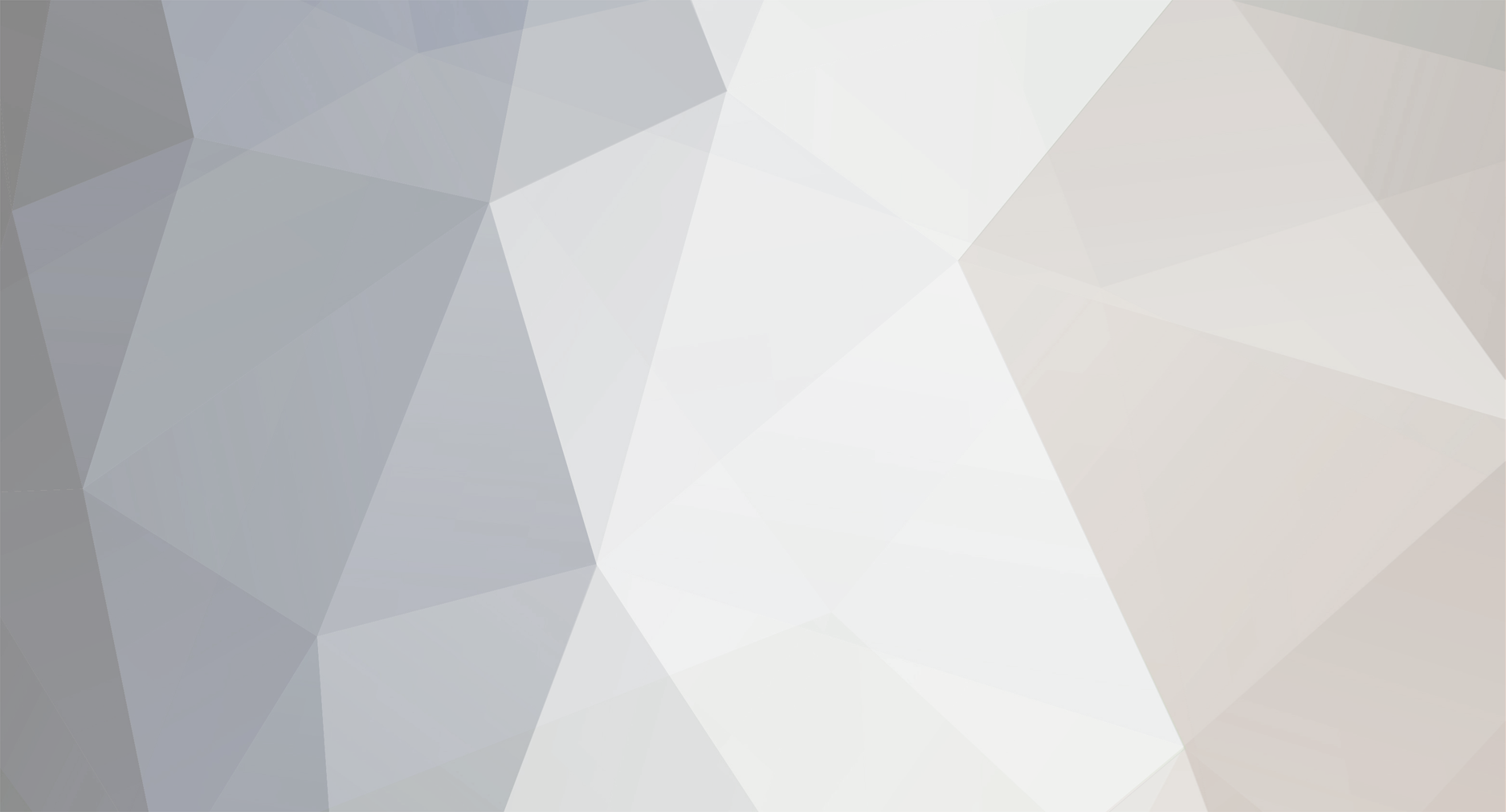
jarvis
Members-
Posts
543 -
Joined
-
Last visited
Everything posted by jarvis
-
Right, I've got this working how I want but need to 'tidy' the code up as it's not ideal. I think this can be solved by means of a loop or loops. Any help is very much appreciated! <?php $page_title = 'Locate A Centre'; include ('includes/header.html'); require_once('mysql_connect.php');// Connect to the db if (isset($_POST['submitted'])) { // Handle the form. if (!empty($_POST['start'])) { $start = escape_data($_POST['start']); } else { $start = FALSE; echo '<p>Please enter a valid postcode!</p>'; } $get_destination = escape_data($_POST['finish']); $query = "SELECT centre_id, centre_name, postcode FROM centres LEFT JOIN centre_associations USING (centre_id) WHERE category_id=$get_destination LIMIT 5"; $result = mysql_query ($query); $i=0; while ($row = mysql_fetch_array($result, MYSQL_NUM)) { $finish[$i] = $row[2]; $centre_name[$i] = $row[1]; $i++; } echo 'your nearest centre are <br/>'; echo $centre_name['0'].' '.$finish['0'].'<br/>'; echo $centre_name['1'].' '.$finish['1'].'<br/>'; echo $centre_name['2'].' '.$finish['2'].'<br/>'; echo $centre_name['3'].' '.$finish['3'].'<br/>'; echo $centre_name['4'].' '.$finish['4'].'<br/>'; #Assign values $finish0 = $finish['0']; $finish1 = $finish['1']; $finish2 = $finish['2']; $finish3 = $finish['3']; $finish4 = $finish['4']; #Convert the post code to upper case and trim the variable $start = strtoupper(trim($start)); $finish0 = strtoupper(trim($finish0)); $finish1 = strtoupper(trim($finish1)); $finish2 = strtoupper(trim($finish2)); $finish3 = strtoupper(trim($finish3)); $finish4 = strtoupper(trim($finish4)); #Remove any spaces $start = str_replace(" ","",$start); $finish0 = str_replace(" ","",$finish0); $finish1 = str_replace(" ","",$finish1); $finish2 = str_replace(" ","",$finish2); $finish3 = str_replace(" ","",$finish3); $finish4 = str_replace(" ","",$finish4); #Trim the last 3 characters off the end $start = substr($start,0,strlen($start)-3); $finish0 = substr($finish0,0,strlen($finish0)-3); $finish1 = substr($finish1,0,strlen($finish1)-3); $finish2 = substr($finish2,0,strlen($finish2)-3); $finish3 = substr($finish3,0,strlen($finish3)-3); $finish4 = substr($finish4,0,strlen($finish4)-3); #query the db $query_start = "SELECT latitude, longitude FROM postcodes WHERE postcode = '$start' LIMIT 1"; $result_start = mysql_query ($query_start); $num = mysql_num_rows ($result_start); // How many users are there? if ($num > 0) { // If it ran OK, display the records. while ($row = mysql_fetch_array ($result_start, MYSQL_ASSOC)) { #Assign variables $lat1 = $row["latitude"]; $long1 = $row["longitude"]; } } else { echo '<p>post code not found</p>'; } $query_finish = "SELECT latitude, longitude FROM postcodes WHERE postcode = '$finish0' LIMIT 1"; $result_finish = mysql_query ($query_finish); $num = mysql_num_rows ($result_finish); // How many users are there? if ($num > 0) { // If it ran OK, display the records. while ($row = mysql_fetch_array ($result_finish, MYSQL_ASSOC)) { #Assign variables $lat0 = $row["latitude"]; $long0 = $row["longitude"]; } } else { echo '<p>post code not