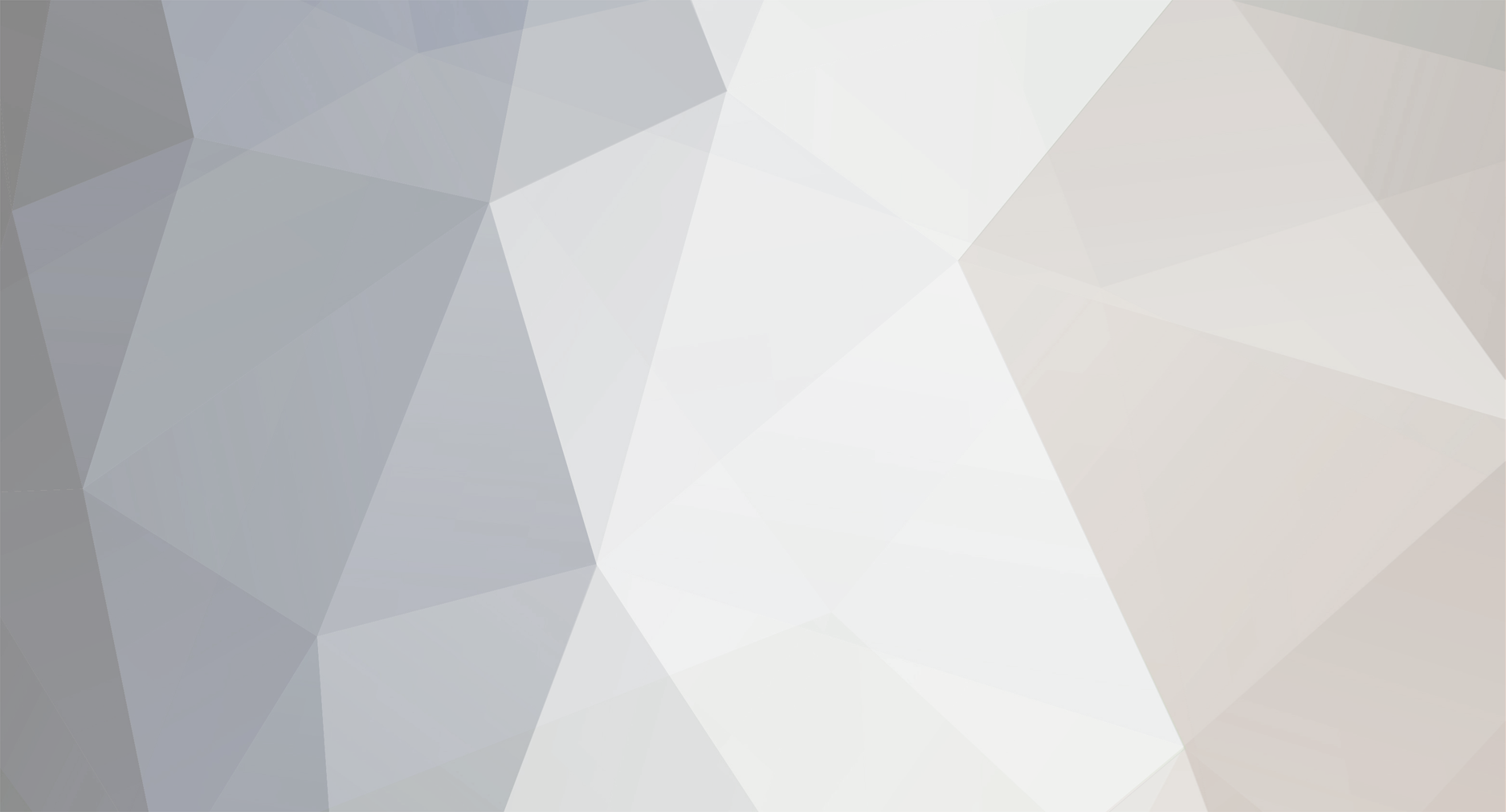
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
if its selecting 1 record from the database, then thiers no need to use a while loop. This is fine: [code=php:0]$com = mysql_query("SELECT * FROM `comments` WHERE `attraction` = '{$id}'") or die(mysql_error()); $comment = mysql_fetch_array($com); $username = mysql_query("SELECT `username` FROM `users` WHERE `id` = '" . $comment['userid'] . "'") or die(mysql_error()); $username = mysql_fetch_array($username); echo "<b>Posted by:</b> " . $username["username"]; echo "<br /><b>Comment:</b> " . $comment["comment"];[/code] Also the correct syntax is this: [code=php:0]while ($comment = mysql_fetch_array($com)) [/code] Otherwise it'll go into a never ending loop.
