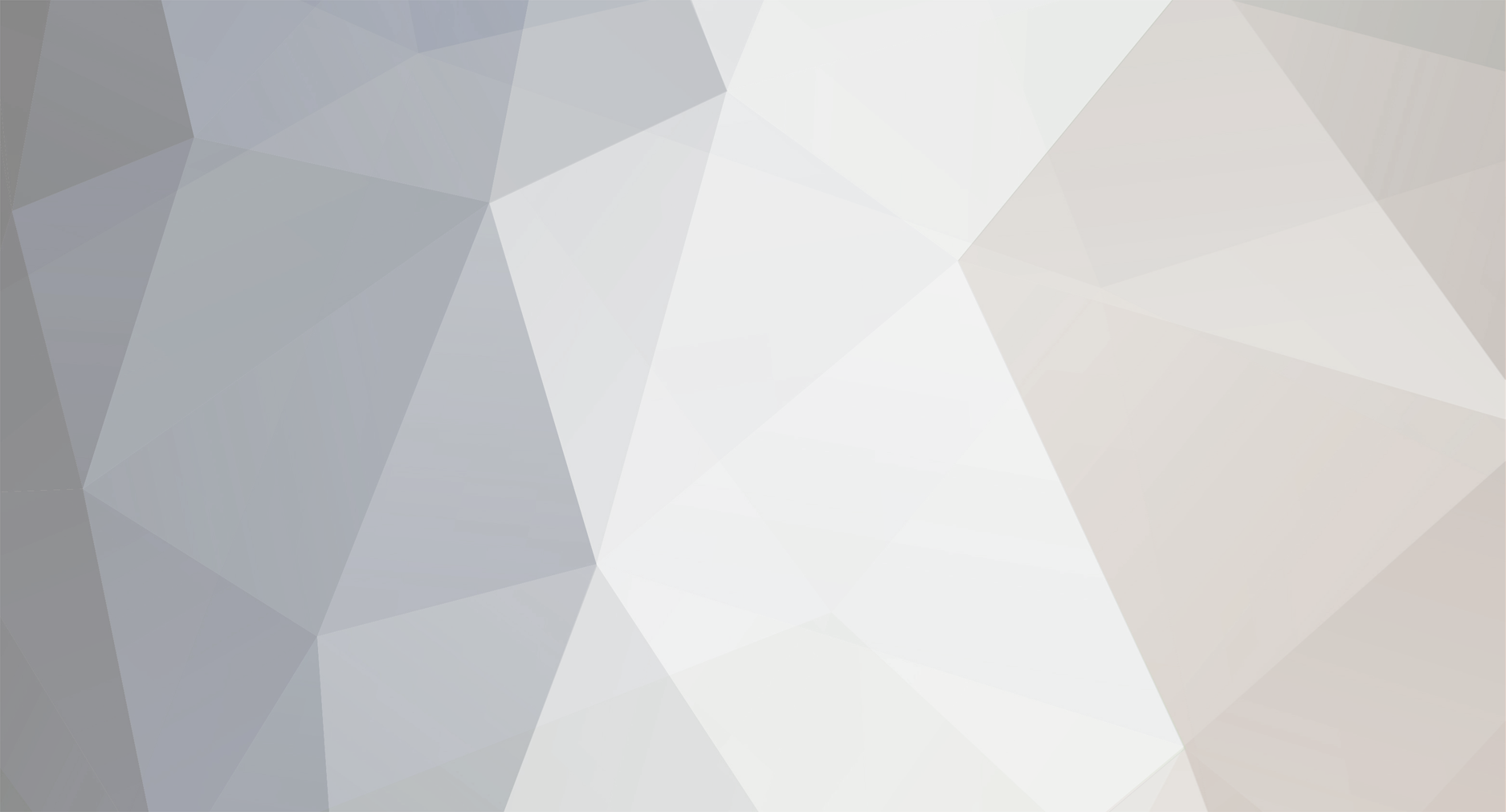
Deks1948
-
Posts
9 -
Joined
-
Last visited
Posts posted by Deks1948
-
-
<?php $con = mysql_connect('localhost', 'root', ''); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db('realestate'); $query="SELECT * FROM country"; $result=mysql_query($query); ?> <script language="javascript" type="text/javascript"> function getXMLHTTP() { //fuction to return the xml http object var xmlhttp=false; try{ xmlhttp=new XMLHttpRequest(); } catch(e) { try{ xmlhttp= new ActiveXObject("Microsoft.XMLHTTP"); } catch(e){ try{ xmlhttp = new ActiveXObject("Msxml2.XMLHTTP"); } catch(e1){ xmlhttp=false; } } } return xmlhttp; } function getState(countryId) { var strURL="findState.php?country="+countryId; var req = getXMLHTTP(); if (req) { req.onreadystatechange = function() { if (req.readyState == 4) { // only if "OK" if (req.status == 200) { document.getElementById('statediv').innerHTML=req.responseText; document.getElementById('citydiv').innerHTML='<select name="city">'+ '<option>Select City</option>'+ '</select>'; } else { alert("Problem while using XMLHTTP:\n" + req.statusText); } } } req.open("GET", strURL, true); req.send(null); } } function getCity(countryId,stateId) { var strURL="findCity.php?country="+countryId+"&state="+stateId; var req = getXMLHTTP(); if (req) { req.onreadystatechange = function() { if (req.readyState == 4) { // only if "OK" if (req.status == 200) { document.getElementById('citydiv').innerHTML=req.responseText; } else { alert("Problem while using XMLHTTP:\n" + req.statusText); } } } req.open("GET", strURL, true); req.send(null); } } </script>
<form method="post" action="" name="form1"> <center> <table width="45%" cellspacing="0" cellpadding="0"> <tr> <td width="75">City</td> <td width="50">:</td> <td width="150"><select name="country" onChange="getState(this.value)"> <option value="">Select City</option> <?php while ($row=mysql_fetch_array($result)) { ?> <option value=<?php echo $row['id']?>><?php echo $row['city']?></option> <?php } ?> </select></td> </tr> <tr style=""> <td>Type</td> <td width="50">:</td> <td ><div id="citydiv"><select name="city"> <option>Select Type</option> </select></div></td> </tr> </table> </center> </form> <?php $countryId=intval($_GET['country']); $stateId=intval($_GET['type']); $con = mysql_connect('localhost', 'root', ''); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db('realestate'); $query="SELECT id,city FROM city WHERE countryid='$countryId' AND stateid='$stateId'"; $result=mysql_query($query); ?> <select name="city"> <option>Select City</option> <?php while($row=mysql_fetch_array($result)) { ?> <option value><?php echo $row['type']?></option> <?php } ?> </select>
like that?
-