found</p>'; } $query_finish = "SELECT latitude, longitude FROM postcodes WHERE postcode = '$finish1' LIMIT 1"; $result_finish = mysql_query ($query_finish); $num = mysql_num_rows ($result_finish); // How many users are there? if ($num > 0) { // If it ran OK, display the records. while ($row = mysql_fetch_array ($result_finish, MYSQL_ASSOC)) { #Assign variables $lat11 = $row["latitude"]; $long11 = $row["longitude"]; } } else { echo '<p>post code not found</p>'; } $query_finish = "SELECT latitude, longitude FROM postcodes WHERE postcode = '$finish2' LIMIT 1"; $result_finish = mysql_query ($query_finish); $num = mysql_num_rows ($result_finish); // How many users are there? if ($num > 0) { // If it ran OK, display the records. while ($row = mysql_fetch_array ($result_finish, MYSQL_ASSOC)) { #Assign variables $lat2 = $row["latitude"]; $long2 = $row["longitude"]; } } else { echo '<p>post code not found</p>'; } $query_finish = "SELECT latitude, longitude FROM postcodes WHERE postcode = '$finish3' LIMIT 1"; $result_finish = mysql_query ($query_finish); $num = mysql_num_rows ($result_finish); // How many users are there? if ($num > 0) { // If it ran OK, display the records. while ($row = mysql_fetch_array ($result_finish, MYSQL_ASSOC)) { #Assign variables $lat3 = $row["latitude"]; $long3 = $row["longitude"]; } } else { echo '<p>post code not found</p>'; } $query_finish = "SELECT latitude, longitude FROM postcodes WHERE postcode = '$finish4' LIMIT 1"; $result_finish = mysql_query ($query_finish); $num = mysql_num_rows ($result_finish); // How many users are there? if ($num > 0) { // If it ran OK, display the records. while ($row = mysql_fetch_array ($result_finish, MYSQL_ASSOC)) { #Assign variables $lat4 = $row["latitude"]; $long4 = $row["longitude"]; } } else { echo '<p>post code not found</p>'; } function getDistance0($lat1, $long1, $lat0, $long0){ #$earth = 6371; #km change accordingly $earth = 3960; #miles #Point 1 cords $lat1 = deg2rad($lat1); $long1= deg2rad($long1); #Point 2 cords $lat0 = deg2rad($lat0); $long0= deg2rad($long0); #Haversine Formula $dlong=$long0-$long1; $dlat=$lat0-$lat1; $sinlat=sin($dlat/2); $sinlong=sin($dlong/2); $a=($sinlat*$sinlat)+cos($lat1)*cos($lat0)*($sinlong*$sinlong); $c=2*asin(min(1,sqrt($a))); $d=round($earth*$c); return $d; } function getDistance1($lat1, $long1, $lat11, $long11){ #$earth = 6371; #km change accordingly $earth = 3960; #miles #Point 1 cords $lat1 = deg2rad($lat1); $long1= deg2rad($long1); #Point 2 cords $lat11 = deg2rad($lat11); $long11= deg2rad($long11); #Haversine Formula $dlong=$long1-$long1; $dlat=$lat11-$lat1; $sinlat=sin($dlat/2); $sinlong=sin($dlong/2); $a=($sinlat*$sinlat)+cos($lat1)*cos($lat11)*($sinlong*$sinlong); $c=2*asin(min(1,sqrt($a))); $d=round($earth*$c); return $d; } function getDistance2($lat1, $long1, $lat2, $long2){ #$earth = 6371; #km change accordingly $earth = 3960; #miles #Point 1 cords $lat1 = deg2rad($lat1); $long1= deg2rad($long1); #Point 2 cords $lat2 = deg2rad($lat2); $long2= deg2rad($long2); #Haversine Formula $dlong=$long2-$long1; $dlat=$lat2-$lat1; $sinlat=sin($dlat/2); $sinlong=sin($dlong/2); $a=($sinlat*$sinlat)+cos($lat1)*cos($lat2)*($sinlong*$sinlong); $c=2*asin(min(1,sqrt($a))); $d=round($earth*$c); return $d; } function getDistance3($lat1, $long1, $lat3, $long3){ #$earth = 6371; #km change accordingly $earth = 3960; #miles #Point 1 cords $lat1 = deg2rad($lat1); $long1= deg2rad($long1); #Point 2 cords $lat3 = deg2rad($lat3); $long3= deg2rad($long3); #Haversine Formula $dlong=$long3-$long1; $dlat=$lat3-$lat1; $sinlat=sin($dlat/2); $sinlong=sin($dlong/2); $a=($sinlat*$sinlat)+cos($lat1)*cos($lat3)*($sinlong*$sinlong); $c=2*asin(min(1,sqrt($a))); $d=round($earth*$c); return $d; } function getDistance4($lat1, $long1, $lat4, $long4){ #$earth = 6371; #km change accordingly $earth = 3960; #miles #Point 1 cords $lat1 = deg2rad($lat1); $long1= deg2rad($long1); #Point 2 cords $lat4 = deg2rad($lat4); $long4= deg2rad($long4); #Haversine Formula $dlong=$long4-$long1; $dlat=$lat4-$lat1; $sinlat=sin($dlat/2); $sinlong=sin($dlong/2); $a=($sinlat*$sinlat)+cos($lat1)*cos($lat4)*($sinlong*$sinlong); $c=2*asin(min(1,sqrt($a))); $d=round($earth*$c); return $d; } #Returns the distance in miles $distance0 = getDistance0($lat1, $long1, $lat0, $long0); #Moved inside isset so wont show when page loads echo '<p>That\'s a distance of '.$distance0.' miles</p>'; #Returns the distance in miles $distance1 = getDistance1($lat1, $long1, $lat11, $long11); echo '<p>That\'s a distance of '.$distance1.' miles</p>'; #Returns the distance in miles $distance2 = getDistance2($lat1, $long1, $lat2, $long2); echo '<p>That\'s a distance of '.$distance2.' miles</p>'; #Returns the distance in miles $distance3 = getDistance3($lat1, $long1, $lat3, $long3); echo '<p>That\'s a distance of '.$distance3.' miles</p>'; #Returns the distance in miles $distance4 = getDistance4($lat1, $long1, $lat4, $long4); echo '<p>That\'s a distance of '.$distance4.' miles</p>'; } ?> <form method="post" action="locate.php"> start: <input type="text" name="start" value="<?php if (isset($_POST['start'])) echo $_POST['start']; ?>" /> course: <select name="finish" size="5"> <?php // Create the pull-down menu information. $query = "SELECT category_id, category FROM categories ORDER BY category ASC"; $result = @mysql_query ($query); while ($row = mysql_fetch_array ($result, MYSQL_NUM)) { echo "<option value=\"$row[0]\""; echo ">$row[1]</option>\n"; } ?> </select> <input type="submit" name="submit" value="Go" /> <input type="hidden" name="submitted" value="TRUE" /> </form> <?php include ('includes/footer.html'); ?>
-
Hi, Sorry, am struggling with this. Right, thanks to those above who've helped, i'm now getting somewhere! The following code returns Am struggling with 2 parts: 1) Getting the postcode next to the company name 2) Getting the distance of each location At the mo it says 'post code not found'. And won't show any distances either. <?php $page_title = 'Locate A Centre'; include ('includes/header.html'); require_once('mysql_connect.php');// Connect to the db if (isset($_POST['submitted'])) { // Handle the form. if (!empty($_POST['start'])) { $start = escape_data($_POST['start']); } else { $start = FALSE; echo '<p>Please enter a valid postcode!