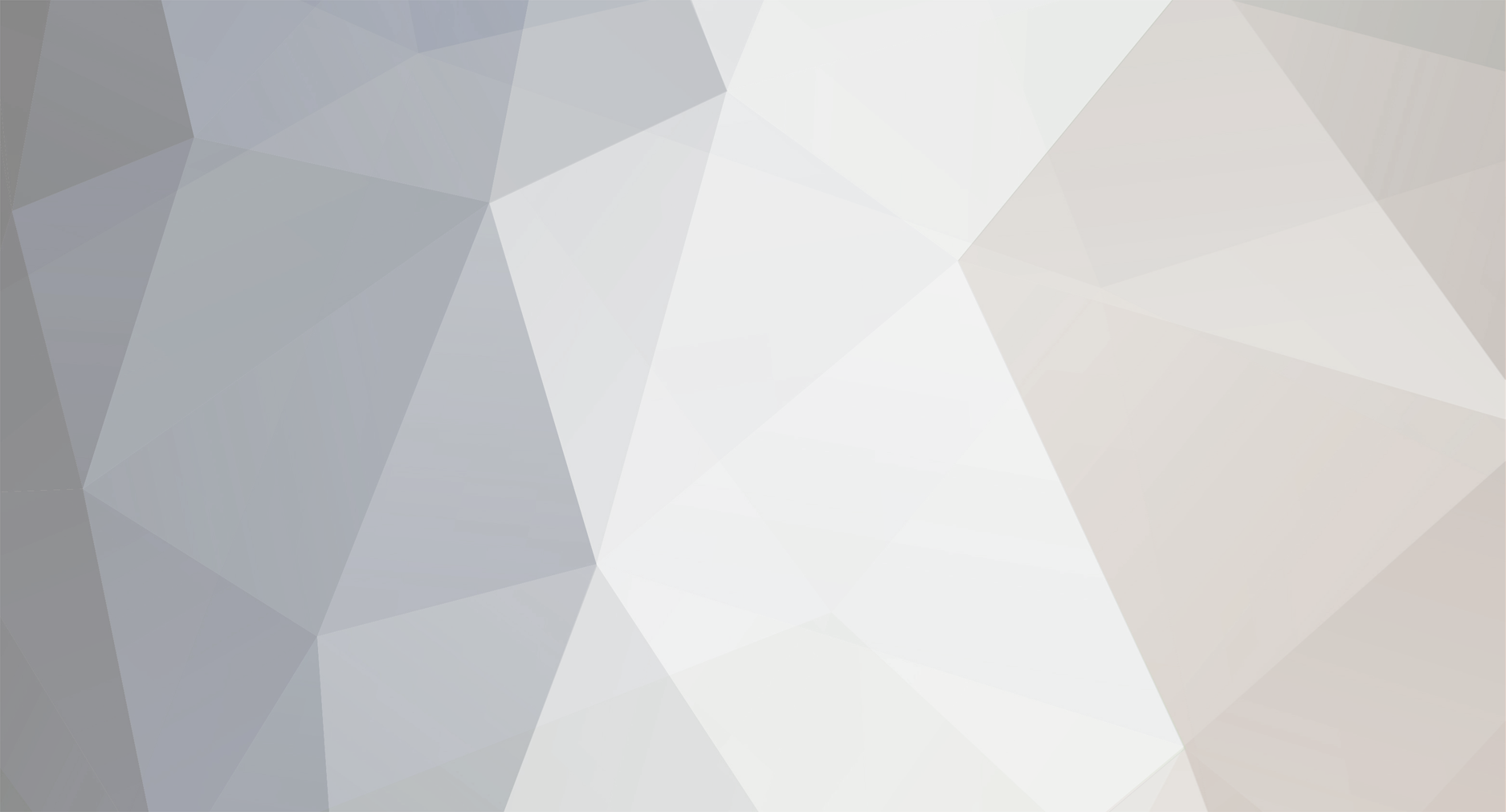
Edward
Members-
Posts
119 -
Joined
-
Last visited
Never
Everything posted by Edward
-
Hi, Yes! That is EXACTLY what I want to be able to do, I just have no idea how. Here is the basics of what I have so far, it's based on simple AJAX requests you see in beginner tutorials: index.php: <script type="text/javascript" src="js/jquery-1.2.6.min.js"></script> <script type="text/javascript" src="date_select_ajax.js"></script> <select id="select_date" onchange="date_change();"> <option>Select a Date</option> <option value="" disabled="disabled">----------</option> <?php $sql = 'SELECT * FROM Data_2009 ORDER BY id ASC;'; $result = mysql_query($sql); while($row = mysql_fetch_array($result, MYSQL_ASSOC)) { $date = strtotime($row['date']); echo '<option value="'.$row['date'].'">'.date(d.' '.M.' '.Y, $date).'</option>'; } ?> </select> <div id="output_form"></div> date_select_ajax.js var xmlHttp_date_change; function GetXmlHttpObject_date_change() { var xmlHttp_date_change=null; try { // Firefox, Opera 8.0+, Safari xmlHttp_date_change=new XMLHttpRequest(); } catch (e) { // Internet Explorer try { xmlHttp_date_change=new ActiveXObject("Msxml2.XMLHTTP"); } catch (e) { xmlHttp_date_change=new ActiveXObject("Microsoft.XMLHTTP"); } } return xmlHttp_date_change; } function stateChanged_date_change() { if (xmlHttp_date_change.readyState==4) { document.getElementById("output_form").innerHTML=xmlHttp_date_change.responseText; } } function date_change() { xmlHttp_date_change=GetXmlHttpObject_date_change(); if (xmlHttp_date_change==null) { alert ("Your