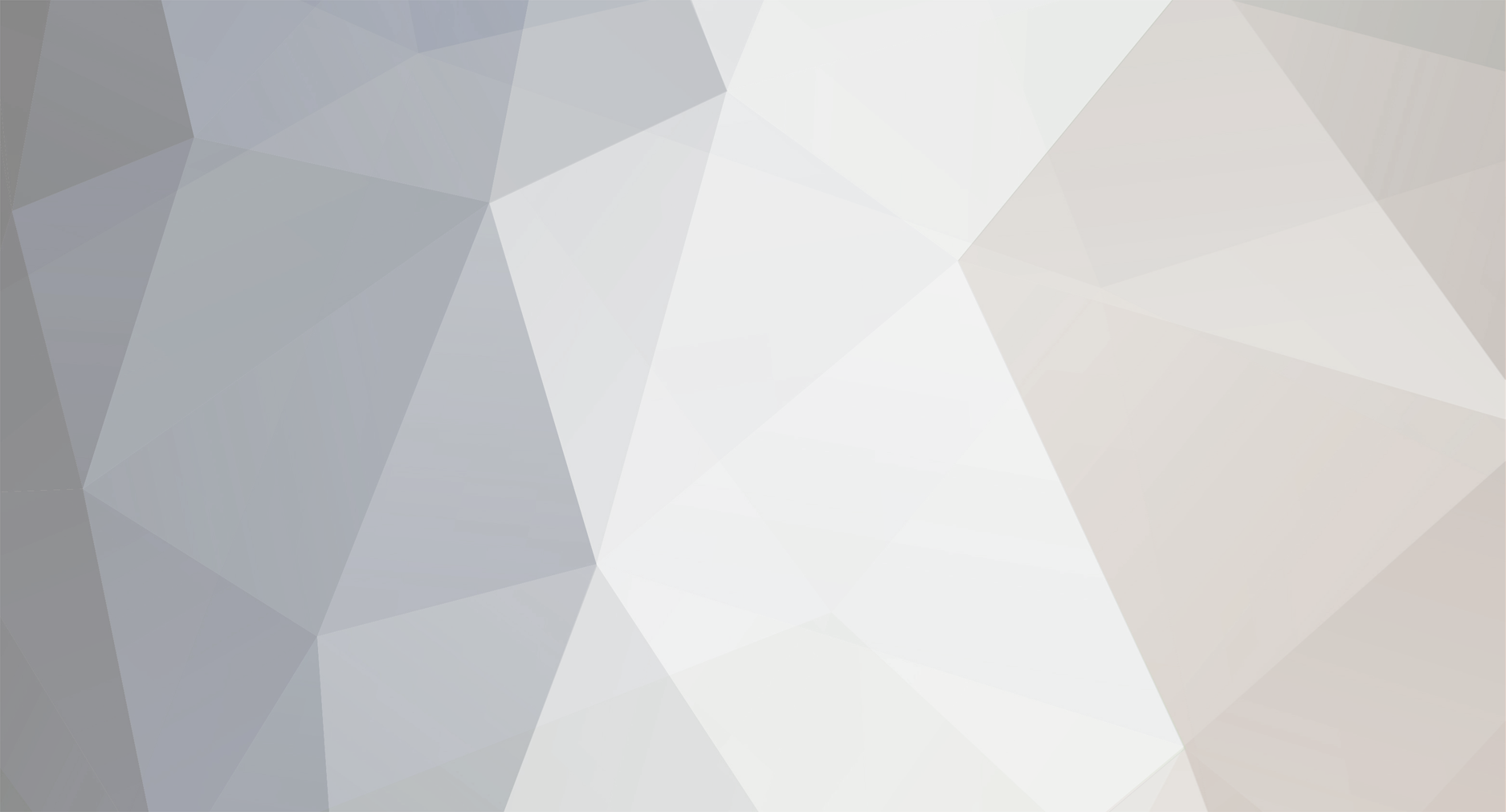
XCalibre3
Members-
Posts
49 -
Joined
-
Last visited
Everything posted by XCalibre3
-
<!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>Login</title> <link rel="stylesheet" href="style.css"/> </head> <body> <?php mysqli_report(MYSQLI_REPORT_ERROR | MYSQLI_REPORT_STRICT); $servername = "localhost"; $username = "root"; $password = ""; $dbname = "calendar"; $conn = new mysqli($servername, $username, $password, $dbname); if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } session_start(); if (isset($_POST['submit '])) { $email=$_POST['username']; $password=$_POST['password']; $stmt = $mysqli->prepare("SELECT username,password FROM `admins` WHERE (username=? AND password=?)"); $stmt->bind_param('ss',$username,$password); $stmt->execute(); $stmt->bind_result($username,$password); $rs= $stmt->fetch (); if (!$rs) { echo "<div class='form'> <h3>Incorrect Username/password.</h3><br/> <p class='link'>Click here to <a href='login.php'>Login</a> again.</p> </div>"; } else { $_SESSION['username']=$username; header('location:welcome.php'); } } else { ?> <form class="form" method="post" name="login"> <h1 class="login-title">Login</h1> <input type="text" class="login-input" name="username" placeholder="Username" autofocus="true"/> <input type="password" class="login-input" name="password" placeholder="Password"/> <input type="submit" value="Login" name="submit" class="login-button"/> <p class="link"><a href="registration.php">New Registration</a></p> </form> <?php } ?> </body> </html>
-
Notice: Only variables should be passed by reference
XCalibre3 replied to XCalibre3's topic in PHP Coding Help
Thank you... I changed $result = mysqli_stmt_get_result($stmt); TO: $result = mysqli_stmt_affected_rows($stmt); It now shows registered successfully. TYTY -
Notice: Only variables should be passed by reference
XCalibre3 replied to XCalibre3's topic in PHP Coding Help
Okay, thank you very much. Sorry, I'm new to prepared statements... that's why I can't login to the admin panel yet. I have to change all that to prepared statements. I still get that the required fields are missing, even though it puts it all in the database. And I thought the datetime was set correctly? Here is the code I have changed: <!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>Registration</title> <link rel="stylesheet" href="style.css"/> </head> <body> <?php mysqli_report(MYSQLI_REPORT_ERROR | MYSQLI_REPORT_STRICT); $servername = "localhost"; $username = "root"; $password = ""; $dbname = "calendar"; // Establish connection $conn = new mysqli($servername, $username, $password, $dbname); // Verify connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } ?> <?php if (isset($_POST['submit'])) { $username = $_POST['username']; $email = $_POST['email']; $phone = $_POST['phone']; $address = $_POST['address']; $state = $_POST['state']; $password = $_POST['password']; $reg_date = date("Y-m-d h:i:s"); $isadmin = '0'; $password = password_hash($password, PASSWORD_DEFAULT); $stmt = $conn->prepare("INSERT INTO `admins` (username, email, phone, address, state, password, isadmin, reg_date) VALUES (?, ?, ?, ?, ?, ?, ?, ?)"); $stmt->bind_param("ssssssis", $username, $email, $phone, $address, $state, $password, $isadmin, $reg_date); $stmt->execute(); $result = mysqli_stmt_get_result($stmt); if ($result) { echo "<div class='form'> <h3>You are registered successfully.