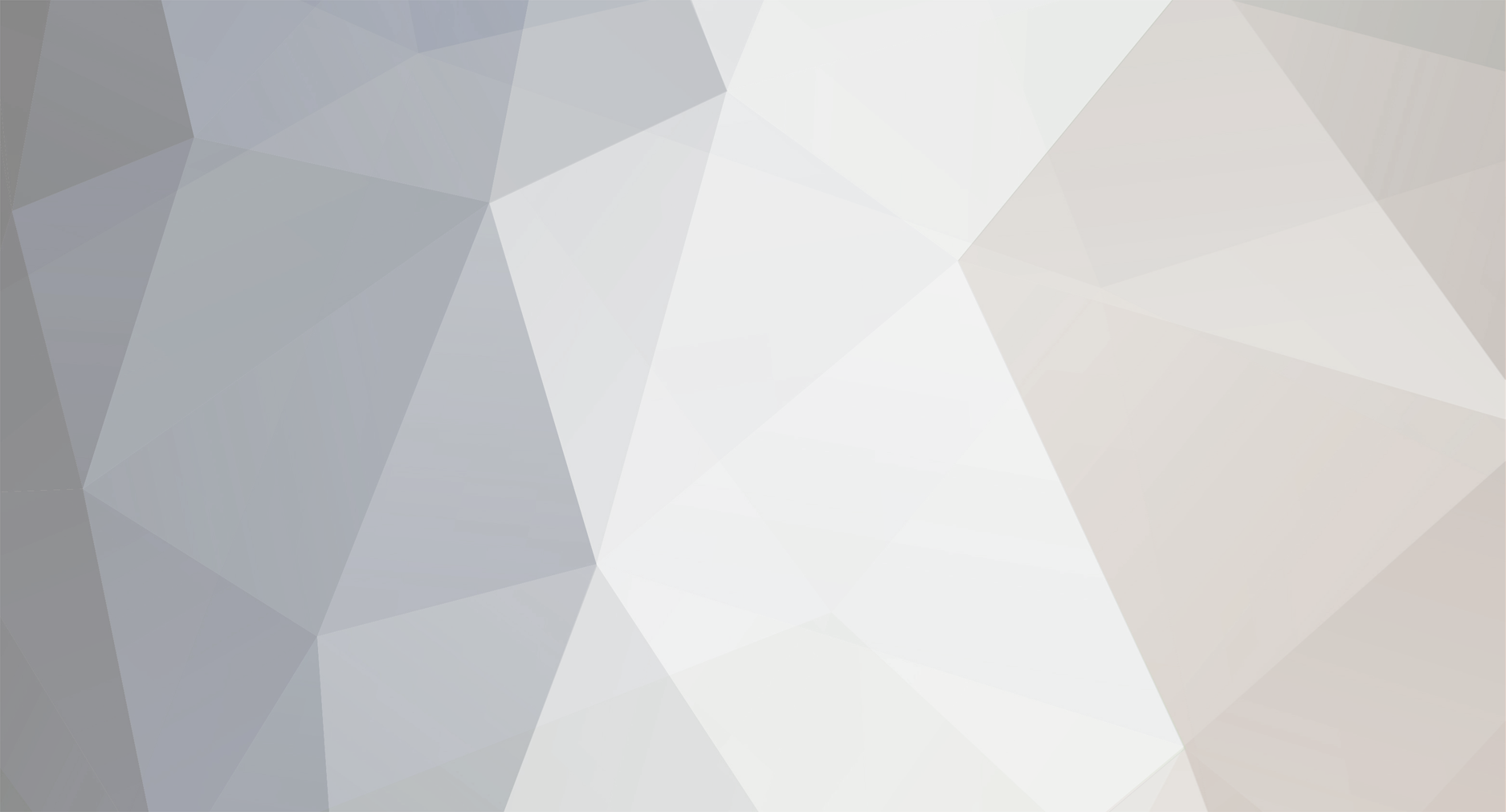
jevgienij
-
Posts
1 -
Joined
-
Last visited
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.
PHP+MySQL game server player database
in PHP Coding Help
Posted · Edited by jevgienij
Hello. I just inherited a game stats system but it's not working properly. I'm gonna post the entire script:
The problem is that for some nicknames it returns a proper output which should look like this:
But sometimes it just returns lots and lots of data even if the nickname I ask for is unique enough:
Here's how the database looks:
I don't know what's wrong with the script because I'm a PHP beginner. If someone could take a look at it and tell me what's the issue I'd appreciate.