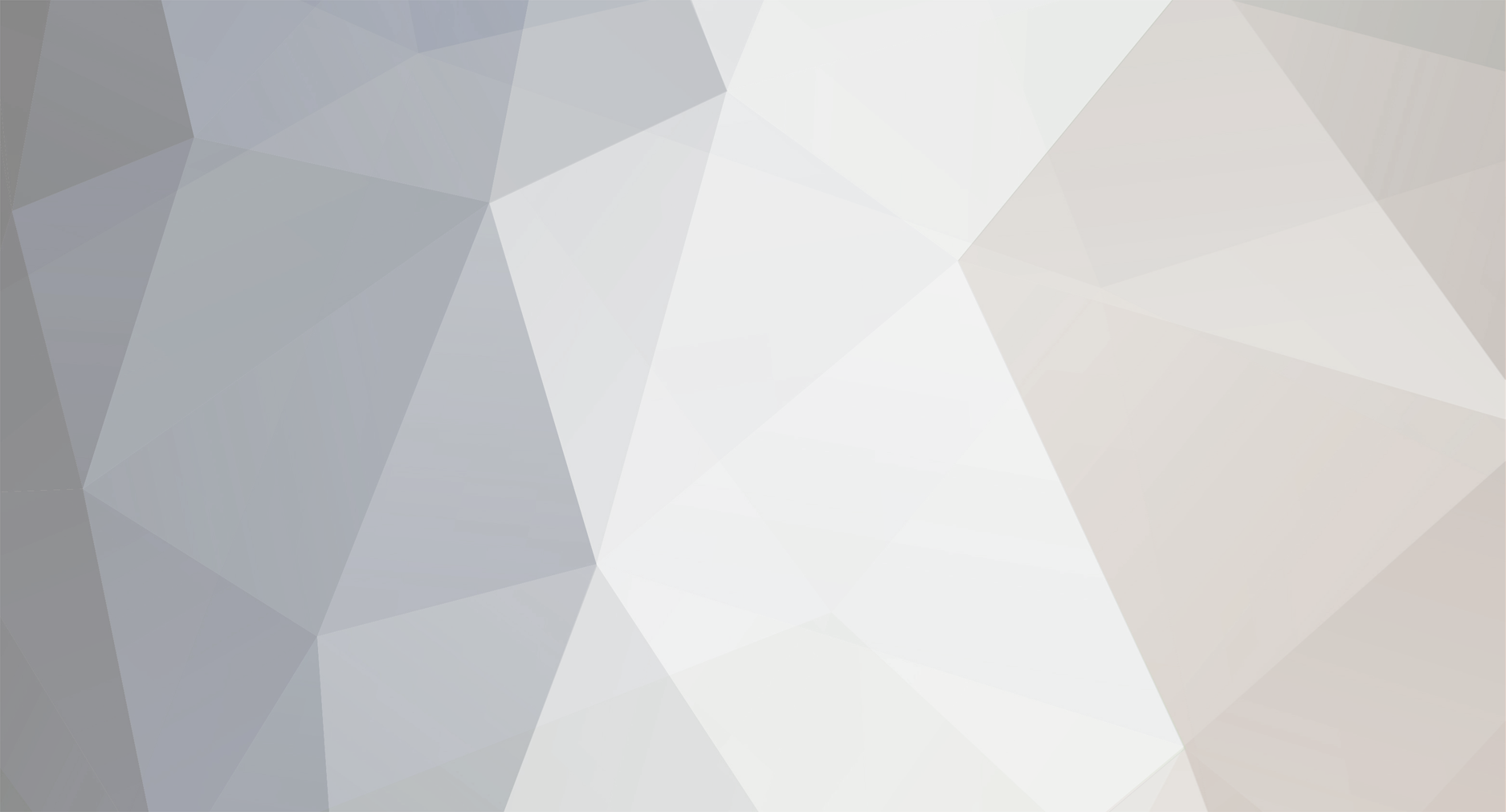
Adrian4263
-
Posts
11 -
Joined
-
Last visited
Posts posted by Adrian4263
-
-
Product table
prodID prodName prodPrice prodCat prodDet homepage prodImage
-------------------------------------------------------------------------
123 Razer 150.00 Mouse
122 Pendrive 30.00 Accessories
Categories table
category
--------
Mouse
Accessories
this two table is part of my database table structures
-
$query = "SELECT COUNT(*) as totalno FROM product GROUP BY prodCat ORDER BY prodName"; $result = mysql_query($query); while($data=mysql_fetch_array($result)) { $count = $data['totalno']; $query6="SELECT * FROM categories ORDER BY category"; $result6=mysql_query($query6); while($row6=mysql_fetch_array($result6)) { ?> <li> <?php echo $row6['category']; ?> (<strong><?php echo $count['prodID']; ?></strong>) </br> </br> </li> <?php $query="SELECT * FROM product where prodCat='".$row6['category']."' LIMIT 6"; $result=mysql_query($query); while($row=mysql_fetch_array($result)) { ?> <div id="product"> <a href="pDetails.php?id=<?php echo $row['id']; ?>"> <img src="data:image/jpeg;base64,<?php echo base64_encode($row["prodImage"]);?>" width="190px" height="190px" /> </a> <p> <b><?php echo $row["prodID"]; ?></b> </p> <p><?php echo $row["prodName"]; ?></p> <p>RM<?php echo $row["prodPrice"]; ?></p> <p> <a href="pDetails.php?id=<?php echo $row['id']; ?>" class="button">Product Details</a> </p> </div> <?php } } } ?>
prodCat is product category.prodName is product Name.
now my output is..
Category - product quantity
---------------------------
Accessories - 4
Mouse - 4
Monitor - 4
CPU - 4
Bags - 4
this was wrong.
I want the output is...
Category - product quantity
---------------------------
Accessories - 11
Mouse - 2
Monitor - 0
CPU - 5
Bags - 0
