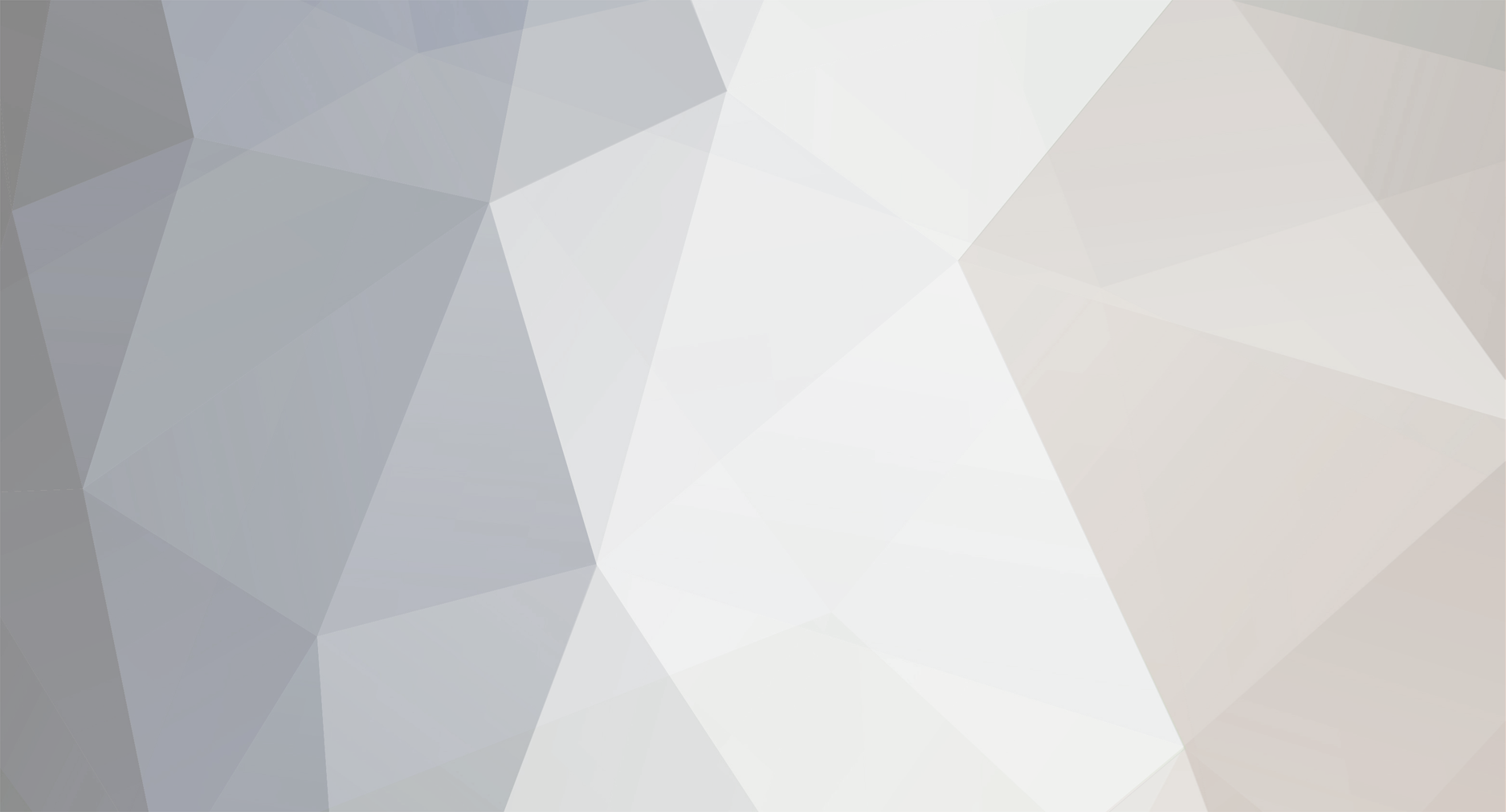
bjesltomvi
-
Posts
1 -
Joined
-
Last visited
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.
Array of objects with nested objects
in PHP Coding Help
Posted
Hello,
I have an array that I want to transfer it into another format. The array look like:
$data = [ [ "id"=>1, "type"=>"title", "data"=> "Title goes here" ], [ "id"=>2, "type"=>"repeat", "number"=>3, ], [ "id"=>3, "type"=>"media", "data"=> "path to media" ], [ "id"=>4, "type"=>"close_repeat" ], [ "id"=>5, "type"=>"repeat", "number"=>3, ], [ "id"=>6, "type"=>"title", "data"=> "Title goes here" ], [ "id"=>7, "type"=>"repeat", "number"=>2, ], [ "id"=>8, "type"=>"text", "data"=> "Text goes here" ], [ "id"=>9, "type"=>"close_repeat" ], [ "id"=>10, "type"=>"close_repeat" ], [ "id"=>11, "type"=>"cover", "data"=> "Cover data goes here" ], ];
And I want to transform it into:
{ "0": [ { "id": 1, "type": "title", "data": "Title goes here" } ], "1": [ { "id": 3, "type": "media", "data": "path to media" }, { "id": 3, "type": "media", "data": "path to media" }, { "id": 3, "type": "media", "data": "path to media" } ], "2":[ { "id": 6, "type": "title", "data": "Title goes here" }, { "id": 8, "type": "text", "data": "Text goes here" }, { "id": 8, "type": "text", "data": "Text goes here" } ], "3":[ { "id": 6, "type": "title", "data": "Title goes here" }, { "id": 8, "type": "text", "data": "Text goes here" }, { "id": 8, "type": "text", "data": "Text goes here" } ], "4":[ { "id": 6, "type": "title", "data": "Title goes here" }, { "id": 8, "type": "text", "data": "Text goes here" }, { "id": 8, "type": "text", "data": "Text goes here" } ], "5": [ { "id": 11, "type": "cover", "data": "Cover data goes here" } ] }
The idea is that each object can appear once or multiple times. If an object comes between objects of type repeat and close_repeat then it should be repeated based on number:x. The problem is when the repeat is nested as in id=5 to id=10. Here it should be that id=6 appear once with two repeated id=8. But in total, this whole id=6 and id=8 (twice) should appear three times since the repetition at id=5 is 3. I hope this is clear. I tried using loops:
public function dataTraversLoop($data, &$newData, $i){ $dataCount = count($data); if($i>=$dataCount){ return; } $objectIndex=0; for(;$i<$dataCount;$i++){ if($data[$i]['type']=='repeat'){ $objectIndex++; $repeat = $data[$i]['number']; $i++; for($j=0;$j<$repeat;$j++){ $tmpData = $data[$i]; $newData [$objectIndex][$j] = $tmpData; } } else if($data[$i]['type']=='close_repeat'){ $i++; $objectIndex++; } else{ $tmpData = $data[$i]; $newData [$objectIndex][] = $tmpData; } } }
And also recursion:
public function dataTravers($data, &$newData, &$repeat, $i, $objectIndex){ $dataCount = count($data); for( ; $i<$dataCount; $i++){ if($data[$i]['type']=='repeat'){ $repeat = $repeat * $data[$i]['number']; return $this->dataTravers($data, $newData, $repeat, $i+1, $objectIndex); } if($data[$i]['type']=='close_repeat'){ $repeat = 1; return $this->dataTravers($data, $newData, $repeat, $i+1, $objectIndex+1); } if($i >= $dataCount){ return $newData; } $tmpData = $data[$i]; $newData [$objectIndex][] = $tmpData; return $this->dataTravers($data, $newData, $repeat, $i+1, $objectIndex); $objectIndex++; }