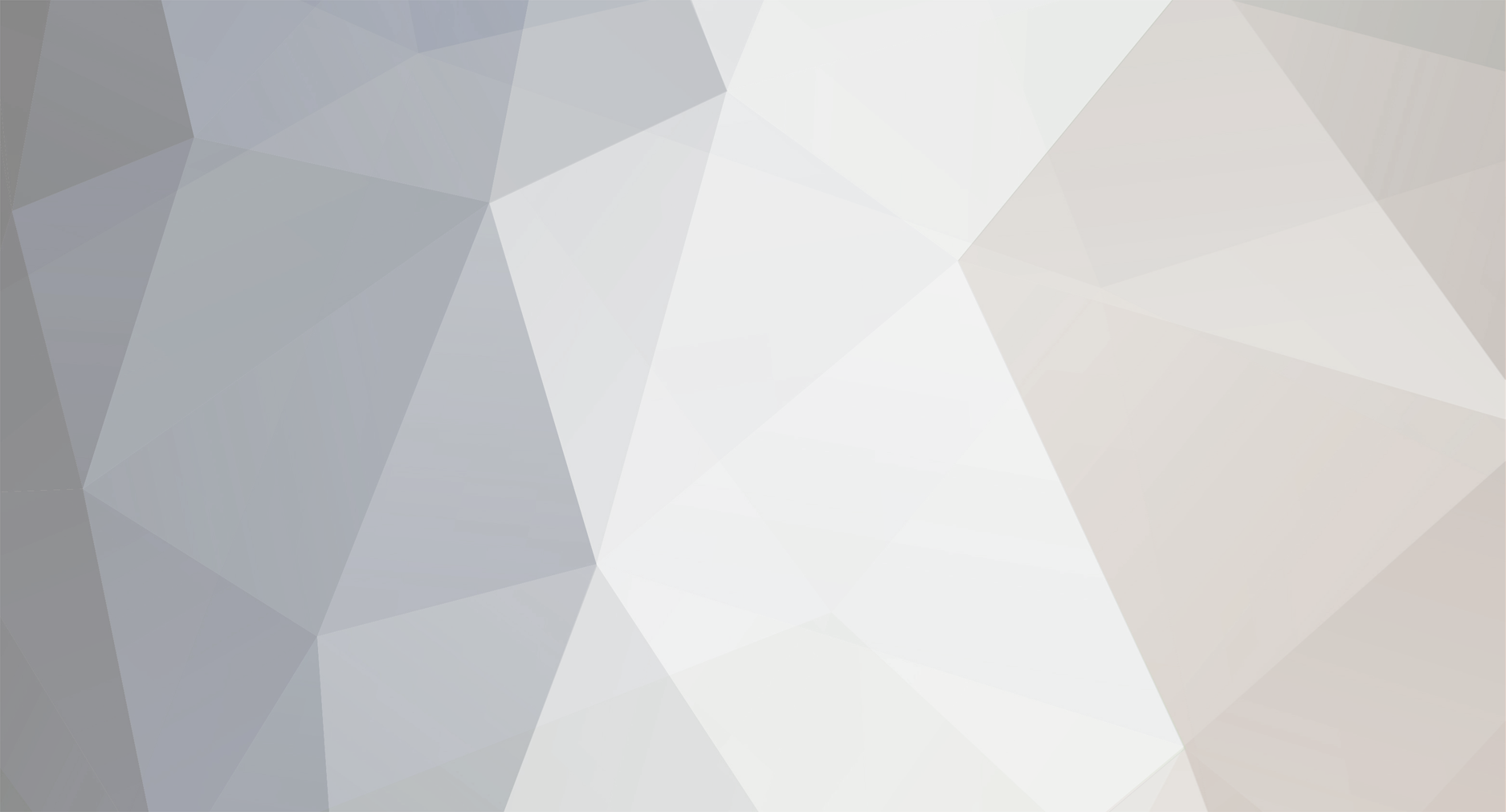
adam_bray
Members-
Posts
101 -
Joined
-
Last visited
-
Days Won
1
Everything posted by adam_bray
-
First impression - it's your first attempt at building a website, which is fine. A few things - 1. Your doctype is XHTML 1.0 - we're now at HTML 5, time for an upgrade! 2. Why have you confined your content to a scrolling box? It gives the effect of a frame, which is an old design feature - make it easy for people to read your content. 3. Your site name / logo is in a heading 4 (h4) tag, but you have multiple h1 tags. Look at improving your page structure as accessibility has an effect on SEO. 4. Look at choosing a nicer font, Times isn't the nicest to read. Set yourself some more realistic targets, you're not going to get rich from this site just yet. Install Google Analytics and Webmaster tools to understand what's going on with your site and how to draw in a larger audience. Once you understand that, you could start by aiming for 100 uniques per day, then 200, etc... but to earn "a lot of money", you're probably looking at minimum 20,000 users per day, more like 50,000 - 100,000.