browser does not support AJAX!"); return; } var url_date_change="date_select_sql.php"; url_date_change=url_date_change+"?selected_date=" + document.getElementById("select_date").value; url_date_change=url_date_change+"&rand="+Math.random(); xmlHttp_date_change.onreadystatechange=stateChanged_date_change; xmlHttp_date_change.open("GET",url_date_change,true); xmlHttp_date_change.send(null); } date_select_sql.php <?php // Includes include('inc_connect.php'); # MySQL Connection header("Expires: Mon, 1 Jan 2000 00:00:00 GMT"); header("Last-Modified: " . gmdate("D, d M Y H:i:s") . " GMT"); header("Cache-Control: no-store, no-cache, must-revalidate"); header("Cache-Control: post-check=0, pre-check=0", false); header("Pragma: no-cache"); // Begin Output Buffering ob_start(); $errors = connect_to_mysql(); if (!$errors) { $sql = 'SELECT * FROM Data_2009 WHERE date = "'.$_GET['selected_date'].'" LIMIT 1;'; $result = mysql_query($sql); while($row = mysql_fetch_array($result, MYSQL_ASSOC)) { $date = strtotime($row['date']); echo '<p>'; echo '<input id="update_date" value="'.$row['date'].'" type="hidden">'; echo '<input style="float: left; margin-right: 10px;" id="update_score" value="'.$row['score'].'" type="text">'; echo '<input style="float: left; margin-right: 10px;" class="submit" type="button" value="Save" onclick="date_save()" />'; echo '</p>'; } mysql_close(); } ?> I've tried to cut my code down to omit the parts that don't relate to this issue. If there are any errors it's probably due to that, as the code does work as I have it now, I just need to add fades, thanks!