based on database to show the product quantity.
How could i write the code..
please help.
Thanks in advance
Regards.
-
<?php @session_start(); ?> <html> <head> <title>De Closet | Home</title> <link rel="icon" type="image/png" href="images/set5.png" /> <link rel="stylesheet" type="text/css" href="css/lstyle.css" /> <link rel="stylesheet" type="text/css" href="css/catalog.css" /> <link rel="stylesheet" type="text/css" href="css/button.css" /> </head> <body> <?php include("oHeader.php"); ?> <?php include("config.php"); $queryPromo="SELECT * FROM promo"; $resultPromo=mysql_query($queryPromo); while($rowPromo=mysql_fetch_array($resultPromo)) { echo '<div id="promo">'; echo '<img src="data:image/jpeg;base64,'.base64_encode($rowPromo["image"]).'" width="940px" height="260px" />'; echo '</div>'; } ?> <p> <div id="cslay"> <?php include("oSide.php"); ?> <div id="content"> <?php include("config.php"); $query="SELECT * FROM product ORDER BY id DESC"; $result=mysql_query($query); while($row=mysql_fetch_array($result)) { ?> <div id="product"> <a href="pDetails.php?id=<?php echo $row['id']; ?>"> <img src="data:image/jpeg;base64,<?php echo base64_encode($row["prodImage"]);?>" width="190px" height="190px" /> </a> <p> <b><?php echo $row["prodID"]; ?></b> </p> <p><?php echo $row["prodName"]; ?></p> <p>RM<?php echo $row["prodPrice"]; ?></p> <p> <a href="pDetails.php?id=<?php echo $row['id']; ?>" class="button">Product Details</a> </p> </div> <?php } ?> </div> <div id="blay5"> </div> </div> </p> <?php include("oBottom.php"); ?> </body> </html>
This is my homepage code, i name it as index.phpwhatever i tick(checked) the product,it will show out at this page(homepage)
How should i write the code if checkbox checked then this product will show at homepage(index.php).
Thanks
Regards.
