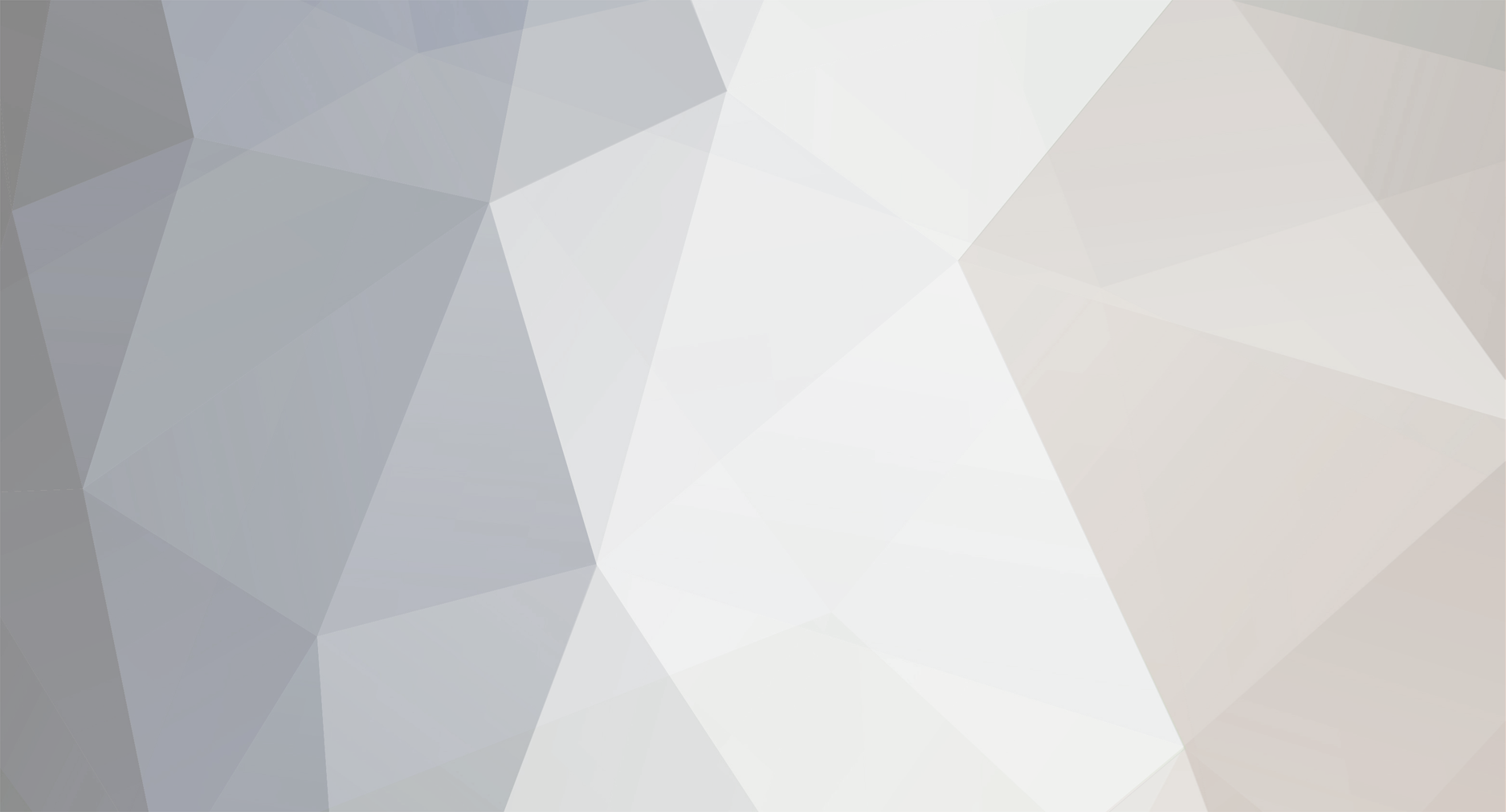
MaaSTaaR
-
Posts
26 -
Joined
-
Last visited
Posts posted by MaaSTaaR
-
-
Use mod_rewrite to do that
There are a lot of tutorials that explain mod_rewrite, just use Google.
-
I'm not sure how CodeIgniter works but I think you need func_get_args : http://php.net/manual/en/function.func-get-args.php
-
In these lines :
$sql = "UPDATE orders SET sent_order_confirm = 'yes' WHERE order_num = $order_num"; $result=mysql_query($sql);
Change the variable name "$result" to anything else.
Example :
$sql = "UPDATE orders SET sent_order_confirm = 'yes' WHERE order_num = $order_num"; $update=mysql_query($sql);
-
Hello
Before this line : while ($row = mysqli_fetch_array($result))
Add : $items = array();
-
Try to put it before this line : $dir_length = count($list);
-
Hello ...
try :
echo "\"First name\",\"belong's to\"";
-
Useful information, Thanks.
autocomplete="off" - but its not universally supported.
Gecko support it? (http://developer.mozilla.org/en/docs/How_to_Turn_Off_Form_Autocompletion)
-
Hello ...
use "while" to get all results :
while ($info = mysqli_fetch_array($result)) { echo $info['email'] . '<br />'; }
-
Hello ...
In the head of submit page add :
print_r($_POST);
and tell us what is the result.
-
Hello ..
I don't know if I understood you correctly or not, but you may want to do something like that :
if (!$get_email) { die("You are missing some information!"); }
-
Hmmm , i think you need to hacking PHP code to do something like that , becuase it's a statement and not function .
you can do it with PHP by compile it , i mean write function open the php source file and search the "loop" statement in the php source file , if find the statement replace it by real loop statement like "while" or "for" , i mean do something like templates engine (for example Smarty) . -
i know a very very bit of assembly , and i plan to be good in it but it think it will need very long time .