-
placing an element at the bottom of a page centered
adam_bray replied to atlantis72's topic in CSS Help
The easiest way to do it is with flexbox, but it may require some browser prefixing to work universally. -
Have a read up on using a singleton design with your classes. I've applied it to your current code and removed the unnecessary implementations. Each constructor checks whether the class is already active, if it isn't then the class is constructed and stored. One function that's missing from this is the autoload function, something else to read up on. <?php class Database { // Store the class instance private static $instance = null; private function __construct() { // Add DB connection here } // Use a function to run the class constructor // Check if the class has already been initiated // Return class object public static function getInstance() { if( !isset( self::$instance ) ) { self::$instance = new Database; } return self::$instance; } } // create team class class Team { // Store the class instance private static $instance = null; // DB object private $db = null; private $team_name; private $team_id; private $result; // Check if the DB is active // If not get the DB instance private function __construct() { if( !isset( $this->db ) ) { $this->db = Database::getInstance(); } } // Singleton function // Call the constructor if the class hasn't been created public static function getInstance() { if( !isset( self::$instance ) ) { self::$instance = new Team; } return self::$instance; } //function to get team name public function getName( $team_id ) { $this->team_id = $team_id; $this->db->query( "SELECT team_name, nickname, founded FROM club WHERE team_id=:teamid" ); $this->db->bind( ':teamid', $this->team_id ); if( $result = $this->db->single() ); { return $result[]; } return false; } }// end class // Get the team id $team_id = 1; // Build the team object $team = Team::getInstance(); // call Team method $team_display = $team->getName($team_id); ?> I hope this answers your question.
-
This if statement isn't doing what you want it to - //If found, do next thing if(isset($_POST['Epost'])) If you're going to use that then place the select query within it as well. You want to replace that line (the one wrapping the delete query) with a count of the select results, checking the number of rows from the query doesn't equal 0. Also, use PDO or mysqli_ instead of mysql_, those functions are depreciated.
-
You're using $id in the select list where you want to use $cat_name, change this - <option value="<?php echo $id;?>"><?php echo $cat_name; ?></option>
-
Sorry, my bad! OP: Here's some slightly better code (untested). I know you might not understand most of what's in it, but Jacques1 will kindly explain it for you if you get confused. <?php /* * * DB CONNECTION INFO * */ $_mysql_info = array( 'user' => 'root', 'password' => '', 'host' => 'localhost', 'db' => 'User_Management', ); /* * * DEFINITIONS * */ if( !defined( 'MAX_USERNAME_LENGTH' ) ) { define( 'MAX_USERNAME_LENGTH', 15 ); } /* * * member.php * */ $mcuser = ( isset( $_GET['user'] ) ) ? $_GET['user'] : false; if( $mcuser ) { $mcuser = preg_replace("/[^A-Za-z0-9 ]/", '', $mcuser); $mcuser = ( strlen( $mcuser ) > MAX_USERNAME_LENGTH ) ? substr($mcuser, 0, MAX_USERNAME_LENGTH ) : $mcuser; try { $conn = new PDO('mysql:host='.$_mysql_info['host'].';dbname='.$_mysql_info['db'], $_mysql_info['username'], $_mysql_info['password']); $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); $qry = $conn->prepare(' SELECT Player_Data.Username , Player_Data.Rank , Player_Data.Tokens FROM Player_Data WHERE Username = :username LIMIT 1;'); $qry->execute( array( 'username' => $mcuser, )); $result = $qry->fetchAll(); if( count($result) > 0 ) { foreach( $result as $row ) { echo '<h1>Viewing '.$mcuser.' Profile</h1>'; foreach( $row as $key => $val ) { echo '<strong>'.$key.'</strong>:' . $val; } } } else { echo 'No rows returned.'; } } catch( PDOException $e ) { echo 'DB ERROR: ' . $e->getMessage(); } } else { try { $conn = new PDO('mysql:host='.$_mysql_info['host'].';dbname='.$_mysql_info['db'], $_mysql_info['user'], $_mysql_info['password']); $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); $qry = $conn->prepare(' SELECT Player_Data.Username , Player_Data.Rank , Player_Data.Tokens FROM Player_Data;'); $qry->execute( array( 'username' => $mcuser, )); $result = $qry->fetchAll(); if( count($result) > 0 ) { echo '<h1>Select Profile:</h1>'; foreach( $result as $row ) { echo '<a href="member.php?user=' . $row['username'] . '">'.$row['username'].'</a>'; } } else { echo 'No rows returned.'; } } catch( PDOException $e ) { echo 'DB ERROR: ' . $e->getMessage(); } } ?> RE your second question, look into mod_rewrite.
