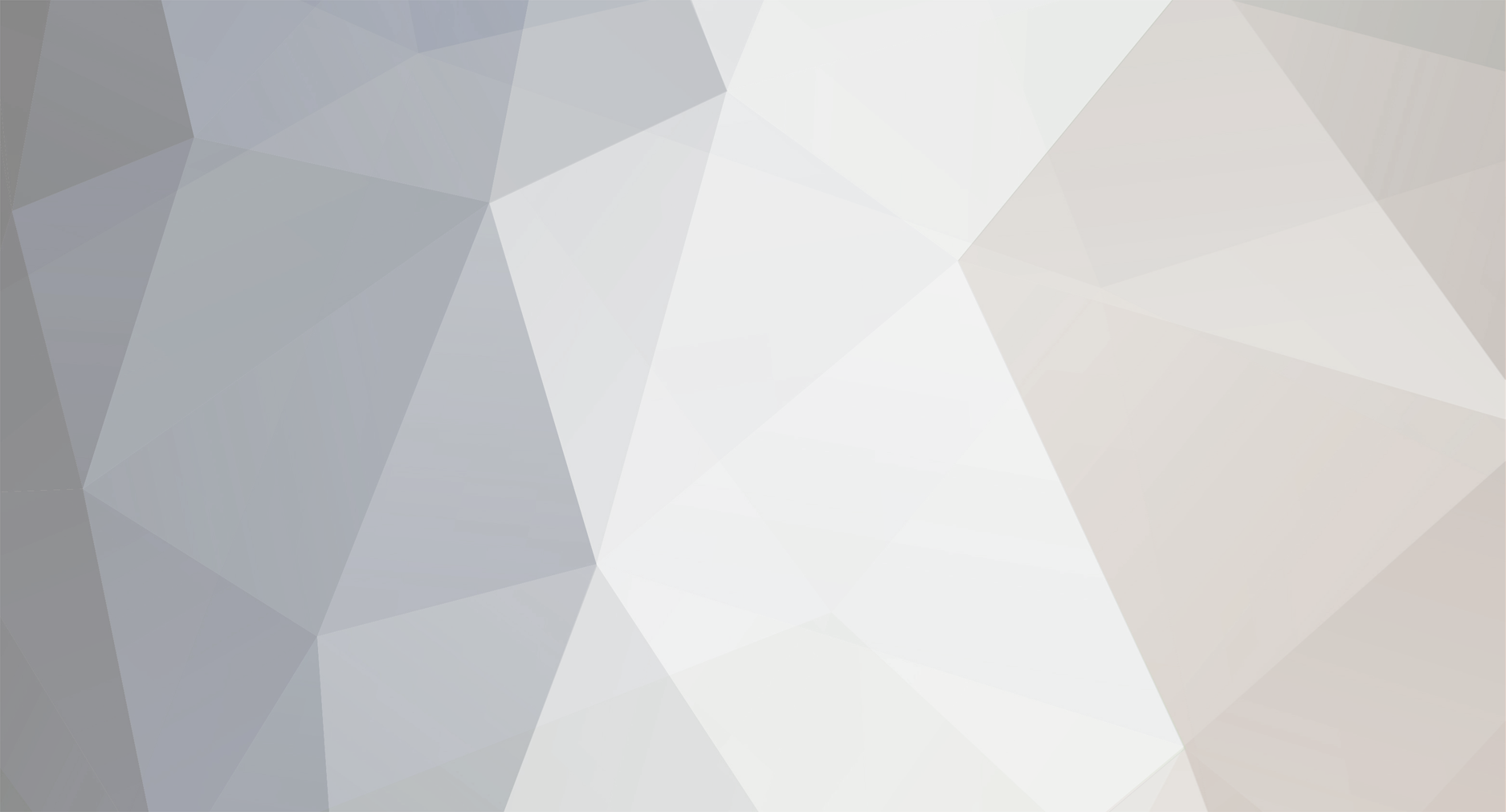
michael222
-
Posts
2 -
Joined
-
Last visited
Posts posted by michael222
-
-
<?php // Database connection details $servername = "localhost"; $username = "root"; $password = ""; $dbname = "invoice"; // Create connection $conn = new mysqli($servername, $username, $password, $dbname); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } // Check if the form is submitted if ($_SERVER["REQUEST_METHOD"] === "POST") { // Sanitize and retrieve form data $grNumber = isset($_POST["gr_number"]) ? intval($_POST["gr_number"]) : 0; $libraryFee = isset($_POST["library_fee"]) ? floatval($_POST["library_fee"]) : 0.0; $beadsMakingFee = isset($_POST["beads_making_fee"]) ? floatval($_POST["beads_making_fee"]) : 0.0; $tuitionFee = isset($_POST["tuition_fee"]) ? floatval($_POST["tuition_fee"]) : 0.0; $boardingFee = isset($_POST["boarding_fee"]) ? floatval($_POST["boarding_fee"]) : 0.0; $maintenanceFee = isset($_POST["maintenance_fee"]) ? floatval($_POST["maintenance_fee"]) : 0.0; $computerFee = isset($_POST["computer_fee"]) ? floatval($_POST["computer_fee"]) : 0.0; $sportsFee = isset($_POST["sports_fee"]) ? floatval($_POST["sports_fee"]) : 0.0; $feedingArrears = isset($_POST["feeding_arrears"]) ? floatval($_POST["feeding_arrears"]) : 0.0; $transportationArrears = isset($_POST["transportation_arrears"]) ? floatval($_POST["transportation_arrears"]) : 0.0; $lastTermArrears = isset($_POST["last_term_arrears"]) ? floatval($_POST["last_term_arrears"]) : 0.0; $totalAmount = isset($_POST["total_amount"]) ? floatval($_POST["total_amount"]) : 0.0; $date=$_POST['date']; $date=date('Y-m-d',strtotime($date)); $status = isset($_POST["status"]) ? $_POST["status"] : ''; // Calculate total amount $paidAmount = $libraryFee + $beadsMakingFee + $tuitionFee + $boardingFee + $maintenanceFee + $computerFee + $sportsFee + $feedingArrears + $transportationArrears; // Prepare and execute the SQL query $insertQuery = $conn->prepare("INSERT INTO fees (gr_number, library_fee, beads_making_fee, tuition_fee, boarding_fee, maintenance_fee, computer_fee, sports_fee, feeding_arrears, transportation_arrears, last_term_arrears, total_amount, paid_amount, date, status) VALUES (?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ?)"); $insertQuery->bind_param("iddddddddddddds", $grNumber, $libraryFee, $beadsMakingFee, $tuitionFee, $boardingFee, $maintenanceFee, $computerFee, $sportsFee, $feedingArrears, $transportationArrears, $lastTermArrears, $totalAmount, $paidAmount, $date, $status); if ($insertQuery->execute()) { // Data inserted successfully $insertQuery->close(); header("Location: schoolfees.php"); exit(); // Make sure to exit after a header redirect } else { // Log the error and provide a user-friendly message die("Error inserting data: " . $conn->error); } } // Retrieve existing fees data from the database $selectQuery = "SELECT * FROM fees"; $result = $conn->query($selectQuery); ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>GTUC Job Board - Dashboard</title> <meta name="description" content="Online Job Management / Job Portal" /> <meta name="keywords" content="job, work, resume, applicants, application, employee, employer, hire, hiring, human resource management, hr, online job management, company, worker, career, recruiting, recruitment" /> <meta name="author" content="BwireSoft"> <meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1"> <meta property="og:image" content="http://your_actual_link/images/banner.jpg" /> <meta property="og:image:secure_url" content="https://your_actual_link/images/banner.jpg" /> <meta