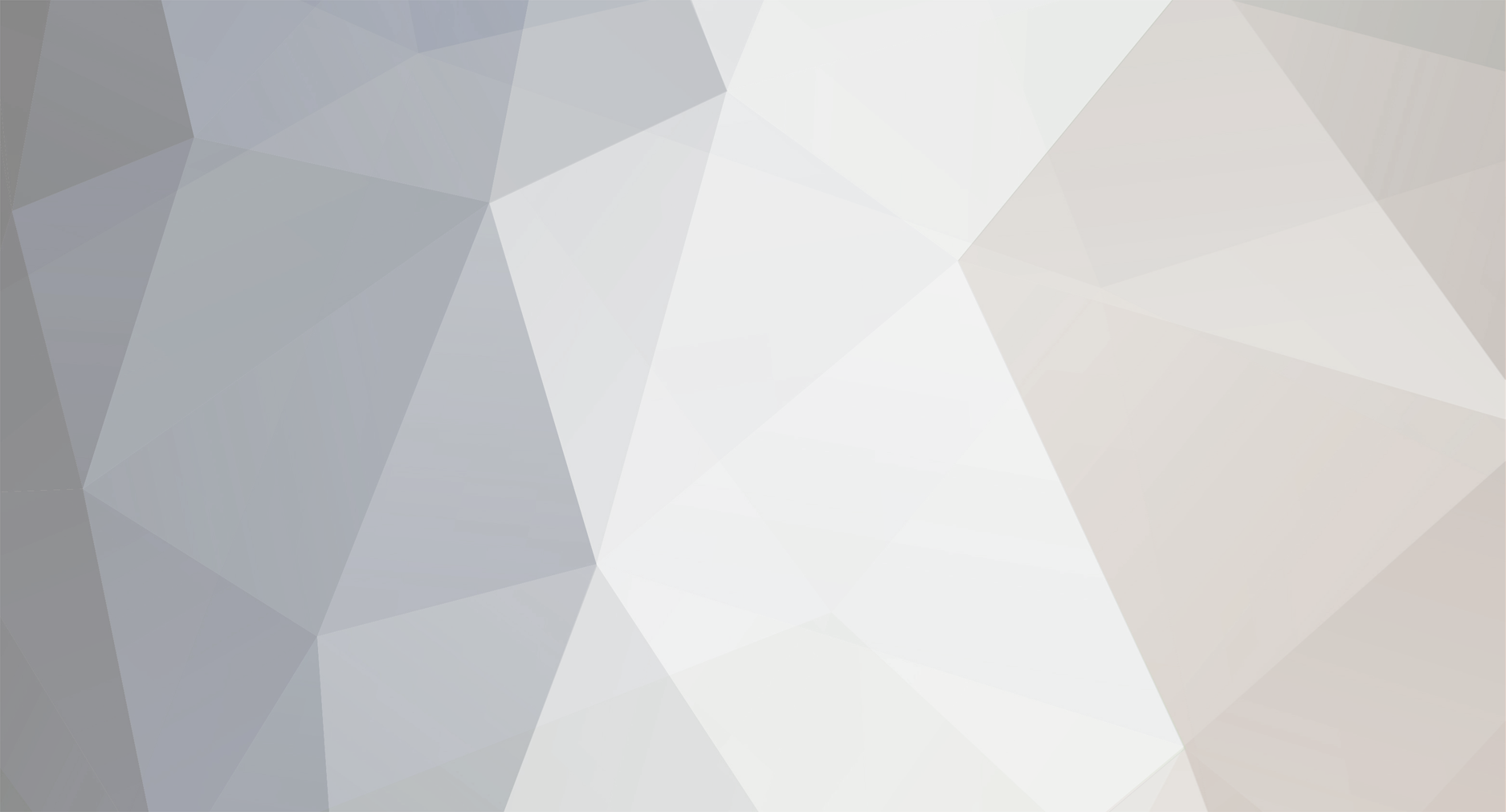
BRUm
-
Posts
28 -
Joined
-
Last visited
Never
Posts posted by BRUm
-
-
Nevermind, fixed it with require_once.