-
<?php @session_start(); ?> <html> <head> <title>De Closet | Home</title> <link rel="icon" type="image/png" href="images/set5.png" /> <link rel="stylesheet" type="text/css" href="css/lstyle.css" /> <link rel="stylesheet" type="text/css" href="css/catalog.css" /> <link rel="stylesheet" type="text/css" href="css/button.css" /> </head> <body> <?php include("oHeader.php"); ?> <?php include("config.php"); $queryPromo="SELECT * FROM promo"; $resultPromo=mysql_query($queryPromo); while($rowPromo=mysql_fetch_array($resultPromo)) { echo '<div id="promo">'; echo '<img src="data:image/jpeg;base64,'.base64_encode($rowPromo["image"]).'" width="940px" height="260px" />'; echo '</div>'; } ?> <p> <div id="cslay"> <?php include("oSide.php"); ?> <div id="content"> <?php include("config.php"); $query="SELECT * FROM product ORDER BY id DESC"; $result=mysql_query($query); while($row=mysql_fetch_array($result)) { ?> <div id="product"> <a href="pDetails.php?id=<?php echo $row['id']; ?>"> <img src="data:image/jpeg;base64,<?php echo base64_encode($row["prodImage"]);?>" width="190px" height="190px" /> </a> <p> <b><?php echo $row["prodID"]; ?></b> </p> <p><?php echo $row["prodName"]; ?></p> <p>RM<?php echo $row["prodPrice"]; ?></p> <p> <a href="pDetails.php?id=<?php echo $row['id']; ?>" class="button">Product Details</a> </p> </div> <?php } ?> </div> <div id="blay5"> </div> </div> </p> <?php include("oBottom.php"); ?> </body> </html>
This is my homepage code, i name it as index.phpwhatever i tick(checked) the product,it will show out at this page(homepage)
How should i write the code if checkbox checked then this product will show at homepage(index.php).
Thanks
Regards.
-
replace:
<input type="checkbox" name="homepage" value="1" <?php echo ($homepage == 1) ? 'checked="checked"' : ''; ?>/>
With:
<input type="checkbox" name="homepage" value="1" <?php echo ($row1['homepage'] == 1) ? 'checked="checked"' : ''; ?>/>
It's work. Thanks.
I got another question to ask..
Let say i have 10 product, only 2 of the product i tick the checkbox, and checkbox checked only list out the product. How should i write the code? I will show you the code after this post.
-
this is action page, i name it as proEditPros.php
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN"
"http://www.w3.org/TR/html4/strict.dtd">
<?php
@session_start();
if(!isset($_POST['update']))
{
header('Location:index.php');
}
?>
<html>
<head>
<title>De Closet | Update Product</title>
<link rel="stylesheet" type="text/css" href="css/lstyle.css" />
<link rel="stylesheet" type="text/css" href="css/tableStyle.css" />
<script>
document.onkeydown = function(){
switch (event.keyCode){
case 116 : //F5 button
event.returnValue = false;
event.keyCode = 0;
return false;
case 82 : //R button
if (event.ctrlKey){
event.returnValue = false;
event.keyCode = 0;
return false;
}
}
}
</script>
</head>
<body>
<?php
include("oHeader.php");
?>
<p>
<div id="cslay">
<?php
include("oSide.php");
?>
<div id="content">
<?php
include("config.php");
$prodID=$_POST["prodID"];
$prodName=$_POST["prodName"];
$prodPrice=$_POST["prodPrice"];
$prodCat=$_POST["prodCat"];
$prodImage=$_POST["prodImage"];
$prodDet=$_POST["prodDet"];
$homepage=$_POST["homepage"];
$queryCheck = "SELECT prodID FROM product WHERE prodID='$prodID'";
$resultCheck = mysql_query($queryCheck);
//if(mysql_num_rows($resultCheck)==0)
//{
/*
$allowedExts = array("gif", "jpeg", "jpg", "png");
$temp = explode(".", $_FILES["prodImage"]["name"]);