</h3><br/> <p class='link'>Click here to <a href='login.php'>Login</a></p> </div>"; } else { echo "<div class='form'> <h3>Required fields are missing.</h3><br/> <p class='link'>Click here to <a href='registration.php'>registration</a> again.</p> </div>"; } } else { ?> <form class="form" action="" method="post"> <h1 class="login-title">Registration</h1> <input type="text" class="login-input" name="username" placeholder="Username" required /> <input type="text" class="login-input" name="email" placeholder="Email Adress" required /> <input type="text" class="login-input" name="phone" placeholder="Phone Number" required /> <input type="text" class="login-input" name="address" placeholder="Address" required /> <input type="text" class="login-input" name="state" placeholder="State" required /> <input type="password" class="login-input" name="password" placeholder="Password" required /> <input type="submit" name="submit" value="Register" class="login-button"> <p class="link"><a href="login.php">Click to Login</a></p> </form> <?php } ?> </body> </html> As stated.. it puts it all in database but doesn't actually register it. -
Notice: Only variables should be passed by reference
XCalibre3 replied to XCalibre3's topic in PHP Coding Help
Okay I changed this to hash password: $stmt->bind_param("ssssssis", $username, $email, $phone, $address, $state, md5($password), $isadmin, $create_datetime); Everything inserts into the database just fine, and the $create_datetime inserts: 2023-05-02 07:16:40. So it all seems to work fine and inserts into database, but this is the error I get Notice: Only variables should be passed by reference in C:\xampp\htdocs\sliders\registration.php on line 39 if (isset($_POST['submit'])) { $username = $_POST['username']; $email = $_POST['email']; $phone = $_POST['phone']; $address = $_POST['address']; $state = $_POST['state']; $password = $_POST['password']; $create_datetime = date("Y-m-d H:i:s"); $isadmin = '0'; $stmt = $conn->prepare("INSERT INTO `admins` (username, email, phone, address, state, password, isadmin, create_datetime) VALUES (?, ?, ?, ?, ?, ?, ?, ?)"); Line 39: $stmt->bind_param("ssssssis", $username, $email, $phone, $address, $state, md5($password), $isadmin, $create_datetime); $stmt->execute(); -
Notice: Only variables should be passed by reference
XCalibre3 replied to XCalibre3's topic in PHP Coding Help
Okay, I have changed it to either 0 or 1.... but here is where I'm at now. <?php if (isset($_POST['submit'])) { $username = $_POST['username']; $email = $_POST['email']; $phone = $_POST['phone']; $address = $_POST['address']; $state = $_POST['state']; $password = $_POST['password']; $create_datetime = date("Y-m-d H:i:s"); $isadmin = "0"; $stmt = $conn->prepare("INSERT INTO `admins` (username, email, phone, address, state, password, isadmin, create_datetime) VALUES (?, ?, ?, ?, ?, ?, ?, ?)"); $stmt->bind_param("ssssssii", $username, $email, $phone, $address, $state, $password, $isadmin, $create_datetime); $stmt->execute(); $conn->close(); $result = mysqli_stmt_get_result($stmt); if ($result) { echo "<div class='form'> <h3>You are registered successfully.</h3><br/> <p class='link'>Click here to <a href='login.php'>Login</a></p> </div>"; } else { echo "<div class='form'> <h3>Required fields are missing.