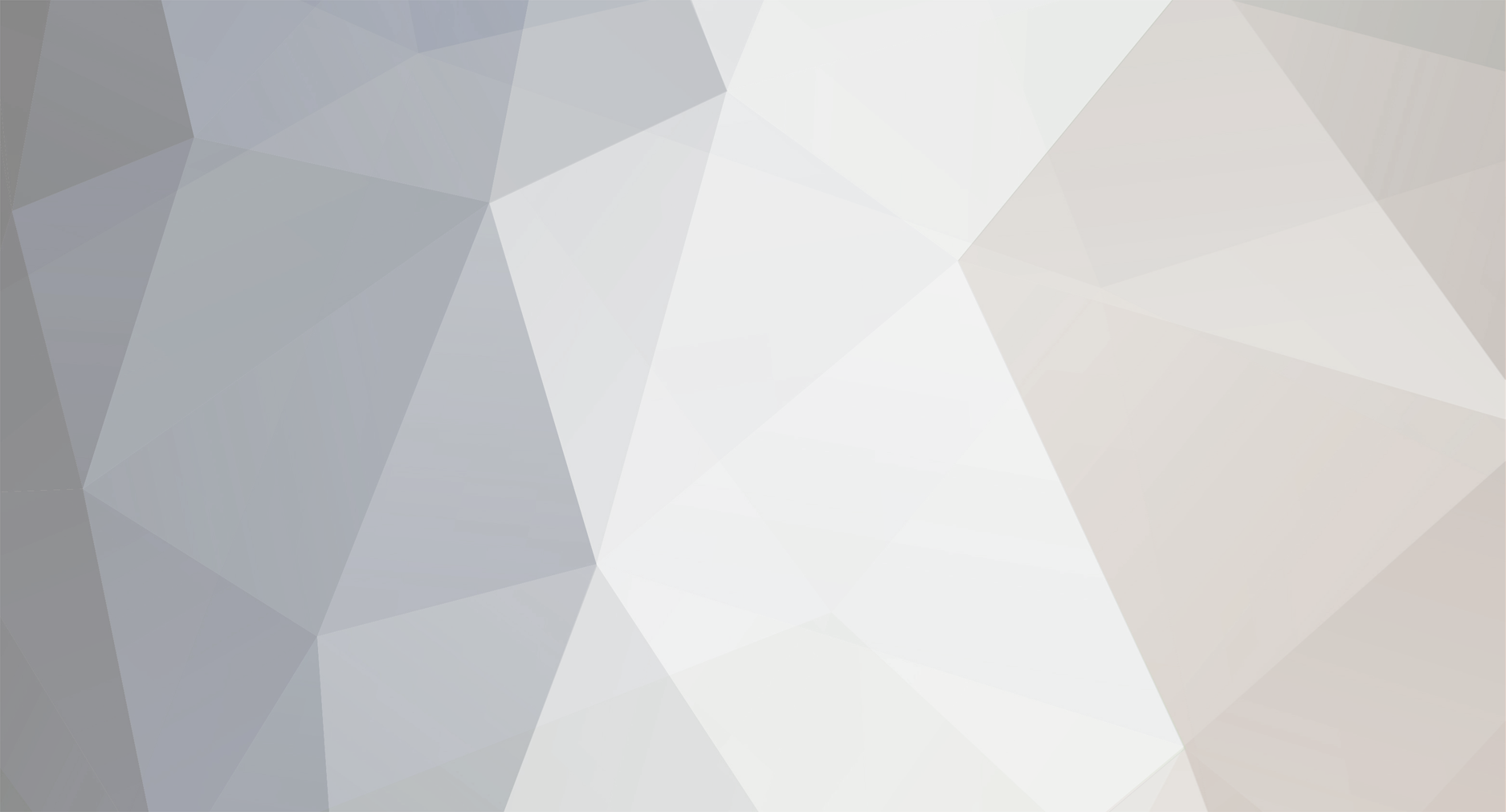
JNew
-
Posts
4 -
Joined
-
Last visited
Posts posted by JNew
-
-
I have a recipe site and I want the user to have the option to edit the recipe they're viewing. The code for the page where they can see the recipe looks like this:
<!-- START THE TEMPLATE --> <?php $id = $_GET['id']; $sql = "SELECT id, category, bilde, title, duration, servings, ingredients, directions FROM oppskrift_table WHERE id=$id"; $result = mysqli_query($con, $sql); if($result) { while($row = mysqli_fetch_assoc($result)){ ?> <div class="container" style="margin-top:3%;"> <div class="row product"> <div class="col-md-5 col-md-offset-0"><img class="img-responsive imgSpace" src="bilder/rBilder/<?=$row['bilde']?>" width="400" alt=""></div> <div class="col-md-7"> <h1> <?=$row['title']?> </h1> <!-- EDIT BUTTON --> <a href="edit.php?id=<?=$row['id']?>" class="btn btn-sample btn-primary btn-sm pull-right">Edit <span class="glyphicon glyphicon-pencil"></span></a> <p style="line-height:200%;"><img src="bilder/misc/time.png" width="25" height="25" style="margin-right:2%;"/> <?=$row['duration']?> </p> <p style="line-height:200%;"><img src="bilder/misc/servings.png" width="25" height="25" style="margin-right:2%;"/> <?=$row['servings']?> </p> </div> </div> <div class="col-lg-6"> <div class="page-header"> <h3>Ingredients:</h3> </div> <p> <?=$row['ingredients']?> </p> </div> <div class="col-lg-6"> <div class="page-header"> <h3>Directions:</h3> </div> <p style="line-height:185%;"> <?=$row['directions']?> </p> </div> </div> <br> <?php } } ?>
And the code to edit the recipe looks like this:
<?php include 'connect.php'; if(isset($_POST['btn_submit'])){ $sql = "UPDATE oppskrift_table SET category = '".$_POST['txt_category']."', bilde = '".$_POST['txt_bilde']."', title = '".$_POST['txt_title']."', duration = '".$_POST['txt_duration']."', servings = '".$_POST['txt_servings']."', ingredients = '".$_POST['txt_ingredients']."', directions = '".$_POST['txt_directions']."' WHERE id = '".$_POST['id']."' "; if(mysqli_query($con, $sql)){ echo "<script> alert('Success!'); window.location.href='edit.php'; </script>"; }else{ echo "An error has occurred. Please go back and check the code: ".mysqli_error($con); } } $id = ''; $category = ''; $bilde = ''; $title = ''; $duration = ''; $servings = ''; $ingredients = ''; $directions = ''; if (isset($_GET['id'])){ $sql = "SELECT id, category, bilde, title, duration, servings, ingredients, directions FROM oppskrift_table WHERE id=".$_GET['id']; $result = mysqli_query($con, $sql); if(mysqli_num_rows($result) > 0){ $row = mysqli_fetch_assoc($result); $id = $row['id']; $category = $row['category']; $bilde = $row['bilde']; $title = $row['title']; $duration = $row['duration']; $servings = $row['servings']; $ingredients = $row['ingredients']; $directions = $row['directions']; } } ?