property="og:image:type" content="image/jpeg" /> <meta property="og:image:width" content="500" /> <meta property="og:image:height" content="300" /> <meta property="og:image:alt" content="Bwire Jobs"> <link rel="shortcut icon" href="../images/ico/favicon.png"> <link rel="stylesheet" type="text/css" href="../bootstrap/css/bootstrap.min.css" media="screen"> <link href="../css/animate.css" rel="stylesheet"> <link href="../css/main.css" rel="stylesheet"> <link href="../css/component.css" rel="stylesheet"> <link rel="stylesheet" href="../icons/linearicons/style.css"> <link rel="stylesheet" href="../icons/font-awesome/css/font-awesome.min.css"> <link rel="stylesheet" href="../icons/simple-line-icons/css/simple-line-icons.css"> <link rel="stylesheet" href="../icons/ionicons/css/ionicons.css"> <link rel="stylesheet" href="../icons/pe-icon-7-stroke/css/pe-icon-7-stroke.css"> <link rel="stylesheet" href="../icons/rivolicons/style.css"> <link rel="stylesheet" href="../icons/flaticon-line-icon-set/flaticon-line-icon-set.css"> <link rel="stylesheet" href="../icons/flaticon-streamline-outline/flaticon-streamline-outline.css"> <link rel="stylesheet" href="../icons/flaticon-thick-icons/flaticon-thick.css"> <link rel="stylesheet" href="../icons/flaticon-ventures/flaticon-ventures.css"> <link href="../css/style.css" rel="stylesheet"> </head> <style> .autofit2 { height: 80px; width: 100px; object-fit: cover; } .statistic-box { background-color: #fff; padding: 20px; margin-bottom: 20px; text-align: center; border-radius: 8px; box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1); } .statistic-box i { font-size: 48px; color: #002e69; } .counter-number { font-size: 24px; font-weight: bold; color: #333; margin-top: 10px; } .statistic-box h5 { font-size: 18px; margin-top: 10px; color: #666; } .statistic-box a { display: block; margin-top: 15px; color: #002e69; text-decoration: none; } /* Responsive Styles */ @media (max-width: 768px) { .statistic-box { padding: 15px; } .statistic-box i { font-size: 36px; } .counter-number { font-size: 18px; } .statistic-box h5 { font-size: 16px; } } /* Add your custom table styles here */ table { width: 100%; border-collapse: collapse; margin-top: 20px; } th, td { border: 1px solid #ddd; padding: 8px; text-align: left; } th { background-color: #4CAF50; color: white; } tr:hover { background-color: #f5f5f5; } /* Add your custom button styles here */ button { background-color: #4CAF50; color: #fff; padding: 10px 15px; border: none; border-radius: 4px; cursor: pointer; } button:hover { background-color: #45a049; } .action-buttons button { margin-right: 10px; /* Adjust the margin as needed */ } .add-fees-form { max-width: 800px; margin: 0 auto; background-color: #fff; padding: 20px; border-radius: 8px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); } .add-fees-form label { display: block; margin-bottom: 5px; font-weight: bold; color: #000000; } .add-fees-form input, .add-fees-form select, .add-fees-form textarea { width: 100%; padding: 8px; margin-bottom: 10px; box-sizing: border-box; border: 1px solid #ccc; border-radius: 4px; font-size: 14px; } .add-fees-form textarea { resize: vertical; } .add-fees-form button { background-color: #4CAF50; color: #fff; padding: 10px 15px; border: none; border-radius: 4px; cursor: pointer; font-size: 16px; } .add-fees-form button:hover { background-color: #45a049; } /* Responsive Styles */ @media (max-width: 768px) { .add-fees-form { width: 100%; } .add-fees-form div { width: 