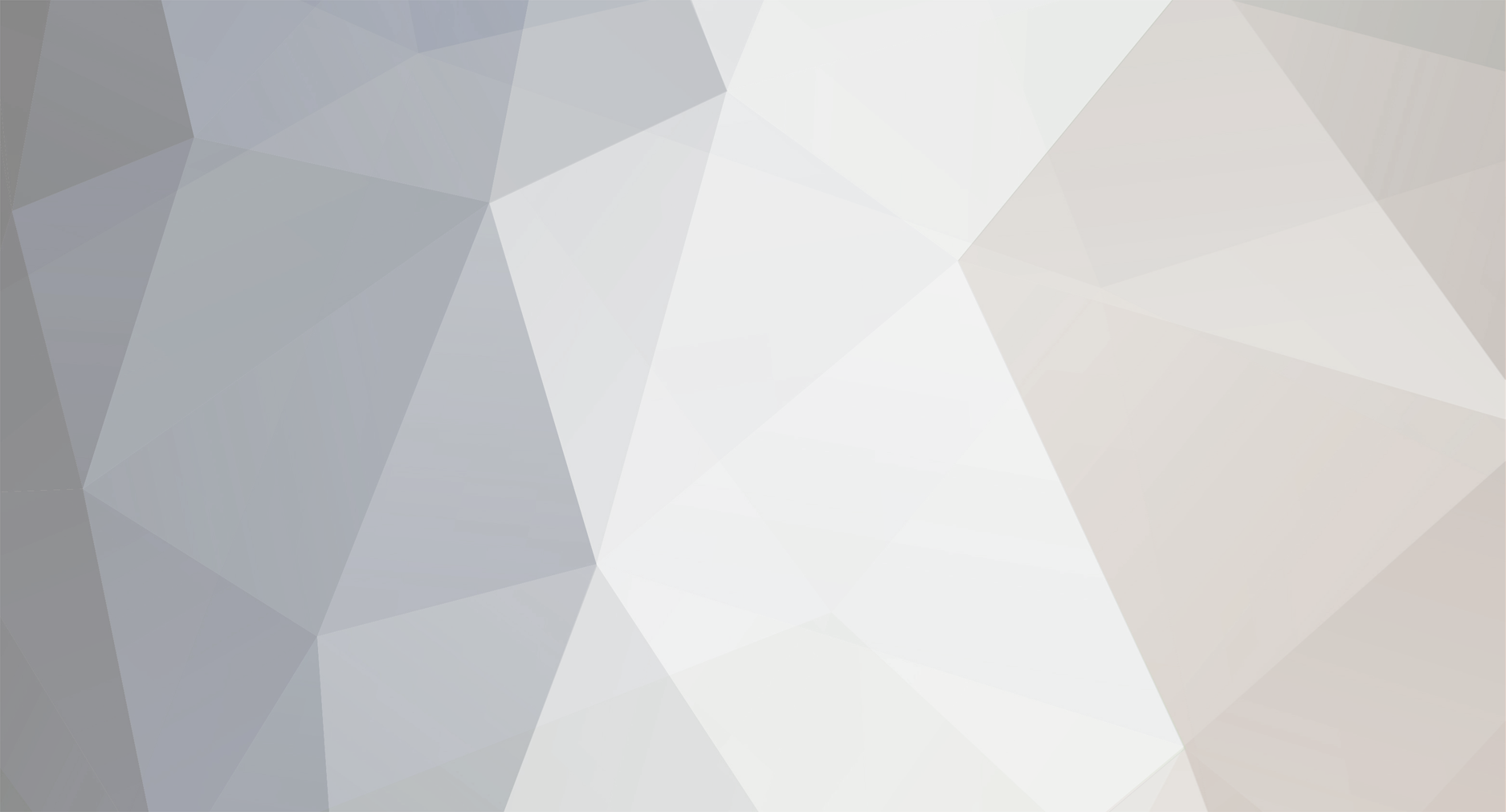
singingsands
Members-
Posts
15 -
Joined
-
Last visited
Everything posted by singingsands
-
I appreciate your advice about prepared statements, I'll look into it further. I have absolutely no experience with PDO but I'll check it out too. As for my particular problem, I have echoed the variables to make sure they are correct and they are. I've put quotes on the variables and it makes no difference to the problem. And as I mentioned, I've run the query directly in the database using the values of the variables and it works fine.