-
You don't need the switch in your code, that's for something else. You're also missing the point of $_GET.. you're using $_GET['username'], but then you're browsing to ?MCUser=about. To do that you need to use $_GET['MCUser']. Try this - <?php $MCUser = ( isset( $_GET['user'] ) ) ? $_GET['user'] : false; // Check if the user variable has been set if( $MCUser ) { $MCUser = mysql_real_escape_string( $MCUser ); // Basic protection against attacks $username = "root"; $password = ""; $host = "localhost"; $dbname = "User_Management"; $db_handle = mysql_connect($host, $username, $password) or die(mysql_error()); $db_found = mysql_select_db($dbname, $db_handle) or die(mysql_error()); $data = mysql_query("SELECT * FROM Player_Data WHERE Username = {$MCUser} LIMIT 1;") or die(mysql_error()); if( mysql_num_rows( $data ) > 0 ) { $user_info = mysql_fetch_array( $data ); print_r( $user_info ); } else { die('User not found!'); } } else { die( 'Please enter a user to search' ); // or show a list of users } ?> You need to browse to member.php?user=username.
-
You need to read up on using $_GET - my basic post on it. In the example above you'd do: <?php $member = $_GET['name']; // Sanitize the string // Query the database // Display the results ?> The comments are the steps following. When using $_GET with a MySQL query you need to check for malicious characters to prevent injections.
-
Looks like a normal array with keys. Try this - echo $image['url'];
-
Do something like this - <?php echo 'Shop ID: ' . $_GET['shop']; ?> <a href="page2.php?shop=<?=$_GET['shop'];?>"></a> The reason your first code isn't working is because you're using the wrong type of quotes. Single quotes don't execute PHP, double quotes do, but they try to execute everything. echo '<input type="hidden" name="shoppingid" value="<?php echo $_POST["shop"]; ?>'; Should be this - echo '<input type="hidden" name="shoppingid" value="'.$_POST["shop"].'" />';
-
Is the user submitting a form between every page? Wouldn't it be easier to use $_GET?
-
It didn't work because you didn't do what he suggested, you jumped to the end without changing anything. Yes^ You need to take the number variable out of the final array and use it as an array key. Here's an example - <?php // Array to store the shopping cart $cart = array(); // Products that are available // Product_id => array of product info // Could be from database instead $products = array( // Product 1 1 => array( 'size' => 'L', 'available' => 3, 'price' => '4.00', ), // Product 2 2 => array( 'size' => 'XL', 'available' => 8, 'price' => '4.00', ), // Product 3 3 => array( 'size' => 'S', 'available' => 1, 'price' => '3.50', ), ); // If a product id has been set if( isset( $_GET['pid'] ) ) { // Add the product to your cart variable $cart[$_GET['pid']][] = $products[$_GET['pid']]; // Tell the user what they've added print 'You have added a shirt in '.$cart[$_GET['pid']]['size'].' for $'.$cart[$_GET['pid']]['price'].' to your cart.'; // Store in session // Check it isn't already in the cart // Etc... } ?>
-
Have you setup the database and assigned a user to it? It doesn't look like a coding error, you just need to enter MySQL login details for the database.
-
This should fix it - <link rel="stylesheet" href="/css/main.css" /> <script type="text/javascript" src="/js/main.js"></script>
-
The HTML is all over the place, look at fixing that and see what happens.
-
Your code and database are really insecure, and you should use mysqli_* instead of mysql_* functions. This should work - <?php $user = mysql_real_escape_string($_SESSION['username']); $queryget = mysql_query('SELECT usertype FROM tbl_usernames WHERE user = \''.$user.'\' LIMIT 1;') or die ('Query failed. ' . mysql_error()); $row = mysql_fetch_assoc($queryget); if($row['usertype'] == 'admin') { echo '<p><a href="create_user.php">Create a new user</a></p>'; } else { echo 'You are a normal user.'; } ?>
-
All you need to do is add another $_GET variable, maybe call it limit? Then do an if statement to change the query depending on the variables value. To change the query you can do 1 of 2 things - Change the whole query Add a $limit variable to the query Something like this - $limit = (isset($_GET['limit']))? $_GET['limit'] : 9; if( $limit == 9 ) { $limit = 'LIMIT 0, 9'; } else { $limit = ''; } $query = mysql_query('SELECT * FROM table ' . $limit . ';');
-
Can't you use a foreach? $radiowarning = ' <div class="'.$bannerStyle.'"> <strong>SEVERE WEATHER ALERT - '.$event.'</strong> ... Listen now to our LIVE NOAA Weather Radio Stream <a href="'.$feed.'">[Click here to listen]</a> </div>'; //If alerts are in the source, set alert message $alerts = array($event); $searchvalue = array('Flood Watch', 'Flood Warning', 'Flash Flood Watch', 'Flash Flood Warning', 'Severe Thunderstorm Watch', 'Severe Thunderstorm Warning', 'Tornado Watch', 'Tornado Warning'); foreach( $searchvalue as $alert ) { if (in_array($alert, $alerts)) { echo $radiowarning; } }
-
How do you get search box's input terms into meta tags?