-
Hi, I m using a basic function to retrieve and update info in a MySQL database. I have a div in my page with the output is fed into when the request is complete. At the moment, it appears instantly (as soon as the request has loaded) and remains on the page. Is there a way to make this fade out, or even fade in and then fade out, perhaps after about 5 seconds? I think Facebook does a similar thing when you save changes, but I don't seem to be able to link my jquery animation techniques to the output of an AJAX request. If this is possible and someone could explain it that would be a great help. Thanks, Ed
-
[SOLVED] Trying to make PHP/MySQL Instant Messaging, INSTANT
Edward replied to Edward's topic in MySQL Help
Thanks xtopolis, a push system is exactly what I want, I didn't know that's what it was called. I guess I'll keep looking, though understand it's not possible yet with PHP. I have it working pretty well with AJAX so far. Thanks again. -
Hi, I'm creating a one-to-one PHP / MySQL chat feature for my website, so people can chat to me when they visit. I'm using AJAX to try and make this instant, but at the moment I can only make the messages refresh on an interval, i.e. every five seconds. What I want to do is try and make it instant, so that as soon as I send a message to someone, they receive it. I guess I only want it to refresh when there's something new to display. The problem is I don't know how to detect this on the visitor's end. I thought I could loop an SQL command until a message was found with the status 'unread', but looping a SELECT SQL statement seems to cause it to freeze, though it's possible I was doing it wrong. Here is what I am using so far, which refreshes on an interval. Index file: <script type="text/javascript" src="chat_event_view_ajax.js"></script> <script type="text/javascript" src="chat_event_add_ajax.js"></script> <?php include('chat_form.php'); ?> AJAX for Submitting a form, triggering function chat_event_add(): var xmlHttp_chat_event_add; function GetXmlHttpObject_chat_event_add() { var xmlHttp_chat_event_add=null; try { // Firefox, Opera 8.0+, Safari xmlHttp_chat_event_add=new XMLHttpRequest(); } catch (e) { // Internet Explorer try { xmlHttp_chat_event_add=new ActiveXObject("Msxml2.XMLHTTP"); } catch (e) { xmlHttp_chat_event_add=new ActiveXObject("Microsoft.XMLHTTP"); } } return xmlHttp_chat_event_add; } function stateChanged_chat_event_add() { if (xmlHttp_chat_event_add.readyState==4) { document.getElementById("thread").innerHTML=xmlHttp_chat_event_add.responseText; $(function() { $("#thread-container").scrollTo(10000000, 500); }); // TRIGGER THE 'VIEW' AJAX FUNCTION interval_chat_event_view = setInterval('chat_event_view()', 5*1000); // milliseconds between requests } } function chat_event_add() { xmlHttp_chat_event_add=GetXmlHttpObject_chat_event_add(); if (xmlHttp_chat_event_add==null) { alert ("Your browser does not support AJAX!"); return; } var url_chat_event_add="chat_event_add_sql.php"; url_chat_event_add=url_chat_event_add+"?form_chat_from=" + document.getElementById("form_chat_from").value; url_chat_event_add=url_chat_event_add+"&form_chat_alias=" + document.getElementById("form_chat_alias").value; url_chat_event_add=url_chat_event_add+"&form_chat_message=" + document.getElementById("form_chat_message").value; url_chat_event_add=url_chat_event_add+"&rand="+Math.random(); xmlHttp_chat_event_add.onreadystatechange=stateChanged_chat_event_add; xmlHttp_chat_event_add.open("GET",url_chat_event_add,true); xmlHttp_chat_event_add.send(null); // Empty the message input field document.getElementById("form_chat_message").value = ''; } The PHP file, chat_event_add_sql.php, adds a message to the database. The AJAX file, for chat_event_view(), is similar to the above, but links to chat_event_view(), which displays the messages from the database. I hope I have included enough information, so that someone can help me make this instant. Thank you!
-
Actually I didn't fix it, so could anyone help? Sorry!
