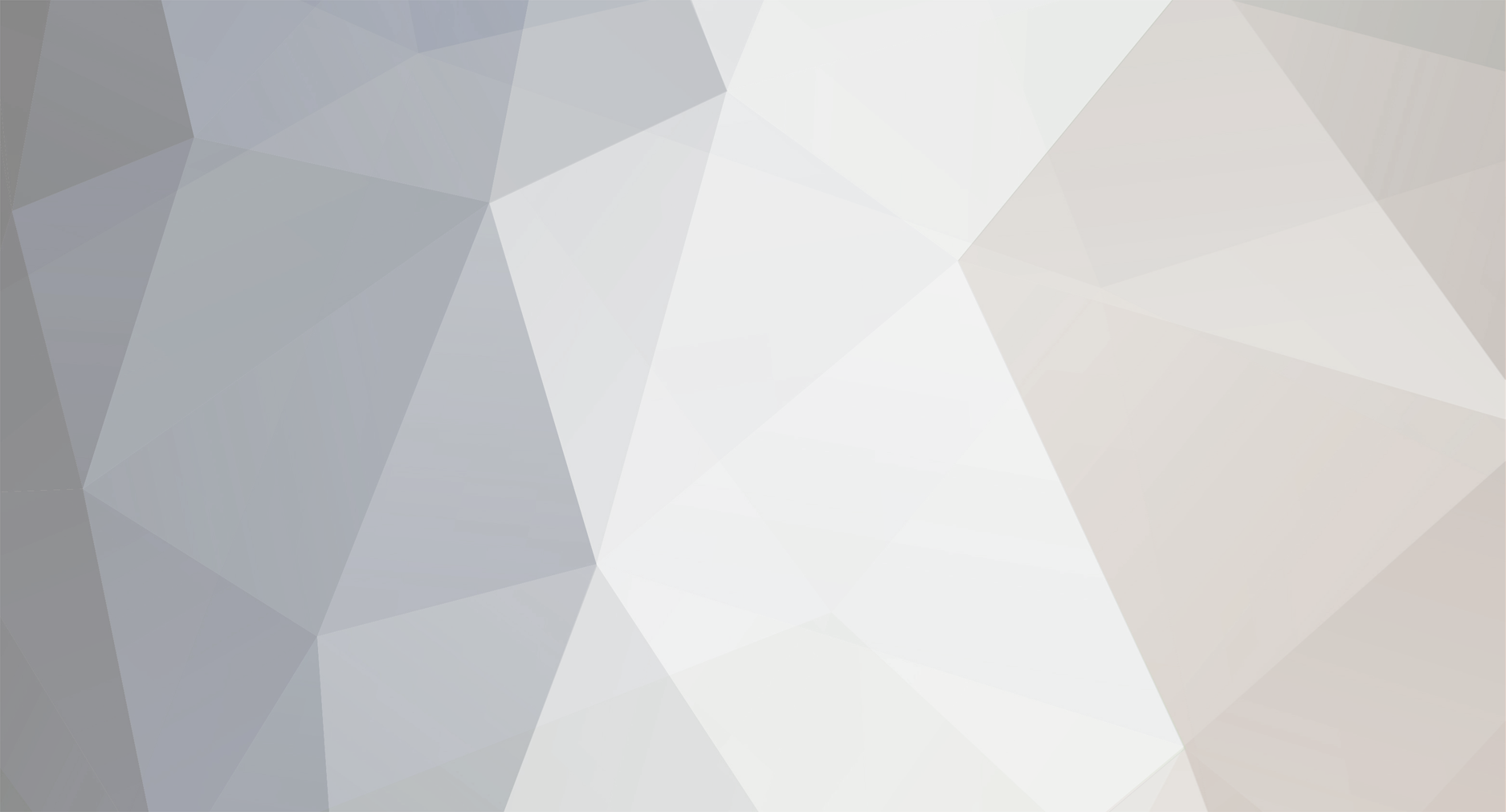
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Adressbook - how to add more fileds to this one
ignace replied to dilbertone's topic in PHP Coding Help
Why would you need 10 tables? One should suffice. Using LOAD DATA you can load the data from the CSV to your table. LOAD DATA INFILE INTO TABLE addressbook (name, address, email, tel, fax) COLUMNS TERMINATED BY '\t' LINES TERMINATED BY '\r\n' -
$query = 'SELECT `name` FROM `table` LIMIT 20'; $result = mysql_query( $query ); list($left_array, $right_array) = array_chunk(array_map('mysql_fetch_assoc', array_fill(0, mysql_num_rows($result), $result)), 10);
-
Clearly PHP isn't installed due to the < and > it's not rendered by the browser. Make sure you started with <?php and not <? (try running it again). If you are running this on your computer, then install WampServer or XAMPP otherwise ask your tutor for guidelines on where you need to put these files and which extension they should have (.php; .php5; .php4; .php3; .phtml; ..).