</p>'; } $get_destination = escape_data($_POST['finish']); $query = "SELECT centre_id, centre_name, postcode FROM centres LEFT JOIN centre_associations USING (centre_id) WHERE category_id=$get_destination"; $result = mysql_query ($query); $i=0; while ($row = mysql_fetch_array($result, MYSQL_NUM)) { $finish[] = $row[2]; $centre_name[] = $row[1]; } #print_r ($finish); $location = "Your nearest ".count($centre_name); # will put an is if only 1 and an are if more than 1 $location .= (count($centre_name) > 1) ? " centres are:<br/> " : " centre is:<br/> "; # now go through the array, and add the values to string foreach($centre_name as $key => $name){ $location .= $name; # will add a comma if we aren't at the end. if not just add a space $location .= ($key != (count($centre_name) - 1)) ? "<br/> " : " "; } echo $location; foreach($finish as $key => $postcode){ $finish .= $postcode; # will add a comma if we aren't at the end. if not just add a space $finish .= ($key != (count($postcode) - 1)) ? "<br/> " : " "; } echo $finish; #Convert the post code to upper case and trim the variable $start = strtoupper(trim($start)); $finish = strtoupper(trim($finish)); #Remove any spaces $start = str_replace(" ","",$start); $finish = str_replace(" ","",$finish); #Trim the last 3 characters off the end $start = substr($start,0,strlen($start)-3); $finish = substr($finish,0,strlen($finish)-3); #query the db $query_start = "SELECT latitude, longitude FROM postcodes WHERE postcode = '$start' LIMIT 1"; $result_start = mysql_query ($query_start); $num = mysql_num_rows ($result_start); // How many users are there? if ($num > 0) { // If it ran OK, display the records. while ($row = mysql_fetch_array ($result_start, MYSQL_ASSOC)) { #Assign variables $lat1 = $row["latitude"]; $long1 = $row["longitude"]; } } else { echo '<p>post code not found</p>'; } $query_finish = "SELECT latitude, longitude FROM postcodes WHERE postcode = '$finish' LIMIT 1"; $result_finish = mysql_query ($query_finish); $num = mysql_num_rows ($result_finish); // How many users are there? if ($num > 0) { // If it ran OK, display the records. while ($row = mysql_fetch_array ($result_finish, MYSQL_ASSOC)) { #Assign variables $lat2 = $row["latitude"]; $long2 = $row["longitude"]; } } else { echo '<p>post code not found</p>'; } function getDistance($lat1, $long1, $lat2, $long2){ #$earth = 6371; #km change accordingly $earth = 3960; #miles #Point 1 cords $lat1 = deg2rad($lat1); $long1= deg2rad($long1); #Point 2 cords $lat2 = deg2rad($lat2); $long2= deg2rad($long2); #Haversine Formula $dlong=$long2-$long1; $dlat=$lat2-$lat1; $sinlat=sin($dlat/2); $sinlong=sin($dlong/2); $a=($sinlat*$sinlat)+cos($lat1)*cos($lat2)*($sinlong*$sinlong); $c=2*asin(min(1,sqrt($a))); $d=round($earth*$c); return $d; } #Returns the distance in miles $distance = getDistance($lat1, $long1, $lat2, $long2); #Show only the message when a forms submitted echo '<p>That\'s a distance of '.$distance.' miles</p>'; } ?> <form method="post" action="locate.php"> start: <input type="text" name="start" value="<?php if (isset($_POST['start'])) echo $_POST['start']; ?>" /> course: <select name="finish" size="5"> <?php // Create the pull-down menu information. $query = "SELECT category_id, category FROM categories ORDER BY category ASC"; $result = @mysql_query ($query); while ($row = mysql_fetch_array ($result, MYSQL_NUM)) { echo "<option value=\"$row[0]\""; echo ">$row[1]</option>\n"; } ?> </select> <input type="submit" name="submit" value="Go" /> <input type="hidden" name="submitted" value="TRUE" /> </form> <?php include ('includes/footer.html'); ?> Sorry to keep posting but am struggling a bit with this one! Thanks