-
I fixed it by re-starting the View function in another part of the AJAX code, like so: function stateChanged_chat_event_add() { if (xmlHttp_chat_event_add.readyState==4) { document.getElementById("thread").innerHTML=xmlHttp_chat_event_add.responseText; $(function() { $("#thread-container").scrollTo(10000000, 500); }); // Following addition of message, refresh View chat_event_view(); } }
-
Hi, Before I go into too much detail unnecessarily, can anyone tell me why this doesn't work? The first code doesn't, but the second code does, however I would prefer to use the first one if possible. ONLY THE LAST LINE IS DIFFERENT. Doesn't work: function chat_event_add() { # Disable the View function clearInterval(interval_chat_event_view); # Do the AJAX xmlHttp_chat_event_add=GetXmlHttpObject_chat_event_add(); if (xmlHttp_chat_event_add==null) { alert ("Your browser does not support AJAX!"); return; } var url_chat_event_add="chat_event_add_sql.php"; url_chat_event_add=url_chat_event_add+"?form_chat_from=" + document.getElementById("form_chat_from").value; url_chat_event_add=url_chat_event_add+"&form_chat_alias=" + document.getElementById("form_chat_alias").value; url_chat_event_add=url_chat_event_add+"&form_chat_message=" + document.getElementById("form_chat_message").value; url_chat_event_add=url_chat_event_add+"&rand="+Math.random(); xmlHttp_chat_event_add.onreadystatechange=stateChanged_chat_event_add; xmlHttp_chat_event_add.open("GET",url_chat_event_add,true); xmlHttp_chat_event_add.send(null); document.getElementById("form_chat_message").value = ''; # Re-start the View function chat_event_view(); } Does work: function chat_event_add() { # Disable the View function clearInterval(interval_chat_event_view); # Do the AJAX xmlHttp_chat_event_add=GetXmlHttpObject_chat_event_add(); if (xmlHttp_chat_event_add==null) { alert ("Your browser does not support AJAX!"); return; } var url_chat_event_add="chat_event_add_sql.php"; url_chat_event_add=url_chat_event_add+"?form_chat_from=" + document.getElementById("form_chat_from").value; url_chat_event_add=url_chat_event_add+"&form_chat_alias=" + document.getElementById("form_chat_alias").value; url_chat_event_add=url_chat_event_add+"&form_chat_message=" + document.getElementById("form_chat_message").value; url_chat_event_add=url_chat_event_add+"&rand="+Math.random(); xmlHttp_chat_event_add.onreadystatechange=stateChanged_chat_event_add; xmlHttp_chat_event_add.open("GET",url_chat_event_add,true); xmlHttp_chat_event_add.send(null); document.getElementById("form_chat_message").value = ''; # Re-start the View function interval_chat_event_view = setInterval('chat_event_view()', 5*1000); // milliseconds between requests } Thank you!
-
@Mark: I think it was pretty obvious I wouldn't expect that. If you re-read my post you'll see that the problem wasn't getting the image to display, the problem was getting the email to send, which failed when the HTML image tag was included. @blueman378: Hi mate, yes the two sites were hosted by different hosting companies and were subsequently on different servers. One of them worked fine but not the other. I think the one that worked was Linux and the one that failed was Windows. @jkkenzie: Hi, I'm trying to remember what the issue was and how I resolved it, as this post was some time ago (July 2007)! If I remember correctly (which I may not), the problem wasn't specifically relating to the HTML image tag, but to the amount of characters in the email, which was obviously changing when I added or deleted the image tag. I think the issue related to base64 encoding. I don't have much knowledge of this but in short, forward slashes were being added in to my email which were affecting the output. I used the following which I think was the solution: $headers .= "Content-Transfer-Encoding: base64\r\n"; // Add this to your headers $body = rtrim(chunk_split(base64_encode($body))); // Add this to your body mail($recipient, $subject, $body, $headers) // This is how I was sending the mail Perhaps you could try that and see if it helps? If not, let me know and I'll think again.
-
[SOLVED] Counting actual rows in addition to GROUP BY?
Edward replied to Edward's topic in MySQL Help
Geeeeenius! Great, I'l try, thank you! That's new to me, I only just discovered SUM. Thanks again. -
Hi, I have just added a rating system to one of my client's sites, so people can rate the artist's CDs from 1 to 5. Here is an example table: cd_name score CD1 5 CD2 4 CD1 3 CD2 4 CD3 2 CD4 4 On the Home page I want to show some information on the highest rated CD, so I am using this code: <?php $mysql_connection_errors = connect_to_mysql(); if (empty($mysql_connection_errors)) { $sql = 'SELECT cd_name, SUM(rating) FROM my_table GROUP BY cd_name ORDER BY SUM(rating) DESC LIMIT 1;'; $result = mysql_query($sql); while($row = mysql_fetch_array($result, MYSQL_ASSOC)) { $cd_name = $row['cd_name']; $rating = $row['SUM(rating)']; } mysql_close(); } ?> However, I need to prepare for the fact that there may be two or more CDs with the same score, so does anyone know how to also order my the number of rows returned? As far as I am aware, using GROUP BY will always return one, and won't count the number of rows it's grouping? Once I can do this, I will also order by cd_release_date so that if any have the same highest score, AND the same amount of votes (as per example table above), it will display the newest CD. This has me stuck, any help wold be grrrrrrrreat! Thanks, Ed
-
using setInterval to loop, only when user inactive?
