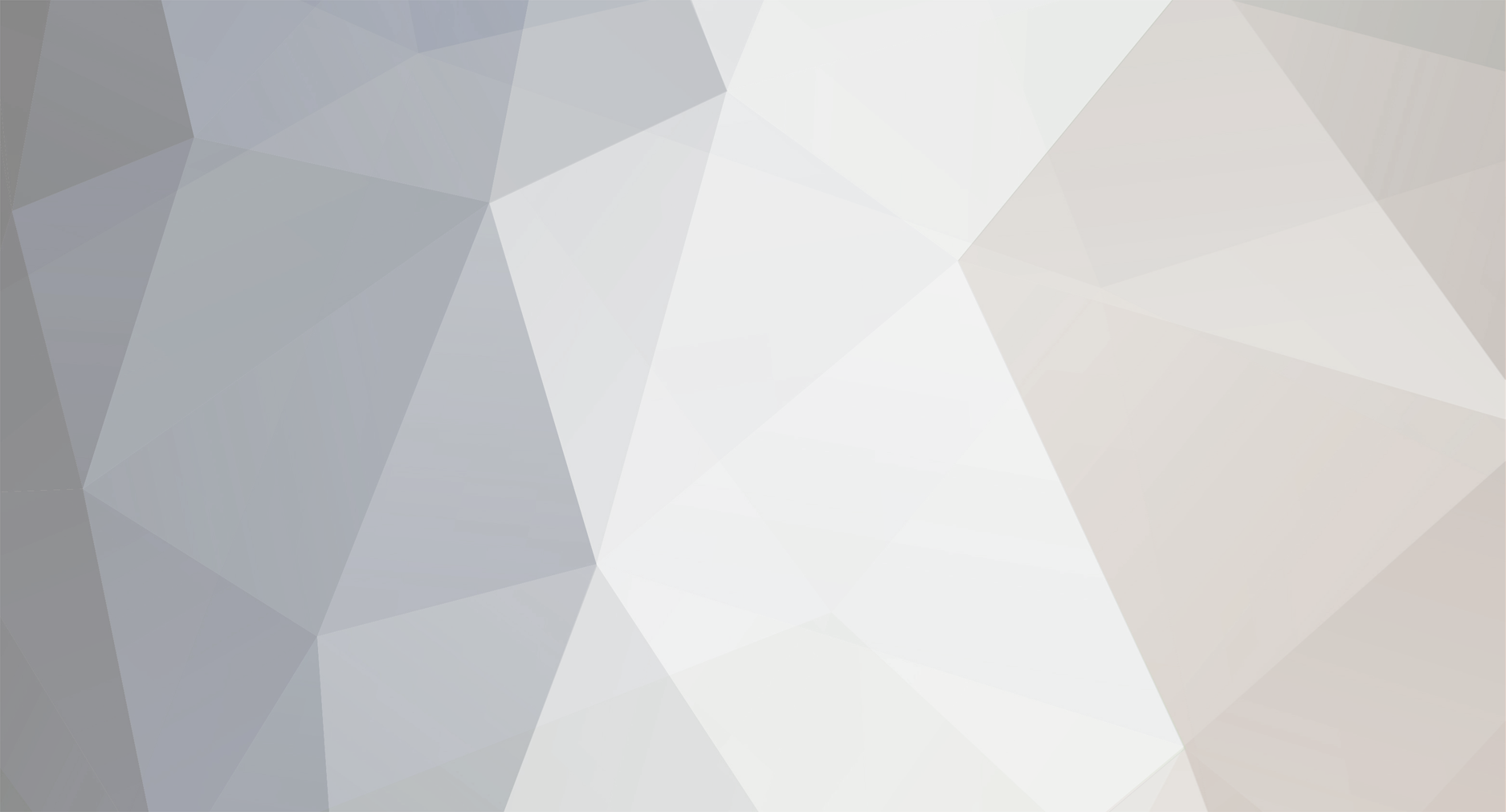
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
Your code will not be prone to SQL Injection attacks as you are not using any data coming form the user in the your queries. If you have hard coded queries in your app then they cannot be hijacked. Only queries which use user data is prone to SQL Injection attacks, eg: $username = $_POST['username']; $password = $_POST['password']; $query = "SELECT * FROM users_table WHERE username='$username' AND password='$password'"; The query used above is prone to SQL Injection as we are using raw user data in the query. For example someone could enter ' OR 1=1 --' as the username. When our script runs the query becomes (user input is highlighted in red): Which tells mysql to Select all rows from the users table where username is blank or select the first entry from the table. It will ignore the rest of the query as mysql sees -- as a comment. This is a perfectly valid SQL Injection attack and very serious one too. There are far more worse ones however there is a very simple way to prevent this attact and thats to use a function called mysql_real_escape_string, eg: $username = mysql_real_escape_string($_POST['username']); $password = mysql_real_escape_string($_POST['password']); $query = "SELECT * FROM users_table WHERE username='$username' AND password='$password'"; Now any quotes (or any other malicious characters) which can affect our query are escaped and thus our very unsecure script is now secured. There are many articles on the web which explains and provides tips to prevent SQL Injection.