-
Argh stupid me! I don't even have a `users` table lol I changed it to `data`.
Can anyone tell me what this error means:
Fatal error: Cannot redeclare class database in connect.php on line 20
-
OK Here's the code:
<?php #Connect.php //Class db connection class database { var $cnx; public function database ( $host, $user, $pass, $db ) { //Run connect info here $this->cnx = mysql_connect($host,$user,$pass); mysql_select_db($db,$this->cnx); } public function query($x) { $query = mysql_query($x,$this->cnx) or die('SQL: '.$x.'<br />Error: '.mysql_error()); $result = mysql_fetch_array($query); return $result; } } $db = new database('localhost','civ_admin','password','civ_db'); ?>
<?php #Calling code - classes.php include "connect.php"; //All classes placed and called from here //Test query function in database class print_r($db); print_r($db->query("select * from `users` where `username` = 'admin'")); ?>
The DB structure is nothing special. It's meant to call the table `users` within the `civ_db` database, and retrieve the row where `user` = `admin`.
-
The name isn't important, I changed the name slightly for the nature of my code ^^
But nevertheless, it's giving me that error when the DB and table given to connect to are correct.
-
OK when I implement error handling it gives me this very strange error:
Error: Table 'civ_table.users' doesn't existI don't have a clue where the .users has come from :/
-
OK I've implemented the code as you've shown, yet I still get an SQL resource error:
Warning: mysql_fetch_array(): supplied argument is not a valid MySQL result resource in .../connect.php on line 31
Connect.php
<?php //Class db connection class database { var $cnx; public function database ( $host, $user, $pass, $db ) { //Run connect info here $this->cnx = mysql_connect($host,$user,$pass); mysql_select_db($db,$this->cnx); } public function query($x) { $query = mysql_query($x,$this->cnx); $result = mysql_fetch_array($query); //This is line 31 return $result; } } $db = new database('localhost','***','***','***'); ?>
The script using the class above:
<?php include "connect.php"; //All classes placed and called from here //Test query function in database class print_r($db->query("select * from `users` where `username` = 'admin'")); ?>
-
Thank you very much for all your helps guys.
-
Thanks for your help. Is there a way to omit the database connection within the class? It would cause quite a memory loadon my server to have a new connection established every time the class is used.
-
Ah I see. So does this mean I'm going to have place a new DB connect in the class? Wouldn't this mean every time I run the class, it will start a new DB connection?
This is my error:
Warning: mysql_fetch_array(): supplied argument is not a valid MySQL result resource in .../classes.php on line 13
-
I've recently started learning OOP, and I have a rather simple problem, yet I don't know how to solve it. Obviously I'm using a certain aspect of OOP wrong:
<?php include "connect.php"; //All classes placed and called from here class database { public function query($x) { $query = mysql_query($x); print_r($query); $result = mysql_fetch_array($query); } } //Test above query $database = new database(); $database->query("select * from `users` where `username` = 'admin'"); print $result[0]; print $result[1]; ?>
It gives me an sql error regarding line 13, so it seems it's not parsing the sql query I'm using the $database->query().
Thanks for any help in advance.
-
Hello everyone,
I run a small MMORPG and I was wondering if any gurus around here would be so kind so as to share some wisdom
We run a script which is executed hourly by CRON. This script cycles through all ~5,200 of our members and updates their various in-game statistics etc. The problem is, we're hosted on a good server, but it's still putting quite excessive load on its resources. At the moment, the script takes 160-170 seconds to complete, and we recently managed to reduce the execution time by 10 seconds after we added
mysql_free_result();
Now, this isn't bad, a few months ago we began optimising and managed to get it from 10-20 minutes! Crazy.
Anyway, the script is posted below, I'd be very grateful if anyone could share some tips, tricks or other SQL functions to help us get the time down even more.
