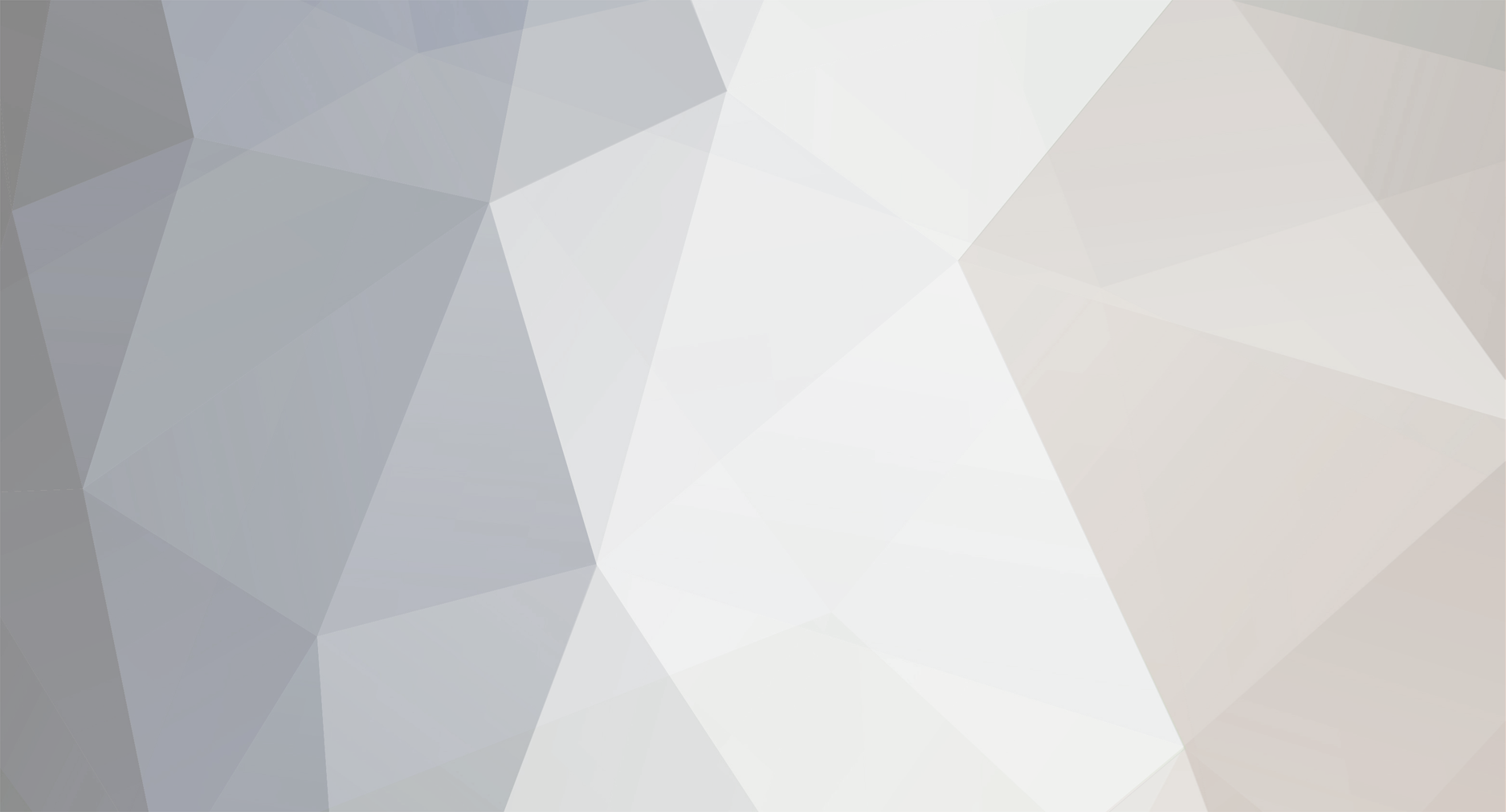
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
You don't have to try to look, your eyes already do this for you. You just have to point them into the right direction.
