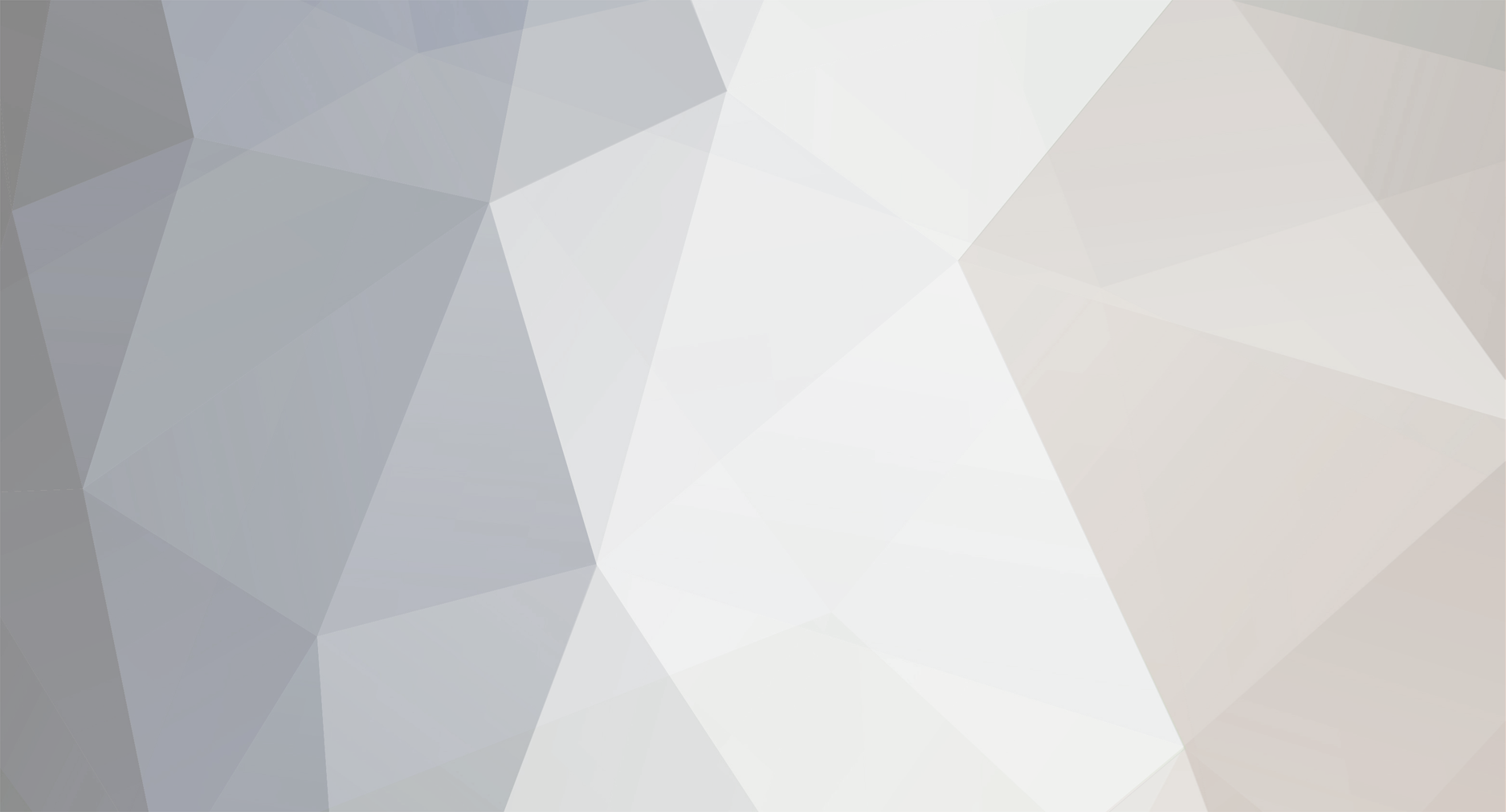
Codin2012
-
Posts
15 -
Joined
-
Last visited
Posts posted by Codin2012
-
-
I have a form to submit a email address and password. I get an undefined index error and if I try to use isset to fix the undefined index error I get the following error Cannot use isset() on the result of an expression (you can use "null !== expression" instead) in and I have tried to use the suggestions to solve the problem but than another error pops up.
Here is my php code . All help greatly appreciated.
if (isset($_POST['submit']=="Sign Up")) {if (!$_POST['email']) $error.="<br />Please enter your email";else if (!filter_var($_POST['email'], FILTER_VALIDATE_EMAIL)) $error.="<br />Please enter a valid email";if (!$_POST['password']) $error.="<br />Please enter your password";and my html<form class="marginTop" method="post"><div class="form-group"><label for="email">Email Address</label><input type="email" name="email" class="form-control" placeholder="Your Email" value="<?php echo addslashes($_POST['email']); ?>" /></div><div class="form-group"><label for="password">Password</label><input type="password" name="password" class="form-control" placeholder="Password" value="<?php echo addslashes($_POST['password']); ?>" /></div><input type="submit" name="submit" value="Sign Up" class="btn btn-success btn-lg marginTop"/></form> -
You apparently have produced this variable (a query result perhaps?) before trying to create the new object. But like so many people who are new to PHP (and/or programming) you fail to check the result of that query(?) to see if you have something in that array. Do that and you won't run into this situation. Or you could add some more code to the constructor to allow for an empty argument if that actually makes sense to do, ie, creating the object when you don't have the input for it.
