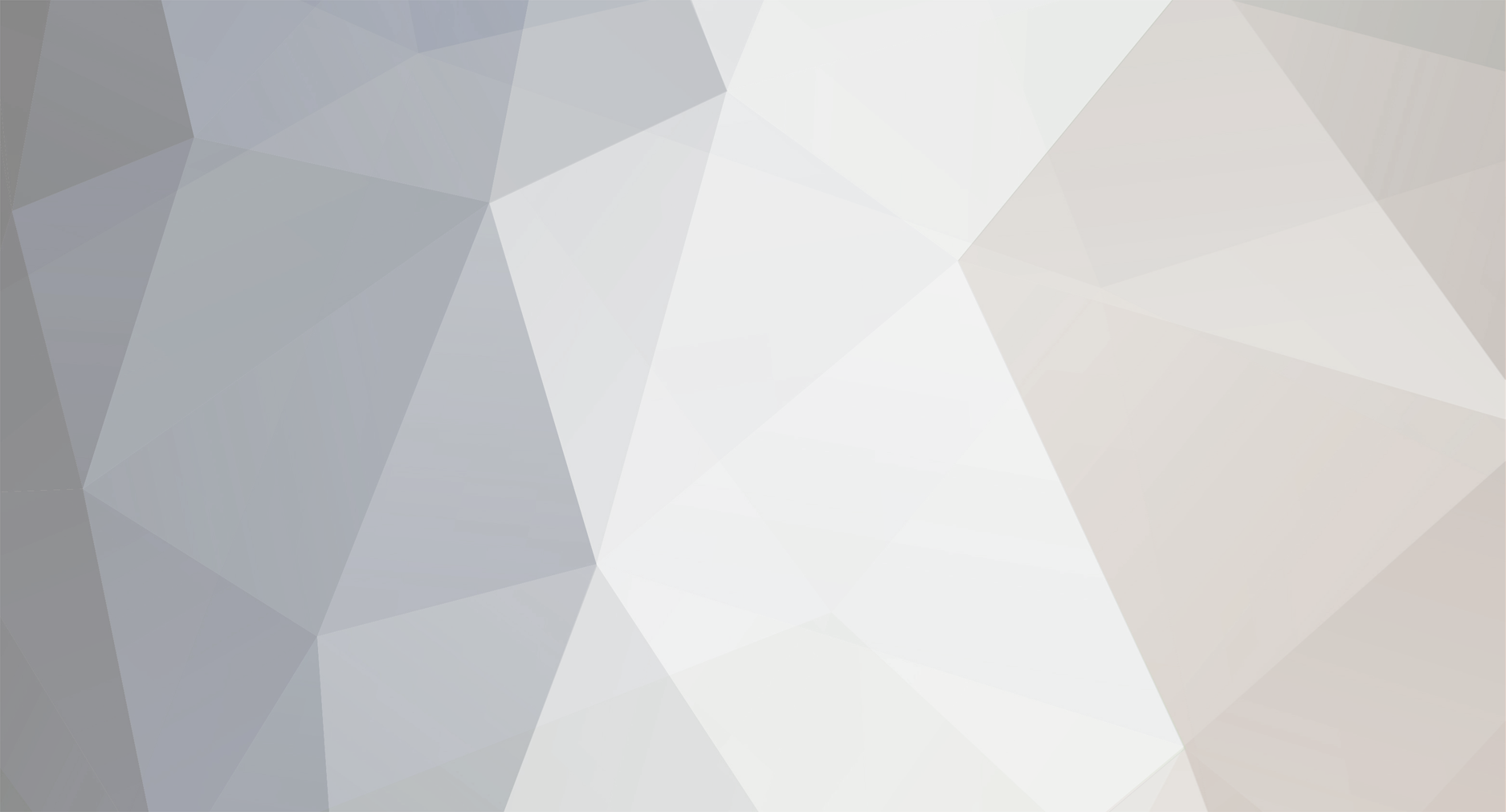
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
I guess you are submitting the form when the user chooses an item from the list, if so use $_POST['name'] or $_GET['name'] depending on your forms submit method.
-
To change the error_reporting/display_error setting in the php.ini go to line 353
-
You can setup PHP to disable certain functions/classes which will prevent users from using these function in thier scripts.
-
You'll need to use a client side language such as Javascript to get the time from the users computer. PHP is server side so it'll always return the time from the server.
