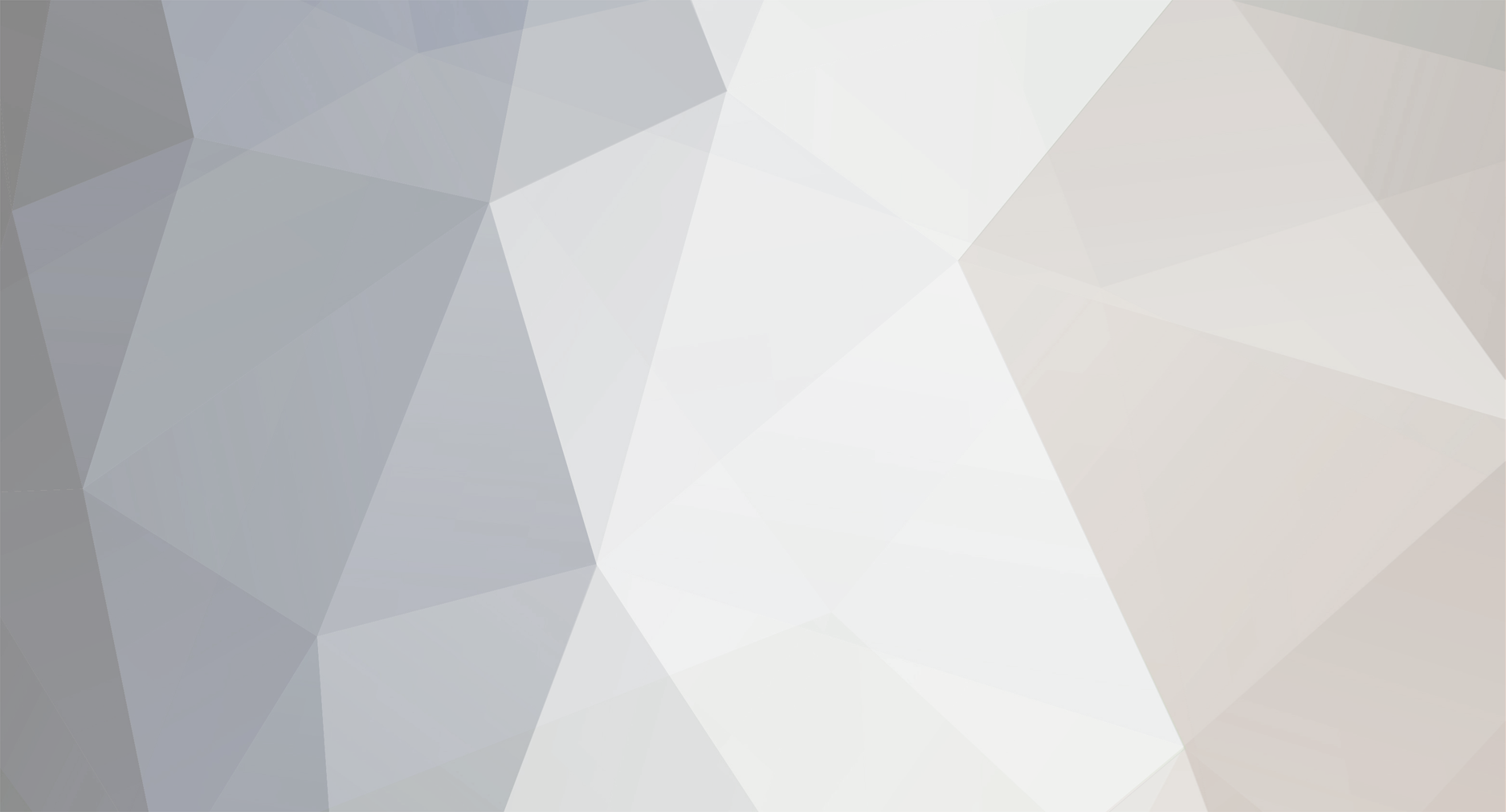
Danishhafeez
-
Posts
41 -
Joined
-
Last visited
-
Days Won
2
Community Answers
-
Danishhafeez's post in How do I change the order of DIVS on small devices? was marked as the answer
To achieve the desired layout where the image and text columns switch order on smaller screens, you can use CSS Flexbox with media queries. The key is to set the flex direction to column and change the order of the elements within the media query.