Edward replied to Edward's topic in Javascript Help
Hi Corbin and F1Fan, Thank you both for your help. I've now got it working like a charm! I decided to stick with SetInterval though, partly because I have already set my functions up in this way, and partly because of the article I read here (http://www.elated.com/articles/javascript-timers-with-settimeout-and-setinterval/) which is in favour of it, which is what I found on Google when I was first trying to find out how to run looped functions. Here is an example of my finished code, in case it helps anyone else. window.onload = function() { chat_event_view(); interval_chat_event_view = setInterval('chat_event_view()', 5000); }; function chat_event_view() { // View messages } function chat_event_add() { clearInterval(interval_chat_event_view); // Add message then view messages interval_chat_event_view = setInterval('chat_event_view()', 5000); } Thanks very much again to you both! -
Hi, I am building an instant messaging system into a website, and am looping an ajax function to retrieve messages from the datase, as follows: var interval window.onload = function() { chat_event_view(); interval = setInterval('chat_event_view()', 10*1000); // 10 secs between requests }; However, when the user clicks 'submit' to add their message, it also retrieves the messages from the database. What I want to avoid is the chat_event_view() function running within 10 seconds of adding a message. Does anyone know if there is a way to stop the chat_event_view() function when the add() function is executed, and then resume it after 10 seconds? This way "new" messages could be styled differently for 10 seconds when they are first retrieved/read. Thank you.
-
[SOLVED] Select query not finding results where searching for number?
Edward replied to Edward's topic in MySQL Help
Thanks Thorpe, I renamed the column and it's working fine. I knew it had to be possible but I had no idea why it wasn't working. I have got error handling in place but I stripped it out to find the problem, I'll put it back in now. Thanks very much, I've learned something today! -
Hi, I'm using a select query to find rows which have the 'from' field matching $_SESSION['id']. I know there are matches, as I have compared the two in a separate IF code, but no matches are coming up. Does anyone know why?? Here is the code: $sql = 'SELECT * FROM chat_messages WHERE \'from\' = "'.$_SESSION['id'].'" ORDER BY date ASC;'; $result = mysql_query($sql); while($row = mysql_fetch_array($result, MYSQL_ASSOC)) { $chat_message_from = $row['from']; $chat_message_alias = $row['alias']; $chat_message_date = $row['date']; $chat_message = $row['message']; echo '<p>'; if (empty($chat_message_from)) { echo 'Guest 1'; } else { echo $chat_message_from; } echo ' on '.$chat_message_date.': '.$chat_message.'</p>'; } The above outputs no matches, however if I run the following, the word 'MATCH' outputs, which means the two values being compared above must be the same! $sql = 'SELECT * FROM chat_messages ORDER BY date ASC;'; $result = mysql_query($sql); while($row = mysql_fetch_array($result, MYSQL_ASSOC)) { $chat_message_from = $row['from']; $chat_message_alias = $row['alias']; $chat_message_date = $row['date']; $chat_message = $row['message']; echo '<p>Session: '.$_SESSION['id'].'</p>'; echo '<p>From: '.$chat_message_from.'</p>'; if ($_SESSION['id'] == $chat_message_from) { echo '<p>MATCH!</p>'; } } Is it not possible to search for numeric values or something?!