</h3><br/> <p class='link'>Click here to <a href='registration.php'>registration</a> again.</p> </div>"; } } else { ?> <form class="form" action="" method="post"> <h1 class="login-title">Registration</h1> <input type="text" class="login-input" name="username" placeholder="Username" required /> <input type="text" class="login-input" name="email" placeholder="Email Adress" required /> <input type="text" class="login-input" name="phone" placeholder="Phone Number" required /> <input type="text" class="login-input" name="address" placeholder="Address" required /> <input type="text" class="login-input" name="state" placeholder="State" required /> <input type="password" class="login-input" name="password" placeholder="Password" required /> <input type="submit" name="submit" value="Register" class="login-button"> <p class="link"><a href="login.php">Click to Login</a></p> </form> <?php } ?> </body> </html> It keeps showing Required fields are missing. Click here to registration again. But I have enetered all the required fields. Makes no sense. It is entering into database though... but the login won't work. -
Notice: Only variables should be passed by reference
XCalibre3 replied to XCalibre3's topic in PHP Coding Help
Thank you, changed to S -
Notice: Only variables should be passed by reference
XCalibre3 replied to XCalibre3's topic in PHP Coding Help
Maybe like this $dummy = "Yes"; $yes = $dummy; -
Notice: Only variables should be passed by reference
XCalibre3 replied to XCalibre3's topic in PHP Coding Help
Maybe because of the 'Yes'? hmmm If so, how would I write that? -
I don't know why I get this error??? Notice: Only variables should be passed by reference in C:\xampp\htdocs\sliders\registration.php on line 38 Fatal error: Uncaught Error: mysqli_stmt::bind_param(): Argument #8 cannot be passed by reference in C:\xampp\htdocs\sliders\registration.php:38 Stack trace: #0 {main} thrown in C:\xampp\htdocs\sliders\registration.php on line 38 $servername = "localhost"; $username = "root"; $password = ""; $dbname = "calendar"; // Establish connection $conn = new mysqli($servername, $username, $password, $dbname); // Verify connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } if (isset($_POST['submit'])) { $username = $_POST['username']; $email = $_POST['email']; $phone = $_POST['phone']; $address = $_POST['address']; $state = $_POST['state']; $password = $_POST['password']; $create_datetime = date("Y-m-d H:i:s"); $stmt = $conn->prepare("INSERT INTO `admins` (username, email, phone, address, state, password, isadmin, create_datetime) VALUES (?, ?, ?, ?, ?, ?, ?, ?)"); $stmt->bind_param("ssissssi", $username, $email, $phone, $address, $state, $password, 'Yes', $create_datetime); $stmt->execute(); $conn->close();
-
AmI getting closer? lol... I'm really trying here... This shows a blank page, no error reporting. <?php ini_set('display_errors', 1); ini_set('display_startup_errors', 1); error_reporting(E_ALL); $database = 'calendar'; $tb_Select = new mysqli("localhost", "root", "", $database ); $sql = "SELECT * FROM `reservations` WHERE id=?"; $stmt = $tb_Select->prepare($sql); $stmt->bind_param('i', $id); $stmt->execute(); $result = $stmt->get_result(); while ($row = $result->fetch_assoc()) { echo $row['id']; echo $row['fname']; echo $row['lname']; echo $row['email']; }?>
-