adam_bray replied to man5's topic in PHP Coding Help
It's simply down to the order you're processing the page - Step 1 - $searchsession = $_SESSION['searchsession']; Let's say $_SESSION['searchsession'] = '1'; This line is setting $searchsession = '1'; Step 2 - <title><?php echo $searchsession; ?></title> You echo $searchsession, so the title will be '1'. Step 3 - $value = $_GET['search']; You assign the variable $value the same value as $_GET['search'].. let's say that value is '2'. Step 4 - $_SESSION['searchsession'] = $value; You change the existing $_SESSION['searchsession'] variable to '2'. Step 5 - <form action="search.php?q=<?php echo $value; ?>" method="GET"> You echo the $value variable in your form, this is '2' as it's come from the $_GET['search'] variable. Look at the order things are happening above. What you're looking for is step 1 - 5 the variable to always be 2. By changing the order of the 5 steps above you'll get what you want. -
Where are you setting $order_by ? Add this at the top - $order_by = $_GET['order_by'];
-
Here's a very basic example of how to improve it. Read through the comments to understand the changes. There's still much more that can be done to improve this, but there's no point doing it all for you. <?php // Process the form if( isset( $_POST['r_p'] ) ) { // Let's add *some* protection to our strings $quantity = mysql_real_escape_string($_POST['r_q']); $name = mysql_real_escape_string($_POST['r_fn']); $sname = mysql_real_escape_string($_POST['r_ln']); $tel = mysql_real_escape_string($_POST['r_tn']); $email = mysql_real_escape_string($_POST['r_e']); $party = mysql_real_escape_string($_POST['r_p']); // Do some very basic checks against the posted variables if( strlen($name) < 3 || strlen($sname) < 3 || strlen($tel) < 1 || strlen($email) < 3 || (strlen($quantity) < 1 || !is_numeric( $quantity )) ) { echo '<p>Your form is incomplete</p> <p>Please fill in details</p>'; include_once('footlayout.html'); exit; } else { $quantity_query = mysql_query('SELECT Quantity FROM PARTY WHERE Name=\''.$party.'\' LIMIT 1;') or die( 'MySQL Error: ' . mysql_error() ); // Get the party info if( mysql_num_rows( $quantity_query ) > 0 ) // Check the party exists { $available = mysql_fetch_array( $quantity_query ); // Fetch the array $available = $available['r_q']; // Make the number of tickets available the variable if( $quantity <= $available ) // Check the user hasn't ordered too many tickets { $sql = mysql_query(' INSERT INTO Bookings (customerFName, customerSName, customerTelNo, customerEmail, tickets) VALUES(\''.$name.'\',\''.$sname.'\',\''.$tel.'\',\''.$email.'\',\''.$quantity.'\');') or die( 'MySQL Error: ' . mysql_error() ); // Insert the booking if( $sql ) // If the booking worked { echo 'Thank you '.$name.' for booking '.$quantity.' tickets to '.$party.'.'; // Tell them it worked! include_once('footlayout.html'); exit; } else // If the booking query didn't work { echo 'There\'s been a problem inserting into the DB!'; include_once('footlayout.html'); exit; } } else // If the user has ordered too many tickets { echo 'You\'ve selected more tickets than we can provide.'; include_once('footlayout.html'); exit; } } else // If the party doesn't exist { echo 'Cannot find '.$party.' in the database!'; include_once('footlayout.html'); exit; } } } // Display the form $party = $_GET['partyName']; ?> <form method="post" action="<?=$_SERVER['PHP_SELF'];?>"> <table border="0" cellspacing="0" cellpadding="5"> <tr> <td><label for="r_fn">First Name:</label></td> <td><input type="text" id="r_fn" name="r_fn" size="35" required="true" /></td> </tr> <tr> <td><label for="r_ln">Surname Name:</label></td> <td><input type="text" id="r_ln" name="r_ln" size="35" required="true" /></td> </td> <tr> <td><label for="r_tn">Telephone:</label></td> <td><input type="tel" id="r_tn" name="r_tn" size="35" required="true" /></td> </tr> <tr> <td><label for="r_e">Email Address:</label></td> <td><input type="email" id="r_e" name="r_e" size="35" required="true" /></td> </tr> <tr> <td><label for="r_e">Ticket Quantity:</label></td> <td><input type="number" id="r_q" name="r_q" required="true" /></td> </tr> <tr> <td colspan="2" align="center"> <input type="hidden" value="<?=$party;?>" name="r_p" /> <input type="submit" value="Request Ticket" /> </td> </tr> </table> </form>
-
Use margin: 0 auto; & floated elements