100%; } } /* Style for Edit Student Modal */ #editTeacherModal { color: #000; } #editTeacherModal .modal-content { background-color: #fff; border-radius: 10px; } #editTeacherModal .modal-header { background-color: #4CAF50; color: #fff; border-radius: 10px 10px 0 0; } #editTeacherModal .modal-body { padding: 20px; } #editTeacherModal label { font-weight: bold; color: #000; } #editTeacherModal input, #editTeacherModal select, #editTeacherModal textarea { width: 80%; padding: 8px; margin-bottom: 10px; box-sizing: border-box; border: 1px solid #ccc; border-radius: 4px; font-size: 14px; } #editTeacherModal button { background-color: #4CAF50; color: #fff; padding: 10px 15px; border: none; border-radius: 4px; cursor: pointer; font-size: 16px; } #editTeacherModal button:hover { background-color: #45a049; } /* Style for View Student Modal */ #viewTeacherModal { color: #000; } #viewTeacherModal .modal-content { background-color: #fff; border-radius: 10px; } #viewTeacherModal .modal-header { background-color: #4CAF50; color: #fff; border-radius: 10px 10px 0 0; } #viewTeacherModal .modal-body { padding: 20px; } #viewTeacherModal label { font-weight: bold; color: #000; } #viewTeacherModal p { font-size: 14px; color: #333; } #viewTeacherModal button { background-color: #4CAF50; color: #fff; padding: 10px 15px; border: none; border-radius: 4px; cursor: pointer; font-size: 16px; } #viewTeacherModal button:hover { background-color: #45a049; } </style> <body class="not-transparent-header"> <div class="container-wrapper"> <header id="header"> <nav class="navbar navbar-default navbar-fixed-top navbar-sticky-function"> <div class="container"> <div class="logo-wrapper"> <div class="logo"> <a href="../"><img src="../images/logo.png" alt="Logo" /></a> </div> </div> <div id="navbar" class="navbar-nav-wrapper navbar-arrow"> <ul class="nav navbar-nav" id="responsive-menu"> <li><a href=""></a></li> </ul> </div> <div class="nav-mini-wrapper"> <ul class="nav-mini sign-in"> <li><a href="logout.php">logout</a></li> <li><a href="my_account.php">Profile</a></li> </ul> </div> </div> <div id="slicknav-mobile"></div> </nav> </header> <div class="main-wrapper"> <div class="breadcrumb-wrapper"> <div class="container"> <ol class="breadcrumb-list booking-step"> <li><a href="../">Home</a></li> <li><span>Class</span></li> </ol> </div> </div> <div class="admin-container-wrapper"> <div class="container"> <div class="GridLex-gap-15-wrappper"> <div class="GridLex-grid-noGutter-equalHeight"> <div class="GridLex-col-3_sm-4_xs-12"> <div class="admin-sidebar"> <div class="admin-user-item"> <div class="image"> <center> <!-- Profile Image --> <img class='img-circle autofit2' src='../images/default.jpg' title='' alt='image' /> </center> </div> <br> <h4>Username</h4> <p class="user-role"></p> </div> <div class="admin-user-action text-center"> <a href="my_account.php" class=" fa fa-user btn btn-primary btn-sm btn-inverse ">View My Account</a> </div> <ul class="admin-user-menu clearfix"> <li> <a href="dashboard.php"><i class="fa fa-tachometer"></i>Dashboard</a> </li> <li > <a href="class.php"><i class="fa fa-building"></i> Class</a> </li> <li> <a href="term.php"><i class="fa fa-building"></i> Term</a> </li> <li > <a href="students.php"><i class="fa fa-building"></i> Students</a> </li> <li > <a href="teachers.php"><i class="fa fa-users"></i> Teachers</a> </li> <li class="active"> <a href="schoolfees.php"><i class="fa fa-folder-open"></i> School Fees</a> </li> <li> <a href="salary.php"><i class="fa fa-folder-open"></i> Salary</a> </li> <li> <a href="change-password.php"><i class="fa fa-key"></i> Change Password</a> </li> <li> <a href="logout.php"><i class="fa fa-sign-out"></i> Logout</a> </li> </ul> </div> </div> <div class="GridLex-col-9_sm-8_xs-12"> <div class="admin-content-wrapper"> <div class="admin-section-title" style="display: flex; justify-content: space-between; align-items: center;"> <h3>Fees</h3> <p style="margin-left: auto;">Today's date: <span class="text-primary">2023-12-29</span></p> </div> <!