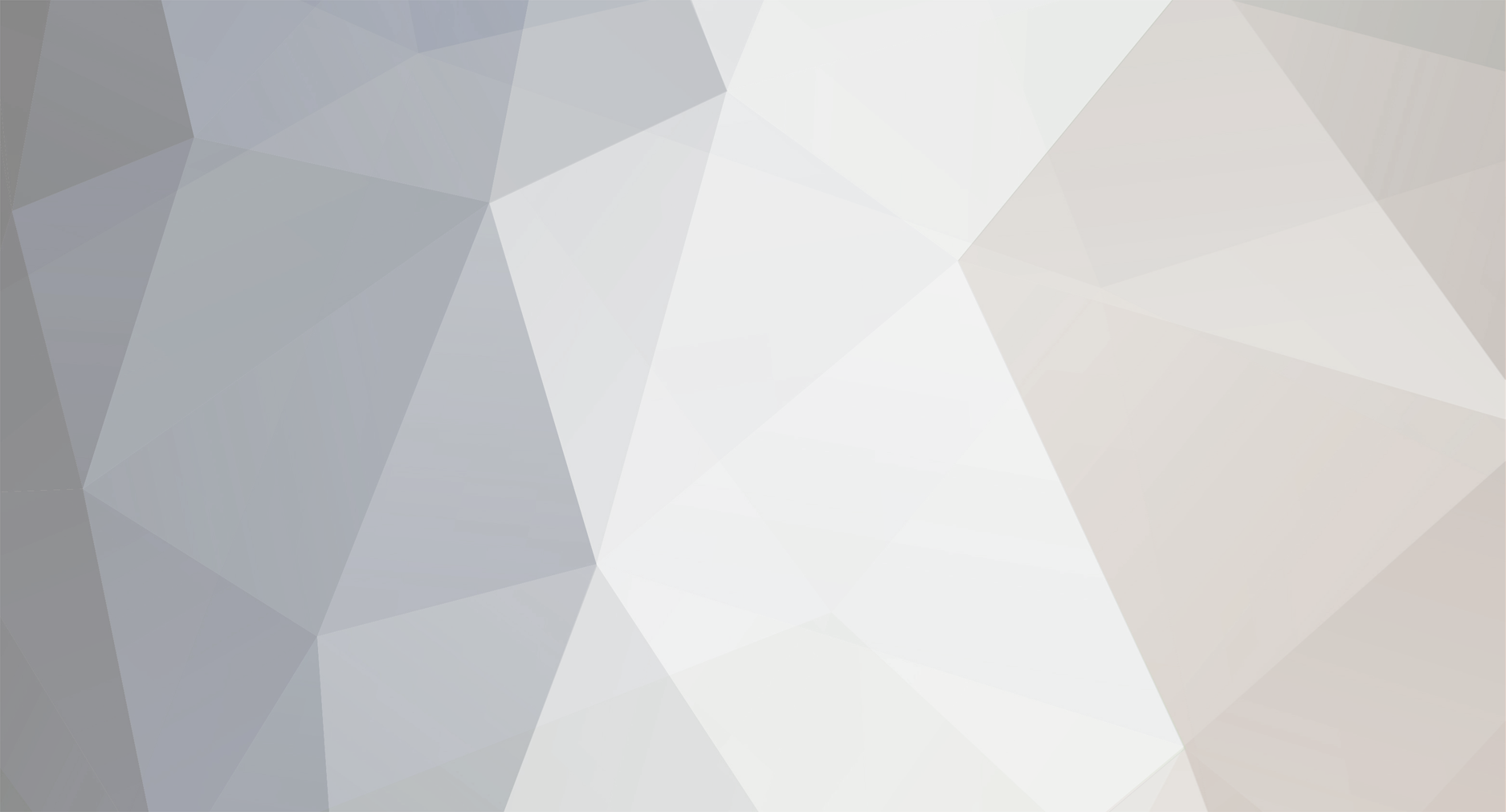
XCalibre3
Members-
Posts
49 -
Joined
-
Last visited
XCalibre3's Achievements
-
<!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>Login</title> <link rel="stylesheet" href="style.css"/> </head> <body> <?php mysqli_report(MYSQLI_REPORT_ERROR | MYSQLI_REPORT_STRICT); $servername = "localhost"; $username = "root"; $password = ""; $dbname = "calendar"; $conn = new mysqli($servername, $username, $password, $dbname); if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } session_start(); if (isset($_POST['submit '])) { $email=$_POST['username']; $password=$_POST['password']; $stmt = $mysqli->prepare("SELECT username,password FROM `admins` WHERE (username=? AND password=?)"); $stmt->bind_param('ss',$username,$password); $stmt->execute(); $stmt->bind_result($username,$password); $rs= $stmt->fetch (); if (!$rs) { echo "<div class='form'> <h3>Incorrect Username/password.</h3><br/> <p class='link'>Click here to <a href='login.php'>Login</a> again.</p> </div>"; } else { $_SESSION['username']=$username; header('location:welcome.php'); } } else { ?> <form class="form" method="post" name="login"> <h1 class="login-title">Login</h1> <input type="text" class="login-input" name="username" placeholder="Username" autofocus="true"/> <input type="password" class="login-input" name="password" placeholder="Password"/> <input type="submit" value="Login" name="submit" class="login-button"/> <p class="link"><a href="registration.php">New Registration</a></p> </form> <?php } ?> </body> </html>
-
Notice: Only variables should be passed by reference
XCalibre3 replied to XCalibre3's topic in PHP Coding Help
Thank you... I changed $result = mysqli_stmt_get_result($stmt); TO: $result = mysqli_stmt_affected_rows($stmt); It now shows registered successfully. TYTY -
Notice: Only variables should be passed by reference
XCalibre3 replied to XCalibre3's topic in PHP Coding Help
Okay, thank you very much. Sorry, I'm new to prepared statements... that's why I can't login to the admin panel yet. I have to change all that to prepared statements. I still get that the required fields are missing, even though it puts it all in the database. And I thought the datetime was set correctly? Here is the code I have changed: <!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>Registration</title> <link rel="stylesheet" href="style.css"/> </head> <body> <?php mysqli_report(MYSQLI_REPORT_ERROR | MYSQLI_REPORT_STRICT); $servername = "localhost"; $username = "root"; $password = ""; $dbname = "calendar"; // Establish connection $conn = new mysqli($servername, $username, $password, $dbname); // Verify connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } ?> <?php if (isset($_POST['submit'])) { $username = $_POST['username']; $email = $_POST['email']; $phone = $_POST['phone']; $address = $_POST['address']; $state = $_POST['state']; $password = $_POST['password']; $reg_date = date("Y-m-d h:i:s"); $isadmin = '0'; $password = password_hash($password, PASSWORD_DEFAULT); $stmt = $conn->prepare("INSERT INTO `admins` (username, email, phone, address, state, password, isadmin, reg_date) VALUES (?, ?, ?, ?, ?, ?, ?, ?)"); $stmt->bind_param("ssssssis", $username, $email, $phone, $address, $state, $password, $isadmin, $reg_date); $stmt->execute(); $result = mysqli_stmt_get_result($stmt); if ($result) { echo "<div class='form'> <h3>You are registered successfully.</h3><br/> <p class='link'>Click here to <a href='login.php'>Login</a></p> </div>"; } else { echo "<div class='form'> <h3>Required fields are missing.</h3><br/> <p class='link'>Click here to <a href='registration.php'>registration</a> again.</p> </div>"; } } else { ?> <form class="form" action="" method="post"> <h1 class="login-title">Registration</h1> <input type="text" class="login-input" name="username" placeholder="Username" required /> <input type="text" class="login-input" name="email" placeholder="Email Adress" required /> <input type="text" class="login-input" name="phone" placeholder="Phone Number" required /> <input type="text" class="login-input" name="address" placeholder="Address" required /> <input type="text" class="login-input" name="state" placeholder="State" required /> <input type="password" class="login-input" name="password" placeholder="Password" required /> <input type="submit" name="submit" value="Register" class="login-button"> <p class="link"><a href="login.php">Click to Login</a></p> </form> <?php } ?> </body> </html> As stated.. it puts it all in database but doesn't actually register it. -
Notice: Only variables should be passed by reference
XCalibre3 replied to XCalibre3's topic in PHP Coding Help
Okay I changed this to hash password: $stmt->bind_param("ssssssis", $username, $email, $phone, $address, $state, md5($password), $isadmin, $create_datetime); Everything inserts into the database just fine, and the $create_datetime inserts: 2023-05-02 07:16:40. So it all seems to work fine and inserts into database, but this is the error I get Notice: Only variables should be passed by reference in C:\xampp\htdocs\sliders\registration.php on line 39 if (isset($_POST['submit'])) { $username = $_POST['username']; $email = $_POST['email']; $phone = $_POST['phone']; $address = $_POST['address']; $state = $_POST['state']; $password = $_POST['password']; $create_datetime = date("Y-m-d H:i:s"); $isadmin = '0'; $stmt = $conn->prepare("INSERT INTO `admins` (username, email, phone, address, state, password, isadmin, create_datetime) VALUES (?, ?, ?, ?, ?, ?, ?, ?)"); Line 39: $stmt->bind_param("ssssssis", $username, $email, $phone, $address, $state, md5($password), $isadmin, $create_datetime); $stmt->execute(); -
Notice: Only variables should be passed by reference
XCalibre3 replied to XCalibre3's topic in PHP Coding Help