[Warning the script is very long]
1. <? 2. INCLUDE "db_connect.php"; 3. $time1=time(); 4. 5. $ol_y = mysql_query("select * from overlords where place = 'yuaktz'"); 6. $y_t=mysql_result($ol_y,0,"tax"); 7. 8. $ol_s = mysql_query("select * from overlords where place = 'shleincyrodil'"); 9. $s_t=mysql_result($ol_s,0,"tax"); 10. 11. $ol_gp = mysql_query("select * from overlords where place = 'great_plains'"); 12. $gp_t=mysql_result($ol_gp,0,"tax"); 13. 14. $ol_wp = mysql_query("select * from overlords where place = 'western_province'"); 15. $wp_t=mysql_result($ol_wp,0,"tax"); 16. 17. $select_weather = mysql_query("select * from `weather`"); 18. while($weather=mysql_fetch_array($select_weather)) 19. { 20. $y_w=$weather['yuaktz']; 21. if($y_w== "rain"){$y_c= 1.1;} 22. if($y_w== "sunny"){$y_c= 1.15;} 23. if($y_w== "over cast"){$y_c= 1;} 24. $s_w=$weather['shleincyrodil']; 25. if($s_w== "rain"){$s_c= 1.1;} 26. if($s_w== "sunny"){$s_c= 1.15;} 27. if($s_w== "over cast"){$s_c= 1;} 28. if($s_w== "frosty"){$s_c= 0.75;} 29. $gp_w=$weather['great_plains']; 30. if($gp_w== "sunny"){$gp_c= 1.15;} 31. if($gp_w== "over cast"){$gp_c= 1;} 32. $wp_w=$weather['western_province']; 33. if($wp_w== "rain"){$wp_c= 1.1;} 34. if($wp_w== "frosty"){$wp_c= 0.75;} 35. } 36. 37. ###################### SELECTING USERS ####################################### 38. $select_users=mysql_query("SELECT * FROM data WHERE bann='0' AND active > '0' "); 39. while($sel_users=mysql_fetch_array($select_users)) 40. { 41. $username=$sel_users['username']; 42. $user_hashed=$sel_users['username_hashed']; 43. $user_net=$sel_users['netscore']; 44. $user_pop=$sel_users['pop']; 45. $user_xp=$sel_users['xp']; 46. $user_money=$sel_users['money']; 47. $user_pop=$sel_users['pop']; 48. $user_place=$sel_users['place']; 49. $user_turns=$sel_users['turns']; 50. $user_food=$sel_users['food']; 51. 52. if($user_place=='yuaktz'){$tax=$y_t/100;} 53. if($user_place=='shleincyrodil'){$tax=$s_t/100;} 54. if($user_place=='great_plains'){$tax=$gp_t/100;} 55. if($user_place=='western_province'){$tax=$wp_t/100;} 56. ############ CIV UNREST ################################################################## 57. $select_user_units=mysql_query("SELECT * FROM units WHERE username_hashed='$user_hashed' "); 58. while($sel_units = mysql_fetch_array($select_user_units)) 59. { 60. $user_hi = $sel_units['heavy_infantry']; 61. $user_mi = $sel_units['medium_infantry']; 62. $user_li = $sel_units['light_infantry']; 63. $user_ww = $sel_units['war_weariness']; 64. $user_as = $sel_units['army_str']; 65. } 66. 67. $army_req = ((sqrt($user_net) + $user_pop) - ($user_as + sqrt($user_xp))); 68. if($army_req<0){$army_req=1;} 69. if($user_as<$army_req){$civil_unrest =number_format(($user_as/$army_req),'2');}else{$civil_unrest=1;} 70. if($user_as==0 || $user_as<0){$civil_unrest=0;} 71. ############################################################################################### 72. 73. $select_user_labs=mysql_query("SELECT * FROM buildings WHERE username_hashed='$user_hashed' "); 74. while($sel_lab = mysql_fetch_array($select_user_labs)) 75. { 76. $user_lab = $sel_lab['lab']; 77. $user_farms = $sel_lab['farms']; 78. $user_homes = $sel_lab['home']; 79. } 80. 