> <div class="container-fluid" style="width:60%; margin-top:3%;"> <!-- Form Name --> <legend>Edit a Recipe</legend> </div> <!-- INSTRUCTIONS --> <div class="container-fluid" style="width:58%; background-color:#ffffff;"> <p class="small" style="margin-top:1%;"><strong>Note:</strong> Entries are html dependent. Common tags to copy and paste:</p> <br> <table class="table small"> <thead> <tr> <th class="smaller">Code</th> <th class="smaller">Result</th> <th class="smaller">Code</th> <th class="smaller">Result</th> <tr> </tr> <td class="smaller"><br> </td> <td class="smaller"><em>line break </em></td> <td class="smaller">″ </td> <td class="smaller"><em>inches symbol </em></td> </tr> <tr> <td class="smaller">° </td> <td class="smaller"><em>° symbol </em></td> <td class="smaller"><b> </td> <td class="smaller"><em>bold text </em></td> </tr> <tr> <td class="smaller"> class="b0" </td> <td class="smaller"><em>style for lists <ul>, <li>, etc.</em></td> <td class="smaller">′ </td> <td class="smaller"><em>apostrophe </em></td> </tr> </table> </div> <!-- FORM --> <div class="container-fluid" style="width:60%; margin-top:3%;"> <form action="" method="post" class="form-horizontal"> <fieldset> <!-- Category --> <div class="form-group"> <label class="col-md-4 control-label">Category</label> <div class="col-md-4"> <input id="txt_category" name="txt_category" value="<?=$category?>" type="text" placeholder="" class="form-control input-md"> </div> </div> <!-- Bilde File --> <div class="form-group"> <label class="col-md-4 control-label">Bilde File</label> <div class="col-md-4"> <input id="txt_bilde" name="txt_bilde" value="<?=$bilde?>" type="text" placeholder="" class="form-control input-md"> </div> </div> <!-- Title --> <div class="form-group"> <label class="col-md-4 control-label" for="txt_title">Title</label> <div class="col-md-4"> <input id="txt_title" name="txt_title" value="<?=$title?>" type="text" placeholder="" class="form-control input-md"> </div> </div> <!-- Duration --> <div class="form-group"> <label class="col-md-4 control-label" for="txt_duration">Duration</label> <div class="col-md-4"> <input id="txt_duration" name="txt_duration" value="<?=$duration?>" type="text" placeholder="" class="form-control input-md"> </div> </div> <!-- Servings --> <div class="form-group"> <label class="col-md-4 control-label" for="txt_servings">Approx. Servings</label> <div class="col-md-4"> <input id="txt_servings" name="txt_servings" value="<?=$servings?>" type="text" placeholder="" class="form-control input-md"> </div> </div> <!