-
Getting confused with quotes (single and double) in databases
Edward replied to Edward's topic in MySQL Help
Hi, Thanks for your comments. I understand what you are saying, but I am struggling to understand why we use mysql_real_escape_string() instead of htmlentities($var, ENT_QUOTES) - do they do the same thing?! Anyway, my real problem is, in this particular case, I am firstly entering data by 'importing' a table, with the following code as a text: CREATE TABLE `test` ( `id` int(11) NOT NULL auto_increment, `title` varchar(500) collate latin1_general_ci NOT NULL, `text` text collate latin1_general_ci NOT NULL, KEY `id` (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci COMMENT='A test table' AUTO_INCREMENT=2 ; INSERT INTO `test` VALUES(1, 'Some Text', '<p style="font-weight: bold;">text<p>test</p><p>a single quote \' is here and a <a href="http://www.website.com/">link</a> is here</p>'); But this is causing a problem as I am unable to edit the table following import, presumably because there is a problem somewhere. If I remove the value with the quotes, it works fine. Do you know what I'm doing wrong? -
Hi, I'm getting confused over quotes in databases. I'm entering the values manually, and some of them contact html. When I enter single and/or double quotes in my values, it seems to crash the database and stops mt editing it, but when I type in HTML entity equivilents, it stops the HTML displaying properly. Does anyone know what I should be doing? An example value could be: <div style="width: 100px;">I'm a website.</div> Thank you!!
-
Hi, I'm starting to learn AJAX and I have completed a simple tutorial online which shows me how to action a form with AJAX. The result is that when the used clicks on a radio button to select their answer to a question, I am able to display the word 'correct' if the user chose the correct answer. However, I want to be able to make the word 'correct' fade in with jQuery. I know how to do this normally, but not by combining it with AJAX. Here is my code: <html> <head> <script type="text/javascript" src="js/jquery-1.2.6.min.js"></script> </head> <body> <script type="text/javascript"> var xmlHttp function GetXmlHttpObject() { var xmlHttp=null; try { // Firefox, Opera 8.0+, Safari xmlHttp=new XMLHttpRequest(); } catch (e) { // Internet Explorer try { xmlHttp=new ActiveXObject("Msxml2.XMLHTTP"); } catch (e) { xmlHttp=new ActiveXObject("Microsoft.XMLHTTP"); } } return xmlHttp; } function stateChanged() { if (xmlHttp.readyState==4) { document.getElementById("txtHint").innerHTML=xmlHttp.responseText; } } function showHint(str) { xmlHttp=GetXmlHttpObject(); var url="results.php?"; url=url+"answer="+str; url=url+"&sid="+Math.random(); xmlHttp.onreadystatechange=stateChanged; xmlHttp.open("GET",url,true); xmlHttp.send(null); } </script> <form id="myForm"> <p>Q1 - House Number</p> <p><input type="radio" name="house_number" id="q1a1" value="36" onclick="showHint('q1a1')"> 36</p> <p><input type="radio" name="house_number" id="q1a2" value="37" onclick="showHint('q1a2')"> 37</p> <p><span id="txtHint"></span></p> <p id="test" style="display: none;">Correct!</p> </form> </body> </html> <?php header("Cache-Control: no-cache, must-revalidate"); header("Expires: Mon, 26 Jul 1997 05:00:00 GMT"); if ($_GET['answer'] == 'q1a1') { ?> <script type="text/javascript"> $(document).ready(function(){ $('#test').animate({opacity: '1'}, 500); }); </script> <?php echo '1 out of 1'; } else { echo '0 out of 1'; } ?> If the answer is correct, I want the item with the id 'test' to fade it, but it doesn't show up at all. Can anyone see what I'm doing wrong? As I'm new to AJAX, perhaps I'm taking the completely wrong approach? Thank you for any help you can offer.
-
Hi, I'm using a Flash component to create a flip book. Sometimes, I want the book to turn to a specific page when it's loaded, and I'm using the URL to initiate this, i.e. index.php?page=25. I can get this to work, but unfortunately everytime the page is changed manually, it runs the function and goes back to page 25. I've tried to set up a variable to stop this happening after the first time, and I've also tried overwriting the page variable, but to no avail. Can anyone think how this can be fixed? Here are the codes I've tried: onEnterFrame = function() { if (goto == 25) { if( zoomed )return; myBook.flipGotoPage(25); } } stop(); onEnterFrame = function() { if (goto_void != 1) { if (goto == 25) { if( zoomed )return; myBook.flipGotoPage(25); } } $goto_void = 1; } stop(); Perhaps I shouldn't be using onEnterFrame, but I don't know what else there is to choose from? I guessed and tried 'onLoad' and '_root.onLoadl' but that didn't work at all. Please can someone help?