-
This is a simple query that for some reason that I'm missing is throwing an error. <?php session_start(); if (!isset($_SESSION['Weekend_Number'])) { header("Location: add_team.php"); exit; } $Number=$_SESSION['Weekend_Number']; $MW=$_SESSION['Men_Women']; $query = mysqli_query ($conn, "Select * FROM weekends WHERE Weekend_Number = $Number AND Men_Women = $MW"); ..../ ?> The error I get is Fatal error: Uncaught mysqli_sql_exception: Unknown column '55th' in 'where clause' in C:\xampp\htdocs\cursillo\add_team_details.php:16 Stack trace: #0 C:\xampp\htdocs\cursillo\add_team_details.php(16): mysqli_query(Object(mysqli), 'Select Weekend_...') #1 {main} thrown in C:\xampp\htdocs\cursillo\add_team_details.php on line 16 Apparently the variable $Number is being taken for the column name instead of the field in the column. If I put the same query into the mysql database it works fine. Am I missing something? Note: Weekend_Number is not the ID field.
-
I want to insert (not replace) data into a record that already exists. The user would not know the ID of the record so I have to use a concatenation of 2 fields to get the correct record. This is the sort of thing I want but I know it isn't right. INSERT INTO table ('field5') values('value1') WHERE ('field1') == 'value2' && ('field2')=='value3'; What's the right way to do this?
-
I adapted it from a login script. When I searched for examples of how to use the session variable all that came up was login scripts. Is there another way to do it? The name "register" was a leftover from the original that I missed. The user variable will be something else. Thanks so much, this does the trick. What is the purpose of ENT_QUOTES? I haven't seen that anywhere else. One more question: where is the best place to put session_destroy?
-
This should be simple but it isn't working and I'm so frustrated I could spit. I'm simply trying to pass 2 session variables from one page to another. I should mention this is not for a login. Following is a pared down version of my code. When I enter name and number and submit nothing happens. Any help would do wonders for my sanity. test-sesh.php <?php session_start(); if (isset($_SESSION['name'])) { header("Location: test-sesh-2.php"); exit; } if ($_SERVER['REQUEST_METHOD'] === 'POST') { if (isset($_POST['submit'])) { $name = $_POST['name']; $number = $_POST['number']; } } ?> <!DOCTYPE html> <html> <head> <title>Test $_SESSION</title> </head> <body> <h1>Test $_SESSION</h1> <form method="post"> <label for="name">Name:</label> <input type="text" name="name" required><br> <label for="number">Number:</label> <input type="text" name="number" required><br> <button type="submit" name="register">Submit</button> </form> </body> </html> test-sesh-2.php <?php session_start(); if (!isset($_SESSION['number'])) { header("Location: test-sesh.php"); exit; } $name = $_SESSION['name']; $number = $_SESSION['number']; ?> <!DOCTYPE html> <html> <head> <title>User Dashboard</title> </head> <body> <h1><?php echo $name." ".$number?></h1> </body> </html>
-
Can a submit button trigger 2 actions?
singingsands replied to singingsands's topic in PHP Coding Help
For several reasons I prefer to stay with MySQL for this project. How do I switch the autosuggest code from POD to MySQL? -
Can a submit button trigger 2 actions?