-
Nope. Should be something like this: [code=php:0]<?php //start the session session_start(); // connect to database first @mysql_connect('localhost', 'db_user', 'db_user_pass') or die("Unable to connect to MySQL at this time"); @mysql_select_db('db_name') or die("Unable to select database"); // make the input safe: $user = mysql_real_escape_string($_POST['username']); // you should encrypt users password. rather than using plain text passwords. $pass = mysql_real_escape_string($_POST['password']); // perform the query first $sql = "SELECT accesslevel FROM members WHERE username='".$user."' AND `password`=".$pass."';"; $result = @mysql_query($sql) or die("Unable to perform query"); // check that 1 row was returned, meaning a match is found if(mysql_num_rows($result) == 1) { // retrieve the access level $row = mysql_fetch_assoc($result); // set the session vars: $_SESSION['username'] = $_POST['nickname']; $_SESSION['accesslevel'] = $row['accessleve;']; }[/code]
-
Well the ./ tells PHP to look in the current working directory. I have no idea why it is failing. Does it work if you remove ./ from the include statement
-
multiple condidtions in an if statement
wildteen88 replied to CircularStopSign's topic in PHP Coding Help
Use: [code=php:0]if($var == $array[0] || $var == $array[1] || $var == $array[2]) { echo "Stuff is equal too 0,1, and 2"; }[/code] How ever what you'll probably want to use is in_array: [code=php:0]if(in_array($var, $array) { echo ; echo "$var us in the array!"; }[/code] -
Man thats shocking news. I used to watch his corcodile diaries program. Apparently he went diving and some sting ray penitrated his skin or something and caused a cardiac arrest, resulting in him dieing almost instantly.
-
How do I send data from 1 page to another?
wildteen88 replied to Pi_Mastuh's topic in PHP Coding Help
What the hell! This is some weird c**p Rather than including the database stuff. Try this: [code]<?php //session_start(); //$session = session_id(); $db_name = "db175253437"; $conn = mysql_connect("db491.perfora.net", "dbo175253437" ,"**********", true) or die("Couldn't connect"); mysql_select_db($db_name, $conn) or die("Couldn't select Database1."); $itemID = $_POST['itemID']; $sql = "SELECT * FROM myitemschibi WHERE itemID ='$itemID'"; $result = mysql_query($sql, $conn) or die("Unable to run query - <code>{$sql}</code><br />" . mysql_error($conn)); if(mysql_num_rows($result) == 1) { $row = mysql_fetch_assoc($result); echo '<pre>' . print_r($row, true) . '</pre>'; } else { echo "Item Id " . $itemID . " was not found"; } ?>[/code] Make sure you change where it says ********** with your actual mysql password. -
Whats in your eval should be in double quotes, and the $description variable should be surrounded in double quotes too. So your eval should be like this: eval("echo \"$description;\"");
-
phpglossar_0.8 - php/MySQL newbie requires assistance please
wildteen88 replied to macnewbie's topic in Third Party Scripts
Could you go to the admin page. When you get there right click any where on the page and click View Source. What does it show. Is the sourcre blank aswell? Also could you attach the script you have downloaded here please, I cannot download the the script form the said site, i get a 404 error for downloading the file. -
Personally I prefer to install AMP (Apache, PHP and MySQL) manaully rather than using a pre packaged product. As pre-packaged products come with alot of [i]bloat[/i]. There'll be features that'll be installed that I wont even use. So its just a waste of space to have them installed. If I want to use a feature I'll install it. For a newb that is starting I'd recommend you to installing Apache, PHP and MySQL manually. There are plenty of sites out their with guides to install them manually.If you're on Windows, which I presume you are then this is a good duide to follow [url=http://www.expertsrt.com/tutorials/Matt/install-apache.html]here[/url].
-
Your mysql extension hasn't been loaded up correctly. Which extension did you enable the normal mysql extension (php_mysql.dll) or the MySQL Improved extenson (php_mysql[b]i[/b].dll). Also run this in a script: [code=php:0]<?php phpinfo(); ?>[/code] Does it have a mysql section anywhere in that file?