i'm having hard time thinking out how can i make a PHP dropdown search for a real estate website. So I have made the MYSQL cities and propertyes but i dont know how to make the categories and when you search like a MobilePhone to come another dropdown menu and to select the phone model and to display the results in another page..
the city list
<?php // // Database `realestate` // // `realestate`.`cities` $cities = array( array('id' => '1','name' => 'Burgas'), array('id' => '2','name' => 'Lovech'), array('id' => '3','name' => 'Pernik'), array('id' => '4','name' => 'Plovdiv'), array('id' => '5','name' => 'Razgrad'), array('id' => '6','name' => 'Rila'), array('id' => '7','name' => 'Ruse'), array('id' => '8','name' => 'Shumen'), array('id' => '9','name' => 'Sofia'), array('id' => '10','name' => 'Stara Zagora'), array('id' => '11','name' => 'Varna'), array('id' => '12','name' => 'Veliko Tarnovo') ); ?>
property type
<?php // // Database `realestate` // // `realestate`.`property` $property = array( array('id' => '1','name' => 'Huizen in de omgeving','property_type' => '0','property_subtype' => '0'), array('id' => '2','name' => 'Appartementen','property_type' => '0','property_subtype' => '0'), array('id' => '3','name' => 'Huizen','property_type' => '0','property_subtype' => '0'), array('id' => '4','name' => 'Bouwgrond','property_type' => '0','property_subtype' => '0'), array('id' => '5','name' => 'Commercieel','property_type' => '0','property_subtype' => '0'), array('id' => '6','name' => 'Te Huur','property_type' => '0','property_subtype' => '0') ); ?>
-
functions.php
<?php function get_menu() { $r = mysql_query ("select * from cats"); $result = "<a href=index.php>Main page</a><br>"; while ($row=mysql_fetch_array($r)) $result .= "<a href=index.php?p=filter&cat=$row[id]>$row[cname]</a><br>"; return $result; } function get_comments($id) { $id = strip_tags($id); $result = ""; if (is_numeric($id)) { $r = mysql_query("select * from comments where rid=$id order by id desc"); while ($row=mysql_fetch_array($r)) { $dt = date('d-m-Y', $row[dt]); $result .= "<hr><a href=mailto:$row[email]>$row[uname]</a> ($dt) <p>$row[txt]"; } } else $result = "Wrong argument in get_comments()"; return $result; } function get_blog_record($id) { $id = strip_tags($id); if (is_numeric($id)) { $r = mysql_query("select * from blog where id=$id limit 1"); $row = mysql_fetch_array($r); $dt = date('d-m-Y', $row[dt]); $comments = get_comments($id); $result = "<h1>$row[header]</h1> <p>$dt<p> <b>$row[anonce]</b> <p>$row[txt] <p><h2>Comments</h2><p><a href=add_comment.php?id=$id>Add comment</a>$comments"; } else $result = "Wrong entry ID"; return $result; } function get_cat_name($id) { $id = strip_tags($id); if (is_numeric($id)) { $r = mysql_query("select * from cats where id=$id limit 1"); $row = mysql_fetch_array($r); return $row[cname]; } else return "Wrong category"; } function get_cat($id) { $cat = $_GET[cat]; if (is_numeric($cat)) { $q="select * from blog where cid=$cat order by id desc"; $r = mysql_query($q); $list = ""; while ($row=mysql_fetch_array($r)) { $dt = date('d-m-Y H:i', $row[dt]); $list .