81. $profit=$user_pop*100*(1-$user_ww); 82. $costs=$user_hi*100+$user_mi*50+$user_li*10; 83. $gain=($profit-$costs)*$civil_unrest; 84. $gain_taxed=$gain*(1-$tax); 85. $gain_over=$gain*$tax; 86. //////////// OVERLORD CASH AND WEATHER BONUSES 87. if($user_place=='yuaktz'){$oy_money=$oy_money+$gain_over;$food_bonus=$y_c;} 88. if($user_place=='shleincyrodil'){$os_money=$os_money+$gain_over;$food_bonus=$s_c;} 89. if($user_place=='great_plains'){$ogp_money=$ogp_money+$gain_over;$food_bonus=$gp_c;} 90. if($user_place=='western_province'){$owp_money=$owp_money+$gain_over;$food_bonus=$wp_c;} 91. /////////////////////////////////////////////// 92. ###################### FAILSAFE CHECK ########################## 93. $second_select=mysql_query("SELECT money,turns,food FROM data WHERE username_hashed='$user_hashed'"); 94. while($sel_sec = mysql_fetch_array($second_select)) 95. { 96. $user_money=$sel_sec['money']; 97. $user_turns=$sel_sec['turns']; 98. $user_food=$sel_sec['food']; 99. } 100. 101. ################################################################ 102. if($user_food<$user_pop){$dead=$user_pop-$user_food;}else{$dead=0;} 103. $new_pop=$user_homes*10-$dead; 104. $food_production=(($user_farms*100)*$food_bonus)*$civil_unrest; 105. $new_user_money=$gain_taxed+$user_money; 106. $new_turns = (($user_turns+$user_lab)+10); 107. $new_user_food=$user_food+$food_production-$new_pop; 108. if($new_user_food<0){$new_user_food=0;} 109. 110. mysql_query("UPDATE data SET money='$new_user_money',turns='$new_turns',food='$new_user_food',pop='$new_pop' WHERE username_hashed='$user_hashed'"); 111. if($user_ww>0) 112. { 113. $new_user_ww=$user_ww-0.05; 114. if($new_user_ww<=0){$new_user_ww=0;} 115. mysql_query("UPDATE units SET war_weariness='$new_user_ww' WHERE username_hashed='$user_hashed'"); 116. } 117. } 118. ############################################################################################## 119. ###################################### EXPLORATIONS ######################### 120. $select_voyage=mysql_query("SELECT * FROM voyages"); 121. while($voyage=mysql_fetch_array($select_voyage)) 122. { 123. $explorer=$voyage['username_hashed']; 124. $timer=$voyage['time']; 125. $timer2=$timer-1; 126. $l_gain=$voyage['land_expected']; 127. $force=$voyage['away']; 128. $id=$voyage['id']; 129. if($timer==0) 130. { 131. $select_explorer=mysql_query("SELECT * FROM data WHERE username_hashed='$explorer'"); 132. while($explo=mysql_fetch_array($select_explorer)) 133. { 134. $user_land=$explo['land']; 135. } 136. $select_unite=mysql_query("SELECT * FROM units WHERE username_hashed='$explorer'"); 137. while($unite=mysql_fetch_array($select_unite)) 138. { 139. $user_lip=$unite['light_infantry']; 140. } 141. $new_land=$user_land+$l_gain; 142. $new_lis=$user_lip+$force; 143. mysql_query("UPDATE data SET land='$new_land' WHERE username_hashed='$explorer'"); 144. mysql_query("UPDATE units SET light_infantry='$new_lis' WHERE username_hashed='$explorer'"); 145. mysql_query("delete from `voyages` where `id` = '$id'"); 146. //MSG 147. $t=time(); 148. $message = "<i>Your <b>".$force."</b> soldiers have arrived back from their voyage. They have discovered <b>".$l_gain."</b> Acres of new land for your empire! - from <b>Your Chief Messenger</b> on <b>".date("d/m/y",$t)."</b> at <b>".date("H:i:s",$t)."