-
Cannot modify header error / how to detect login + rights
wildteen88 replied to markspec87's topic in PHP Coding Help
Try this: [code]<?php $header = <<<HTML <html> <head> <title>Login</title> </head> <body> HTML; $server = "REMOVED"; // db host $db_user = "REMOVED"; // db username $db_pass = "REMOVED"; // db password $database = "REMOVED"; // db name // connect to the mysql server $link = mysql_connect($server, $db_user, $db_pass) or die ("Could not connect to mysql because ".mysql_error()); // select the database mysql_select_db($database) or die ("Could not select database because ".mysql_error()); $match = "select id from member where username = '".$_POST['username']."' and password = '".$_POST['password']."';"; $qry = mysql_query($match) or die ("Could not match data because ".mysql_error()); $num_rows = mysql_num_rows($qry); if ($num_rows <= 0) { echo $header; echo "Sorry, there is no username $username with the specified password.<br />"; echo "<a href=login.php>Try again</a>"; exit; } else { setcookie("loggedin", "TRUE", time()+(3600 * 24)); setcookie("mysite_username", "$username"); echo $header; echo "You are now logged in!<br />"; echo "Continue to the <a href=\"index.php\">members</a> section."; } echo "</body>"; echo "</html>"; ?>[/code] Notice I put the html into a variable and echo'd it later on. This is because you cannot use header or setcookie after any output is sent to the browser. So if theres any html/text that needs outputting it'll have to be done after you use header/setcookie or anyother header function. Ideally what you should do is do all the processing first and then output the result from the processing, rather output > process a bit > output something else > process something else etc -
When you include a php script into another php script. The file that is being included is merged with the parent file, so it becomes one file effectively. So what ever variables you use in the parent file, those variables can be used in the included file. Or am I not understanding what you are asking? [quote]When a html page calls a php script it can post variables to that script.[/quote] By that do you mean variables in the URL, eg mysite.com/page.php?var=something Then in page.php you use $_GET['var'] to get the var variable? If thats the case you can do that. However you'll have to use an absolute path (http://mysqite.com/path/to/file.php) to the file you are including and make sure that the allow_url_fopen direcive is set to on in the php.ini
-
Businessman to optimize an image in Fireworks is a breaze, same for photoshop. Open an image into fireworks, then click the [b]2-Up buttom[/b] to the right of the Preview button. What this will do is open up two versions of the same image into two views, the left is the original image (uncompressed/optimized) the right is the what the final image will look like when it has been optimized/compressed. You'll also see the file size of the orginal and and what the final image file size will be when you have optimized it. To optimixe the image make sure the Optimize pallete is open (press F6 or go to Window > Optimize). Now play around with the options in the otimize pallete. Note: You can reduce the file size of an image quite bit by lowering how many colors are in the image. However if you make it too low it'll affect the images appearence. So rather than having 256 colors or more reduce it down little by little. Once you notice there is a big difference between the left and the right you know you've reduced the colors too much, so you'll want to increase it, so you cant see much difference between the two images, the thing you should notice is the file sizes are different, the right should be lower than whats on the right. Changing the color isnt all of what optimisation is, but its one step towards optimising an image.
-
Its beacuse your are overwritting the result resource in your for loop. $r is the result resource from the SQL Query you have just run. You are assign $r to zeror in the for loop. change $r if your for loop to something else, like $_r that way it wont overwrite the result resource.
-
If you dont want to add aslashes to quotes in your echo statement. Use the [url=http://uk.php.net/manual/en/language.types.string.php#language.types.string.syntax.heredoc]HEREDOC syntax[/url]. You can put anythink into a HEREDOC statement without having to escape characters. If you use PHP variables in heredoc make sure you wrap the variable up in curly braces, eg: {$var_name} ALso if you are including a file that doesnt have a php extension and has PHP code in it. PHP will treat that file as a PHP file and will parse the code in that file.
-
I found out why, I went into AdminCP. I was allowed access with my password BTW. I went to look at the permissions and found that you have the Send PM setting set to Deny. If this happens any moderator in that group will have that permission removed. Prehaps set it to Disallow. This si what the contorl panel says about the Deny permission: [quote=AdminCP Permissions]Remember that if you deny a permission, any member - whether moderator or otherwise - that is in that group will be denied that as well. For this reason, you should use deny carefully, only when necessary. Disallow, on the other hand, denies unless otherwise granted.[/quote] The Deny permission doesnt affect Admins. I havn't changed anythink in the AdminCP. Just browsed the permissions.
-
Just noticed this from php.net, which I quoted below: [quote=http://uk2.php.net/manual/en/function.ftp-ssl-connect.php][b]Why this function may not exist: ftp_ssl_connect()[/b] is only available if OpenSSL support is enabled into your version of PHP. If it's undefined and you've compiled FTP support then this is why. For Windows you must compile your own PHP binaries to support this function.[/quote] By looks of things you need to recompile PHP to support this, even if you are on windows.