-
Get data from mysql table from different records of a field
wildteen88 replied to jkkenzie's topic in PHP Coding Help
Can you explain what you're trying to do with the variables $point1, $point2 etc? -
Use $array[0]['Day'], $array[0]['Time'] and $array[0]['TOD'] to output the Day, Time and TOD for the first row returned from the query, $array[1]['Day'], $array[1]['Time'] and $array[1]['TOD'] for the secound row, $array[2]['Day'], $array[2]['Time'] and $array[2]['TOD'] for the third etc. You can simply loop through the $array variable using a foreach: foreach($array as $row_data) { list($day, $time, $tod) = array_values($row_data); echo '<p>Day: ' . $day . '<br >'; echo '<p>Time: ' . $time . '<br >'; echo '<p>TOD: ' . $tod . '</p>'; }
-
Get data from mysql table from different records of a field
wildteen88 replied to jkkenzie's topic in PHP Coding Help
mysql_query does not return data from a query, it only returns a result resource identifier which will be used with functions such as mysql_fetch_array to retrieve the data from the database. What are you trying to do? Retrieve the the first 19 rows from the tblkenya table? If you only want 19 results then use LIMIT to limit the amount of rows to be returned, eg: $result = mysql_query("SELECT * FROM tblkenya ORDER BY ID ASC LIMIT 19"); while($row = mysql_fetch_assoc($result)) { echo '<pre>' . $row . '</pre>'; } when usinga ny of the mysql_fetch_* functions in a while loop it'll return each row one by one every time PHP iterates through the while loop. -
You'd use CSS to apply to styles on your webpage, eg: pre { background-color: #e1e1e1; } That will apply a light grey background colour to all pre tags.
-
Imagemagick installation troubles.
wildteen88 replied to quiettech's topic in PHP Installation and Configuration
You can only use extensions which are of the same build (API version) as the PHP executable. You have PHP5.2.5 installed and you are using an extension released for PHP5.2.1 you'll need to find an updated version. -
Web browsers ignore any whitespace you'll need to force the browser to display such whitespace as discomatt suggests.
-
I need help getting my SQL database onto my apache web server
wildteen88 replied to cshell_1987's topic in PHP Coding Help
If you have download WAMPServer 2, just run the installer to install the AMP stack (Apache, MySQL and PHP). WAMP will setup AMP for you, it also comes with phpMyAdmin too. You will not need to configure anything. You save all your web files (html/php/image/css files etc) in C:\wamp\www you access these files by opening your browser and going to http://localhost to run your php scripts. For transferring your database you can go into phpMyAdmin and export any database you like to a dump file. This dump file will contain all the data in your database tables. Then on your other site you login to phpMyAdmin and create an empty database, when the new database is created simply click the Import tab at the top of the page to import your database dump file. Your database tables will be created and populated with your data. There are many tutorials for using MySQL with PHP, one such site is http://www.php-mysql-tutorial.com/ also techs you how to install the AMP stack manually on Windows. -
You have an erro in your sql query if your are getting that error, what does the following return: $query = 'SELECT * FROM name'; $getData = mysql_query($query) or die('SQL Query Error: ' . mysql_error() . '<br />Query: <pre>' . $query . '</pre>'); while ($result = mysql_fetch_assoc($getData)) { echo $result['fieldname'] . "<br />"; }
-
Is it possible to achieve with mod_rewrite...
wildteen88 replied to quickstopman's topic in Apache HTTP Server
If Apache is installed on a Linux server then the following should work: RewriteRule ^action:([a-z]+)$ /action.php?action=$1 [NC,L] -
Because your are using $_SERVER['REQUEST_URI'] it contains everything in the url, including query strings. This is why it keeps adding &diff=whatever when you go PS2.php Use $_SERVER['SCRIPT_NAME'] or $_SERVER['PHP_SELF']
-
Before using user input variables ($_GET, $_POST, $_COOKIE etc) you should always check to see if they exists first and apply some form of validation: if(isset($_POST['chkno']) && is_array($_POST['chkno'])) { $chknotest = $_POST['chkno']; print_r($chknotest); } else { echo '$_POST[\'chkno\'] empty'; }
-
Is it possible to achieve with mod_rewrite...
wildteen88 replied to quickstopman's topic in Apache HTTP Server
I have done a bit of googling and this is an issue to do with libapr when Apache is installed on Windows. Due to Windows not allowing characters such as colons within file names libapr is not allowing the requested url. However strangely enough if your url is say - mysite.com/foo/bar:test - then it'll work fine. Whether a fix is going to be released or not in the next/future versions of Apache for Windows I don't know. This only affects Apache installations on Windows. -
Is it possible to achieve with mod_rewrite...
