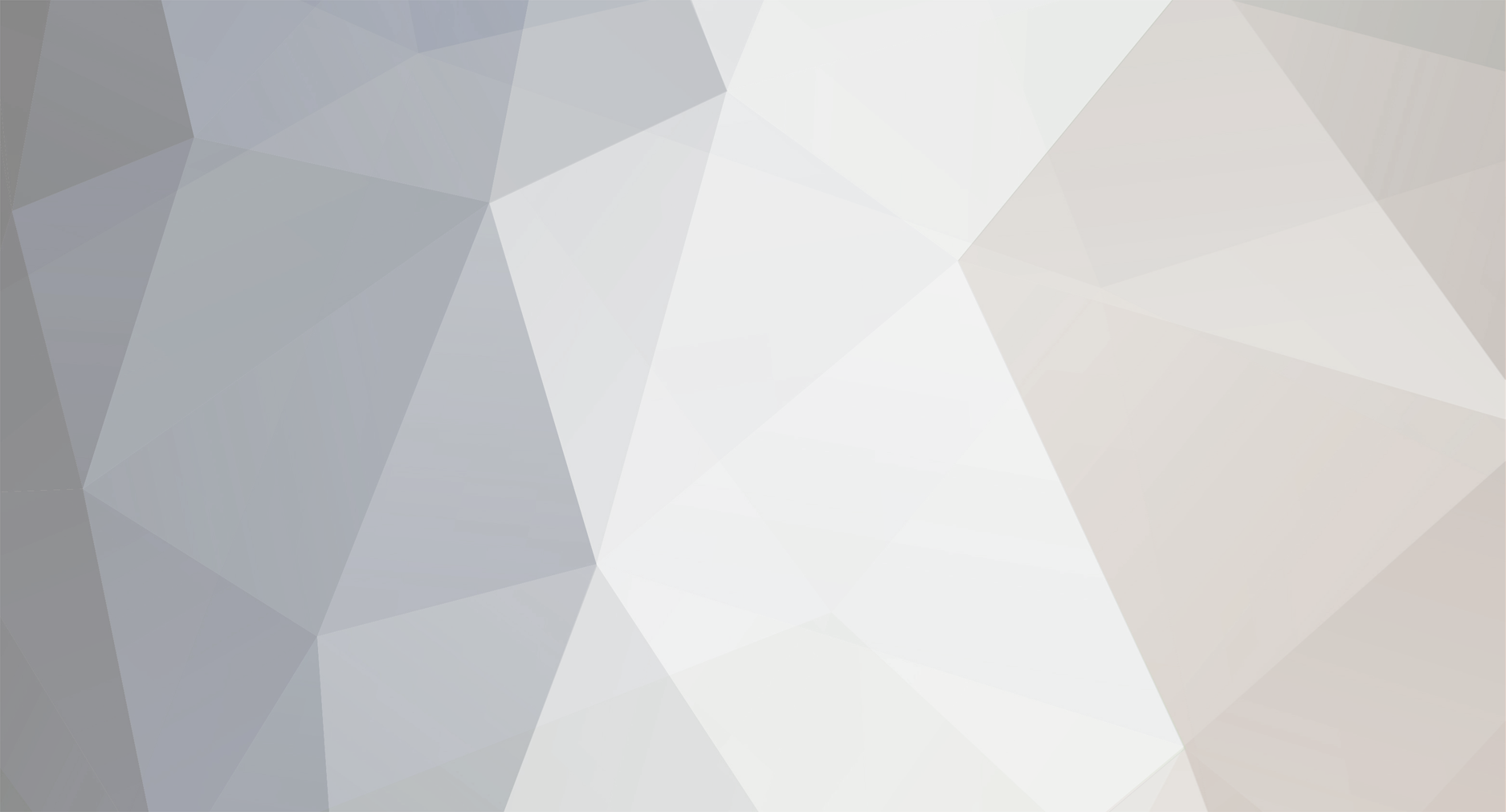
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
The Controller belongs in the Application Layer this means that it knows everything Application specific like POST and GET. So in your Controller you would write: $album = new AlbumRepository(); $this->ui->assign('album', $album->find($_REQUEST['id']); Avoid the use of static as much as possible. It's OO not procedural.
-
class Core { public function server_status($what) $what = mysql_real_escape_string($what); mysql_query("SELECT " . $what . " FROM server_status") // * being value } } Or are you just after how to access an object like an array? class Core implements ArrayAccess { public function offsetGet($offset) { switch ($offset) { case 'server_status': return $this->getServerStatus(); } } public function offsetSet($offset, $value) {/*dummy*/} public function offsetExists($offset) { return in_array($offset, array('server_status', ..)); } public function offsetUnset($offset) {/*dummy*/} } $core = new Core(); print $core['server_status'];
-
Something like this? $onlineUsers = $system->getModule('online_users'); print '<pre>' . $onlineUsers->toString() . '</pre>'; $server = $system->getModule('server'); print '<pre>' . $server->toString() . '</pre>';
-
It should be: c:\xampp\php not c:\xampp\php\php.exe That said you may want to use your own set of env vars, I do it like this: I add a new env var: PHP=D:\wamp\bin\php\php5.3.5 I then add that env var to my PATH env var: PATH=%PATH%;%PHP% If I want to change to where the PHP env var points I just change it: PHP=D:\wamp\bin\php\php5.2 and I'm done. I don't have to go mess in some massive string. I have the same thing set-up for MySQL and other services like SVN and GIT.