-
Adressbook - how to add more fileds to this one
ignace replied to dilbertone's topic in PHP Coding Help
UNION INSERT INTO addressbook (name, address, email tel, fax) VALUES (SELECT name, address, email, tel, telefax FROM addressbook1) UNION ALL (SELECT name, company adress, email, telefon, fax FROM addressbook2) UNION ALL .. -
Any learning is pretty much hands-on, skimming through the reference manual when my test say's WTF?! Zend framework assumes that anyone taking it on has strong roots in OOP or at least knows how to make his boat float!
-
The obvious problem with TLG's solution is: what if there's a missing translation? Currently the customer would see {DICT.somethingHere} or whitespace, worst case would be that your entire menu would be invisible due to missing translations or file permissions. Personally I think the best approach is to simply write the website in a default language, pass it through some function that returns the input if it fails to load a translation. <li><?php print $this->translate('Home'); ?></li> <li><?php print $this->translate('About us'); ?></li> <li><?php print $this->translate('Contact us'); ?></li>
-
class Form { public function addElement(FormElement $element) { array_push($this->_elements, $element); return $element; } } $form = new LoginForm(); $element = $form->addElement(new SingleLineInputElement()); $element->addValidator(new EmailAddressValidator()); // $this->validatorChain->stopOnFirstError(false)->isValid($this->getValue()); class UserRepository { private $validationRules = array('email_address' => 'EmailAddressValidator', 'username' => 'UsernameValidator'); public function createRow() { $record = new ActiveRecord($this->getAdapter(), $this->tableColumns); $record->addValidator('email_address', new EmailAddressValidator()); return $record; } } $row = $userRepo->createRow(); $row->email_address = 'foo'; $row->save(); // ERROR: INSERT FAILED 'foo' is not a valid email address
-
Indeed, I also accounted for quantity. In cmattoon's design at best this is spread between 2 tables which makes it hard to maintain and prone to error. The tables product, product_color, and product_size respectively holds the product, the possible colors, and the possible sizes (duh!). The product_quantity ties them together: INSERT INTO product_quantity (product_id, product_color_id, product_size_id, quantity) VALUES ('T-Shirt #1', 'Red', 'S', 2), ('T-Shirt #1', 'Red', 'M', 10), ('T-Shirt #1', 'Red', 'L', 5) ('T-Shirt #1', 'Blue', 'S', 1), ('T-Shirt #1', 'Blue', 'M', 5); For convenience I used text instead of numbers although in the case of size you could use the label as PK. Due to foreign key constraints you won't have a Blue and Bleu T-Shirt which was possible in cmattoon's design. The design also makes it easy to find how many of T-Shirt #1 you have in stock regardless of size or color: SELECT name, sum(quantity) FROM product_quantity JOIN product USING (product_id) WHERE product_id = 1 Or per color of a particular T-Shirt: SELECT color, sum(quantity) FROM product_quantity JOIN product_color USING (product_color_id) WHERE product_id = 1 AND product_color_id = 1
-
product (product_id); product_color (product_color_id); product_size (product_size_id); product_quantity (product_id, product_color_id, product_size_id, quantity);
-
Way of accessing static variables inside class with expression returns?
