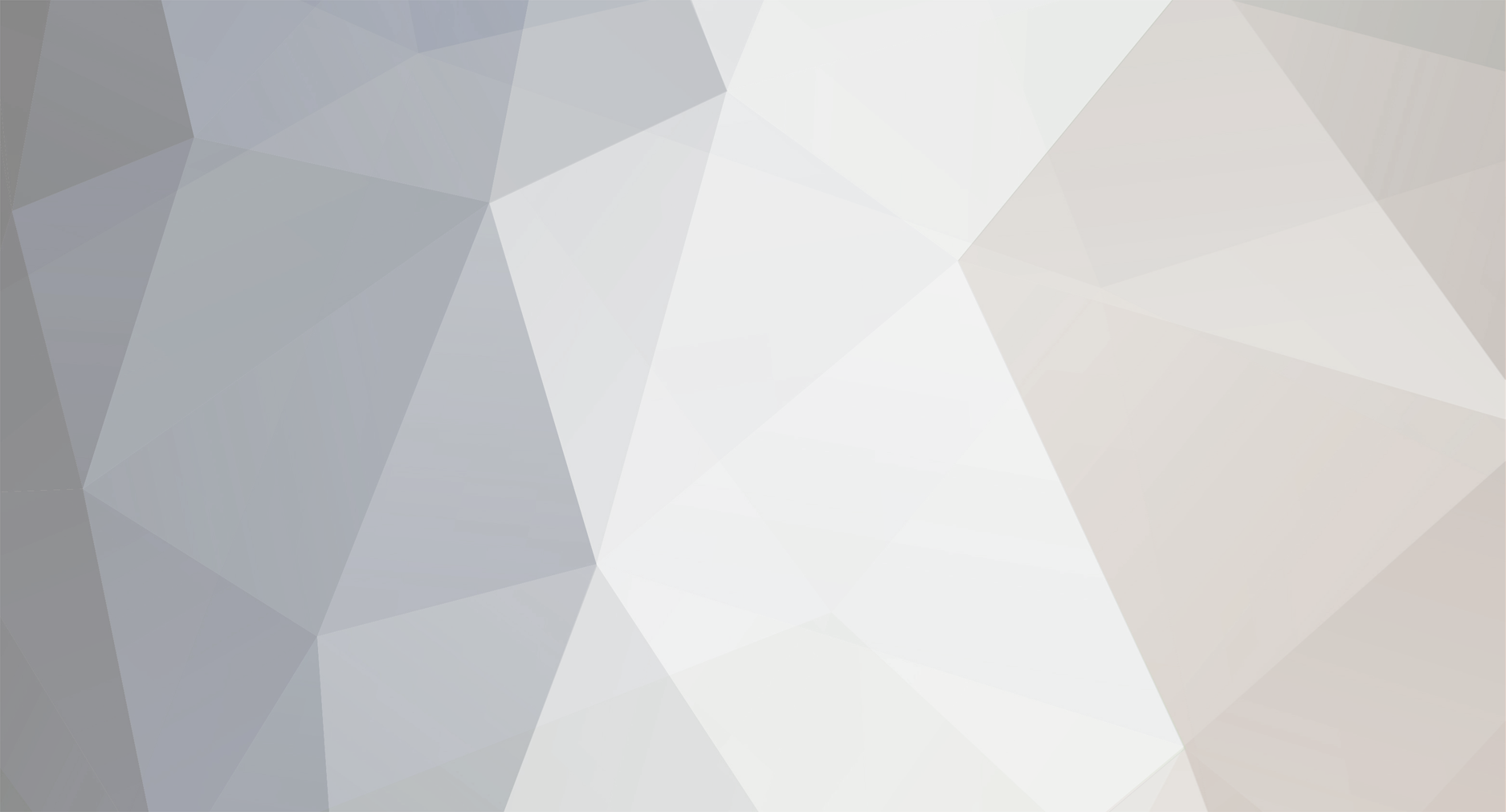
Markuzi94
-
Posts
4 -
Joined
-
Last visited
Posts posted by Markuzi94
-
-
Post the code that you are trying.
The code is on the first post. It's working except the sorting.
-
Use the sort() function.
Well the problem is that I don't know how to use it correctly on this code. I have tried sort(), usort(). I don't think we have studied that in school... I'm not sure though. At least our teacher does not use it in any examples even some of them includes sorting.
-
Hello. First of all, English is not my main language so I'm sorry if I make some mistakes in my text...
Anyway, my problem is that I really have no clue how to do this task I was given: I need to create a form where I can write names and then save them and do this for unlimited times. After I have added names, I need to sort them alphabetically from A-Z. I have done it like this so far, just the sorting is missing.
This job has two files, index.php and persons.php.
index.php:<?php include "persons.php"; ?> <!DOCTYPE html> <!-- --> <html> <head> <meta charset="UTF-8"> <title><Task</title> </head> <body> <form action=""> First name: <input type="text" name="first_name"> Last name: <input type="text" name="last_name"><br> <input type="submit" name="do" value="Add"><br> Alphabetical order by Last name:<input type="radio" name="alphabetical" value="ln_first" checked><br> Alphabetical order by First name:<input type="radio" name="alphabetical" value="fn_first"><br> <input type="submit" name="do" value="Sort"> </form> <?php if (isset($_GET["first_name"]) && isset($_GET["last_name"]) && $_GET["first_name"] != "" && $_GET["last_name"] != "" || $_GET["do"] == "Sort") { $persons = new persons($_GET["first_name"], $_GET["last_name"]); $str = ""; switch ($_GET["do"]) { case "Add": $persons->Add($_GET["first_name"], $_GET["last_name"]); break; case "Sort": $str = $persons->Sort(); break; default: $str = "Something went wrong."; } print($str); } else print("Give a real name."); ?> </body> </html>
persons.php:
<?php session_start(); /** */ class persons { private $persons; public function __construct() { if (isset ($_SESSION["persons"])) $this->persons = $_SESSION["persons"]; else $this->persons = array(); } public function __destruct() { $_SESSION["persons"] = $this->persons; } /** * * @param type $first_name * @param type $last_name * @return string */ public function Add($first_name, $last_name) { $this->persons[$first_name] = $last_name; return "Added."; } /** * * @return string */ public function Sort() { $str = "<table>\n"; foreach ($this->persons as $first_name => $last_name) { $str .= "<tr><td>$first_name</td><td>$last_name</td></tr>\n"; } $str .= "</table>\n"; return $str; } }
So I just need to add the sorting somewhere in the code, but I just don't know where. This task was given in my school and it is the first course of php coding. I am a newbie in this thing...
Adding unlimited amount of names into an array and then printing them in alphabetical order
in PHP Coding Help
Posted
I got the task to work like it should now. I just changed that "sort($this->persons);" to "natsort($this->persons);" because with "sort" it printed the names like:
(Added names Name A, Name B and Name C)
[0] A
[1] B
[2] C
with natsort, it prints them as:
Name A
Name B
Name C
so it now works perfectly. Thank you.