-
Here's a little class I wrote that will do what you want: class CaesarCode { private static $alfabetUpper; private static $alfabetLower; private $steps; private function _getNewPosition($originalPosition) { return ($originalPosition + $this->steps) % 26; } private function _getCharFromArray($char, $fromArray) { if (($charKey = array_search($char, $fromArray)) !== false) { return $fromArray[$this->_getNewPosition($charKey)]; } return false; } private function _translateChar($char) { $translatedChar = $this->_getCharFromArray($char, $this->_getAlfabetUpper()); if ($translatedChar === false) { $translatedChar = $this->_getCharFromArray($char, $this->_getAlfabetLower()); } return $translatedChar; } private function _getAlfabetUpper() { if (empty(self::$alfabetUpper)) { self::$alfabetUpper = range('A', 'Z'); } return self::$alfabetUpper; } private function _getAlfabetLower() { if (empty(self::$alfabetLower)) { self::$alfabetLower = range('a', 'z'); } return self::$alfabetLower; } public function __construct($steps) { $this->steps = $steps; } public function translate($string) { $encr = ''; foreach (str_split($string) as $key => $char) { if (($newChar = $this->_translateChar($char)) !== false) $char = $newChar; $encr .= $char; } return $encr; } } Use it like: $code = new CaesarCode(13); echo $code->translate('Hello World!');
-
You could have easily found this by just inspecting the element in your browser. F12 brings up the developer tools. I copy-pasted this from their website: ::selection{ background:#E92C6C; color:#fff; text-shadow: 0 1px rgba(0, 0, 0, .2); } It's not supported in all browsers though: http://reference.sitepoint.com/css/pseudoelement-selection
-
Add the below line to your script: ignore_user_abort(true); http://php.net/manual/en/function.ignore-user-abort.php This will continue the script regardless if the user closed the browser.
-
Try this: include("config.php"); if(isset($_GET['username']) and ($_GET['password']) and ($_GET['hwid'])){ $username = $_GET['username']; $password = md5($_GET['password']); $sql = "SELECT banned FROM `members` WHERE `login`='$username'"; $query=mysql_query($sql) or exit("ERROR: " . mysql_error() . "<br>QUERY: " . $sql); $result = mysql_num_rows($query); $outcome = ($result > 0) ? 'true' : 'false'; echo $outcome; }
-
Read the documentation? http://be2.php.net/strftime
-
Please post your code.
-
$this->load->library('layout'); if (!isset($this->layout)) $this->layout = get_instance()->load->library('layout'); var_dump($this->layout); What does this tell you?
-
Write it down very thoroughly how everything works and how future components should be structured. Any programmer should be able to commence working on the project after reading it. Use the below document for inspiration, not copying since most will go beyond what you need, to create your own document: http://www.cmcrossroads.com/bradapp/docs/sdd.html
-
How about: $this->configure() ->option('name')->required()->unless('event') ->option('params')->required()->unless('event')->type('array') ... The Option dependency is than hidden and allows for some abstraction. interface Option { public function option($name); }
-
The first question that comes to mind: did you try it? Are you afraid a syntax/runtime error may crash your computer? A small test-case: switch ('will the switch correctly handle this string?') { case 'will the switch correctly handle this string?': echo 'yes'; break; case 'oh NOES!!': echo 'no'; break; default: echo 'at this point you should start running...'; break; }
-
Just like any JS library just create a normal and a minified version. You can control which version is used through something like: PHP define('USE_MINIFIED', getenv('USE_MINIFIED') ?: true); .htaccess SetEnv USE_MINIFIED 0 In your code: <script src="<?php print asset('js/script.js'); ?>"></script> function asset($script) { $info = pathinfo($script); // you can also do other stuff here, like append the last modified time: '.' . filemtime($script) return $info['dirname'] . '/' . $info['filename'] . (USE_MINIFIED ? '.min' : '') . '.' . $info['extension']; } You can download the jar files to compress CSS and JS. Just create a little shell script to minify CSS and JS. JavaScript http://code.google.com/intl/nl/closure/compiler/ JS+CSS http://developer.yahoo.com/yui/compressor/ You can also find a few minifiers for your images online. If you use PageSpeed or YSlow you'll get a few links to online services to minify your images while preserving quality.
-
'Twas the night before Christmas, and mySQL won't accept my Update query.
ignace replied to Mavent's topic in PHP Coding Help
I LOL'ed when I saw your code. For your database to actually ever receive the query you need to call mysql_query() with your actual query as a parameter. Something like this: $result = mysql_query($query) or trigger_error('ERROR: ' . mysql_error() . '<br>QUERY: ' . $query, E_USER_ERROR); if ($result && mysql_num_rows($result)) { // query executed successful AND it returned a result. } -
1) The best thing to do is load jQuery from a CDN (like: https://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js). Chances are that the user visited another website that also used the same CDN and thus using the file from cache when visiting your website. 2) Use at your own discretion: Have one CSS file with all your CSS rules for all your pages (publicly visible ones) and one JS file with all your JS functions for all your pages (publicly visible ones), minify it, enable gzip on your server, and cache them till the end-of-days (that's till 12-21-2012 12:00:01 as some believe). Pro: - You have access to every defined function (no need to lazy-load extra JS files when you need access to another set of functions) - No need to share/duplicate CSS rules between pages - Subsequent visits use the cached CSS and JS regardless of page Con: - First-time visit may take a while to load the files - Too much data can crash the user's browser
-
Probably because 1 page has multiple states now across multiple requests which is otherwise not the case. if (isset($_GET['new_game'])) { // user wants to start a new game // store info in sessions header('Location: /'); } else if (isset($_GET['guess'])) { // user has guessed a letter or word // store info in sessions header('Location: /'); } else if (isset($_SESSION['game_over'])) { if ($_SESSION['game_over']) { // game over, try again? // clear session data } else { // display game progress } } else { // display main menu }
-
UPDATE negative_words SET word = TRIM(word);
-
Censorship doesn't work on the internet. In Belgium a few websites have already been blocked, but we just register new DNS names, and they are back at square one. The same with the copyrighted material. All links will be encoded/indirectly linked to the copyrighted material. For every item they block/censor. 1000 new appear. Bureaucrats don't understand why we have called it the Web --> There is more than one way to get to it.