-
How can I alias the virtual host document root to / ?
wildteen88 replied to mesh2005's topic in Apache HTTP Server
PHP does not request for files over the http protocol. Instead it does it via the OS You should never begin file paths with a /. Starting file paths in this way tells PHP to look for files from the root of the hard drive. Rather than your sites document root. I recommend you to use include "./header.php"; or a better way would be include $_SERVER['DOCUMENR_ROOT'] . "/header.php"; -
403 errors with any file beginning with "wht"
wildteen88 replied to tsw's topic in Apache HTTP Server
Have a look through Apaches error logs too. Seems a strange issue to me. -
In order for GD to be enabled on *nix based servers, PHP must be complied with the --with-gd configure command and you must enable the php_gd2.so extension in the php.ini too. Read the docs for the official steps.
-
Remove the quotes perhaps? if(isset($_POST[$fields[1]])) $row['FirstName']=$_POST[$fields[1]]; if(isset($_POST[$fields[2]])) $row['LastName']=$_POST[$fields[2]]; if(isset($_POST[$fields[3]])) $row['CompanyName']=$_POST[$fields[3]];
-
How is the id coming to the script? If its via the url then you'd use $_GET['id'] rather than $_POST['id'] $_POST is used when getting data from a form. $_GET returns data in the url.
-
mysql_real_escape_string wont affect the @ symbol. Does the email address validate if you don't run mysql_real_escape_string Hmm no it doesn't it still returns false Then there must be a problem with your regular expression if a valid email address format is not passing your checks.
-
mysql_real_escape_string wont affect the @ symbol. Does the email address validate if you don't run mysql_real_escape_string
-
Its basically the same as your add/edit pages. Accept this time you run a DELETE query, eg: DELETE FROM news where id=$id
-
try: <?php require 'includes/config.php'; // Make a MySQL Connection mysql_connect($path, $username, $password) or die(mysql_error()); mysql_select_db($database) or die(mysql_error()); $query1 = "SELECT * FROM news ORDER BY sticky, id ASC"; $result1 = mysql_query($query1) or die(mysql_error()); function printNews($result) { if ( mysql_num_rows($result) < 1 ) { echo "<h1>No news!</h1>"; } else { echo "<table cellspacing=\"2\" cellpadding=\"5\">\n"; while($news = mysql_fetch_assoc($result)) { $sticky = ($news['sticky'] == 'y') ? "<font color='red'><u>Announcement:</u></font> " : ''; echo <<<HTML <tr> <th align="left">{$sticky}<b>{$news['title']}</b></th> <td rowspan="2" valign="bottom" align="left"> <a href="news_edit.php?id={$news['id']}">Edit</a><br /> <a href="news_delete.php?id={$news['id']}">Delete</a> </td> </tr> <tr> <td>{$news['news']}</td> </tr> HTML; } echo "\n</table><br />"; } } printNews($result1); ?> Note: make sure you change news_edit.php and news_delete.php to the correct page which edits/deletes the news.