singingsands replied to singingsands's topic in PHP Coding Help
This is the code as I copied it from the post. I changed the names of my table and relevant field names to correspond with this code. <?php require 'includes/db-inc.php'; # # HANDLE AJAX REQUESTS # if (isset($_GET['ajax'])) { if ($_GET['ajax'] == 'names') { $res = $pdo->prepare("SELECT id, concat(fname, ' ', lname) as name FROM person WHERE fname LIKE ? OR lname LIKE ? ORDER BY fname, lname "); $res->execute([ $_GET['search'], $_GET['search'] ]); $results = $res->fetchAll(); exit(json_encode($results)); } } ?> <!DOCTYPE html> <html lang="en"> <head> <title>Example</title> <meta charset="utf-8"> <script src="https://code.jquery.com/jquery-3.6.4.min.js"></script> <style type='text/css'> </style> <script type='text/javascript'> $(function() { $("#search").on("input",function() { let srch = $(this).val() + "%" $.get( "", {"ajax":"names", "search":srch}, function(resp) { $("#person").html("") $.each(resp, function(k,v) { let opt = $("<option>", {"value":v.id, "text":v.name}); $("#person").append(opt); }) }, "JSON" ) }) }) </script> </head> <body> <form> Enter first part of name: <input type='text' id='search' > <br><br> <select name='person_id' id='person' size='20'> <!-- names returned from search go here --> </select> </form> -
Can a submit button trigger 2 actions?
singingsands replied to singingsands's topic in PHP Coding Help
Yes I have the connection code as an include. I added a simple query to the same form to check I'm getting the info <select name="person" class="form-control col-sm-10"> <?php $query = "SELECT id, concat(fname, ' ', lname) as name FROM person ORDER BY fname, lname "; if ($result = $conn->query($query)) { while ($row = $result->fetch_assoc()) { echo "<option value=".$row["id"].">".$row["name"]."</option>"; } } ?> </select> And I do get a drop down list with the first and last name in alphabetical order. But when I check the network tab I get an error: Unchecked runtime.lastError: Could not establish connection. Receiving end does not exist. That makes no sense to me. -
Can a submit button trigger 2 actions?
singingsands replied to singingsands's topic in PHP Coding Help
I'm having trouble with the code above. I changed the names of the database table and fields where appropriate, spent 2 days troubleshooting, finally copied my table to give it and the fields the same name as in the code. All I'm getting is this: Nothing comes up at all. I know the php part is correct but I'm a total noob with ajax so I'm out of my depth here. -
Can a submit button trigger 2 actions?
singingsands replied to singingsands's topic in PHP Coding Help
Barand thanks for the code! I'll try it out. -
Can a submit button trigger 2 actions?
singingsands replied to singingsands's topic in PHP Coding Help
That sounds ideal, I'll check it out. Thanks -
Can a submit button trigger 2 actions?
singingsands replied to singingsands's topic in PHP Coding Help
There are several hundreds of people to draw from, that's a heck of a drop-down list. -
I need to assign people to jobs in projects. There are 3 tables involved: names of people, list of jobs, and the projects they will be on. What I want to be able to do is 1) enter the first and last name of the person 2) search for the person by name 3) return the ID of that person 4) select the job they will be doing and get that ID (I have the dropdown for that already) 5) enter the jobID and personID into the project table. Can this be done in one action or do I need to use 2 different forms?
-
I have a form to submit information about weekend retreats to a database, one of which is location. I have a lookup table for locations so the user can select from a list. The Locations table has a Primary Key called Location_ID and the Weekends table has a Foreign Key called Location_ID_FK. I'm having trouble saving the Location_ID (from a query) to Location_ID_FK. This is the query to select the location: This in itself works, I get the list of locations. But when I try to submit the form I keep getting the message "Warning: Undefined array key "Location_ID"..." I've tried everything I can think of, including changing the name of the field Location_ID_FK to Location_ID, I've also tried putting this line after the 'while' statement. I've also tried adding this same line in the section defining variables to be inserted. I should add that when I use a simple input text for Location the insert query works, and I've triple-checked that I'm using the correct variable in the insert query. What am I missing? Any help is gratefully appreciated.