<div id="flex"> <div id="left"> <img src="image.jpg" alt="Image"> </div> <div id="right"> <p>Your text goes here.</p> </div> </div> Default Styles for Larger Screens:
By default, the elements will be displayed side by side (e.g., using Flexbox or Grid).
#flex { display: flex; } #left, #right { flex: 1; /* or you can use a specific width like flex: 0 0 50%; */ } #left { order: 1; } #right { order: 2; } Use a media query to change the flex direction to column and adjust the order of the elements.
@media only screen and (max-width: 950px) { #flex { display: flex; flex-direction: column; } #left { order: 2; } #right { order: 1; } } display: flex;: Ensures that the parent container uses Flexbox.
flex-direction: column;: Stacks the child elements vertically instead of horizontally.
order: Adjusts the order of elements. Lower values appear first. In the media query, setting #left to order: 2 and #right to order: 1 swaps their positions.
here is complete example:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Responsive Layout</title> <style> /* Default Styles for Larger Screens */ #flex { display: flex; } #left, #right { flex: 1; } #left { order: 1; } #right { order: 2; } /* Styles for Smaller Screens */ @media only screen and (max-width: 950px) { #flex { display: flex; flex-direction: column; } #left { order: 2; } #right { order: 1; } } </style> </head> <body> <div id="flex"> <div id="left"> <img src="image.jpg" alt="Image" style="width: 100%;"> </div> <div id="right"> <p>Your text goes here.</p> </div> </div> </body> </html>
Best Regard
Danish Hafeez | QA Assistant
ICTInnovations
-
Danishhafeez's post in print the output inside a function/method... was marked as the answer
Here’s how you can achieve that:
Separate Logic from Output: Move the output logic outside the core function.
Use Dependency Injection: Inject a callable (e.g., a function or a method) that handles the output. This allows you to control the output during testing.
<?php function copyFileExample($outputCallback = null) { $verboseText = "Copying file... please wait...\n"; if ($outputCallback) { call_user_func($outputCallback, $verboseText); } // Simulate the file copy with sleep(5) sleep(5); // replaces copy() function return $verboseText; } class FileTest extends PHPUnit\Framework\TestCase { static function copyProvider() { return [ [ "outputCallback" => null // recommended [HIGHLIGHT] ], [ "outputCallback" => function($text) { echo $text; } // not recommended for testing [HIGHLIGHT] ], ]; } /** * @dataProvider copyProvider */ function testCopy($outputCallback) { $expectedOutput = "Copying file... please wait...\n"; ob_start(); $result = copyFileExample($outputCallback); $output = ob_get_clean(); if ($outputCallback) { $this->assertEquals($expectedOutput, $output); } else { $this->assertEquals($expectedOutput, $result); } } } ?> Function Definition:
The copyFileExample function now accepts an optional $outputCallback parameter, which can be any callable (function, method, etc.).
If $outputCallback is provided, it is called with the verbose text. This decouples the printing logic from the core function.
Test Class:
The copyProvider method returns different scenarios, including one without any callback and one with a callback that echoes the text.
The testCopy method captures any output printed by the callback using output buffering (ob_start, ob_get_clean).
The assertions check the output if a callback is provided and the return value otherwise.
$ phpunit FileTest.php PHPUnit 10.5.20 by Sebastian Bergmann and contributors. Runtime: PHP 8.1.2-1ubuntu2.17 .. OK (2 tests, 2 assertions) With this approach, both tests should pass, ensuring that the function behaves correctly with and without output, and making it easier to test and maintain.
Best Regard
Danish hafeez | QA Assistant
ICTInnovations
-
Danishhafeez's post in Repeat a drop down list on each row of a HTML table was marked as the answer
To ensure the dropdown appears in the correct position within each row, you need to place the <select> element inside the loop that iterates over your $details array. The issue arises because the <select> element is currently being added outside the loop, which causes it to appear only once at the end of the table. Additionally, the form tags should also be inside the loop to ensure each row has its own form, dropdown, and submit button.
