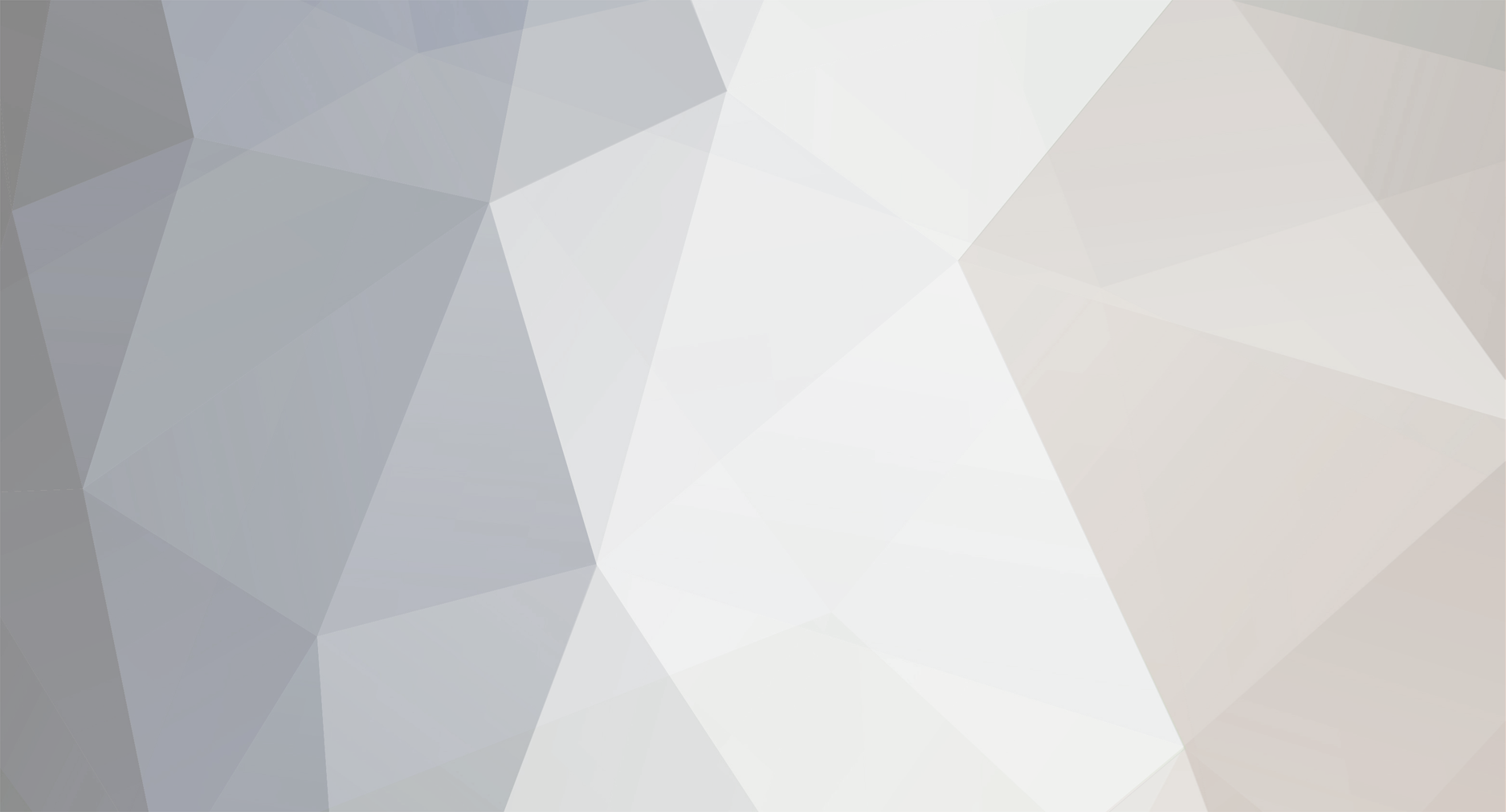
xyph
-
Posts
3,711 -
Joined
-
Last visited
-
Days Won
1
Posts posted by xyph
-
-
Alternately,
<?php $values = array( // Number => Weighted chance 1 => 1, 2 => 1, 3 => 5, // 5x more likely than 1,2 4 => 3 // 3x more likely than 1,2 ); // The total of all the chances $total = array_sum($values); // Will track the sum of all tested chances $i = 0; // The number we're looking to find $find = mt_rand(1,$total); // Loop through value=>chance array foreach( $values as $val => $chance ) { // Add current chance to the running total $i += $chance; // Check if the running total equals or exceeds the number we're trying to find if( $find <= $i ) // If so, break out of the loop break; } // The last assigned value before breaking will contain the number echo $val; ?>
The process is more complex, but the input is more simplified.