</b></i>"; 149. mysql_query("INSERT INTO `news` (`username_hashed`,`latest`)VALUES('$explorer','$message')"); 150. /////// 151. } 152. else 153. { 154. mysql_query("UPDATE voyages SET time='$timer2' WHERE id='$id'"); 155. } 156. } 157. ################################################################################################## 158. ################################### WEATHER ########################################### 159. $weather1 = RAND(1,2); 160. $weather2 = RAND(1,2); 161. $weather3 = RAND(1,3); 162. $weather4 = RAND(1,4); 163. IF($weather1 == 1){$weath1 = "sunny";} 164. IF($weather1 == 2){$weath1 = "over cast";} 165. 166. IF($weather2 == 1){$weath2 = "frosty";} 167. IF($weather2 == 2){$weath2 = "rain";} 168. 169. IF($weather3 == 1){$weath3 = "rain";} 170. IF($weather3 == 2){$weath3 = "sunny";} 171. IF($weather3 == 3){$weath3 = "over cast";} 172. 173. IF($weather4 == 1){$weath4 = "rain";} 174. IF($weather4 == 2){$weath4 = "sunny";} 175. IF($weather4 == 3){$weath4 = "over cast";} 176. IF($weather4 == 4){$weath4 = "frosty";} 177. mysql_query("update `weather` set `great_plains` = '$weath1',`western_province` = '$weath2',`yuaktz` = '$weath3',`shleincyrodil` = '$weath4'"); 178. ################################################################################################## 179. 180. $war_sel=mysql_query("select * from war_timer "); 181. while($war=mysql_fetch_array($war_sel)) 182. { 183. $username_has = $war['username_hashed']; 184. $target = $war['target']; 185. $time1s=$war['time']; 186. $time2s=$time1s-1; 187. mysql_query("update `war_timer` set `time` = '$time2s' WHERE username_hashed='$username_has' AND target='$target'"); 188. } 189. mysql_query("delete from `war_timer` where `time` = '0'"); 190. mysql_query("delete from `war_timer` where `time` < '0'"); 191. ####################################### OVERLORD PAY ############################################# 192. $o_y = mysql_query("select * from overlords where place = 'yuaktz'"); 193. $y_o=mysql_result($o_y,0,"username"); 194. if($y_o!='-') 195. { 196. $sel_o=mysql_query("SELECT * FROM data WHERE username='$y_o'"); 197. $y_m=mysql_result($sel_o,0,"money"); 198. $new_money=number_format(($oy_money+$y_m),'0','',''); 199. mysql_query("update `data` set `money` = '$new_money' WHERE username='$y_o' "); 200. //MSG 201. $receiver=md5($y_o); 202. $message = "Sire You have received £ <b>".number_format($oy_money,'0')."</b> as your overlord pay."; 203. mysql_query("INSERT INTO `news` (`username_hashed`,`latest`)VALUES('$receiver','$message')"); 204. /////// 205. } 206. 207. $o_s = mysql_query("select * from overlords where place = 'shleincyrodil'"); 208. $s_o=mysql_result($o_s,0,"username"); 209. if($s_o!='-') 210. { 211. $sel_o=mysql_query("SELECT * FROM data WHERE username='$s_o'"); 212. $s_m=mysql_result($sel_o,0,"money"); 213. $new_money=number_format(($os_money+$s_m),'0','',''); 214. mysql_query("update `data` set `money` = '$new_money' WHERE username='$s_o' "); 215. //MSG 216. $receiver=md5($s_o); 217. $message = "Sire You have received £ <b>".number_format($os_money,'0')."</b> as your overlord pay."; 218. mysql_query("INSERT INTO `news` (`username_hashed`,`latest`)VALUES('$receiver','$message')"); 219. /////// 220. } 221. 222. $o_gp = mysql_query("select * from overlords where place = 'great_plains'"); 223. $gp_o=mysql_result($o_gp,0,"username"); 224. if($gp_o!='-') 225. { 226. $sel_o=mysql_query("SELECT * FROM data WHERE username='$gp_o'"); 227. $gp_m=mysql_result($sel_o,0,"money"); 228. $new_money=number_format(($ogp_money+$gp_m),'0','',''); 229. mysql_query("update `data` set `money` = '$new_money' WHERE username='$gp_o' "); 230. //MSG 231. $receiver=md5($gp_o); 232. $message = "Sire You have received £ <b>".number_format($ogp_money,'0')."