= "<p><table width=100%> <tr><td bgcolor=grey width=80%> <font color=white>$row[header]</td> <td bgcolor=grey width=20%> <font color=white>$dt</td> </tr></table>"; $list .= "<p>$row[anonce]" $cat = get_cat_name($row[cid]); $list .= "<p><table width=100%> <tr><td width=60%><a href=index.php?p=show&id=$row[id]>Read more</a></td> <td width=20%>$cat</td> <td width=20%>Comments: $row[comments]</td> </tr></table>"; } return $list; } else return "Wrong category ID"; } ?>
index.php
<?php include "config.php"; mysql_connect($server, $username, $password); mysql_select_db($db); include "functions.php"; $header = join('', file('header.html')); $footer = join('', file('footer.html')); echo $header; $left = get_menu(); $content = ""; if (isset($_GET['p'])) { $p = $_GET['p']; $id = $_GET['id']; if ($p == "show") $content = get_blog_record($id); elseif ($p == "filter") $content = get_cat($cat); else $content = "Wrong page"; } else { $N = 5; $r1=mysql_query("select count(*) as records from blog"); $f = mysql_fetch_row($r1); $rec_count = $f[0]; if (!isset($_GET['page'])) $page=0; else $page = $_GET['page']; $records = $page * $N; $q='select * from blog order by id desc limit '.$records.", $N"; $result = mysql_query($q); $max = mysql_num_rows($result); if ($page > 0) { $p = $page - 1; $content .= "<a href=index.php?page=$p>Back</a> "; } $page++; if ($records+$N < $rec_count) $content .= "<a href=index.php?page=$page>Next</a>"; for ($i=0; $i<$max; $i++) { $row=mysql_fetch_array($result); $dt = date('d-m-Y H:i', $row[dt]); $content .= "<p><table width=100%> <tr><td bgcolor=grey width=80%> <font color=white>$row[header]</td> <td bgcolor=grey width=20%> <font color=white>$dt</td> </tr></table>"; $content .= "<p>$row[anonce]"; $cat = get_cat_name($row[cid]); $content .= "<p><table width=100%> <tr><td width=60%><a href=index.php?p=show&id=$row[id]>Read more</a></td> <td width=20%>$cat</td> <td width=20%>Comments: $row[comments]</td> </tr></table>"; } } echo "<table border=0 width=100%> <tr><td valign=top width=20%>$left</td><td valign=top>$content</td></tr></table> $footer"; ?>
-
And still the only thing that loads to my website is the simple main page html...
-
XAMPP.
Even if i get it on a hosting that i have I get the same error!?!
-
Error 404 localhost
Apache/2.4.7 (Win32) OpenSSL/1.0.1e PHP/5.5.9
-
Everything in the other files are Ok, but I thought that it was something to do with my database config file
-
so this is my database name and when i get it to a hosting or anything it just gives me Error 404?
<?php $server = "localhost"; $user = "deks"; $password = "cskasofia"; $db = "test.php"; ?>
Multiply search box
in PHP Coding Help
Posted
Is there any tutorial that I could find to make it more simple??