-- Form to add teachers --> <form class="add-fees-form" method="post" action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]); ?>"> <div style="display: flex; justify-content: space-between; gap: 10px;"> <!-- First Column of Form Fields --> <div style="width: 30%;"> <label for="gr_number">GR Number:</label> <input type="text" name="gr_number" required> <label for="library_fee">Library Fee:</label> <input type="number" name="library_fee" required> <label for="beads_making_fee">Beads Making Fee:</label> <input type="number" name="beads_making_fee" required> </div> <!-- Second Column of Form Fields --> <div style="width: 30%;"> <label for="tuition_fee">Tuition Fee:</label> <input type="number" name="tuition_fee" required> <label for="boarding_fee">Boarding Fee:</label> <input type="number" name="boarding_fee" required> <label for="maintenance_fee">Maintenance Fee:</label> <input type="number" name="maintenance_fee" required> </div> <!-- Third Column of Form Fields --> <div style="width: 30%;"> <label for="computer_fee">Computer Fee:</label> <input type="number" name="computer_fee" required> <label for="sports_fee">Sports Fee:</label> <input type="number" name="sports_fee" required> <label for="feeding_arrears">Feeding Arrears:</label> <input type="number" name="feeding_arrears" required> </div> </div> <!-- Additional Row for Transportation and Last Term Arrears --> <div style="display: flex; justify-content: space-between; gap: 10px; margin-top: 10px;"> <!-- Fourth Column of Form Fields --> <div style="width: 30%;"> <label for="transportation_arrears">Transportation Arrears:</label> <input type="number" name="transportation_arrears" required> </div> <!-- Fifth Column of Form Fields --> <div style="width: 30%;"> <label for="last_term_arrears">Last Term Arrears:</label> <input type="number" name="last_term_arrears" required> </div> </div> <!-- Total Amount, Date Paid, and Status Fields --> <div style="display: flex; justify-content: space-between; gap: 10px; margin-top: 10px;"> <!-- Sixth Column of Form Fields --> <div style="width: 30%;"> <label for="total_amount">Total Amount:</label> <input type="number" name="total_amount" required> </div> <!-- Seventh Column of Form Fields --> <div style="width: 30%;"> <label for="date_paid">Date Paid:</label> <input type="date" name="date"> </div> <!-- Eighth Column of Form Fields --> <div style="width: 30%;"> <label for="status">Status:</label> <select name="status" required> <option value="paid">Paid</option> <option value="part">Part</option> <option value="unpaid">Unpaid</option> </select> </div> </div> <!