-
We think alike! Is it intended to calculate this for everyone in the bday table?
-
function calculate_age($birthday) { $time = strtotime($birthday); if ($time === FALSE || $time === -1) return FALSE; $a = idate('Y') - idate('Y', $time); $b = mktime(0, 0, 0, idate('n', $time), idate('j', $time), idate('Y')); if (time() < $b) --$a; return $a; } print calculate_age('1986-09-05').PHP_EOL; // 24 print calculate_age('1986-08-05').PHP_EOL; // 24 print calculate_age('1986-07-05').PHP_EOL; // 25 Just an idea. I prefer phpSensei's solution.
-
http://www.campaignmonitor.com/testing/
-
Take a look at hMailServer. At work I use yet another program but I can't find it by a quick search on the net and I can't recall the name. I'll update this topic as soon as I get the name. EDIT: The program's name is Papercut. The website currently returns a 404. Darn. EDIT 2: It's now hosted on CodePlex: http://papercut.codeplex.com/
-
If you could give us an example that we can work with instead of firstClass and secondClass I'm sure we can come up with even better approaches. Template Method for example is a design pattern specifically targeted at this.
-
Post your (relevant) code.
-
how to set default controller in codeigniter based on cookie value ?
ignace replied to linux1880's topic in Frameworks
Have you tried overwriting the fetch_class() method on the Routing class? -
Pinging won't solve anything. Pinging only means that the host is reachable not that they are still chatting. So no, there is no real way except maybe using the onunload event of which I'm unsure if it triggers correctly on all browsers/platforms. What you actually need is a socket which is available as of HTML5: WebSockets, you can find a tutorial of how to use it on NetTuts+
-
Just ask them All jokes aside! Yes, you would poll your server every n seconds to fetch the new contents and update their status, anyone where the delta between last update time and the current time exceeds the idle time is considered offline. Preferably you should return this to any active clients so they can update their list of users in the chat.
-
Git is a great tool except it does not run out-of-the-box under Windows (which is I think your main OS), so you'll either need Cygwin or msysgit. Neither are advisable if you have never used Linux or the Windows CLI before. Subversion is only an option if you have a live SVN server running otherwise you won't even be able to do the basics: commit source code. Some server hosts provide SVN hosting but not many do. You can find a free Subversion host at Unfuddle and download TortoiseSVN to have nice GUI to work with SVN. All commits you do are against the SVN server so this may become a bottleneck if the commit is quite large since you can't use SVN until the commit is complete. Another great option on the market is Mercurial (which comes pre-bundled with TortoiseHG). Mercurial is like Git a distributed SCM and all commits are done against your local copy, which is fast and there is no need for a server. However in order to collaborate both developers have to be online at the same time so you can push your changes and pull their changes (and resolve any conflicts in the process). This is of course becomes a problem if you aren't always online when the other developers are so for that you'll still have the strengths of Mercurial but you'll also need a server to push your changes to (BitBucket being one of these). Now you can work any time you like as do the other developers and they push their changes to BitBucket and pull your changes. So Mercurial gives you a little more flex than Subversion does as you can commit while offline and push your changes whenever you get the chance to be online. Pick whatever suits you best and if you are in for adventure give Git a try
-
You should look at the bigger picture here. Once you put your website live, you can't just go and do some live editing since that could ruin a user's experience therefor you need to have your website running locally on your computer with a development database, not the real live database. And patch the online code after you verified it works (be sure to notify your users of a scheduled maintenance and put your website "offline" till all code has been patched (uploaded)). You can put your website "offline" by using an .htaccess to redirect all traffic (expect you) to a maintenance page (be sure to return a proper HTTP status code for any spiders/crawlers on your website to come back later). An SCM (Source Code Management) tool like SVN helps you to merge conflicting files instead of having to do the process manually, in which you may even remove some work of your fellow developer. Other options you have with an SCM tool is to tag a specific release like 0.1, 0.2, .. which is nice if for example if you have a bug since v2.5 and found the root-cause in v2.9, you can then go back (checkout) to v2.5 and commit the fix and your SCM tool will internally patch all code till the latest version 2.9 (imagine doing that manually). Which you'll thank your SCM for many times if you have a customer running on an older codebase. Another great option SCM provides you with is branches. This is specifically great if for example you want to implement a new feature but you don't want to hinder bug fixes. So you can develop the new feature while bug fixes can be released on a regular basis. Once your feature is complete you merge it back to the main codebase and resolve any conflicts.