-
Ah ok, I'm getting somewhere (have had a very strong coffee now!) My code $i=0; while ($row = mysql_fetch_array($result, MYSQL_NUM)) { $finish[] = $row[2]; $centre_name[] = $row[1]; } $location = "Your nearest ".count($centre_name); $location .= (count($centre_name) > 1) ? " centres are " : " centre is "; //will put an is if only 1 and an are if more than 1 //now go through the array, and add the values to string foreach($centre_name as $key => $name){ $location .= $name; $location .= ($key != (count($centre_name) - 1)) ? "<br/> " : " "; //will add a comma if we aren't at the end. if not just add a space } echo $location; This now works without error. However, I need to add the postcode into the loop and it needs to show the distance too. For example: The distance is calculated by the function, which is why I wondered if you need to loop through to get the postcodes for each centre, than pass that into the function, looping that to provide the above info? Thanks
-
Thank mikesta707 Why do I not need the i++ in the loop [php while ($row = mysql_fetch_array($result, MYSQL_NUM)) { $finish[] = $row[2]; $centre_name[] = $row[1]; } [/code] Like it was here while ($row = mysql_fetch_array($result, MYSQL_NUM)) { $finish[$i] = $row[2]; $centre_name[$i] = $row[1]; $i++; } I also get the error: When I use [php $string = "Your nearest ".count($centre_name); string .= (count($centre_name) > 1) ? " events are " : " event is ";//will put an is if only 1 and an are if more than 1 //now go through the array, and add the values to string foreach($centre_name as $key => $name){ $string .= $name; $string .= ($key != (count($centre_name) - 1)) ? ", " : " ";//will add a comma if we aren't at the end. if not just add a space } echo $string [/code] line 43 is string .= (count($centre_name) > 1) ? " events are " : " event is "; //will put an is if only 1 and an are if more than 1 Finally, do I need this line: $centre_name = array("dog", "cat", "mouse"); Or is it just to illustrate what the array would like like? Thanks & apologies!
-
Hi ldb358 Thanks again for the reply. I think that will sort the issue if there is only 1 postcode. The other part was me trying to say, once I loop through 5 times to produce 5 postcodes, I need to pass these in to the code so it says location 1 - 25 miles location 2 - 50 miles etc So once the loop generates the postcode and centre name,rather than list them out, I need to pass them into the function so it will then say the above Does that make more sense? thanks again!
-
I've added this bit echo 'your nearest 5 centre are '.$finish['0'] . ", " .$finish['1'] . ", " .$finish['2'] . ", " .$finish['3'] . ", " .$finish['4']; This is needed to show the postcodes for each centre. I guess I now need to loop the whole thing passing in the postcodes? That should do it I guess, if I then sort the distance by ascending??