-- Ingredients --> <div class="form-group"> <label class="col-md-4 control-label" for="txt_ingredients">Ingredients</label> <div class="col-md-6"> <textarea style="height:200px;" class="form-control lrgTextAreas" value="<?=$ingredients?>" id="txt_ingredients" name="txt_ingredients"></textarea> </div> </div> <!-- Directions --> <div class="form-group"> <label class="col-md-4 control-label" for="txt_directions">Directions</label> <div class="col-md-6"> <textarea style="height:200px;" class="form-control lrgTextAreas" value="<?=$directions?>" id="txt_directions" name="txt_directions"></textarea> </div> </div> <!-- Button --> <div class="form-group"> <label class="col-md-4 control-label" for="btn_submit"></label> <div class="col-md-4"> <button id="btn_submit" name="btn_submit" class="btn btn-primary">Submit</button> </div> </div> </fieldset> </form> </div>
The problem is that the edit page will populate the info into the form only until the end of the ingredients section. It will look like this on the page:
Somewhere in my code there is an error that's preventing the rest of the info (the directions section) from populating in the edit form. (It looks like a misplaced quotation mark since that's where the trouble starts.) I cannot find the error for the life of me. ?
-
High five. Thanks!
-
I created a recipe site that displays various dishes, and I'm tickled pink that I got it to work (first time doing this!) But I'd like to have pages that displays dishes by its category. The column that houses this is called "category" which has 7 total: Appetizers & Beverages, Soups & Salads, Side Items, Main Dishes, Baked Goods, Desserts, and Cookies & Candy.
I have this page connected to a "connection.php" page, but here's the code in question:
<!-- Page Title--> <div class="row"> <div class="col-lg-12"> <h1 class="page-header">Appetizers & Beverages</h1> </div> </div> <!-- /Page Title --> <!-- Displayed Data--> <?php $sql = "SELECT id, category, bilde, title FROM oppskrift_table ORDER BY title "; $result = mysqli_query($con, $sql); if(mysqli_num_rows($result) > 0 ){ while($row = mysqli_fetch_assoc($result)){ ?> <div align="center" class="col-md-3"> <a href="#"> <img class="img-responsive img-cat" src="bilder/rBilder/<?=$row['bilde']?>" width="250" alt=""> </a> <h4 style="max-width:250px;"> <a href="#" class="dishes"> <?=$row['title']?> </a> </h4> </div> <?php } } ?> <!-- END Displayed Data--> </div>
How can I tweak this so that only the category Appetizers & Beverages shows up on the page?
My code is broken *somewhere*
in PHP Coding Help
Posted
Thanks! They're going to write folk songs about you one day, mac_gyver.