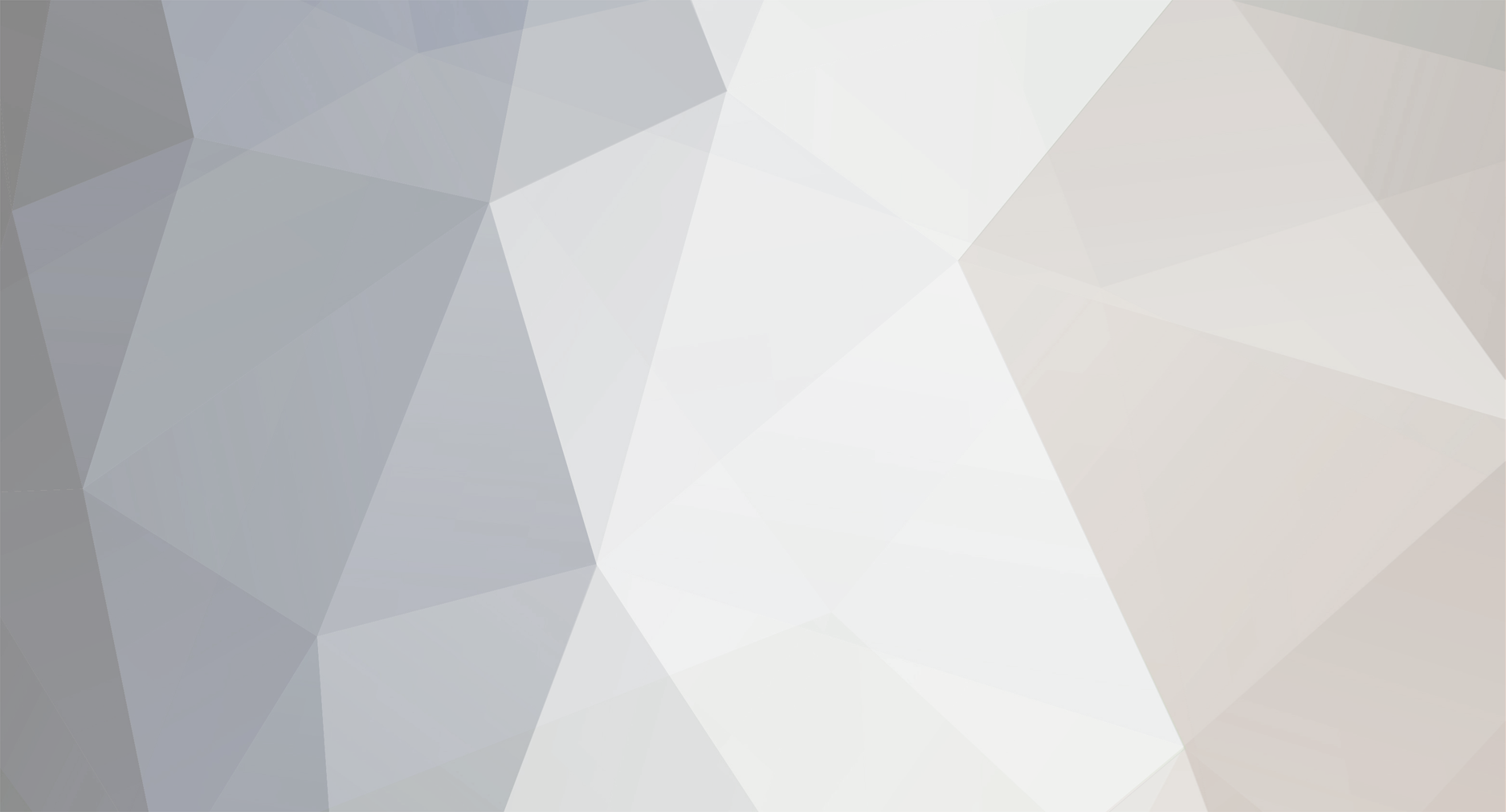
Metoriium
-
Posts
13 -
Joined
-
Last visited
Posts posted by Metoriium
-
-
Sorry for many posts, trying to make my website
When I press the register button on my website it will just act as if the page is refreshing and not send any information to mysql
I believe I have connected everything up correctly, can anyone tell my what I have done wrong please?
