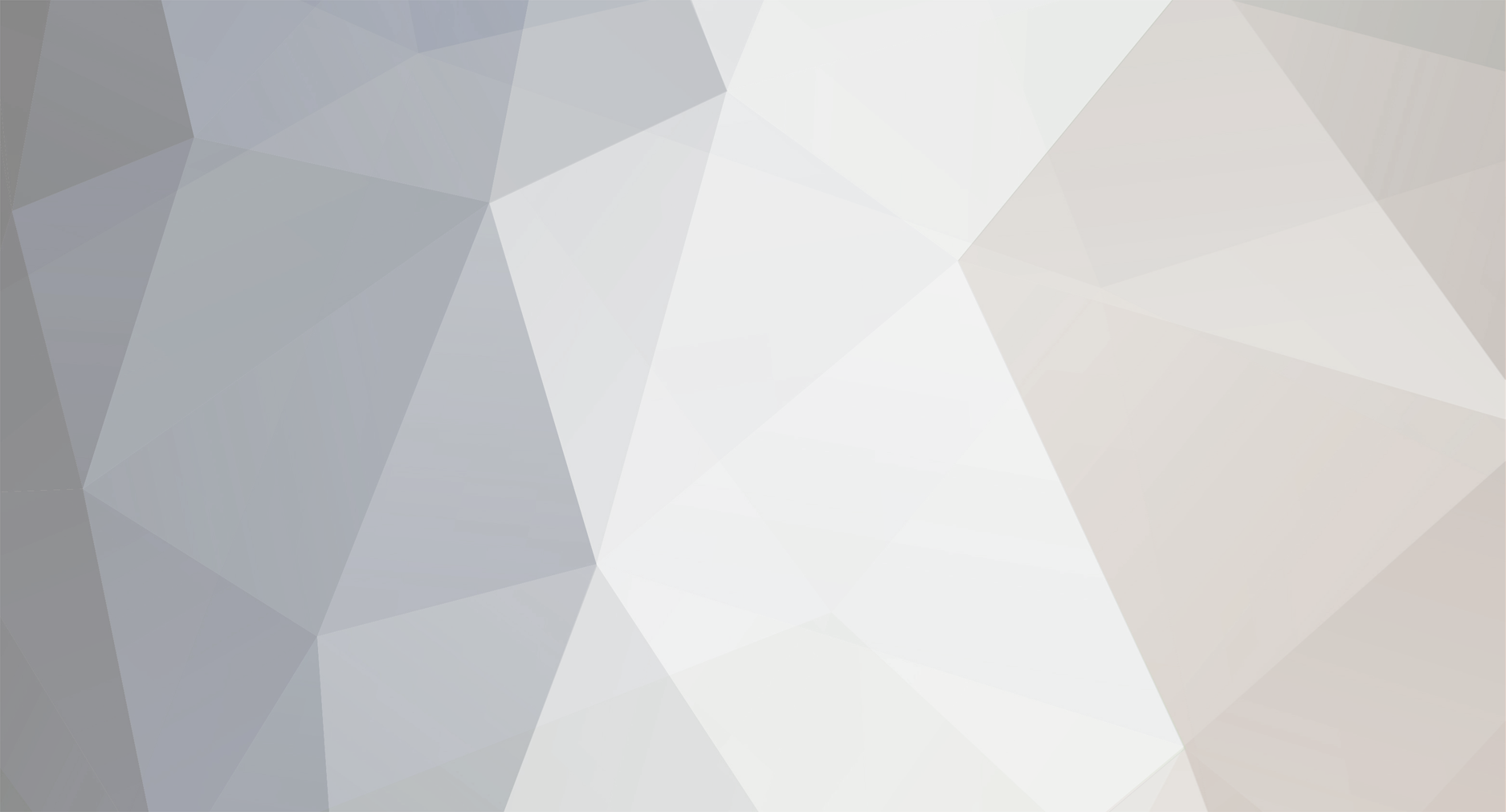
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
"Your line has been disconnected due to increased complaints."
-
Then you need to stop copy-pasting code and actually learn what the code does that you write. Take the code that you already have and learn what each line does. And then try to alter the code slightly and check if it works as expected. Keep repeating until you have mastered PHP.
