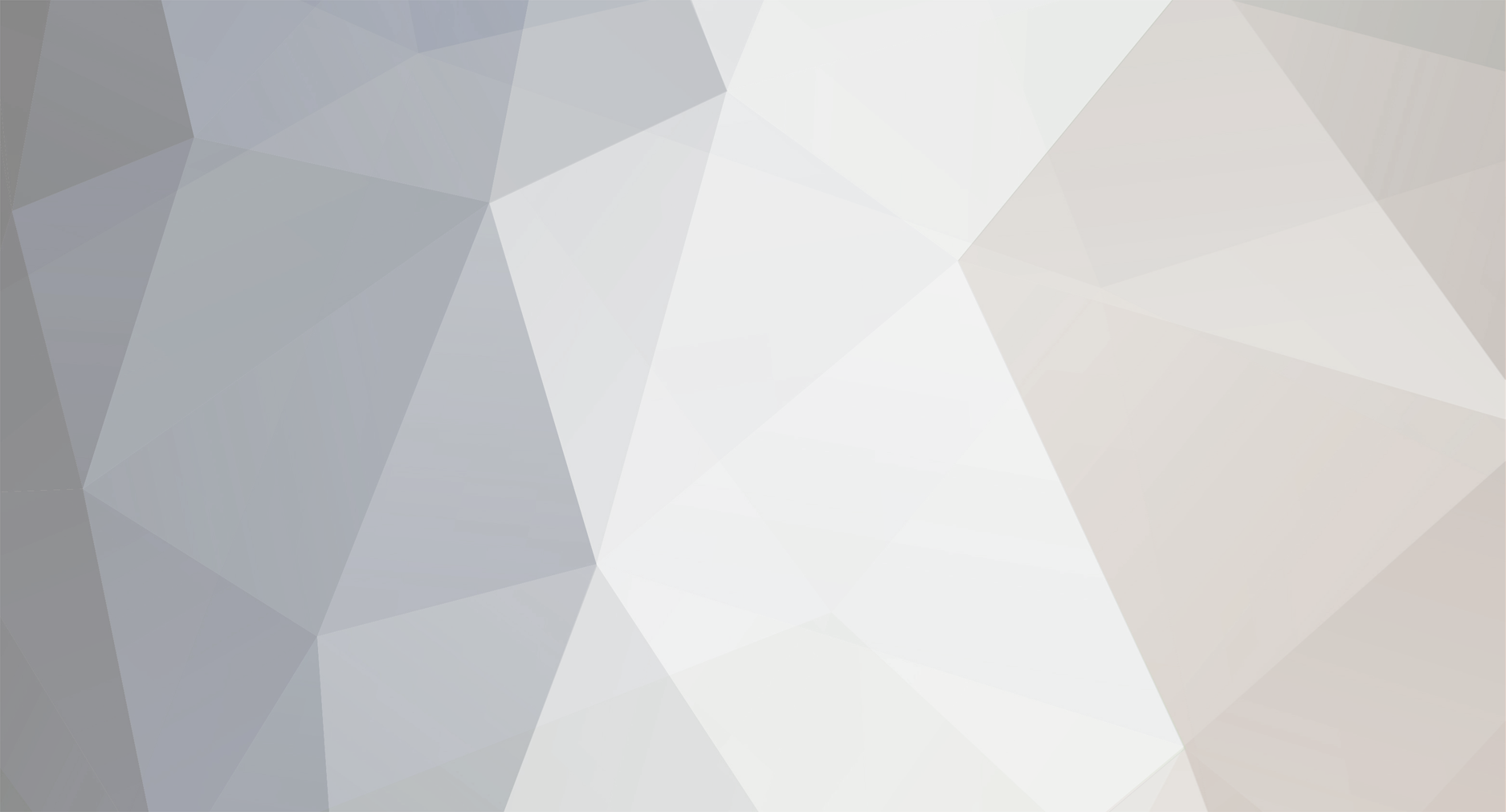
$cripts
-
Posts
33 -
Joined
-
Last visited
Never
Posts posted by $cripts
-
-
i found this a while back dont remeber where from but even if magic quotes is enabled u still need to check everything a user inputs this will filture out everything
function cleanstring($string)
{
$search = array ('@<script[^>]*?>.*?</script>@si', // Strip out javascript
'@<[\/\!]*?[^<>]*?>@si', // Strip out HTML tags
'@([\r\n])[\s]+@', // Strip out white space
'@&(quot|#34);@i', // Replace HTML entities
'@&(amp|#38);@i',
'@&(lt|#60);@i',
'@&(gt|#62);@i',
'@&(nbsp|#160);@i',
'@&(iexcl|#161);@i',
'@&(cent|#162);@i',
'@&(pound|#163);@i',
'@&(copy|#169);@i',
'@(\d+);@e'); // evaluate as php
$replace = array ('',
'',
'\1',
'"',
'&',
'<',
'>',
' ',
chr(161),
chr(162),
chr(163),
chr(169),
'chr(\1)');
return preg_replace($search, $replace, $string);
}
-
try this
$timestamp = mktime($hour,$minute,$second,$month,$day,$year);
-
long way...