also tried this but doesn't recognize variable tb_Select <?php $database = calendar $tb_Select = new mysqli("localhost", "root", "", $database ); $query = "SELECT * from `reservations` WHERE ID=? "; $stmt = $mysqli->prepare($tb_Select, $query); $stmt->bind_param("i", $id); $stmt->execute(); $res = $stmt->get_result(); $data = $res->fetch_all(MYSQLI_ASSOC); while($data = mysqli_fetch_array($stmt)){ ?> <h2 align="center"> <?php echo $row['id']; ?> </h2><br> <div class="paracenter"> <p id="cont"><?php echo $row['fname']; ?></p> <hr color="black" width="10%"> </div> <?php } ?> This either: <?php $database = 'calendar'; $tb_Select = new mysqli("localhost", "root", "", $database ); $sql = "SELECT * FROM reservations WHERE id=?"; $stmt = $tb_Select->prepare($sql); $stmt->bind_param("i", $id); $stmt->execute(); $result = $stmt->get_result(); while ($row = $result->fetch_assoc()) { echo $row['fname']; }?>
-
I see one issue lol, I'm trying to call ID before ID is defined.... I define i after.... again, I'm not used to this prepared statement stuff. Sorry for so many questions. But it also says: Parse error: syntax error, unexpected variable "$tb_Select"
-
Okay, I'm working on SELECT now and it won't work. <?php $database = calendar $tb_Select = new mysqli("localhost", "root", "", $database ); $stmt = $tb_Select->prepare("SELECT * FROM reservations WHERE id = ?"); $stmt->bind_param("s", $_GET['id']); $stmt->execute(); $stmt->store_result(); if($stmt->num_rows === 0) exit('No rows'); $stmt->bind_result($id, $fname, $lname); while($stmt->fetch()) { $ids[] = $id; $names[] = $fname; $ages[] = $lname; } echo $ids; var_export($ids); $stmt->close(); ?>
-
Thanks for the info... looks hard though.
-
Is there a prepeared statement that's ust mysqli, or does it always have to have PDO? I can't find anyting on it. Thank you. II mean for fetch all and select all together
-
UPDATE: Is working if(isset($_POST['flagged'])) { $tb_Update = new mysqli("localhost", "root", "", $database ); $stmt = $tb_Update->prepare("UPDATE `reservations` SET flagged = 'Yes' WHERE id = ?"); $stmt->bind_param('i', $_POST['flagged']); echo "<style> {background-color:red;}</style>"; $stmt->execute(); $stmt->close(); }
-
Okay, I came up with this. Still have learn insert and the other but thank you for the link. Then Got to learn how to transfer to the history table and then delete it from the regular table. if(isset($_POST['delete'])) { $tb_Delete = new mysqli("localhost", "root", "", $database ); $stmt = $tb_Delete->prepare('DELETE FROM `reservations` WHERE id = ?'); $stmt->bind_param('i', $_POST['delete']); $stmt->execute(); $stmt->close(); }
-
I still only know the old code, so new to this. This is what I came up with but doesn't work. if(isset($_POST['delete'])) { $stmt->bind_param("s",$_POST['delete']); $mysqli = new mysqli(`localhost`, `root`, '', `reservations` ); $stmt = $mysqli -> prepare('DELETE FROM `reservations` WHERE id = ?'); $stmt -> bind_param('s', $id); $stmt -> execute();
-
Hey all, trying to do this but nothing works. $sql = "SELECT * FROM `reservations` WHERE id = $_POST[delete]"; $sql1 = "INSERT INTO `history_reservations` (fname,lname,email,phone,dived,dropdown,reserved,resnum) WHERE id = $_POST[delete]"; $result = $mysqli->query($sql1); $mysqli->close(); Basically delete a reservation and put it into a history table.