If you want to check out the website to see what is going on check out www.jokestary.comli.com
<?php //This function will display the registration form function register_form(){ $date = date('D, M, Y'); echo "<form action='?act=register' method='post'>" ."Username: <input type='text' name='username' size='30'><br>" ."Password: <input type='password' name='password' size='30'><br>" ."Confirm your password: <input type='password' name='password_conf' size='30'><br>" ."Email: <input type='text' name='email' size='30'><br>" ."<input type='hidden' name='date' value='$date'>" ."<input type='submit' value='Register'>" ."</form>"; } //This function will register users data function register(){ //Connecting to database include('connect.php'); if(!$connect){ die(mysql_error()); } //Selecting database $select_db = mysql_select_db("database", $connect); if(!$select_db){ die(mysql_error()); } //Collecting info $username = $_REQUEST['username']; $password = $_REQUEST['password']; $pass_conf = $_REQUEST['password_conf']; $email = $_REQUEST['email']; $date = $_REQUEST['date']; //Here we will check do we have all inputs filled if(empty($username)){ die("Please enter your username!<br>"); } if(empty($password)){ die("Please enter your password!<br>"); } if(empty($pass_conf)){ die("Please confirm your password!<br>"); } if(empty($email)){ die("Please enter your email!"); } //Let's check if this username is already in use $user_check = mysql_query("SELECT username FROM users WHERE username='$username'"); $do_user_check = mysql_num_rows($user_check); //Now if email is already in use $email_check = mysql_query("SELECT email FROM users WHERE email='$email'"); $do_email_check = mysql_num_rows($email_check); //Now display errors if($do_user_check > 0){ die("Username is already in use!<br>"); } if($do_email_check > 0){ die("Email is already in use!"); } //Now let's check does passwords match if($password != $pass_conf){ die("Passwords don't match!"); } //If everything is okay let's register this user $insert = mysql_query("INSERT INTO users (username, password, email) VALUES ('$username', '$password', '$email')"); if(!$insert){ die("There's little problem: ".mysql_error()); } echo $username.", you are now registered. Thank you!<br><a href=login.php>Login</a> | <a href=index.php>Index</a>"; } switch($act){ default; register_form(); break; case "register"; register(); break; } ?>
Here is the connect.php code
<?php $hostname="mysql6.000webhost.com"; //local server name default localhost $username="a5347792_users"; //mysql username default is root. $password=""; //blank if no password is set for mysql. $database="a5347792_users"; //database name which you created $con=mysql_connect($hostname,$username,$password); if(! $con) { die('Connection Failed'.mysql_error()); } mysql_select_db($database,$con); ?>
-
I have changed this information but still doesnt work
Also how can i change this to mysqli?
