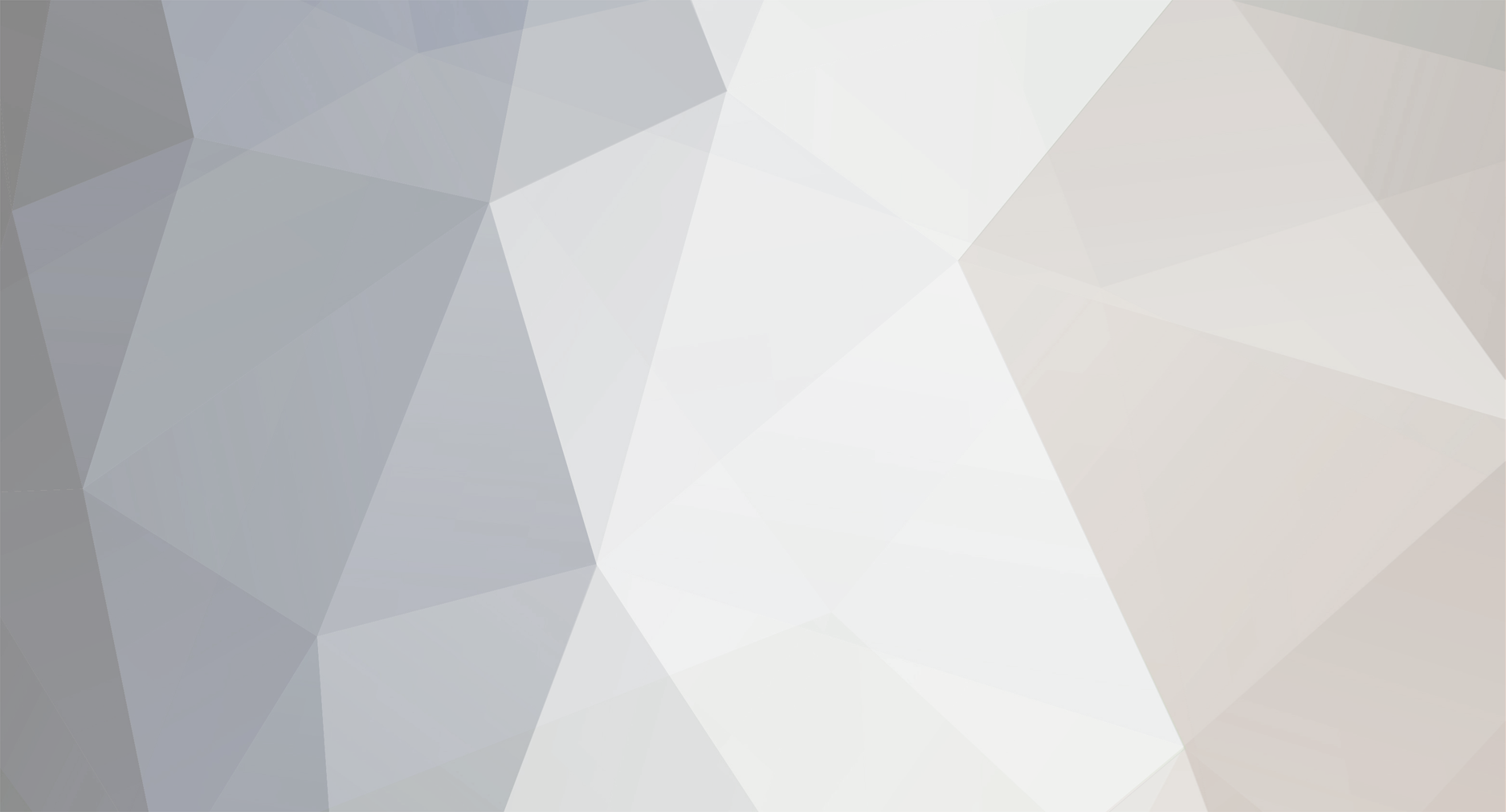
php_bad_boy
-
Posts
4 -
Joined
-
Last visited
php_bad_boy's Achievements

Newbie (1/5)
0
Reputation
php_bad_boy has no recent activity to show
0
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.