</b> as your overlord pay."; 233. mysql_query("INSERT INTO `news` (`username_hashed`,`latest`)VALUES('$receiver','$message')"); 234. /////// 235. } 236. 237. $o_wp = mysql_query("select * from overlords where place = 'western_province'"); 238. $wp_o=mysql_result($o_wp,0,"username"); 239. if($wp_o!='-') 240. { 241. $sel_o=mysql_query("SELECT * FROM data WHERE username='$wp_o'"); 242. $wp_m=mysql_result($sel_o,0,"money"); 243. $new_money=number_format(($owp_money+$wp_m),'0','',''); 244. mysql_query("update `data` set `money` = '$new_money' WHERE username='$wp_o' "); 245. //MSG 246. $receiver=md5($wp_o); 247. $message = "Sire You have received £ <b>".number_format($owp_money,'0')."</b> as your overlord pay."; 248. $timeris=time(); 249. mysql_query("INSERT INTO `news` (`username_hashed`,`latest`,`time`)VALUES('$receiver','$message','$timeris')"); 250. /////// 251. } 252. ############################ RESETING ELECTION TABLE 253. $check_votes_num2=mysql_query("SELECT * FROM `voting`"); 254. $num_checkings=mysql_num_rows($check_votes_num2); 255. $t=time(); 256. $date=date("D",$t); 257. #echo"$date"; 258. if($date=='Sun' && $num_checkings>=0) 259. { 260. mysql_query("TRUNCATE TABLE `voting`"); 261. mysql_query("UPDATE `election_nominees` SET `votes`='0'"); 262. } 263. ######################################################################################################## 264. $time2=time(); 265. $time3=$time2-$time1; 266. #echo"Execution time : $time3 seconds"; 267. #echo"<br>Taxes : y=$y_t , s=$s_t , gp=$gp_t , wp=$wp_t"; 268. #echo"<br>Overlord money: y=$oy_money , s=$os_money , gp=$ogp_money , wp=$owp_money"; 269. mysql_query("INSERT INTO `execution` (`time`,`exec`)VALUES('$time2','$time3')"); 270. echo"$num_checkings"; 271. ?>
Thanks!
Lee.
-
ok thanks. Do you know where I can find some?
-
Hello avid PHPers!
I hope you can help me. I have been asked to work on a project from scratch and it really is a huge task. It will most probably take several months of solid work. Now, I am experienced in PHP and average at MySQL, what I'm asking is: With such a huge task, what's the best way to go about with organisation? I've taught myself PHP and SQL roughly over 3 years, but I know I am lacking in the efficiency of programming. I know serious programmers would assign classes etc. yet I've not used these before.
Any advice?
Greatly appreciated,
Lee.
-
I've tried that. By the way I am using a local server on my PC which has cURL enabled.
-
You shouldn't be asking how safe MD5 is, you should be asking "How can I make my hashes safer".
Now, these hash "cracking" sites are utter useless if you use MD5() properly.
Have you ever heard of a salt or key?
It would be practically impossible for these sites to crack a hash with my own personal salt:
md5("$username$password$key");
Where $salt is something personal. For example, say I want a secure login for my blog, I may have the $key as the name of my blog + the name of my cat.
-
Hello,
I'm trying to use curl_init() and curl_setopt() to send some data via POST to another script where it will be parsed.
This script is basic, yet I don't understand why it's not working. My experience with cURL functions is little.