As you pointed out I did not test to see, whether $request actually has a 'controller' entry. so this is what I did to solve the problem
public function __construct($request){ $this->request = $request; if (isset($request['controller'])) { if ($this->request['controller'] == ""){ $this->controller = 'home'; } else { $this->controller = $this->request['controller']; } if($this->request['action'] == ""){ $this->action = 'index'; } else { $this->action = $this->request['action']; } } }
-
Actually I would do that print of the input argument before calling the constructor, ie, before creating the object. Obviously the item you are passing is either not an array or not an array containing what you think it does.
Could you possibly give me an example of what you are suggesting? Thank you
-
Have you tried outputting $request to see what it contains?
public function __construct($request){ print '<pre>' . print_r($request, true) . '</pre>'; //...
Thank you for the suggestion I tried what you suggested and it was an empty array.
-
I am trying to build an OOP PHP following MVC formatt. I do not understand why these lines of code would give an undefined index error.
public function __construct($request){ $this->request = $request; if($this->request['controller'] == ""){ $this->controller = 'home'; } else { $this->controller = $this->request['controller']; } if($this->request['action'] == ""){ $this->action = 'index'; } else { $this->action = $this->request['action']; } }
It is my two if statements causing the errors. All help greatly appreciated. -
What is the best way using OOP PHP to find the total quantity of a shopping cart. The customer can have more than one item per page to add to the cart. So whenever the quantity of the cart changes I want the total to change. So I have a method in my cart class that will calculate the total quantity. Here is the method from my cart class.
public function get_total_qty($total_qty = '') { $total_qty = 0; if (isset($_SESSION['cart'])) { foreach($_SESSION['cart'] as $qty) { $total_qty = $total_qty + $qty; } } return $total_qty; echo $total_qty; die(); }
And here is the code on my index page
$total_qty = new Cart(); $total_qty->get_total_qty($cart_itm["qty"]);
cart_itm["qty] is the variable used to receive the quantity when an item is added to the cart.
-
I am trying to get the total quantity of products ordered and added to my shopping cart. I am trying to do this in OOP. I have a class called cart and I have a method called get_total_qty. So every time the cart gets updated and the quantity changes I need my total quantity to change also. Here is my class
class Cart { public function __construct() { }
-
Makes sense: there isn't actually any code in there to execute the query (which is supposedly contained in the $mysql variable), nor to display the results. Does the tutorial add that code later?
And what does it say about the query? Because what you have in $mysql is incorrect.
Thank you for responding but to answer your question it does not add the code later. How is the mysql query incorrect.
-
I have been going through a couple of PHP OOP pagination tutorials I followed several and decided to use this one but it just does not work. I am sure I am connected to the database but it will not pull the information from the database.
I am just going to include the index and pagination class.
Here is the index.php page
<?php // Initialize both configuration and pagination file one by one include_once("config.php"); include_once("pagination.php"); //get the page number using request method $myoffset = $_REQUEST["page"]; // check whether the requested page variable is empty. If empty start with one $page = !empty($myoffset) ? (int)$myoffset:1; //Assign per page results $per_page = 5; //Assign total count of records am manually entered value here. //If you receive data from database see the next code $total_count = 25; //Query For getting total count $sql = "SELECT COUNT(*) FROM City"; $totalcount = mysqli_query($sql); echo "total count is $totalcount"; //Query For Fetching Results as per Pagination count from Database $mysql = "SELECT * FROM City ORDER by id DESC LIMIT {$per_page} OFFSET {$myoffset}"; //Assign all value to populate pagination with oops $pagination = new Pagination($page,$per_page,$total_count); // Store all pagination values in this variable called $res $res = ""; //Checking total page, if total page not more than one than hide pagination if($pagination->total_pages() > 1) { //check if it has previous page if($pagination->has_previous_page()) { $res .= '<a href="index.php?page='.$pagination->previous_page().'"> « Previous </a>'; } //populating looping values to show navigation for($i=$page;$i<=$pagination->total_pages();$i++) { $res .= '<a href="index.php?page='.$i.'" class="active"> '.$i.'</a>'; } //check if it has next page if($pagination->has_next_page()) { $res .= '<a href="index.php?page='.$pagination->next_page().'">Next »</a>'; } } ?> <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Pagination</title> <!-- Adding style for pagination --> <style> .paginationblock{ width:350px; height:auto; margin:0 auto; margin-top:300px } .paginationblock a{ width:auto; height:auto; float:left; padding:10px 15px 10px 15px; text-decoration:none; font-family: Helvetica, Arial, 'lucida grande',tahoma,verdana,arial,sans-serif; font-size:16px; background-color:#222222; color:#ffffff; margin-right:3px; } .paginationblock a:hover{ background-color:#09F; } </style> </head> <body> <!-- Initialize pagination script --> <div class="paginationblock"> <?php echo $res; ?> </div> </body> </html>
now my pagination.php page
<?php class Pagination { public $current_page; public $per_page; public $total_count; public function __construct($page=1,$per_page=0,$total_count=0) { $this->current_page = (int)$page; $this->per_page = (int)$per_page; $this->total_count = (int)$total_count; } public function offset(){ return ($this->current_page - 1) * $this->per_page ; } public function total_pages(){ return ceil($this->total_count/$this->per_page); } public function previous_page(){ return $this->current_page-1; } public function next_page(){ return $this->current_page+1; } public function has_previous_page(){ return $this->previous_page() >= 1 ? true:false; } public function has_next_page(){ return $this->next_page() <= $this->total_pages() ? true:false; } } // Declaring Object here $pagination = new Pagination(); ?>
All help greatly appreciated.
-
Trying to grow in PHP so I thought this would be a great place to learn and share.
Can not use isset to fix undefined index
in PHP Coding Help
Posted
Thank for your answer it solved my problem.