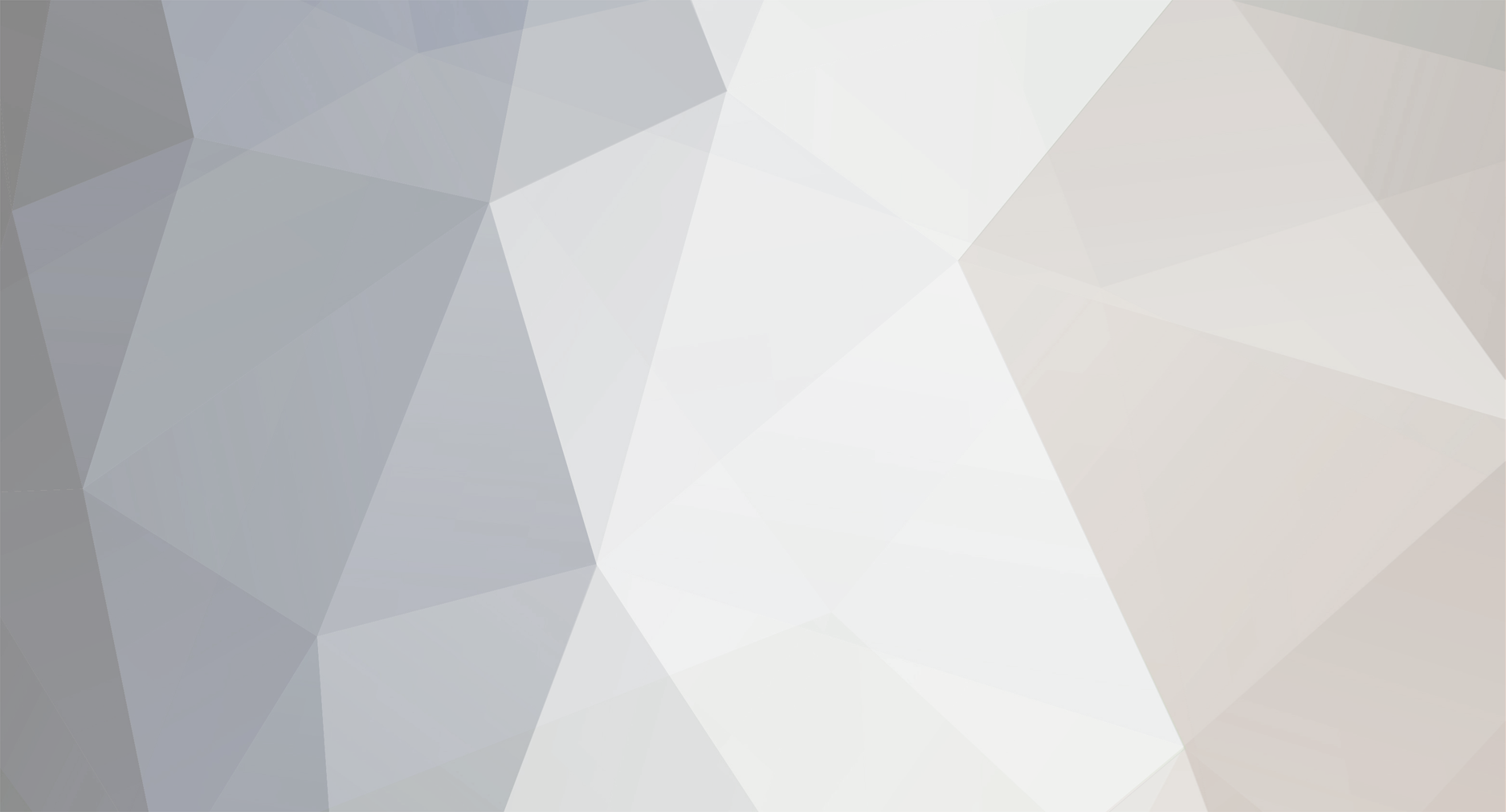
applebiter
-
Posts
1 -
Joined
-
Last visited
Never
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.
OOP in PHP
in PHP Coding Help
Posted
First, the class:
[code]class DataConnection{
var $hostname;
var $username;
var $password;
var $database;
var $datalink;
function setHostname($pV){
$this->hostname = $pV;
}
function getHostname(){
return $this->hostname;
}
function setUsername($pV){
$this->username = $pV;
}
function getUsername(){
return $this->username;
}
function setPassword($pV){
$this->password = $pV;
}
function getPassword(){
return $this->password;
}
function setDatabase($pV){
$this->database = $pV;
}
function getDatabase(){
return $this->database;
}
function setDatalink($pV){
$this->datalink = $pV;
}
function getDatalink(){
return $this->datalink;
}
function DataConnection($pV1,$pV2,$pV3,$pV4){
$this->hostname = $pV1;
$this->username = $pV2;
$this->password = $pV3;
$this->database = $pV4;
$this->datalink = mysql_pconnect($this->hostname,$this->username,$this->password);
if(!$this->datalink){
return 0;
}
else if(!mysql_select_db($this->database,$this->datalink)){
return 0;
}
else return $this->datalink;
}
}[/code]
Then, the definitions file (values deleted):
[code]include "DataConnection.php";
function getDatalink(){
if($_SERVER['WINDIR']){
$db_link = new DataConnection("","","","");
return $db_link;
}
if(!$_SERVER['WINDIR']){
$db_link = new DataConnection("","","","");
return $db_link;
}
}[/code]
Finally, part of the working document:
[code]require_once('../inc/DataConnection.php');
session_start();
$query_myResult = "SELECT * FROM rickhomepage WHERE pageNumber = 1";
$myResult = mysql_query($query_myResult, $datalink) or die(mysql_error());
$row_myResult = mysql_fetch_assoc($myResult);
$totalRows_myResult = mysql_num_rows($myResult);[/code]
Any help would be appreciated!