-
substr to limit characters, rounding to nearest space?
Edward replied to Edward's topic in PHP Coding Help
Hi, That seems to be working great, thanks! I did actually make two minor amendments, which I think change it to how I wanted it. Perhaps you could check that what I've done is ok?? $blurb = ""; $blurb_len = 97; // max number of characters allowed in the blurb $ed_parts = explode(" ",$acc_desc); foreach ($ed_parts AS $a_word) { // Leave if this word breaks our strlen limit if (strlen($blurb . "$a_word") > $blurb_len) { // I REMOVED THE SPACE BEFORE $a_word SO THAT A WORD WITH n AMOUNT OF CHARACTERS WILL FIT IN A GAP OF n CHARACTERS break; } $blurb .= "$a_word "; } // if no period on the end, add ellipsis $blurb = trim($blurb); if (substr($blurb, -1, 1) != '.') { $blurb .= "..."; // I REMOVED THE SPACE BEFORE THE ELLIPSES } Thanks again! -
Hi, I am using the following code to display a preview of a long variable, like so: if ((strlen($blog_article)) > 100) { $blog_article_preview = substr($blog_article,0,97).'...'; } However, I don't want the preview value to end during a word. Is there a way to limit the characters at the most recent space? i.e. 'Welcome to my new websi...' would become 'welcome to my new'. Thanks in advance.
-
Retrieving a variable from a parent movie in Flash
Edward replied to Edward's topic in Other Programming Languages
I've now managed to get it work but not completely. I was defining my variable within a function so it wasn't global. My defining it outside a function I am able to access it with the following code. test_txt.text = _root.current_page; However, I NEED to define the 'current_page' variable within a function. How can I do this but still make it global? -
Hi, I am using a flash movie with a compentent on the canvas at root level called 'myBook'. Within the component I am loading other flash movies. On my root canvas, I have a text field (instance name 'display_txt') and I am able to create a variable called 'res' and display the value of it in my text field using the script below: res = hello; display_txt.text = res; But I can't get the value of it to display inside the embedded movie, in the text field with the instance name 'test_txt'. Does anyone know how to do this? Is my variable available or do I need to make it global? I've tried the following: test_txt.text = res; test_txt.text = _root.res; test_txt.text = _parent.res; test_txt.text = level0.res; Thanks for any help you can offer.
-
[SOLVED] mysql timestamp column displays as 01/01/1970 - why?
Edward replied to Edward's topic in PHP Coding Help
Thank you all! I've used this which works: $date = strtotime($row['date']);[code] Thanks Ken. PFMaBiSmAd, I trust you have no objections to this method, i.e. using TIMESTAMP in my table and converting it with strtotime() before displaying it on my site? I'm not sure if you're saying I should use DATE_FORMAT over TIMESTAMP? Thanks again! [/code] -
[SOLVED] mysql timestamp column displays as 01/01/1970 - why?
Edward replied to Edward's topic in PHP Coding Help
So far I've only created the rows in PHP MyAdmin and the timestamps have been created automatically. I've just checked the value in the table and it looks fine. 2008-07-01 13:24:45 -
Hi, I have a MySQL table which includes a TIMESTAMP column to record the time and date a comment was posted on a blog. When I display the TIMESTAMP on the site, I am formatting it in the same way I would with date(), but it's not using the database value, it's using 1st Jan 1970. I know this date has some significance, but I'm not sure why it's showing up here. while($row = mysql_fetch_array($result, MYSQL_ASSOC)) { $date = $row['date']; echo '<p>Date: '.date(l.', '.jS.' '.F.' '.Y, $date).'</p>'; } Does anyone know what I've done wrong? Thank you.