-
why not just...copy()? Does the server disable remote url access?
If cURL is gone, I doubt allow_url_fopen is true
-
Force everyone to use OSX?
Expecting a browser to render fonts like a design application is just silly
I believe most just piggy-back on the OS' rendering engine.
Use images, static or dynamic, if you need fonts to render in a certain way.
-
BTW - you should tie your mind up tighter next time
-
Well, there's 256 valid ASCII characters, so he's nowhere near half-way.
-
session_register is long depricated. On top of that, you're using in incorrectly. Please, check out the manual on how to assign and use session variables.
-
listing them individually is the easiest way, but at this point you're allowing most of the ascii range. Might as well make a blacklist, or specify your ranged by hand using an ascii chart.
If you use an array as a string, it becomes the word "Array." Implode turns an array into a string so you can actually see the values.
I think it's preg_quote that's messing up the ranges (\-).
IMO, using a blacklist is a bad idea unless you're limiting all input to the ASCII range, and even then it would be pretty big.
A solution could be to keep ranges in a separate array, and not preg_quote those.
-
Is the PHP script being used to authenticate running on the same server as their application?
-
Can you please give us a small snippet of code to recreate your issue. You are being vague and using some confusing language to try and explain your problem.
-
INSERT INTO tablename (counter, unique) VALUES (1, $unique_value)
ON DUPLICATE KEY UPDATE counter = counter + 1;
http://dev.mysql.com/doc/refman/5.0/en/insert-on-duplicate.html
You should read the manual entry. There are some specific by-products of using this method.
-
Probably, because that function doesn't exist?
The manual is a necessary reference, you have to learn to check there first
http://www.php.net/m...mysqli-stmt.php
As to your problem,
http://www.php.net/m...-stmt.fetch.php
Ninja'd.
-
You're missing the money_format call in your log.
-
Seems legit.
-
Why not use the example that works then.
The language barrier here seems too large to overcome. I'm really lost.
-
Variables are parsed within a double-quoted string.
http://www.php.net/manual/en/language.types.string.php#language.types.string.syntax.double
As you can see in the manual, in order to get a literal $, you must escape it with a leading backslash.
-
Assuming the algorithm is well built - the shorter the digest, the greater chance of collision.
The data returned in a digest is always binary, just generally in hex form. In this case, a 32-bit integer is returned. They have quite a bit written in the big, red warning box in the manual entry - including how to convert it to hex.
http://php.net/manua...ction.crc32.php
Keep in mind, a 10-digit integer can be 'shorter' than a 5 character string, in a storage sense. Hex is generally an inefficient way to store binary data (assuming it's a string)
-
MC is missing a closing single quote.
-
What have you tried so far? Have you managed to get a list of the files in a given directory.
-
I think he wants the token to stay consistent over multiple requests.
I don't think using the session ID is a good idea, even if obfuscated or hashed.
Your best bet is to generate a string based on random data, then store this in a cookie with a FALSE expiration, so it expires with the user.
We'd need more details to give you a better solution
-
Isn't time stored in the CMOS/BIOS? Wouldn't changing the time potentially change the time for other VPSs on the box?
You can configure Linux to not use the BIOS clock. I prefer to always get my time through ntp.
As do I, but then I sync my BIOS clock with NTP.
Perhaps I'm a little crazy.
-
-
Isn't time stored in the CMOS/BIOS? Wouldn't changing the time potentially change the time for other VPSs on the box?
-
Where are you getting
$reference_points.[2]=9
There's no 9 in
5.2.80.0/21 5.11.128.0/17
You probably want this
<?php $string = '5.2.80.0/21 5.11.128.0/17'; $pattern = '%(\d+)\.(\d+)\.(\d+)\.(\d+)/(\d+)%'; preg_match_all($pattern, $string, $matches, PREG_SET_ORDER); var_dump($matches); ?>
Output
array (size=2) 0 => array (size=6) 0 => string '5.2.80.0/21' (length=11) 1 => string '5' (length=1) 2 => string '2' (length=1) 3 => string '80' (length=2) 4 => string '0' (length=1) 5 => string '21' (length=2) 1 => array (size=6) 0 => string '5.11.128.0/17' (length=13) 1 => string '5' (length=1) 2 => string '11' (length=2) 3 => string '128' (length=3) 4 => string '0' (length=1) 5 => string '17' (length=2)
-
Read the article in my signature, if you have any questions about it, ask here.
It pretty much covers everything you need to know, and more.
Interesting Behavior In Php's Handling Of $This
in PHP Coding Help
Posted
Well, wacky code will produce wacky results. PHP being so forgiving, it doesn't just explode - There are definitely positives and negatives of that overall behaviour.