-
This: echo "<gallery> <image>\n"; should be: echo "\n<gallery> <image>\n"; Also this: echo ' </image> <gallery>'; should be: echo ' </image> </gallery>';
-
[SOLVED] Quick question, add Slash in the end
wildteen88 replied to mendoz's topic in Apache HTTP Server
Add the following: RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_URI} !(.*)/$ RewriteRule ^(.*)$ http://yoursite.com/$1/ [R=301,L] at the top of your .htaccess after RewriteEngine On. Change yoursite.com to your website address. This will check to see if the url ends in a / if it doesn't it'll add it in. -
I missed a period from my code, I forgot to correct it before I posted the code. Fixed code <?php //define the xml standard header("Content-type: text/xml"); echo "<?xml version='1.0' encoding='ISO-8859-1'?>"; //this section calculates the image names in the directory $imgdir = 'img/'; // the directory, where your images are stored $allowed_types = array('png','jpg','jpeg','gif'); // list of filetypes you want to show $dimg = opendir($imgdir); $url = 'http://' . $_SERVER['HTTP_HOST'] . '/' . $imgdir; echo "<gallery> <image>\n"; while($imgfile = readdir($dimg)) { if(in_array(strtolower(substr($imgfile,-3)),$allowed_types)) { echo ' <url>' . $url . $imgfile . "</url>\n"; } } echo ' </image> <gallery>'; ?> If you still get a blank screen then you should turn on display_errors
-
It is because your code relies up on register_globals. register_globals is depreciated and is being removed from PHP6. <?php $title = "MASTER RESET"; include "header.php"; if ($stat['rank'] != 'Admin') { print "You're not an admin."; include "footer.php"; exit; } Print "<form method=post action=masterreset.php?step=reset> Password: <input type=text name=pass></form>"; if ($_GET['step'] == 'reset') { if ($_POST['pass'] != 'abc') { Print "Wrong Password"; } else { Print "Password Correct!"; } } include "footer.php" ; ?> You should use the newer superglobals
-
Try: <?php //define the xml standard header("Content-type: text/xml"); echo "<?xml version='1.0' encoding='ISO-8859-1'?>"; //this section calculates the image names in the directory $imgdir = 'img/'; // the directory, where your images are stored $allowed_types = array('png','jpg','jpeg','gif'); // list of filetypes you want to show $dimg = opendir($imgdir); $url = 'http://' . $_SERVER['HTTP_HOST'] . '/' . $imgdir; echo "<gallery> <image>\n"; while($imgfile = readdir($dimg)) { if(in_array(strtolower(substr($imgfile,-3)),$allowed_types)) { echo ' <url>' $url . $imgfile . "</url>\n"; } } echo ' </image> <gallery>'; ?>
-
If you post your current code we maybe able to help you.
-
Helps if you post the code and provide a helpful description of what you're trying to do too.
-
<?php require 'includes/config.php'; // Make a MySQL Connection mysql_connect($path, $username, $password) or die(mysql_error()); mysql_select_db($database) or die(mysql_error()); if(isset($_POST['submit'])) { if(is_numeric($_POST['id'])) { $id = $_POST['id']; $title = mysql_real_escape_string($_POST['title']); $news = mysql_real_escape_string($_POST['news']); //Update it! $query = "UPDATE news SET title = '$title', news = '$news' WHERE id = '$id'"; mysql_query($query) or die(mysql_error()); } else { die( 'invalid action' ); } } elseif(isset($_GET['id']) && is_numeric($_GET['id'])) { $id = $_GET['id']; $query = "SELECT title, news FROM news WHERE id='$id'"; $result = mysql_query($query); list($news_title, $news_text) = mysql_fetch_row($result); ?> <form action="<?php echo $_SERVER['PHP_SELF'] ?>" method="post"> <p>Title:<br> <input type="text" name="title" value="<?php echo $news_title ?>" /> </p> <p>Text:<br> <textarea rows="10" cols="50" name="news"><?php echo $news_text ?></textarea> </p> <input type="hidden" name="id" value="<?php echo $id; ?>"> <input type="submit" name="submit" value="Submit"> </form> <?php } ?>
-
Got there in the end
-
That is because it'll only work if you submit the form. YOu'll need to change: if(isset($_POST['submit'])) { //Set the variables! $id = basename($_SERVER['PHP_SELF']); to: $id = (isset($_GET['id']) && is_numeric($_GET['id'])) ? $_GET['id'] : null; if(isset($_POST['submit'])) {
-
Where are you using the code and what is it supposed to do?