-
Thanks ldb358, I've replaced my code with the loop, I think it's working. It shows my centres but says postcode not found Hmmm... Also, what if only one centre runs that course? If I try that, I get: The distance of 3516 returns if a postcode isn't found i've discovered! I figured a loop was needed somewhere but wasn't sure if that would just show the same result 5 times or not (sorry if thats a daft thing to say!) Thanks
-
Afternoon All, Following on from a previous post, I've now managed to get my postcode script to work, i.e. I can enter a postcode of a start & finish and it returns the distance. To extend on that, I've set up a centres table, a courses table and an association table. When you add a centre, you select (multiple) courses that the place will hold. This all works well. I then have a script (below) which you enter your postcode and select the course you want to do, it should then return the 5 nearest centres. At the mo it only returns 1. Can someone point me in the right direction as I've now looked at this way too long. Thanks <?php $page_title = 'Locate A Centre'; include ('includes/header.html'); require_once('mysql_connect.php');// Connect to the db if (isset($_POST['submitted'])) { // Handle the form. if (!empty($_POST['start'])) { $start = escape_data($_POST['start']); } else { $start = FALSE; echo '<p>Please enter a valid postcode!</p>'; } $get_destination = escape_data($_POST['finish']); $query = "SELECT centre_id, centre_name, postcode FROM centres LEFT JOIN centre_associations USING (centre_id) WHERE category_id=$get_destination"; $result = mysql_query ($query); while ($row = mysql_fetch_array($result, MYSQL_NUM)) { $finish = $row[2]; $centre_name = $row[1]; } #echo $finish; echo 'your nearest centre is '.$centre_name; #Convert the post code to upper case and trim the variable $start = strtoupper(trim($start)); $finish = strtoupper(trim($finish)); #Remove any spaces $start = str_replace(" ","",$start); $finish = str_replace(" ","",$finish); #Trim the last 3 characters off the end $start = substr($start,0,strlen($start)-3); $finish = substr($finish,0,strlen($finish)-3); #query the db $query_start = "SELECT latitude, longitude FROM postcodes WHERE postcode = '$start' LIMIT 1"; $result_start = mysql_query ($query_start); $num = mysql_num_rows ($result_start); // How many users are there? if ($num > 0) { // If it ran OK, display the records. while ($row = mysql_fetch_array ($result_start, MYSQL_ASSOC)) { #Assign variables $lat1 = $row["latitude"]; $long1 = $row["longitude"]; } } else { echo '<p>post code not found</p>'; } $query_finish = "SELECT latitude, longitude FROM postcodes WHERE postcode = '$finish' LIMIT 1"; $result_finish = mysql_query ($query_finish); $num = mysql_num_rows ($result_finish); // How many users are there? if ($num > 0) { // If it ran OK, display the records. while ($row = mysql_fetch_array ($result_finish, MYSQL_ASSOC)) { #Assign variables $lat2 = $row["latitude"]; $long2 = $row["longitude"]; } } else { echo '<p>post code not found</p>'; } function getDistance($lat1, $long1, $lat2, $long2){ #$earth = 6371; #km change accordingly $earth = 3960; #miles #Point 1 cords $lat1 = deg2rad($lat1); $long1= deg2rad($long1); #Point 2 cords $lat2 = deg2rad($lat2); $long2= deg2rad($long2); #Haversine Formula $dlong=$long2-$long1; $dlat=$lat2-$lat1; $sinlat=sin($dlat/2); $sinlong=sin($dlong/2); $a=($sinlat*$sinlat)+cos($lat1)*cos($lat2)*($sinlong*$sinlong); $c=2*asin(min(1,sqrt($a))); $d=round($earth*$c); return $d; } #Returns the distance in miles $distance = getDistance($lat1, $long1, $lat2, $long2); } echo '<p>That\'s a distance of '.$distance.' miles</p>'; ?> <form method="post" action="locate.php"> start: <input type="text" name="start" value="<?php if (isset($_POST['start'])) echo $_POST['start']; ?>" /> course: <select name="finish" size="5"> <?php // Create the pull-down menu information. $query = "SELECT category_id, category FROM categories ORDER BY category ASC"; $result = @mysql_query ($query); while ($row = mysql_fetch_array ($result, MYSQL_NUM)) { echo "<option value=\"$row[0]\""; echo ">$row[1]</option>\n"; } ?> </select> <input type="submit" name="submit" value="Go" /> <input type="hidden" name="submitted" value="TRUE" /> </form> <?php include ('includes/footer.html'); ?> Hope that makes sense and thanks in advanced!