$extension = end($temp);
if ((($_FILES["prodImage"]["type"] == "image/gif")
|| ($_FILES["prodImage"]["type"] == "image/jpeg")
|| ($_FILES["prodImage"]["type"] == "image/jpg")
|| ($_FILES["prodImage"]["type"] == "image/pjpeg")
|| ($_FILES["prodImage"]["type"] == "image/x-png")
|| ($_FILES["prodImage"]["type"] == "image/png"))
&& ($_FILES["prodImage"]["size"] < 20000)
&& in_array($extension, $allowedExts))
{
if ($_FILES["prodImage"]["error"] > 0)
{
echo "Return Code: " . $_FILES["prodImage"]["error"] . "<br>";
}
else
{
}
}
else
{
//echo "Invalid file";
}
*/
if(!empty($_FILES['image']['tmp_name']))
{
$tmpName = $_FILES['prodImage']['tmp_name'];
// Read the file
$fp = fopen($tmpName, 'r');
$data = fread($fp, filesize($tmpName));
$data = addslashes($data);
fclose($fp);
$query="UPDATE product SET prodID='$prodID', prodName='$prodName', prodPrice='$prodPrice', prodCat='$prodCat', prodDet='$prodDet', prodImage='$data', homepage='$homepage' WHERE prodID='$prodID'" ;
$result = mysql_query($query) or die ('Error: '.mysql_error ()) ;
}
else
{
$query="UPDATE product SET prodID='$prodID', prodName='$prodName', prodPrice='$prodPrice', prodCat='$prodCat', prodDet='$prodDet', homepage='$homepage' WHERE prodID='$prodID'" ;
$result = mysql_query($query) or die ('Error: '.mysql_error ()) ;
}
if($result)
{
$query1="SELECT * FROM product WHERE prodID='$prodID'";
echo "You have update a product.<a href='products.php'>Click here</a> to continue.";
$querySelect="SELECT * FROM product WHERE prodID='$prodID'";
$resultSelect=mysql_query($querySelect) or die ('Error: '.mysql_error ()) ;
while($row=mysql_fetch_array($resultSelect))
{
?>
<table cellspacing='0' id="tStyle"> <!-- cellspacing='0' is important, must stay -->
<!-- Table Header -->
<thead>
<tr>
<th></th>
<th>Product Detail</th>
</tr>
</thead>
<!-- Table Header -->
<!-- Table Body -->
<tbody>
<tr>
<td>Product ID</td>
<td><?php echo $row["prodID"]; ?></td>
</tr><!-- Table Row -->
<tr class="even">
<td>Product Name</td>
<td><?php echo $row["prodName"]; ?></td>
</tr><!-- Darker Table Row -->
<tr>
<td>Price</td>
<td><?php echo "RM".$row["prodPrice"].""; ?></td>
</tr>
<tr class="even">
<td>Category</td>
<td><?php echo $row["prodCat"]; ?></td>
</tr>
<tr>
<td>Image</td>
<td>
<img src="data:image/jpeg;base64,<?php echo base64_encode($row["prodImage"]); ?>" width="190px" height="190px" />
</td>
</tr>
</tbody>
<!-- Table Body -->
</table>
<?php
}
}
else
{
echo "Failed to update.";
}
//}
//else
//{
//echo '<h4>Sorry! Product ID has been in the database. <a href="newProduct.php"> Try again </a></h4>';
//}
?>
</div>
<div id="blay5">
</div>
</div>
</p>
</body>
</html> -
this is interface name it as proEdit.php
<?php
@session_start();
if(!isset($_SESSION['admin']))
{
header('Location:index.php');
}
?>
<html>
<head>
<title>De Closet | Update Product</title>
<link rel="stylesheet" type="text/css" href="css/lstyle.css" />
<link rel="stylesheet" type="text/css" href="css/login.css" />
</head>
<body>
<?php
include("oHeader.php");
?>
<p>
<div id="cslay">
<?php
include("oSide.php");
?>
<div id="content">
<div id="login" style="width:648px">
<form action="proEditPros.php" method=POST style="width:420px" enctype="multipart/form-data">
<?php
include("config.php");
//$querySelect="SELECT * FROM product WHERE prodID='$prodID'";
//$resultSelect=mysql_query($querySelect) or die ('Error: '.mysql_error ()) ;
//$sql = "SELECT * FROM product WHERE homepage='".$_GET['homepage']."'";