wildteen88 replied to quickstopman's topic in Apache HTTP Server
You cannot use special characters such as colons as part of a url path. You can have your url as /action/signup or just /signup RewriteBase / RewriteRule ^action/([a-z]+)$ /test.php?action=$1 [NC,L] # eg: action/signup RewriteRule ^([a-z]+)$ /test.php?action=$1 [NC,L] # eg: /signup -
You'll need to setup the keys in the form fields so they match up, eg instead of: <input type="text" name="customer[]" value="Bob" /> <input type="checkbox" name="target[]" value="Y" /> Target <input type="text" name="customer[]" value="John" /> <input type="checkbox" name="target[]" value="Y" /> Target <input type="text" name="customer[]" value="Ann" /> <input type="checkbox" name="target[]" value="Y" /> Target DO: <input type="text" name="customer[0]" value="Bob" /> <input type="checkbox" name="target[0]" value="Y" /> Target <input type="text" name="customer[1]" value="John" /> <input type="checkbox" name="target[1]" value="Y" /> Target <input type="text" name="customer[2]" value="Ann" /> <input type="checkbox" name="target[2]" value="Y" /> Target If you let the browser set the keys then your fields/checkboxes values will become out of sync. Then in PHP do: foreach($_POST['customer'] as $key => $value) { echo 'Customer: ' . $_POST['customer'][$key] . ' Checkbox: ' . (isset($_POST['target'][$key]) ? $_POST['target'][$key] : 'N') . '<br />'; }
-
Exporting large data into text file - PHP MySQL
wildteen88 replied to shergar1983's topic in PHP Coding Help
mysql_result is rather old fashioned. Use mysql_fetch_assoc instead allows for much cleaner code: <?php set_time_limit(0); $date = date("Y-m-d"); $filename = 'test.csv'; $myFile = '../Export/Reports/'.$filename; $fh = fopen($myFile, 'w') or die('can\'t open file'); $stringData = '"Our Ref","Ref2","Client Type","Segment","Customer Name","Add 1","Add 2","Add 3","Add 4","Add 5",'. '"Post Code","Phone available","Birthday","Last Actioned","Balance","Last Payment","Mode","Status"'."\r\n"; fwrite($fh, $stringData); include '../../files/library/connection.php'; $query = 'SELECT * FROM ids, details, adds, short_status WHERE details.name_id=ids.id AND details.address_id=adds.add_id AND short_status.short_status=details.short_status and details.birthday>=\'2006-01-01\''; $result = mysql_query($query); while ($row = mysql_fetch_assoc($result)) { $row['phones'] = ($row['phones'] >= '1') ? 'Yes' : 'No'; $row['birthday'] = ($row['birthday'] == '0000-00-00') ? 'Error' : $row['birthday']; $row['last_action'] = ($row['last_action'] == '0000-00-00') ? 'Never' : $row['last_action']; $row['balance'] = sprintf("%01.2f", $row['balance']); $stringData = '"' . implode('","', $row) . '"'."\r\n"; fwrite($fh, $stringData); } fclose($fh); ?> If results are still slow you may need to optimize your SQL query, such as rather than doing SELECT * FROM ..etc... list only the rows you are going to be pulling from the database. -
Say $this->input->files('file') returned an array like so: array (path, filename, filesize) you could use list to get the filename list(,$filename,) = $this->input->files('file'); or create your own function which will return what ever item you want from the array.
-
Why are you wanting to create a new folder for each user? You're better of using just one file rather than having multiple user files. You can then use mod_rewrite so it looks as though you create a folder for every user. WIth mod_rewrote you can use have the following yoursite.com/user.php?user=username rewritten as yoursite.com/username
-
[SOLVED] every fifth entry create a break
wildteen88 replied to dennismonsewicz's topic in PHP Coding Help
Sorry, it is outputting 6 images because the counter starts at zero. If you only want 5 images per row change this line: if($i == 5) to if($i == 4) -
This looks like a file type issue. Make sure you are saving the file with ANSII encoding
-
Exporting large data into text file - PHP MySQL
wildteen88 replied to shergar1983's topic in PHP Coding Help
Well we'll need to see your code. Without seeing your code we cant suggest improvements. -
That code allows users to upload an image and the script will store the image in the database. Are you wanting to allow users to upload 10 images at once? Or just display 10 images from the database?