<?php session_start(); //include connect.php page for database connection include('connect.php'); //if submit is not blanked i.e. it is clicked. if($_SERVER['REQUEST_METHOD'] == 'POST') { if($_POST['name']=='' || $_POST['email']=='' || $_POST['password']==''|| $_POST['repassword']=='') { echo "please fill the empty field."; } else { $sql="insert into student(name,email,password,repassword) values('".$_POST['name']."', '".$_POST['email']."', '".$_POST['password']."', '".$_POST['repassword']."')"; $res=mysql_query($sql); if($res) { echo "Record successfully inserted"; } else { echo "There is some problem in inserting record"; } } } ?>
-
Hey again chris, And no error message, just takes me to registration.php but doesnt add any information to mysql
-
I want people to be able to post images on to my page but i want them to be able to add a caption when they post their image
This is the format I want the image and caption to be posted like
<div class="spacer"> </div> <div class="jokestary-img" title="Siri will screw up your relationship"> <img src="uploads/Siri will screw up your relationship.jpg" /> <div class="caption"><div>Siri will screw up your relationship.</div></div></div>
The code for the upload form also wont work
The page says "Upload complete!" before i even choose a file and doesnt send to my database
?php // properties of the uploaded file $name = $_FILES ["myfile"] ["name"]; $type = $_FILES ["myfile"] ["type"]; $size = $_FILES ["myfile"] ["size"]; $temp = $_FILES ["myfile"] ["tmp_name"]; $error= $_FILES ["myfile"] ["error"]; if ($error > 0) die ("Error uploading file! code $error."); else { if ($type== "video/av/png || $size 1000000") //conditions for file { die("That format is not allowed or file size too big"); } else move_uploaded_file($temp,"uploads/".$name); echo "Upload complete!"; } ?>
-
Each time i submit this form it wont send to mysql, can anyone help?
<?php $hostname="mysql6.000webhost.com"; //local server name default localhost $username="a5347792_meto"; //mysql username default is root. $password=""; //blank if no password is set for mysql. $database="a5347792_login"; //database name which you created $con=mysql_connect($hostname,$username,$password); if(! $con) { die('Connection Failed'.mysql_error()); } mysql_select_db($database,$con); //include connect.php page for database connection include('connect.php'); //if submit is not blanked i.e. it is clicked. if($_SERVER['POST_METHOD'] == 'POST') { if($_POST['name']=='' || $_POST['email']=='' || $_POST['password']==''|| $_POST['repassword']=='') { echo "please fill the empty field."; } else { $sql="insert into student(name,email,password,repassword) values('".$_REQUEST['name']."', '".$_REQUEST['email']."', '".$_REQUEST['password']."', '".$_REQUEST['repassword']."')"; $res=mysql_query($sql); if($res) { echo "Record successfully inserted"; } else { echo "There is some problem in inserting record"; } } } ?>
-
Yes this is fixed, i am going to link you to the webpage that i am working on via message. Thank you so much chrisrulez001
-
one more question aha.
What normally goes into connect.php
//include connect.php page for database connectioninclude('connect.php'); -
I have fixed it, Thank you very much
-
I am using 000webhost at the moment (this is temporary) and i dont think it supports mysqli
-
Thank you very much
chrisrulez001This works now although i am now getting this message
Warning: mysql_connect() [function.mysql-connect]: Access denied for user 'root'@'localhost' (using password: NO) in /home/a5347792/public_html/register.php on line 6
Connection FailedAccess denied for user 'root'@'localhost' (using password: NO)
Sorry i am new to this stuff
-
Just tried that but still says error message
-
Not sure what is wrong with line 17
<?php $hostname="localhost"; //local server name default localhost $username="root"; //mysql username default is root. $password=""; //blank if no password is set for mysql. $database="login"; //database name which you created $con=mysql_connect($hostname,$username,$password); if(! $con) { die('Connection Failed'.mysql_error()); } mysql_select_db($database,$con); //include connect.php page for database connection Include('connect.php') //if submit is not blanked i.e. it is clicked. If(isset($_REQUEST['submit'])!='') { If($_REQUEST['name']=='' || $_REQUEST['email']=='' || $_REQUEST['password']==''|| $_REQUEST['repassword']=='') { Echo "please fill the empty field."; } Else { $sql="insert into student(name,email,password,repassword) values('".$_REQUEST['name']."', '".$_REQUEST['email']."', '".$_REQUEST['password']."', '".$_REQUEST['repassword']."')"; $res=mysql_query($sql); If($res) { Echo "Record successfully inserted"; } Else { Echo "There is some problem in inserting record"; } } } ?>
How do I allow users to upload images with caption
in PHP Coding Help
Posted
Can anyone solve this?