-
Use: if($user_check > '0') { if(!isset($_SESSION['time']) || ((time()-$_SESSION['time']) > 15)) { $_SESSION['time'] = time(); } }
-
Just looking at your code why use two queries to update the news title/news content in two queries: $query1 = "UPDATE news SET title = '$title' WHERE id = '$id'"; $query2 = "UPDATE news SET news = '$news' WHERE id = '$id'"; //Update the title and text! mysql_query($query1) or die(mysql_error()); mysql_query($query2) or die(mysql_error()); Use just one: //Update the title and text! $query = "UPDATE news SET title = '$title', news = '$news' WHERE id = '$id'"; mysql_query($query) or die(mysql_error()); To get the id from the url use $_GET['id']
-
Document Root Issue - Sub Directory on Godaddy
wildteen88 replied to trunker's topic in PHP Coding Help
You might want to fix your html/css as your layout is screwed in firefox. -
<?php require('config.php'); // Make a MySQL Connection mysql_connect("$path", "$username", "$password") or die(mysql_error()); mysql_select_db("$database") or die(mysql_error()); if(isset($_GET['action']) && $_GET['action'] == 'add') { $title = mysql_real_escape_string($_POST['title']); $news = mysql_real_escape_string($_POST['news']); //Check thate status of the sticky radio button $sticky = (isset($_POST['sticky']) && $_POST['sticky'] == 'y') ? 'y' : 'n'; //Insert the news $query = mysql_query("INSERT INTO news (title, news, sticky) VALUES ('$title', '$news', '$sticky')") or die(mysql_error()); echo "<meta http-equiv='refresh' content='1;url={$_SERVER['PHP_SELF']}'> News posted!"; } elseif(isset($_GET['action']) && $_GET['action'] == 'del') { //Delete all non-sticky news if(isset($_POST['deln'])) { $qry = 'DELETE FROM news WHERE sticky=\'n\''; $msg = 'All <b>non-stickied</b> news deleted!'; } //Delete all stickied news elseif(isset($_POST['dels'])) { $qry = 'DELETE FROM news WHERE sticky=\'y\''; $msg = 'All <b>stickied</b> news deleted!'; } //Delete all news elseif(isset($_POST['dela'])) { $qry = 'TRUNCATE TABLE news'; $msg = 'All news deleted!'; } else { die('Invalid action'); } mysql_query($qry)or die(mysql_error()); echo "<meta http-equiv='refresh' content='1;url={$_SERVER['PHP_SELF']}'> $msg"; } else { ?> <title>Add/Delete Recent News</title> <a href="../">Click Here to go home.</a> <form action="<?php echo $_SERVER['PHP_SELF'] ?>?action=add" method="post"> <p>Title:<br> <input type="text" name="title" /></p> <p>Text:<br> <textarea rows="10" cols="50" name="news"></textarea></p> <p>Sticky: <input type="radio" name="sticky" value="y" /> YES | <input type="radio" name="sticky" value="n" /> NO</p> <input type="submit" name="postnews" value="Post News"> </form> </form> <br><br> <h4>Options:</h4> <form action="<?php echo $_SERVER['PHP_SELF'] ?>?action=del" method="post"> <input type="submit" name="dela" value="Delete All News"> <input type="submit" name="deln" value="Delete Non-Sticky News"> <input type="submit" name="dels" value="Delete Sticky News"> </form> <u>Current features:</u> <br> <i>Quick Post News <br> Delete all regular news with one click <br> Delete all stickied news with one click <br> Option to title your news <br> "Sticky" News <br> Neatly displays all posted news in order from sticky to regular</i> <br><br><br> <u>Coming soon:</u> <br> <i>Ability to edit news <br> Ability to make stickied news regular and vise versa</i> <?php } ?>