-- Submit Button --> <button type="submit">Add Fees</button> </form> <br> <div class="row"> <div class="col-md-12"> <div class="table-responsive"> <table class="table table-bordered table-responsive"> <thead> <tr> <th>GR Number</th> <th>Library Fee</th> <th>Beads Making Fee</th> <th>Tuition Fee</th> <th>Boarding Fee</th> <th>Maintenance Fee</th> <th>Computer Fee</th> <th>Sports Fee</th> <th>Feeding Arrears</th> <th>Transportation Arrears</th> <th>Last Term Arrears</th> <th>Total Amount</th> <th>Date Paid</th> <th>Balance</th> <th>Status</th> <th>Action</th> </tr> </thead> <tbody> <?php while ($row = $result->fetch_assoc()) { echo "<tr>"; echo "<td>" . $row["gr_number"] . "</td>"; echo "<td>" . $row["library_fee"] . "</td>"; echo "<td>" . $row["beads_making_fee"] . "</td>"; echo "<td>" . $row["tuition_fee"] . "</td>"; echo "<td>" . $row["boarding_fee"] . "</td>"; echo "<td>" . $row["maintenance_fee"] . "</td>"; echo "<td>" . $row["computer_fee"] . "</td>"; echo "<td>" . $row["sports_fee"] . "</td>"; echo "<td>" . $row["feeding_arrears"] . "</td>"; echo "<td>" . $row["transportation_arrears"] . "</td>"; echo "<td>" . $row["last_term_arrears"] . "</td>"; echo "<td>" . $row["total_amount"] . "</td>"; echo "<td>" . $row["date"] . "</td>"; $balance = $row["total_amount"] + $row["last_term_arrears"] - $row["paid_amount"]; echo "<td>" . $balance . "</td>"; echo "<td>"; $totalDue = $row["total_amount"] + $row["last_term_arrears"]; if ($totalDue == $row["paid_amount"]) { echo "Paid"; } elseif ($totalDue > $row["paid_amount"] && $row["paid_amount"] != 0) { echo "Part Payment"; } elseif ($totalDue > $row["paid_amount"] && $row["paid_amount"] == 0) { echo "Unpaid"; } else { // Handle any other cases or leave it empty echo "Unknown"; } echo "</td>"; // Merge Action column echo "<td>"; echo "<button onclick='viewFees(\"" . $row["gr_number"] . "\", \"" . $row["library_fee"] . "\", \"" . $row["beads_making_fee"] . "\", \"" . $row["tuition_fee"] . "\", \"" . $row["boarding_fee"] . "\", \"" . $row["maintenance_fee"] . "\", \"" . $row["computer_fee"] . "\", \"" . $row["sports_fee"] . "\", \"" . $row["feeding_arrears"] . "\", \"" . $row["transportation_arrears"] . "\", \"" . $row["last_term_arrears"] . "\", \"" . $row["total_amount"] . "\", \"" . $row["date"] . "\", \"" . $row["status"] . "\")'>View</button>"; echo "<button onclick='editFees(\"" . $row["gr_number"] . "\", \"" . $row["library_fee"] . "\", \"" . $row["beads_making_fee"] . "\", \"" . $row["tuition_fee"] . "\", \"" . $row["boarding_fee"] . "\", \"" . $row["maintenance_fee"] . "\", \"" . $row["computer_fee"] . "\", \"" . $row["sports_fee"] . "\", \"" . $row["feeding_arrears"] . "\", \"" . $row["transportation_arrears"] . "\", \"" . $row["last_term_arrears"] . "\", \"" . $row["total_amount"] . "\", \"" . $row["date"] . "\", \"" . $row["status"] . "\")'>Edit</button>"; echo "<form style='display:inline;' method='post' action='delete_fees.php'>"; echo "<input type='hidden' name='fees_id' value='" . $row["gr_number"] . "'>"; echo "<button type='submit' class='btn btn-danger'>Delete</button>"; echo "</form>"; echo "</td>"; echo "</tr>"; } ?> </tbody> </table> </div> </div> </div> </div> </div> </div> </div> </div> </div> </div> </div> <div id="back-to-top"> <a href="#"><i class="ion-ios-arrow-up"></i></a> </div> <!-- Edit Fees Modal --> <div class="modal fade" id="editFeesModal" tabindex="-1" role="dialog" aria-labelledby="editFeesModalLabel" aria-hidden="true"> <div class="modal-dialog modal-dialog-centered" role="document"> <div class="modal-content"> <div class="modal-header"> <h5 class="modal-title" id="editFeesModalLabel">Edit Fees Details</h5> <button type="button" class="close" data-dismiss="modal" aria-label="Close"> <span aria-hidden="true">×</span> </button> </div> <div class="modal-body"> <!-- Your form for editing fees --> <form class="edit-fees-form" method="post" action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]); ?>" id="editFeesForm"> <!