Okay, I have changed it to either 0 or 1.... but here is where I'm at now. <?php if (isset($_POST['submit'])) { $username = $_POST['username']; $email = $_POST['email']; $phone = $_POST['phone']; $address = $_POST['address']; $state = $_POST['state']; $password = $_POST['password']; $create_datetime = date("Y-m-d H:i:s"); $isadmin = "0"; $stmt = $conn->prepare("INSERT INTO `admins` (username, email, phone, address, state, password, isadmin, create_datetime) VALUES (?, ?, ?, ?, ?, ?, ?, ?)"); $stmt->bind_param("ssssssii", $username, $email, $phone, $address, $state, $password, $isadmin, $create_datetime); $stmt->execute(); $conn->close(); $result = mysqli_stmt_get_result($stmt); if ($result) { echo "<div class='form'> <h3>You are registered successfully.</h3><br/> <p class='link'>Click here to <a href='login.php'>Login</a></p> </div>"; } else { echo "<div class='form'> <h3>Required fields are missing.</h3><br/> <p class='link'>Click here to <a href='registration.php'>registration</a> again.</p> </div>"; } } else { ?> <form class="form" action="" method="post"> <h1 class="login-title">Registration</h1> <input type="text" class="login-input" name="username" placeholder="Username" required /> <input type="text" class="login-input" name="email" placeholder="Email Adress" required /> <input type="text" class="login-input" name="phone" placeholder="Phone Number" required /> <input type="text" class="login-input" name="address" placeholder="Address" required /> <input type="text" class="login-input" name="state" placeholder="State" required /> <input type="password" class="login-input" name="password" placeholder="Password" required /> <input type="submit" name="submit" value="Register" class="login-button"> <p class="link"><a href="login.php">Click to Login</a></p> </form> <?php } ?> </body> </html> It keeps showing Required fields are missing. Click here to registration again. But I have enetered all the required fields. Makes no sense. It is entering into database though... but the login won't work. -
Notice: Only variables should be passed by reference
XCalibre3 replied to XCalibre3's topic in PHP Coding Help
Thank you, changed to S -
Notice: Only variables should be passed by reference
XCalibre3 replied to XCalibre3's topic in PHP Coding Help
Maybe like this $dummy = "Yes"; $yes = $dummy; -
Notice: Only variables should be passed by reference
XCalibre3 replied to XCalibre3's topic in PHP Coding Help
Maybe because of the 'Yes'? hmmm If so, how would I write that? -
I don't know why I get this error??? Notice: Only variables should be passed by reference in C:\xampp\htdocs\sliders\registration.php on line 38 Fatal error: Uncaught Error: mysqli_stmt::bind_param(): Argument #8 cannot be passed by reference in C:\xampp\htdocs\sliders\registration.php:38 Stack trace: #0 {main} thrown in C:\xampp\htdocs\sliders\registration.php on line 38 $servername = "localhost"; $username = "root"; $password = ""; $dbname = "calendar"; // Establish connection $conn = new mysqli($servername, $username, $password, $dbname); // Verify connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } if (isset($_POST['submit'])) { $username = $_POST['username']; $email = $_POST['email']; $phone = $_POST['phone']; $address = $_POST['address']; $state = $_POST['state']; $password = $_POST['password']; $create_datetime = date("Y-m-d H:i:s"); $stmt = $conn->prepare("INSERT INTO `admins` (username, email, phone, address, state, password, isadmin, create_datetime) VALUES (?, ?, ?, ?, ?, ?, ?, ?)"); $stmt->bind_param("ssissssi", $username, $email, $phone, $address, $state, $password, 'Yes', $create_datetime); $stmt->execute(); $conn->close();
-
AmI getting closer? lol... I'm really trying here... This shows a blank page, no error reporting. <?php ini_set('display_errors', 1); ini_set('display_startup_errors', 1); error_reporting(E_ALL); $database = 'calendar'; $tb_Select = new mysqli("localhost", "root", "", $database ); $sql = "SELECT * FROM `reservations` WHERE id=?"; $stmt = $tb_Select->prepare($sql); $stmt->bind_param('i', $id); $stmt->execute(); $result = $stmt->get_result(); while ($row = $result->fetch_assoc()) { echo $row['id']; echo $row['fname']; echo $row['lname']; echo $row['email']; }?>
-
also tried this but doesn't recognize variable tb_Select <?php $database = calendar $tb_Select = new mysqli("localhost", "root", "", $database ); $query = "SELECT * from `reservations` WHERE ID=? "; $stmt = $mysqli->prepare($tb_Select, $query); $stmt->bind_param("i", $id); $stmt->execute(); $res = $stmt->get_result(); $data = $res->fetch_all(MYSQLI_ASSOC); while($data = mysqli_fetch_array($stmt)){ ?> <h2 align="center"> <?php echo $row['id']; ?> </h2><br> <div class="paracenter"> <p id="cont"><?php echo $row['fname']; ?></p> <hr color="black" width="10%"> </div> <?php } ?> This either: <?php $database = 'calendar'; $tb_Select = new mysqli("localhost", "root", "", $database ); $sql = "SELECT * FROM reservations WHERE id=?"; $stmt = $tb_Select->prepare($sql); $stmt->bind_param("i", $id); $stmt->execute(); $result = $stmt->get_result(); while ($row = $result->fetch_assoc()) { echo $row['fname']; }?>
-
I see one issue lol, I'm trying to call ID before ID is defined.... I define i after.... again, I'm not used to this prepared statement stuff. Sorry for so many questions. But it also says: Parse error: syntax error, unexpected variable "$tb_Select"
-
Okay, I'm working on SELECT now and it won't work. <?php $database = calendar $tb_Select = new mysqli("localhost", "root", "", $database ); $stmt = $tb_Select->prepare("SELECT * FROM reservations WHERE id = ?"); $stmt->bind_param("s", $_GET['id']); $stmt->execute(); $stmt->store_result(); if($stmt->num_rows === 0) exit('No rows'); $stmt->bind_result($id, $fname, $lname); while($stmt->fetch()) { $ids[] = $id; $names[] = $fname; $ages[] = $lname; } echo $ids; var_export($ids); $stmt->close(); ?>
-
Thanks for the info... looks hard though.
-
Is there a prepeared statement that's ust mysqli, or does it always have to have PDO? I can't find anyting on it. Thank you. II mean for fetch all and select all together