-
function getOverdueString($dueDate, $currentDate = 'now', $timezone = 'Europe/Brussels', $formatString = '%s%dd %dh %dm') { $old_timezone = date_default_timezone_get(); date_default_timezone_set($timezone); $a = strtotime($dueDate); if ($a === FALSE || $a === -1/*<5.1*/) return FALSE; $b = strtotime($currentDate); if ($b === FALSE || $b === -1/*<5.1*/) return FALSE; date_default_timezone_set($old_timezone); $diff = $a - $b; $sign = $diff < 0 ? '-' : ''; $diff = abs($diff); $days = floor($diff / 86400); $hrs = floor(($diff % 86400) / 3600); $mnts = floor((($diff % 86400) % 3600) / 60); return sprintf($formatString, $sign, $days, $hrs, $mnts); } I have made a few adjustments: 1) added floor to $hrs and $mnts 2) added in timezone support since your customers will likely be in a different timezone so the overdue date should be calculated according to their timezone otherwise they would buy/rent your product and lose a few or lots of hours on the meter before overdue. So be sure to ask the timezone of your customer. Proof-of-Concept: // 'now' => 2011-07-31 8:16:00 print getOverdueString('2012-07-31 8:12:00').PHP_EOL; // 365d 23h 55m print getOverdueString('2011-07-31 8:12:00').PHP_EOL; // -0d 0h 4m print getOverdueString('2010-07-31 8:12:00').PHP_EOL; // -365d 0h 4m Be sure to change Europe/Brussels with yours. You can find the supported timezones on php.net
-
Too much typing.
-
I am not aware of any standard functions that mimic DateTime perfectly I have tested both strftime() and date() none of them got it correct date() f.e. returned 1 day 30 mins for 1800, that may be my bad either though not sure. Here's an example that works like the DateTime function did. function getOverdueString($dueDate, $currentDate = 'now', $formatString = '%s%dd %dh %dm') { $a = strtotime($dueDate); if ($a === FALSE || $a === -1/*<5.1*/) return FALSE; $b = strtotime($currentDate); if ($b === FALSE || $b === -1/*<5.1*/) return FALSE; $diff = $a - $b; $sign = $diff < 0 ? '-' : ''; $diff = abs($diff); $days = floor($diff / 86400); $hrs = ($diff % 86400) / 3600; $mnts = (($diff % 86400) % 3600) / 60; return sprintf($formatString, $sign, $days, $hrs, $mnts); } // echo getOverdueString( '2011-08-05 09:00:00', '2011-08-05 08:30:00'); // 0d 0h 30m
-
That said you could just write: if(!mysql_num_rows($result))
-
In mjdamato's code is a bug: if(mysql_num_rows($result)=0) Should be if(mysql_num_rows($result)==0)
-
That's because Dreamweaver doesn't parse your PHP code. You need to have a webserver installed like XAMPP and have your files hosted in it's htdocs directory (unless you know, which I doubt, how to re-configure Apache to point to the current location of your directory). You can then see the output, if you are lucky formatted like you did in Dreamweaver since DW is a WYSIWYAG: What You See Is What You Almost Get, if you navigate your browser to either: http://localhost:80/ or http://localhost:8080/ localhost (the reserved 127.0.0.1 ip address) is not a website like www.google.com it's a loopback address or in other words it points back to your computer with a certain protcol (http) and a port (80) this will trigger your local XAMPP installation as it's listening on one of these ports with a http protocol handler (Apache).
-
If this is for debugging purposes you should just extend your class to accept a log object and use that inside your class. $log->methodCalled(__METHOD__);
-
The above function should be self-explanatory. It gives you complete control over the used format (the DateInterval format). Only the due date is required all other parameters are optional. So you could just write: echo getOverdueString($dueDate); If the date is invalid or it couldn't calculate a diff the output will be '' (maybe change that to FALSE?) Another option could be to just return the DateInterval object and go from there since it provides you with handy properties for even more control than format() does.
-
function getOverdueString($dueDate, $currentDate = 'now', $formatString = '%r%dd %hh %im') { try { $a = new DateTime($currentDate); $b = new DateTime($dueDate); $diff = $a->diff($b); if ($diff instanceof DateInterval) { return $diff->format($formatString); // formats: (positive) 1d 1h 0m (negative) -1d 3h 0m } } catch (Exception $e) {} return ''; } // echo getOverdueString( '2011-08-05 09:00:00', '2011-08-05 08:30:00'); // 0d 0h 30m
-
If you already have a class say Database, you can create a postgresql class that extends your Database object and overrides all methods. class Database {/*mysql/mssql*/} class PostGreSQL extends Database {/*override all methods of Database*/} Better would be if you made Database an interface and then create a MySQL, MSSQL, and PostGreSQL class. It wouldn't take you more than just changing the original $db = new MySQL(..); To the new: $db = new PostGreSQL(..); If you are in to Design Patterns I suggest using a Factory to get the DB driver.