Here's the main script which sends the data:
<? $path = "form.php"; //Relative path to the file with $_POST parsing $ch = curl_init($path); //Initialise curl resource with above file $data = "submit=submit&name=bob&age=100"; //Data to be sent to the file curl_setopt($ch, CURLOPT_POSTFIELDS, $data); //Send the data to the file? curl_close($ch); //Close curl session ?>
Here's the recipient script which parses the request, and on success should make a directory named "success". I have tested the directory creation without the first script, as I thought it may be permission problems, however everything in that area is fine.
<? if($_POST['submit']) { print "Your name is ".$_POST['name']." and your age is ".$_POST['age']; mkdir("C:/Users/Lee/Desktop/xampp/htdocs/PHP/".$_POST['name']); } ?>
When executing the first script I receive noting but a blank screen. I tried curl_exec($ch); to generate some output yet it gave nothing of use.
Any help would be greatly appreciated.
Thanks.
-
The global variable is wrong.
Change:
<form method="post" action="<?php echo $PHP_SELF;?>">
To:
<form method="POST" action="<? $_SERVER['PHP_SELF']; ?>">
So, I would totally re-do with:
IF ((isset($_POST['done'])) && ($_POST['done'] == 'y')) { $pres = $_POST['pres']; <form method='POST' action='<? $_SERVER['PHP_SELF']; ?>'> Preservative to be included in price (Y or N)- <input type='text' name='pres' value='<? print $pres; ?>' size='10'>
Lee.
-
It seems that complains are coming from a range of email servers, such as:
- Comcast.net
- Gmail.com
- wp.pl
- optusnet.com.au
- 1337g4m3r.com
- mcshi.com
- aol.com
The list goes on
This seriously isn't good for a game...
-
Dear PHP enthusiasts!
I'm in need of some quite critical help. At the moment, I have created a fairly successful MMORPG using PHP and MySQL. However, the activation link I send via email using the standard PHP Mail() function seems to be giving a suspicious number of complaints regarding people not receiving the emails. Here is how I have used the function, I have tried to use the extra parameters in hope it would solve the problem, yet it hasn't:
$headers = "From: team@civ-online.com \r\n"; $headers.= "Content-Type: text/html; charset=ISO-8859-1 "; $headers .= "MIME-Version: 1.0 "; /*notice there aren't any \r\n after the second two header additions. This is what made this version work correctly*/ mail("$form_email_address", "Civ-Online Confirmation Email", $body, $headers);
I had taken this from another website's example.
All help is greatly appreciated! I await any help
Lee.
-
Hi,
Could someone please show me a pattern that will match a website's description meta tag?
Thanks,
Lee. -
Hi,
I'm currently coding a search engine, and everything seems to be going smoothly, until I reach regular expressions -_- (I hate them so much..). So far, the script can crawl a site from an array, and grab a sentence with the keywords. However, it's a bit messy, and I wondered if someone would be so kind as to show a pattern that would do it properly.
Basically, I need a pattern that matches a sentence with letters and numbers only (to filter out HTML) and with the sentence must have this in: [code=php:0]$pieces[0][/code]
Here's the code I'm using at the moment: [code=php:0]/$pieces[0].*/[/code] I must say it's not too good.
Any help greatly appreciated,
Thanks. -
Anyone know? :/
-
It is allowed because when I manually use:
[code=php:0]
$test = Get_File_Contents("http://www.example.com");
echo $test;
[/code]
That streams and returns fine.. :/ -
Ah right, yes I understand. Still, I get:
[code]Warning: file_get_contents("http://www.carsthemovie.com/"): failed to open stream: No such file or directory in /home/hydropow/public_html/izearch/searched.php on line 161[/code]
Check out my new message board
in Beta Test Your Stuff!
Posted
Because it gives people a clue when using SQL Injection, or people can just replicate a cookie with anyone's username in it and post as them.
If you want to use cookies, at least hash the username and use a personal salt. Oh, make sure the cookies are relative to the domain too.