ignace replied to Slips's topic in PHP Coding Help
I don't see why you need a utility class that holds the alphabet as a static variable. The alphabet isn't likely to change any time soon. Since you supposedly need this I think you haven't yet identified certain models or thought well about your OO-design. -
In your specific instance you could have: spl_autoload_register(array('LibraryAutoloader', 'autoload')); spl_autoload_register(array('ApplicationAutoloader', 'autoload')); So that instead of having to add yet another if to your __autoload each new module can have it's own autoloader without having to modify any code. You should avoid static methods as much as possible as this is procedural programming and not true OO. This actually hurts re-usability. Better would be to use a setup like Zend where there is a class for each item so that Form's and Model's can use validation/filtering. $form->addValidate('username', new Zend_Validate_Username()); $model->addValidate('username', new Zend_Validate_Username());
-
Need ideas for database design for html alt tags
ignace replied to rusty338's topic in Application Design
Or store the image in your DB, very handy if it turns out your hosting company only creates back-ups of the DB files. -
General advice on plan of attach for quiz website
ignace replied to galvin's topic in Application Design
If you are hiding the correct possible answers in your HTML then everyone with some HTML knowledge will be able to answer your questions correctly. Let the user enter the answer match that in PHP with the correct possible answers synchronously (reload the page) or asynchronously (AJAX, reload part of a page). -
Meet the method argument list: $model->test($form);
-
function __autoload($class_name) { include $class_name . '.php'; } You should take a look at spl_autoload_register as this allows you to use an OO-approach to autoloading. class controller extends mysql A Controller does not extend MySQL. Such implementation details should be abstracted so that your application becomes not dependent on the presence of any specific extension/technology. class model A Model is not explicitly named Model, it's a concept. If you have ever programmed an application where you would have a file like contact.php that did the processing and called a separate file for all HTML-related stuff then you have programmed an MVC-architecture (sorta). The Controller is responsible for all Application-logic (POST, GET, ..), the Model lives in the Domain-layer and is responsible to enforce business rules (eg login after verification) and use some data store to persist information, the View has the only responsibility of showing stuff (not do any real work).
-
I do not only have the codes, I can even see them: 000001110001001010000101010001010101010101010101010101010101010 100101000010101000101010101010101010101010101010101001010010101 111000100101000010101000101010101010101010101010101010101010101 000001110001001010000101010001010101010101010101010101010101010 100101000010101000101010101010101010101010101010101001010010101 111000100101000010101000101010101010101010101010101010101010101 000001110001001010000101010001010101010101010101010101010101010 100101000010101000101010101010101010101010101010101001010010101 111000100101000010101000101010101010101010101010101010101010101 000001110001001010000101010001010101010101010101010101010101010 100101000010101000101010101010101010101010101010101001010010101 111000100101000010101000101010101010101010101010101010101010101 000001110001001010000101010001010101010101010101010101010101010 100101000010101000101010101010101010101010101010101001010010101 111000100101000010101000101010101010101010101010101010101010101 000001110001001010000101010001010101010101010101010101010101010 100101000010101000101010101010101010101010101010101001010010101 111000100101000010101000101010101010101010101010101010101010101 000001110001001010000101010001010101010101010101010101010101010 100101000010101000101010101010101010101010101010101001010010101 111000100101000010101000101010101010101010101010101010101010101
-
How to send post data and process returned XML?
ignace replied to Craig Roberts's topic in PHP Coding Help
I am more clever then I appear -
How to send post data and process returned XML?
ignace replied to Craig Roberts's topic in PHP Coding Help
Try this: function post($url, array $data) { // http://www.php.net/manual/en/context.http.php $config = array( 'method' => 'POST', 'content' => http_build_query($data), 'ignore_errors' => true ); $stream = stream_context_create($config); $response = file_get_contents($url, false, $stream); $reader = new XMLReader(); $reader->xml($response); return $reader; } $xmlReader = post('http://domain.top/resource', array('foo' => 'bar')); -
strip_tags (it also has an optional list to allow certain tags)
-
How to send post data and process returned XML?
ignace replied to Craig Roberts's topic in PHP Coding Help
Untested function post($url, $content) { // http://www.php.net/manual/en/context.http.php $config = array( 'method' => 'POST', 'ignore_errors' => true ); $stream = stream_context_create($config); $fopen = fopen($url, 'r+', false, $stream); $response = ''; fwrite($fopen, http_build_query($content)); while($chunk = fread($fopen, 1024)) { $response .= $chunk; } fclose($fopen); $reader = new XMLReader(); $reader->xml($response); return $reader; } -
$controller = getParam('c', $_GET); switch($controller) { case 'about': include 'about.php'; break; case 'contact': include 'contact.php'; break; default: include 'home.php'; break; } function getParam($offset, &$array, $default = null) { if(array_key_exists($offset, $array)) { return $array[$offset]; } return $default; } Is a (very) simple example of MVC. What level of framework are you looking for? CodeIgniter (easy) Symfony (medium) Zend (hard)
-
class OnePlusOneSimpleEchoInPhp { const STDOUT = 'php://output'; const OUTPUT = '1+1'; public static function output() { file_put_contents(self::STDOUT, self::OUTPUT); } } OnePlusOneSimpleEchoInPhp::output(); I think this is the simplest example I could think of how to do this effectively
-
Use database or individual files and then best way to setup tables?
ignace replied to galvin's topic in MySQL Help
You should create a new entry for each answer given by the user. UserResult (question_id, answer_id) But like I already said, you should mind the edge-cases. -
preload images is about the only critic I have