-
Start out by identifying your pages and what they will do. What will the user see? Does it have a form, how does it different states look (submit error, submit success, ...)? Does it have AJAX, how will it communicate state changes (service unavailable, service hang, ..)? It isn't rocket science, you know/can think of this stuff.
-
The code for the shopping cart module has to be completely ignorant of the 3 systems. Once this is complete you need to write a specific script for each of the 3 shopping cart systems that proxies to your own code. This is a bad idea as this will prohibit you from using it on another website once it is added unless additional code is written.
-
Web 3.0 is when the web becomes your personal assistant. You ask it: I'm in the mood for a good action movie released in the last 5 years with an IMDB rating of no less than 7 and got great reviews and I'm also out of popcorn where can I get some? And the web would reply with good action movies with an IMDB rating of no less than 7 that got great reviews and on which channels they play and when, and it also points me to a store where they have popcorn in stock! That may also just be my imagination toying with me when I read that memo It may also have been an april fools but it stuck with me Web n.0 is not colors, rounded corners, or some other techno gizmo. Web 2.0 was/is social publishing for example (in other words: people with no clue can publish stuff on the internet). Web 1.0 is when only those gifted with the knowledge of HTML could publish stuff on the internet.
-
Try this: var $cc = $('.cc'); $(window).scroll(function() { var yScrollOffset = window.pageYOffset; $cc.each(function() { var $this = $(this); $this.css({top:$this.position().top + yScrollOffset}); }); });
-
http://freedcamp.com is an online solution. It's not open-source though, but offers all that you need. Some more examples can be found at: http://www.denbagus.net/fresh-open-source-project-management-invoice-and-tracking-you-should-know ArgentumInvoice is what I think you are looking for.
-
NetBeans: Expand Tabs to Spaces
ignace replied to doubledee's topic in Editor Help (PhpStorm, VS Code, etc)
Obviously, if you are the only one developing. Try opening that in notepad++ or another IDE. Behold the almighty SHIFT + TAB. Which tabs in the other direction. Maybe take a day off to learn the tools you are working with? Pretty hard to punch a nail if you hold the hammer backwards. -
Uninstall - reinstall has gone horribly wrong
ignace replied to JohnMike's topic in PHP Installation and Configuration
CTRL + SHIFT + ESC --> Services tab, below press Services button. Look for Apache and MySQL. It's called experimenting, and is generally a good thing. You can't break anything if you never do anything. Just look at what you all learned by just uninstalling something. -
Question on the best way to set up OOP class relationships
ignace replied to dgruetter's topic in Application Design
$brides = new BrideRepository($db); $brides->addBride($_POST); $vendors = new VendorRepository($db); $vendors->findByCategory($_POST['category']); $vendors->findByRegion($_POST['region']); $vendors->findByRegionAndCategory($_POST['region'], $_POST['category']); OR $vendors = new VendorRepository($db); $find = new VendorSpec; $find->whereCategoryIs($_POST['category']); $find->inRegion($_POST['region']); $find->matchAny(); $vendors->findBySpec($find); $brides = new BrideRepository($db); $brides->find($id)->bookmarkVendor($_POST['vendor']); $brides = new BrideRepository($db); $brides->find($id)->publishReview($_POST['title'], $_POST['body']); $vendors = new VendorRepository($db); $vendors->addVendor($_POST); $vendors->find($id)->.. I do not encourage though that you will start to use this as it's serious overkill for your needs. OOP should only be used when your application has some serious business rules that are harder to accomplish/maintain when programming procedural. The above may seem to be not that much work, but that's because you are only looking at the abstracted layer. The BrideRepository for example communicates through different Table Data Gateway's to retrieve it's data: bride table, bride_bookmarked_vendor table, and bride_published_article table. defining/finding new responsibilities and boundaries are easy for me, it's always figuring out a damn name that clearly communicates what the class does that trips me up. Having conventional names kinda helps but not entirely.