<?php // Connection details section.. $email = current_user(); $statement = db()->prepare("EXEC fe_uspConnectionDetails ?;"); $statement->bindParam(1, $email, PDO::PARAM_STR); $statement->execute(); $details = $statement->fetchAll(PDO::FETCH_ASSOC); // Fetch agencies for dropdown $statement = db()->prepare("EXEC fe_uspAdminUserShowRegisteredAgencies"); $statement->execute(); $agencies = $statement->fetchAll(PDO::FETCH_ASSOC); ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> </head> <body> <p> <table> <thead> <tr> <th><i>name</i></th> <th><i>email</i></th> <th><i>skill</i></th> <th><i>mobile</i></th> <th><i>region</i></th> <th><i>days</i></th> <th><i><center>refer to</center></i></th> </tr> </thead> <tbody> <?php if ($details) { foreach ($details as $detail) { echo "<tr>"; echo "<td><left>" . $detail['fullname'] . "</td>"; echo "<td><left>" . $detail['connectionemail'] . "</td>"; echo "<td><left>" . $detail['skill'] . "</td>"; echo "<td><left>" . $detail['phonenumber'] . "</td>"; echo "<td><left>" . $detail['region'] . "</td>"; echo "<td><left>" . $detail['daysremaining'] . "</td>"; echo "<td><left>"; echo "<form action='LogReferal.php?email=referto' method='POST'>"; echo "<input type='hidden' name='referred' value='" . $detail['connectionemail'] . "'>"; echo "<select name='referto' id='referto'>"; foreach ($agencies as $agencylist) { echo "<option value='" . $agencylist['email'] . "'>" . $agencylist['email'] . "</option>"; } echo "</select>"; echo "<input type='submit' value='refer'>"; echo "</form>"; echo "</td>"; echo "</tr>"; } } ?> </tbody> </table> </body> </html> Data Fetching: First, fetch both the connection details and the agency list.
HTML Structure: The HTML structure now includes the <select> dropdown within each row of the table.
Loop and Form: The loop iterates over each $detail and adds a row in the table. The form, including the hidden input for the referred value and the dropdown for referto, is inside this loop. This ensures each row has its own form and dropdown, associated correctly with the row's data.
Form Method: Ensure the form uses the POST method to securely send data.
Best Regard
Danish Hafeez | QA Assistant
ICTInnovations
-
Danishhafeez's post in Issue with passing Array Data from Loop1 to Loop2 was marked as the answer
It seems like you're trying to dynamically retrieve column names from the first loop and then use them to fetch corresponding data from the database in the second loop. However, you're facing issues with the data retrieval part. Let's correct the code:
fputcsv($csvfile, $export_column_name_array, $delimiter); $stmt2 = $conn->prepare($sql_built_statement); //$stmt2->bind_param("ii", $company_id, $company_id); WILL ADD THIS LATER ONCE WORKING $stmt2->execute(); $result2 = $stmt2->get_result(); if($result2 != NULL){ // Retrieve data while($row2=$result2->fetch_assoc()){ // Initialize an empty data array for each row $data_array = array(); // Add data for each column foreach ($export_column_name_array as $value) { // Push data for each column into the data array $data_array[] = $row2[$value]; } // Write the data array to the CSV file fputcsv($csvfile, $data_array, $delimiter); } } fclose($csvfile); In this corrected code:
We loop through each row of the fetched data and initialize an empty $data_array for each row.
Within the row loop, we iterate through each column name from $export_column_name_array.
For each column name, we fetch the corresponding data from the $row2 associative array and add it to the $data_array.
After populating the $data_array with data for all columns, we write it to the CSV file using fputcsv().
The process repeats for each row fetched from the database until all rows have been processed.
i hope This should correctly populate your CSV file with the data fetched from the database, using the dynamic column names obtained from $export_column_name_array.
Best Regard
Danish hafeez | QA Assistant
ICTInnovations