create on long if statement for it using an array for each element ( days ) for each sign and months
-
you can use this script i wrote a simple little pagination but it uses a range so u can put a range of 4 and it go 1 2 [ 3 ] 4 5
function pages($url,$total_items,$per_page,$range,$current_page) { // Figure out the total number of pages. Always round up using ceil() $total_pages = ceil($total_items / $per_page); $range = ceil($range / 2); if($current_page != 1) { $return = '[<a href="'.$url.'p=1" title="Go to page 1 of '.$total_pages.'"> « </a>]'; } if($current_page > 1){ $prev = ($current_page - 1); $return .= '<a href="'.$url.'p='.$prev.'" title="Go to page '.$prev.' of '.$total_pages.'"> « </a>'; } // Build Backward Links for($i = 1; $i <= $range; $i++){ $p = $current_page-$i; if($p > 0) { $back .= $p.','; } } $back_rev = strrev($back); $back_rev = explode(',',$back_rev); foreach($back_rev as $rev) { $rev = strrev($rev); $return .= ' <a href="'.$url.'p='.$rev.'" title="Go to page '.$rev.' of '.$total_pages.'">'.$rev.'</a> '; } $return .= ' [ <b>'.$current_page.'</b> ] '; for($i = 1; $i <= $range; $i++){ $p = $current_page+$i; if($p <= $total_pages) { $return .= '<a href="'.$url.'p='.$p.'" title="Go to page '.$p.' of '.$total_pages.'">'.$p.'</a> '; } } // Build Next Link if($current_page < $total_pages && $total_pages != '0') { $next = $current_page + 1; $return .= '<a href="'.$url.'p='.$next.'" title="Go to page '.$next.' of '.$total_pages.'"> » </a>'; } if($current_page != $total_pages && $total_pages != '0') { $return .= '[ <a href="'.$url.'p='.$total_pages.'" title="Go to page '.$total_pages.' of '.$total_pages.'"> » </a> ]'; } return $return; }
-
-
anyone got some ideas?
-
i really see no point in doing this but try caching
-
you could run a cronjob for this and store that variable for later use if thats what your trying to do
-
u can still use pagination simply do it like this
$query = mysql_query('SELECT ....... LIMIT 5,20'); while(.........) { $array[$id] = $idortext; } shuffle($array); foreach($array ...... ) { echo ......; }
the only thing about using shuffle is if you need to keep the array keys they will be changed
-
get all values put them into an array then output them using shuffle
-
because ini_get will only retrieve the value of a php setting ini_get(setting_name);
u need to use ini_set(setting_name,value);
-
try this
asd; print_r(error_get_last());
and see if it outputs anything
if it doesnt work put this at the top of your page and try again
error_reporting(E_ALL ^ E_NOTICE);
and try again
-
put it in the uploader script it will change the maximum upload size in the php.ini file
and it will be ini_set('upload_max_filesize',whateversizeinbytes);
it will need to be in the upload script because once the script is done executing it will reset back to its default value
-
header("form1.php?package={$_GET['package']}&pack={$_GET['pack']}");
-
Ok for some reason this cookie wont keep the array values and it will simply reset to only on value in the array
$shopitems = (isset($_COOKIE['shop_items'])) ? unserialize($_COOKIE['shop_items']) : array(); if(!$_GET['itemid'] || !is_numeric($_GET['itemid'])) { } else { $itemid = $_GET['itemid']; $shopitems[$itemid] = $itemid.',1'; setcookie('shop_items',serialize($shopitems),0,'',''); }
wut this should do is when there is an itemid it will put it in the array of shopitems serialize it and set the cookie for later use the problem is that when there is allready an array serialized in the cookie it will not take that array values
so instead of saying there is allready a value in the array of shopitems[2] and i want to add shopitems[4] it will simply use shopitems[4] to set the array
And i tested it out on this which does the same thing and it works perfetcly fine....
$forum_review = (isset($HTTP_COOKIE_VARS['forum_view'])) ? unserialize($HTTP_COOKIE_VARS['forum_view']) : array(); $start = (!$_GET['start']) ? '0' : $_GET['start']; $enter = $start + 10; while($start < $enter) { $forum_review[$start] = '2'; setcookie('forum_view',serialize($forum_review),0,'',''); $start++; }
-
print_r will print out an array so we can read it.....
wut i need to do is implode this array into games|team,gameid so i can go back later and explode it back into its original array
-
I have An Array
Array ( [0] => games,
[teams] => Array ( [0] => gameid )
);
The problem im having is I made a function to implode this array
foreach($array as $parent) { if(is_array($parent)) { while(list($key, $value) = each($parent)) { echo $key.value; } } else { echo $parent; } }
From this i can only get 0,gameid,games While i need to get team,gameid,games
and i cannot fiqure out how to do this....
-
no im running from the server side but i need to use this for a pagination script as i call it from a function and it creates the url and i need to remove the page= argument
-
ok.......well sense ur not helping me.....
does it matetr what i use it for?
i need a way to proccess the url arguments and remove the page arument from it
so i would have
like test.php?f=view&t=1&page=5&sort=ASC
$arguments = $_SERVER['argv'];
// code to remove certain arg
so i now have
test.php?f=view&t=1&sort=ASC
and i need to do it from calling a function -
what do u mean??
i want to check the $_SERVER['argv'] if page=somethign exists if it does it want to remove it from the $_SERVER['argv'] variable and stick the argv variable at the end a link so id have
test.php?this=that&page=3
become
test.php?this=that
without useing $_GET or $_REQUEST -
Ok i have test.php?p=userr&f=signature&page=2
how would i check if the URL contains page= argument and get rid of it if it does exist -
How do u go about making zip files of folders in php say i wanted to zip the folder /work on my site
or just basically zip files..... -
o i fixed the prob it was being encoded by phpdesigner....weird....
yall should make it so we can change the topic by adding solved :) -
Why's this happening?
[a href=\"http://www.team-ki.com/csys/files/classes/user.php\" target=\"_blank\"]http://www.team-ki.com/csys/files/classes/user.php[/a]
switch problem in firefox
in PHP Coding Help
Posted
need to explain ur problem a bit better and u have no default value....