-
ober I got your PM, couldnt reply though. No buttons to reply/quote etc. If I click Outbox link I get the 'You are not allowed to send personal messages.' Or if I click the 'New Message' link I get the same result. EDIT: I looked at the permissions I have by clicking the [url=http://www.phpfreaks.com/forums/index.php?action=profile;u=17232;sa=showPermissions]Show Permissions[/url] link and I found this: [quote][s]pm_send[/s] Send personal messages [color=red]Denied by:[/color] Super Guru[/quote] Looks like I'm part of the Super Guru member group, but am masked as a moderator. Also its not just me thats effected, Its all of the moderators! However some moderators are asisgned to different member groups.
-
[quote author=steelmanronald06 link=topic=106580.msg427264#msg427264 date=1157310912] I do believe that the problem is now fixed. Can anyone verify that they cannot send/reply to PM's? NOTE: Admins and Moderators are exempt from this; they can still send PM's [/quote] Steelman I cannot send PMs :( I dont think anyone can.
-
Parse Error, unexpected $ - very fustrating. Please help.
wildteen88 replied to digi duck's topic in PHP Coding Help
I think you do as when I was formatting the code I was a little confused. Go througb the code and check that everythink is whetre it should be. One Thing I was a little confused with was when I found this - [code=php:0]for(;;)[/code] - Where's the variables/conditions. You cant have a blank for loop. -
Do you have display_errors turned in the php.ini and is error_reporting set ot E_ALL? How do you know it doesnt work. What does it do. Could you provide more info. Is the following code, whats exactly in test.php [code=php:0]include ("./sqlfunctions.php") // have code that sets up a variable called $statment. $statment = "select * from debtors" $res = sql($statment)[/code] If it is it should be this: [code=php:0]include ("./sqlfunctions.php"); // missing a ; // have code that sets up a variable called $statment. $statment = "select * from debtors"; // missing a ; $res = sql($statment); // misssing a ;[/code] You have forgotten to add the semi-colon on the end of each line. You must make sure every line has a semi-colon at the end of it, unless you are defining a function, using an if statement/while loop etc.
-
How do I send data from 1 page to another?
wildteen88 replied to Pi_Mastuh's topic in PHP Coding Help
Try this: [code]<?php session_start(); $session = session_id(); $itemID = $_POST['itemID']; include ("secure/config3.php"); $sql = "SELECT * FROM myitemschibi WHERE itemID ='$itemID'"; $result = mysql_query($sql, $connection) or die("Unable to run query<br />" . mysql_errno($connection) . ': ' . mysql_error($connection)); if(mysql_num_rows($result) == 1) { $row = mysql_fetch_assoc($result); echo '<pre>' . print_r($row, true) . '</pre>'; } else { echo "Item ID '" . $itemID . "' was not found"; } $image = str_replace(" ", "", $itemName); $spacedname = str_replace(" ", "%20", $itemName); ?>[/code] -
You mean these two: [code]define('ROOT_DIR', realpath(dirname(__FILE__))); define('MP3FLASHLIST_DIR',ROOT_DIR."/uploads/playlist");[/code] They should fine as they are. No need to tinker them. the only bit you might want to change is where it says '[i]"/uploads/playlist"[/i]'. WHat ever you are uploading will be uploaded to '[your sites root dir)/uploads/playlist' (http://yoursite.com/uploads/playlist)
-
Your intentions are completly wrong. PHP runs on the server and generates the output back to the browser. You canno do this: [code]input name="imageField" type="image" ... onClick="<?php $Username = $_POST['txtLogInUserName']; ?>" >[/code] As befoe the HTML is even shown PHP would have created the username var and assign it it the value of whats in $_POST['txtLogInUserName'] variable. What you'll want to do is something like this: [code=php:0]<?php //check that the form has been submitted: if(isset($_POST['imageField_x'])) { // form has been submitted! // now we set up the username variable: $Username = $_POST['txtLogInUserName'] // Welcome the user: echo 'Welcome <i>' . $Username . '</i>, How are you today?<br /><br />'; } else { // Foprm hasnt been submitted so we'll tell them to: echo "Please fill out the form below, click submit when done<br /><br />"; } ?> <div id="lyrLogin" style="position:absolute; left:578px; top:60px; width:166px; height:45px; z-index:20; visibility: visible;"> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post" name="frmLogIn" id="frmLogIn"> <p align="left"> <input name="txtLogInUserName" type="text" id="txtLogInUserName" /> </p> <p> <input name="imageField" type="image" src="login_button_up.gif" width="105" height="36" border="0" value="Submit" /> </p> </form> </div>[/code] When you first go to the page, [i]Please fill out the form below, click submit when done[/i] will be displayed above the form. But when you fill in the form and press sumit, you'll get a welcome message with the username you put in the txtLogInUserName text field.