-
I also wouldn't show the overlap in the middle calendar. I confused the 8 and 9 in july with the one in august. Maybe also increase the number of reps you can compare with. Currently it is set to max. 10 while people who train on strength go for much higher reps. And is this tool meant to log what you did on a day or is this to plan your week/month too? EDIT: I noticed I can't log out nor can I manage my account? Also why don't you ask for gender, bodyweight, waist, fat percentage on registration? You need gender for most calculators like Wilks and Sinclair. The bodyweight is useful so you don't have to ask it on every log entry.
-
Also what is the Volume meant to do? It shows 4800kg, so can I now lift my car? I did 4 sets of 20 push-ups with a weighted vest of 60kg? Also how do I specify when I have no weight? 0x20x4 is kinda a problem
-
The UI is not intuitive. I had no idea how to add a new workout. Also the formatting help returned nothing. Not everything has a weight like push-up or sit-up (which is not equal to your bodyweight) unless you would use a weighted vest.
-
Like scootsah already mentioned. Now you are the only one guarding the security whereas otherwise thousands. I also didn't say you need to go and download the entire Zend framework. I don't even like Zend. I tried Apigility (by Zend on ZF), yet a simple composer update breaks the entire thing (it could not find it's own core tools ZFTool for some reason)... I proposed simple libraries that do ONE thing and do it WELL. The unix philosophy.
-
Add this line to finaladd.php $json = $_POST['output']; var_dump($json, json_decode(stripslashes($json)));And post the output here.
-
Don't reinvent the wheel. It's okay to create your own little framework but be sure to utilize available libraries. Like Doctrine for example as your ORM or DB Abstraction Layer. Something like Silex as a way to utilize a CMS. Build on the shoulders of giants. your far better off this way.
-
What do you call the text that web developers work with?
ignace replied to greenace92's topic in Miscellaneous
I guess you are referring to compiled and interpreted code. Compiled code is translated to CPU instructions where as interpreted code like PHP, HTML, CSS, and JavaScript are translated to instructions in whatever language they were programmed in. For PHP it's C. For HTML, CSS, and JavaScript it depends on the language of the browser. For more information: https://en.wikipedia.org/wiki/Abstract_syntax_tree You can create your own programming language using a BNF generator: https://en.wikipedia.org/wiki/Extended_Backus–Naur_Form Some libraries provide a working lexer: https://github.com/doctrine/lexer/blob/master/lib/Doctrine/Common/Lexer/AbstractLexer.php -
It's really simple. Add a referred_by to your table and when IS NOT NULL then apply 5% to the ID.
-
No, he probably saw them editing JavaScript. In this case all occurrences are changed at the same time.
-
How does one overload Arithmetic operators for a class?
ignace replied to Fligex's topic in PHP Coding Help
Like scootsah already pointed out, you can only pass instances of A. That said to make it more natural to arithmetic operations you can use value objects. class Operand { private $value; public function __construct($val) { $this->value = $val; } public function add(self $other) { return new static($this->value + $other->value); } public function __toString() { return $this->value; } }So your program can look like this: $seven = new Operand(7); $four = new Operand(4); $eleven = $seven->add($four); var_dump($seven, $four, $eleven); $twentytwo = $eleven->add($seven)->add($four); var_dump($seven, $four, $eleven, $twentytwo);As you can see $seven remains the value 7 as does $four remain the value 4 throughout the application. -
You can print a cheat sheet by going to Help > Default Keymap Reference. I must admit I use Sequel PRO for actual querying (no real reason just force of habit I guess). The only reason I have the database navigator active is for autocompletion of tables and columns in my code.