//$result = mysql_query($sql);
//$row = mysql_fetch_array($result);
//$checked = $result['homepage'];
?>
<?php
include("config.php");
$query1="SELECT * FROM product WHERE id='".$_GET['id']."'";
$result1=mysql_query($query1);
while($row1=mysql_fetch_array($result1))
{
?>
<label for="prodID">Product ID:</label>
<input type="text" id="prodID" name="prodID" value="<?php echo $row1['prodID']; ?>" />
<br />
<label for="prodName">Product Name:</label>
<input type="text" id="prodName" name="prodName" value="<?php echo $row1['prodName']; ?>" />
<label for="prodPrice">Price(MYR):</label>
<input type="text" id="prodPrice" name="prodPrice" value="<?php echo $row1['prodPrice']; ?>" />
<label for="prodCat">Category:</label>
<input type="text" id="prodCat" name="prodCat" value="<?php echo $row1['prodCat']; ?>" />
<br />
<label for="pDetails">Product Details:</label>
<textarea rows="29" cols="50" name="prodDet" id="prodDet" value="<?php echo nl2br($row1['prodDet']); ?>"></textarea>
<br />
<td>
<!--<label><input type="checkbox" id="homepage" name="homepage" value="1" />Tick for show at Homepage for this product</label> -->
<input type="checkbox" name="homepage" value="1" <?php echo ($homepage == 1) ? 'checked="checked"' : ''; ?>/>
</td>
<br />
<br />
<label for="prodImage">New Main Image:</label>
<input type="file" name="prodImage" id="prodImage" />
<?php
?>
<!--<label for="prodImage">Images:</label>
<input type="file" name="prodImage" id="prodImage" />-->
<?php
}
?>
<div id="lower" style="background: #F5D0A9">
<input type="submit" name="update" value="Update Product" style="width:150px" />
</form>
<button style="width:100px"><a href="products.php">Back</a></button>
</div><!--/ lower-->
</div>
</div>
<div id="blay5">
</div>
</div>
</p>
<?php
include("oBottom.php");
?>
</body>
</html> -
Thanks for your answer. I tried the code that you gave. If i add the code, it will add 2 more checkbox out, 1 is checked and the other one is unchecked. 1 of the checkbox always show checked, even the value is 0.Here is a sample that works:
<?php $homepage = "0"; ?> <input type="checkbox" name="homepage" value="1" <?php echo ($homepage == 1) ? 'checked="checked"' : ''; ?>/> <?php $homepage = "1"; ?> <input type="checkbox" name="homepage" value="1" <?php echo ($homepage == 1) ? 'checked="checked"' : ''; ?>/>
I suspect your database value is not in $homepage.
try:
<?php var_dump($homepage); ?>
To see if the value is actually there from the DB.
<?php var_dump($homepage); ?>
It come out value is "NULL"I put this code at after the checkbox code.
What's wrong?
-
<input type="checkbox" name="homepage" value="1" <?php echo ($homepage == 1) ? 'checked="checked"' : ''; ?>/>
Where $homepage is the data retrieved from your database.
Thanks for your answer, i had tried your code, but the checkbox still show unchecked even my database value is 1
-
How could I declare checkbox, if checkbox is checked = 1 else = 0.And insert to mySQL.
<input type="checkbox" name="homepage" value="1" /> <-----this is my checkbox code. Only this i know.
somemore,if i already insert data to mySQL, how could i display checkbox is checked based on database, 1 = checked, 0 = unchecked.
I had try many code,but it won't work as well..Now i've no idea.
P/S:I'm using php code.
Please help.
Thanks.
Regards.
How to select count?
in PHP Coding Help
Posted
thanks for your answered.
if i do not want this part of code ($db = new mysqli(HOST,USERNAME,PASSWORD,DATABASE)
then