-- Fields for editing fees --> <div class="mb-3"> <label for="editGrNumber" class="form-label">GR Number:</label> <input type="text" class="form-control" id="editGrNumber" name="editGrNumber" required> </div> <div class="mb-3"> <label for="editLibraryFee" class="form-label">Library Fee:</label> <input type="number" class="form-control" id="editLibraryFee" name="editLibraryFee" required> </div> <div class="mb-3"> <label for="editBeadsMakingFee" class="form-label">Beads Making Fee:</label> <input type="number" class="form-control" id="editBeadsMakingFee" name="editBeadsMakingFee" required> </div> <div class="mb-3"> <label for="editTuitionFee" class="form-label">Tuition Fee:</label> <input type="number" class="form-control" id="editTuitionFee" name="editTuitionFee" required> </div> <div class="mb-3"> <label for="editBoardingFee" class="form-label">Boarding Fee:</label> <input type="number" class="form-control" id="editBoardingFee" name="editBoardingFee" required> </div> <div class="mb-3"> <label for="editMaintenanceFee" class="form-label">Maintenance Fee:</label> <input type="number" class="form-control" id="editMaintenanceFee" name="editMaintenanceFee" required> </div> <div class="mb-3"> <label for="editComputerFee" class="form-label">Computer Fee:</label> <input type="number" class="form-control" id="editComputerFee" name="editComputerFee" required> </div> <div class="mb-3"> <label for="editSportsFee" class="form-label">Sports Fee:</label> <input type="number" class="form-control" id="editSportsFee" name="editSportsFee" required> </div> <div class="mb-3"> <label for="editFeedingArrears" class="form-label">Feeding Arrears:</label> <input type="number" class="form-control" id="editFeedingArrears" name="editFeedingArrears" required> </div> <div class="mb-3"> <label for="editTransportationArrears" class="form-label">Transportation Arrears:</label> <input type="number" class="form-control" id="editTransportationArrears" name="editTransportationArrears" required> </div> <div class="mb-3"> <label for="editLastTermArrears" class="form-label">Last Term Arrears:</label> <input type="number" class="form-control" id="editLastTermArrears" name="editLastTermArrears" required> </div> <div class="mb-3"> <label for="editTotalAmount" class="form-label">Total Amount:</label> <input type="number" class="form-control" id="editTotalAmount" name="editTotalAmount" required> </div> <div class="mb-3"> <label for="editDatePaid" class="form-label">Date Paid:</label> <input type="date" class="form-control" id="editDatePaid" name="editDatePaid"> </div> <div class="mb-3"> <label for="editStatus" class="form-label">Status:</label> <select class="form-select" id="editStatus" name="editStatus" required> <option value="paid">Paid</option> <option value="part">Part</option> <option value="unpaid">Unpaid</option> </select> </div> <!-- Add other form fields as needed --> <!-- ... --> </form> </div> <div class="modal-footer"> <button type="button" class="btn btn-primary" onclick="submitEditFeesForm()">Save Changes</button> <button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button> </div> </div> </div> </div> <!