-
i don't know if i understand you correctly , but as i understand you want to unactive some accounts when their users aren't in the company .
If i right , i think you have dual solution , the first solution is unactive these accounts after X clock , i mean in the login script you can check server time , if the server time is 3 PM or high stop the log in , otherwise if the time is between 8 AM to 2 PM login without any problem .
the second solution you can do it if you have Unix server by Corn jobs . -
try this :)
[code=php:0]
<?php
$string = '<td width="50%" class="playerbio">
<b>Height/Weight: </b> 6-6/229<br>
<b>Birthdate: </b> 04/22/1983<br>
<b>Birthplace: </b> Fort Smith, AR, USA<br>
</td>
<td width="50%" class="playerbio">
<b>Team: </b> Jacksonville Jaguars<br>
<b>College: </b> Arkansas<br>';
$search = "~</b>\s*(.*?)\s*<br>~ise";
$replace = "replace('\\1')";
$do_search = preg_replace($search,$replace,$string);
function replace($text)
{
echo $text . '<br />';
}
?>
[/code]
try this page : http://gnosis.cx/publish/programming/regular_expressions.html it's great :) -
Hello ...
great it's work fine now thanks :) , i have another question please .
in the article (http://www.zend.com/php/internals/extension-writing1.php) the writer said "After running each of these commands, you should have a hello.so file in ext/hello/modules/" , The problem is i don't have hello.so i have hello.o only , so how i can generating the hello.so to use it as php extension ? -
Hello ...
sorry for my bad English .
My os is Ubuntu Linux and i have Bison 2.3 , Flex 2.5.31 , GCC 4.0.3 .
i don't have any problem with compile php , but my problem exactly is i get this problem from config.m4 file :
maastaar@MaaSTaaR:~/php-4.4.4/ext/hello$ ./config.m4
./config.m4: line 1: syntax error near unexpected token `hello,'
./config.m4: line 1: `PHP_ARG_ENABLE(hello, whether to enable Hello World support,'
config.m4 :
PHP_ARG_ENABLE(hello, whether to enable Hello World support,
[ --enable-hello Enable Hello World support])
if test "$PHP_HELLO" = "yes"; then
AC_DEFINE(HAVE_HELLO, 1, [Whether you have Hello World])
PHP_NEW_EXTENSION(hello, hello.c, $ext_shared)
fi
hello.c :
#ifdef HAVE_CONFIG_H
#include "config.h"
#endif
#include "php.h"
#include "php_hello.h"
static function_entry hello_functions[] = {
PHP_FE(hello_world, NULL)
{NULL, NULL, NULL}
};
zend_module_entry hello_module_entry = {
#if ZEND_MODULE_API_NO >= 20010901
STANDARD_MODULE_HEADER,
#endif
PHP_HELLO_WORLD_EXTNAME,
hello_functions,
NULL,
NULL,
NULL,
NULL,
NULL,
#if ZEND_MODULE_API_NO >= 20010901
PHP_HELLO_WORLD_VERSION,
#endif
STANDARD_MODULE_PROPERTIES
};
#ifdef COMPILE_DL_HELLO
ZEND_GET_MODULE(hello)
#endif
PHP_FUNCTION(hello_world)
{
char *str;
str = estrdup("Hello World");
RETURN_STRING(str, 0);
}
php_hello.h :
#ifndef PHP_HELLO_H
#define PHP_HELLO_H 1
#define PHP_HELLO_WORLD_VERSION "1.0"
#define PHP_HELLO_WORLD_EXTNAME "hello"
PHP_FUNCTION(hello_world);
extern zend_module_entry hello_module_entry;
#define phpext_hello_ptr &hello_module_entry
#endif
what is the problem , and how i can solve it ?
-
woow ! Great idea :)
-
if you want the emails store in the variable like 'em@em1.com,em@em2.com' try this :
[code]
$query = mysql_query('SELECT * FROM table');
$mails = '';
while ($r = mysql_fetch_array($query))
{
$mails .= $r['Email'] . ',';
}
[/code] -
if you want to make an array values the emails you should do something like this :
[code=php:0]
<?php
$emails = array();
$query = mysql_query('SELECT * FROM table');
while ($r = mysql_fetch_array($query))
{
$emails[] = $r;
}
?>
[/code]
then you will have $emails array which store all email , now try to print one of these emails :
[code=php:0]
<?php
echo $emails[0]['Email']
?>
[/code]
so use "for" or "while" loop :) -
When you make form in (X)HTML like this :
[code]
<form metod="post" action="page.php">
<input name="b" type="text" />
</form>
[/code]
note the method in form tag :) it's post , so any "input" in this form will save in $_POST array .
try :
[code]print_r($_POST);[/code]
in page.php :) -
hmmm , ok better to use mysql_query not mysql_db_query , try .
and try to add echo '<h1>test</h1>'; above the query , and see will the page print "test" ? -
SCREEM - http://www.screem.org/ :)
-
Ok , try "LIKE" as alternative for "="
[code=php:0]$query="SELECT * FROM Assembly WHERE PARTNUMBER='$search'";[/code]
to
[code=php:0]$query="SELECT * FROM Assembly WHERE PARTNUMBER LIKE '%" . $_POST['search'] . "%'";[/code] -
and better to write this line
[code=php:0]$query="SELECT * FROM Assembly WHERE PARTNUMBER='$search'";[/code]
like this
[code=php:0]$query="SELECT * FROM Assembly WHERE PARTNUMBER='" . $_POST['search'] . "'";[/code]
i mean use $_POST :) mybe you register_globals is off in php.ini
Check array if there is nothing returned
in PHP Coding Help
Posted
You can use empty() to check if $item1 and $item2 are empty as the following :