-
If your project is coupled/synced to the FTP then you don't need to know which file is changed, since it uploads it for you when it saves the file. You'll see it when you CTRL+TAB, it'll say at the bottom: "Uploaded file X to server Y in Z seconds." That said, this is not a typical workflow. Like scootsah already pointed out normally you'd use a VCS then commit and deploy from there. That said, you can also deploy from within the IDE when you commit. CTRL+K (or VCS > Commit Changes) in the right pane it says: "After commit > Upload files to:" You can also add several tools that will execute (like Phing) when you commit etc.. I love this IDE too, the only limit is your imagination I guess
-
How does one overload Arithmetic operators for a class?
ignace replied to Fligex's topic in PHP Coding Help
self points to the current class, so basically it says function add(A $b). You'll see also other like static. However this won't work, since static is late bind, while self is early bind which is why this works. -
How does one overload Arithmetic operators for a class?
ignace replied to Fligex's topic in PHP Coding Help
PHP does not support arithmetic operator overloading. You can however use a simple method to achieve this. class A { public $value; function add(self $b) { $this->value += $b->value; } } -
No, they are not. Your constructor does a require_once() which means that with every instance you'll load the same file over and over. Move it somewhere else or at the top of the file before your class definition. public function __construct(Google_Client $googleClient) { $this->client = $googleClient; if($this->client)Not sure why you think you need to test $this->client, in case PHP wouldn't PHP?
-
How to tame Separation of Concerns overkill
ignace replied to dennis-fedco's topic in PHP Coding Help
Like I already said you know when to load up dependency X and Y when you call the method on the mapper. Loading extra during presentation will lead to problems. Doctrine provides this functionality just to not break your code. And it is also easy to trace back when reviewing your query log. It'll show up something like: SELECT .. FROM table WHERE id = 1 SELECT .. FROM table WHERE id = 2 SELECT .. FROM table WHERE id = 3 .. -
http://forums.phpfreaks.com/topic/262327-bored-looking-for-ideas-heres-a-list/
-
How to tame Separation of Concerns overkill
ignace replied to dennis-fedco's topic in PHP Coding Help
The dependency is "wrong" because your entities depend on a higher layer. In other words the DAO layer should be dependent upon the entities (Motor, Product) not the other way round. Doctrine solves this problem by using Proxy Entity objects. More on that here: http://stackoverflow.com/a/17787070 This is kinda the same as what you did with the exception that this dependency is hidden and inexistent when the full object is loaded. Which is also what the Mapper pattern allows you to do. For example when a User logs in, it may not be required to load the groups he is a member of. So in this case this becomes either null (resulting in an Exception or Fatal Error) or a ProxyCollection (in case of someone does access it, of course for performance reasons it is best to not be used). However when you want to view the groups he is a member of it is required to load the Groups. Since you access business methods on the Mapper object. You know exactly what you should and should not load (or lazy-load). -
Is this the correct implementation of a mvc service layer?
ignace replied to ICJ's topic in Application Design
Your code looks good although I would move the redirect back to the controller and not handle it in your service. -
It would help to post the PHP code. Also mostly these are cut-and-paste/works-out-of-the-box type of code. Since you have a website I assume you have at least a little technical background? And I assume your server supports PHP? Have you tried creating a new file pasting in the code and see what it outputs?
-
How to tame Separation of Concerns overkill
ignace replied to dennis-fedco's topic in PHP Coding Help
The difference is that in my case the Mapper has context so related objects are also loaded. While your chained method calls need to be called every time you need more data, resulting in more queries. Also because your Entity uses the DAO directly it is actually tied to your DB which has the dependency the wrong way like an object created by a Factory object would be tied to its creator. These should be decoupled. As to why the Mapper I link to this article: http://stackoverflow.com/a/814479 -
How to tame Separation of Concerns overkill
ignace replied to dennis-fedco's topic in PHP Coding Help
Yes, you went too far. Also your business classes should not be aware of DB classes. If your Product needs a Motor then make sure your DAO injects it. class ProductMapper { private $SQL_LOAD_PRODUCT_WITH_MOTOR = 'SELECT .. FROM .. JOIN .. WHERE ..'; public function someBusinessIdentifiableMethod() { $rows = db_query($this->SQL_LOAD_PRODUCT_WITH_MOTOR, [..]); foreach ($rows as $row) { $products[] = $product = new Product(); //.. $product->setMotor($motor = new Motor()); } return $products; } }Your Mapper class would query your database and then "map" the result to objects. Much like the ResultSetMapping from Doctrine.