-
I just changed the <td> color instead. Don't know if there's anyone online to help, but I did accomplish changing the <td>; which works for what I wanted.
-
Okay, I ended up doing this instead. I turned the button text color to red, so flagged shows as red, which means they either no showed on the reservation, or gave trouble to the diving instructor. Flagged in red lets us decide if we want to allow them to come again. but it won't change the background color; which I would like. Any suggestions? <?php if ($value['flagged'] == 'Yes') { echo '<td><button type="submit" style=color:red; name="flagged" value="'.htmlspecialchars($value['id']).'">Flagged</button></td>'; }else{ ?> <?php echo '<td><button type="submit" name="flagged" value="'.htmlspecialchars($value['id']).'">Flagged</button></td>'; ?> I tried the basic style= again and didn't work
-
Okay it's working now LOL! SMH..... but how to change from status to flagged on mysql button? Would it be the same php code to change name on a button, or do I need to write it diferently b/c it's in thee database?
-
OMG I'm laughing so hard.... DUH! I'll pput the form and see what happens lol
-
This is the database code: <?php $servername='localhost'; $username="root"; $password=""; try { $con=new PDO("mysql:host=$servername;dbname=calendar",$username,$password); $con->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION); //echo 'connected'; } catch(PDOException $e) { echo ''.$e->getMessage(); } $searchErr = ''; $customer_details=''; if(isset($_POST['save'])) { if(!empty($_POST['search'])) { $search = $_POST['search']; $stmt = $con->prepare("select * from reservations where fname like '%$search%' or lname like '%$search%' or resnum like '%$search%' or email like '%$search%' or phone like '%$search%'"); $stmt->execute(); $customer_details = $stmt->fetchAll(PDO::FETCH_ASSOC); //print_r($customer_details); ?> <div class="table-responsive"> <table class="table"> <tr> <th>#</th> <th>Name</th> <th>Email</th> <th>Phone</th> <th>Reservation #</th> </tr> <?php if(!$customer_details) { } else{ foreach($customer_details as $key=>$value) { ?> <tr> <td><?php echo $key+1;?>  </td> <td><?php echo $value['fname'];?> <?php echo $value['lname'];?></td> <td>    <?php echo $value['email'];?></td> <td>    <?php echo $value['phone'];?></td> <td>    <?php echo $value['resnum'];?></td> <?php echo '<td><button type="submit" name="status" value="'.htmlspecialchars($value['id']).'">status</button></td>'; ?> </tr> <?php } } } else { $searchErr = "Please enter the information"; } } if(isset($_POST['status'])) { echo "working"; } The echo working was just a try to see what happend. I still need button to change to flagged and database to change too 1.
-
I have written this code: <?php if(!$customer_details) { } else{ foreach($customer_details as $key=>$value) { ?> <tr> <td><?php echo $key+1;?>  </td> <td><?php echo $value['fname'];?> <?php echo $value['lname'];?></td> <td>    <?php echo $value['email'];?></td> <td>    <?php echo $value['phone'];?></td> <td>    <?php echo $value['resnum'];?></td> <td><?php echo '<td><button type="submit" name="status" value='.$value['id'].' />status</button></td>'; ?> </tr> <?php I know, the code is not lined and a mess.... ..... but, I've tried using php to make the status button try even the simplest echo something and can't get it figured out. I need the button to change from status to flagged if possible, and then in database change 0 to 1 Thank you!