-
Thanks dave_sticky! I think im now getting somewhere. One odd thing is that although the code works in sql if I run the query direct, it wont run in the script. if (isset($_GET['aid'])) { $id = $_GET['aid']; $query = "SELECT `page_name` FROM `pages` WHERE `page_id`='" . $id . "'"; #echo $query; $result = mysql_query($query); $name = $row['page_name']; while ($row = mysql_fetch_array ($result, MYSQL_ASSOC)) { $default_image_sql = 'SELECT image_description, banner_id, page_id, file_name, web FROM banner_ads WHERE page_id = '.$id.''; echo $default_image_sql; $default_image_rs = mysql_query($default_image_sql) or die(mysql_error()); $default_image_row = mysql_fetch_array($default_image_rs); $online_images_total_sql = 'SELECT count(file_name) AS total_images FROM banner_ads WHERE page_id = '.$id.''; echo $online_images_total_sql; $online_images_total_rs = mysql_query($online_images_total_sql) or die(mysql_error()); $total_images_uploaded = mysql_fetch_array($online_images_total_rs); $image_available = $total_images_uploaded['total_images']; echo $image_available; if ($image_available == 0) { }else{ // There is an image so display it $imgfile = 'banners/'.$default_image_row['page_id'].'/'.$default_image_row['file_name']; if(file_exists($imgfile) && $image = @getimagesize($imgfile)) { $t_imgfile='banners/'.$default_image_row['page_id'].'/t_'.$default_image_row['file_name']; $web = $default_image_row['web']; if ($web !=""){ echo '<a href="http://'.$web.'"><img src="'.$t_imgfile.'" alt="'.$default_image_row['image_description'].'" border="0" /></a>'; } else { echo '<img src="'.$t_imgfile.'" alt="'.$default_image_row['image_description'].'" border="0" />'; } } } } } else { $url = $_SERVER["PHP_SELF"]; $name = Explode('/', $url); $name[count($name) - 1]; $name = $name[count($name) - 1]; $query = "SELECT `page_id` FROM `pages` WHERE `page_name` = '" . $name . "'"; ####THIS LINE### echo $query.'<hr/>'; $result = mysql_query($query) or die(mysql_error()); $id = $row['page_id']; while ($row = mysql_fetch_array ($result, MYSQL_ASSOC)) { $default_image_sql = 'SELECT image_description, banner_id, page_id, file_name, web FROM banner_ads WHERE page_id = '.$name.'' ; #echo $default_image_sql; $default_image_rs = mysql_query($default_image_sql) or die(mysql_error()); $default_image_row = mysql_fetch_array($default_image_rs); $online_images_total_sql = 'SELECT count(file_name) AS total_images FROM banner_ads WHERE page_id = '.$name.''; #echo $online_images_total_sql; $online_images_total_rs = mysql_query($online_images_total_sql) or die(mysql_error()); $total_images_uploaded = mysql_fetch_array($online_images_total_rs); $image_available = $total_images_uploaded['total_images']; echo $image_available; if ($image_available == 0) { }else{ // There is an image so display it $imgfile = 'banners/'.$default_image_row['page_id'].'/'.$default_image_row['file_name']; if(file_exists($imgfile) && $image = @getimagesize($imgfile)) { $t_imgfile='banners/'.$default_image_row['page_id'].'/t_'.$default_image_row['file_name']; $web = $default_image_row['web']; if ($web !=""){ echo '<a href="http://'.$web.'"><img src="'.$t_imgfile.'" alt="'.$default_image_row['image_description'].'" border="0" /></a>'; } else { echo '<img src="'.$t_imgfile.'" alt="'.$default_image_row['image_description'].'" border="0" />'; } } } } } print 'Page ID: ' . $id . '<br />Page Name: ' . $name; I've marked the line which fails. If I browse to the page, it should return the page_id, browse to the dir with that number and then show the image. The query is fine in mysql but wont work above. have tried LIKE %% but that too fails. Why is that? Am at a loss! Thanks
-
I've not found an off the shelf or free solution. Anyone else know of one or will it be a bespoke jobbie? If so, what's the best route please. Thanks
-
Hi all, Not sure if this is the right place to post this or not. I'm in need of building a cms that allows images to be uploaded and resized. The images go into categories and will display in a grid. You can add, edit and delete image. By edit,move it to different/multiple categories. I also need to create user accounts, so only those with accounts can gain access. Each account can have access to all categories or some (which the administrator can set) Before I tear off to build this: a) is there something I can get for free that will do this? b) whats the best way of doing this? Many thanks
-
I think that's it! Thank bundyxc. Just one last thing, how do I just get the file name as that returns the whole path. I've the following but it doesn't work, it just says page name is array rather than the page name! $url = $_SERVER["PHP_SELF"]; $name = Explode('/', $url); $name[count($name) - 1]; #$name = $_SERVER['PHP_SELF']; $query = "SELECT `page_id` FROM `pages` WHERE `page_name`='" . $name . "'"; If i echo $name it shows the correct file name. Am I being stupid?
-
Hi bundyxc, appreciate the time and effort on this one! Basically, all I need to do is grab either the id or page name and pass it to the query, the query will then return the page_id which I tie in with another query (see below), this is used for some code to display the relevant image. When you upload an image to a page, a directory is created and the image uploaded to that dir. So, with the above it would be an image path of something like www.domain.com/banners/1/istock123.jpg Where 1 is the dir created DROP TABLE IF EXISTS `pages`; CREATE TABLE IF NOT EXISTS `pages` ( `page_id` smallint(4) unsigned NOT NULL AUTO_INCREMENT, `page_name` varchar(255) NOT NULL, PRIMARY KEY (`page_id`) ) ENGINE=InnoDB; DROP TABLE IF EXISTS `banner_ads`; CREATE TABLE IF NOT EXISTS `banner_ads` ( `banner_id` int(10) unsigned NOT NULL AUTO_INCREMENT, `page_id` int(10) unsigned NOT NULL DEFAULT '0', `file_name` varchar(255) NOT NULL, `file_size` int(6) unsigned NOT NULL, `file_type` varchar(30) NOT NULL, `image_description` varchar(255) DEFAULT NULL, `web` varchar(150) DEFAULT NULL, `date_entered` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP, PRIMARY KEY (`banner_id`), KEY `file_name` (`file_name`), KEY `date_entered` (`date_entered`) ) ENGINE=InnoDB; I hope that makes sense?