-- JavaScript to handle Edit Fees Modal --> <script> function openEditFeesModal(grNumber, libraryFee, beadsMakingFee, tuitionFee, boardingFee, maintenanceFee, computerFee, sportsFee, feedingArrears, transportationArrears, lastTermArrears, totalAmount, datePaid, status) { // Set values in the modal fields document.getElementById('editGrNumber').value = grNumber; document.getElementById('editLibraryFee').value = libraryFee; document.getElementById('editBeadsMakingFee').value = beadsMakingFee; document.getElementById('editTuitionFee').value = tuitionFee; document.getElementById('editBoardingFee').value = boardingFee; document.getElementById('editMaintenanceFee').value = maintenanceFee; document.getElementById('editComputerFee').value = computerFee; document.getElementById('editSportsFee').value = sportsFee; document.getElementById('editFeedingArrears').value = feedingArrears; document.getElementById('editTransportationArrears').value = transportationArrears; document.getElementById('editLastTermArrears').value = lastTermArrears; document.getElementById('editTotalAmount').value = totalAmount; document.getElementById('editDatePaid').value = datePaid; document.getElementById('editStatus').value = status; // Show the Edit Fees modal $('#editFeesModal').modal('show'); } function submitEditFeesForm() { // Add your logic to submit the form using AJAX or other methods // For now, let's just close the modal $('#editFeesModal').modal('hide'); } </script> <script type="text/javascript" src="../js/jquery-1.11.3.min.js"></script> <script type="text/javascript" src="../js/jquery-migrate-1.2.1.min.js"></script> <script type="text/javascript" src="../bootstrap/js/bootstrap.min.js"></script> <script type="text/javascript" src="../js/bootstrap-modalmanager.js"></script> <script type="text/javascript" src="../js/bootstrap-modal.js"></script> <script type="text/javascript" src="../js/smoothscroll.js"></script> <script type="text/javascript" src="../js/jquery.easing.1.3.js"></script> <script type="text/javascript" src="../js/jquery.waypoints.min.js"></script> <script type="text/javascript" src="../js/wow.min.js"></script> <script type="text/javascript" src="../js/jquery.counterup.min.js"></script> <script type="text/javascript" src="../js/jquery.validate.min.js"></script> <script type="text/javascript" src="../js/jquery.stellar.min.js"></script> <script type="text/javascript" src="../js/jquery.magnific-popup.min.js"></script> <script type="text/javascript" src="../js/owl.carousel.min.js"></script> <script type="text/javascript" src="../js/wow.min.js"></script> <script type="text/javascript" src="../js/masonry.pkgd.min.js"></script> <script type="text/javascript" src="../js/jquery.filterizr.min.js"></script> <script type="text/javascript" src="../js/smoothscroll.js"></script> <script type="text/javascript" src="../js/jquery.countdown.min.js"></script> <script type="text/javascript" src="../js/script.js"></script> <script type="text/javascript" src="../js/handlebars.min.js"></script> <script type="text/javascript" src="../js/jquery.countimator.js"></script> <script type="text/javascript" src="../js/jquery.countimator.wheel.js"></script> <script type="text/javascript" src="../js/slick.min.js"></script> <script type="text/javascript" src="../js/easy-ticker.js"></script> <script type="text/javascript" src="../js/jquery.introLoader.min.js"></script> <script type="text/javascript" src="../js/jquery.responsivegrid.js"></script> <script type="text/javascript" src="../js/customs.js"></script> <script src="https://code.jquery.com/jquery-3.5.1.slim.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/@popperjs/core@2.11.6/dist/umd/popper.min.js"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script> <?php // Close the database connection $conn->close(); ?> </body> </html>
hi there im getting 0000-00-00 date value in database and html table i need helpi have tried all means
in PHP Coding Help
Posted
Thank You Sir it works Now