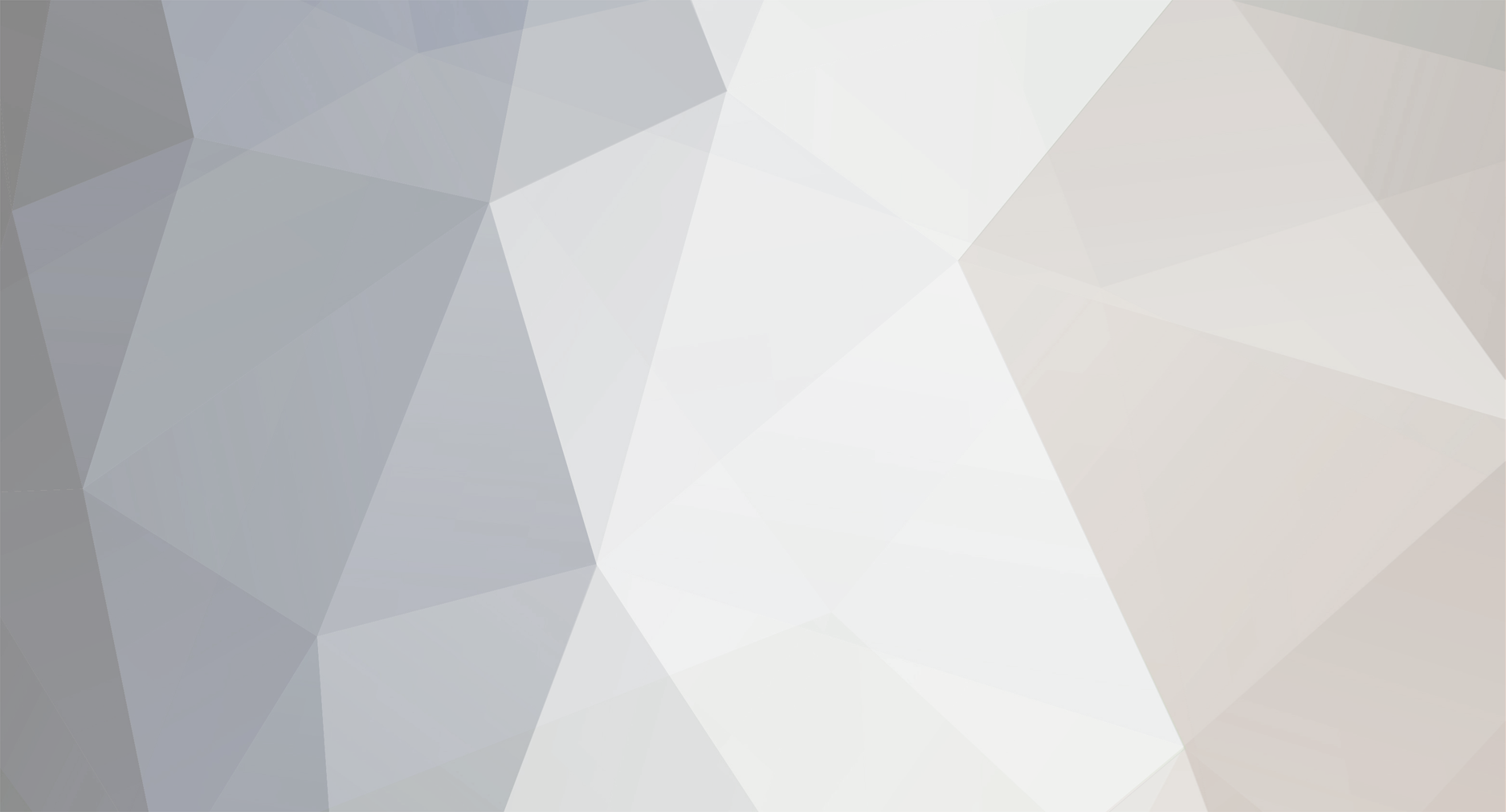
LyDev
-
Posts
2 -
Joined
-
Last visited
Posts posted by LyDev
-
-
I have insert in PDO. I need to write a select query before that and get the count of the rows. Based on that I must write an if condition before insert.
Below is my code.
<?php $dbhost = "localhost"; $dbuser = "root"; $dbpass = ""; $dbname = "test"; function placeholder( $text, $count = 0, $separator = ',' ) { $result = array(); if ($count > 0) { for ($x = 0; $x < $count; $x++) { $result[] = $text; } } return implode( $separator, $result ); } $pdo = new PDO("mysql:host=$dbhost;dbname=$dbname",$dbuser,$dbpass); $datafields = array( 'email' => '', 'var1' => '', 'var2' => '' ); $data[] = array( 'email' => 'a@mail.com', 'var1' => 'test', 'var2' => 'test2' ); $data[] = array( 'email' => 'b@mail.com', 'var1' => 'test', 'var2' => 'test2' ); $data[] = array( 'email' => 'c@mail.com', 'var1' => 'test', 'var2' => 'test2' ); $pdo->beginTransaction(); // Speed up your inserts $insertvalues = array(); foreach ($data as $d) { $questionmarks[] = '(' . placeholder( '?', sizeof($d)) . ')'; $insertvalues = array_merge( $insertvalues, array_values($d) ); } $sql = "INSERT INTO engagements (" . implode( ',', array_keys( $datafields ) ) . ") VALUES " . implode( ',', $questionmarks); $statement = $pdo->prepare($sql); try { $statement->execute($insertvalues); } catch(PDOException $e) { echo $e->getMessage(); } $pdo->commit();
How to use select and insert in single query in PDO
in MySQL Help
Posted
Insert query is there in the code . I need to place select query and an if condn