-
That would be perhaps. I guess what I need to do is determine whether the url is an id or page_name, then from there, pass whichever it is into the sql query!? Would this work $sql = "SELECT `page_id` FROM `pages` WHERE `page_name`='"; if (!isset($_GET['aid']) { $get = $_SERVER['PHP_SELF']; } else { $aid = $_GET['aid']; } "'";
-
@dave_sticky- thanks I think this may work - will have a play & see! @bundyxc - Although I like that, the prob is if the number of pages extends, I need to add to the code & is perhaps a little clumsy? Also, the file name part wont work in that way. As when you add a banner to a page via the cms, it creates a dir with the id number of that entry into the pages table and then the filename remains the same. For example, if I select from my list of pages index.php and upload istock123.jpg, it then adds this to the pages table and an images table. A dir is created numbered 1 if it's the first entry and the image is uploaded. I wonder if something like: if (isset($_GET['aid'])) { //If this there's a $_GET['aid'] in the URL, this page is dynamic. Consult database for the banner. $sql = "SELECT `page_id` FROM `pages` WHERE `page_name`='" . $_GET['aid'] . "'"; $query = mysql_query($sql); $fetch = mysql_fetch_assoc($query); $img = $fetch['page_id']; } else { //Otherwise, this page isn't dynamic. $get = $_SERVER['PHP_SELF']; $sql = "SELECT `page_id` FROM `pages` WHERE `page_name`='" . $get = $_SERVER['PHP_SELF'] . "'"; $query = mysql_query($sql); $fetch = mysql_fetch_assoc($query); $img = $fetch['page_id']; } Would that work?
-
Sorry to bump but any help is much appreciated! Thanks
-
This is great thanks! I've got a query though, my table allows you to enter the file name or select it from a db (via a drop down of article titles from the cms) if it's a dynamic page. My DB table for this part therefore looks like:
-
Ah I think I've sussed this, echo $lat1; echo $long1; $query=" SELECT *, ROUND( SQRT( POW((69.1 * ( $lat1 - latitude)), 2) + POW((53 * ( $long1 - longitude)), 2)), 1) AS `distance` FROM postcodes WHERE latitude< ($lat1+0.12) AND latitude > ($lat1-0.12) AND longitude < ($long1+0.12) AND longitude > ($long1-0.12) ORDER BY `distance` ASC"; $result = mysql_query ($query); $num = mysql_num_rows ($result); // How many users are there? if ($num > 0) { // If it ran OK, display the records. while ($row = mysql_fetch_array ($result, MYSQL_ASSOC)) { #Assign variables $test = $row["distance"]; echo $test.'</br/>'; } } else { echo '<p>post code not found</p>'; } If I get the towns into the db, I guess I can return the town with the distance? At the mo it produces:
-
Thanks markwillis82, that seems like it may be along the right lines. Am just trying to work out how my code can tie in with yours. Thanks
-
Hi, We've a cms which has the following pages: index.php - shows certain article headlines from a db (i.e. last 10 added) article.php shows the actual article by id, i.e. when you click the article title the link is article.php?id=1 this then grabs the info relating to the article with an id of 1 contact.php shows the contact info about.php shows the about info What I need to do is assign a banner ad (image and link) which I add via a CMS script. Question is, how can I assign it to a specific page (article, index, about, contact etc)? Is there a way of given a page a hidden value and when that page is loaded it loads the relevant banner? Hope that makes sense? Many thanks
-
Thanks alexWD but what if it's for multiple options? Like a menu for example?
-
Hi all, Happy Bank holiday! Rather embarassingly, I've never had to use checkboxes on my forms and now I need to. How do I store and retrieve checkbox values using php & mysql? Any sample code would be most helpful!!! Thanks in advanced!
-
Thanks ignace, however, this means I'd need to work out the distances from the locations. Hence just wanting to do it via postcode.
-
Yeah I found the reference on wikipedia - to be honest, it meant diddly to me and went over my head. Is there no other way?
-
Thought as much. Is it rather complex to do what I'm after? Am still quite new to PHP