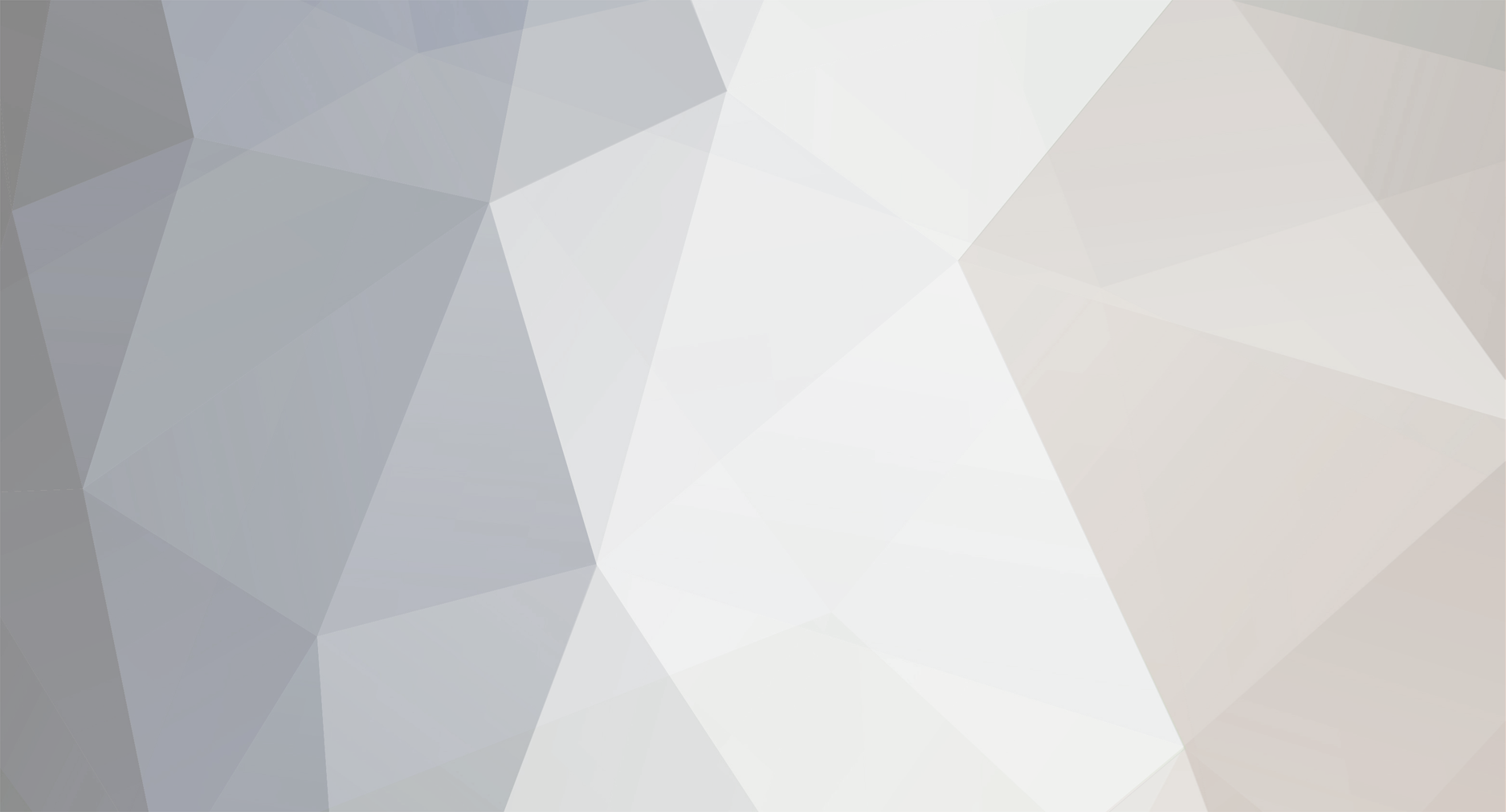
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
That's because you haven't added a join condition, like AND f.id = t.id You'll need to use the appropriate column which links f and t. If you're still having trouble, can you post the table definitions?
-
The documentation says that PHP supports C-style pre-increment and post-increment operators.. and C's pre and post increment have exactly the problem I'm describing. It is certainly not cross-language accurate! Far from it, these operators behave differently between compilers and languages. The documentation states "Returns $a, then increments $a by one." for post-increment. It doesn't say immediate, which is exactly what causes compiler-dependent behaviour in C. The increment will happen before the next statement, but will it happen before evaluation of other children of the current expression is complete? In the absence of anything specific, you might assume that the rules are the same as those in C, where the operators came from. As for left to right evaluation, basic math doesn't specify left to right evaluation. Basic math does say that you can switch the left and right arguments of * and your result will be identical. In the example, switching the left and right arguments changes the result. Edit: Here's a summary of the problem in C: http://c-faq.com/expr/evalorder2.html
-
But who said expressions should be evaluated left to right? And who specified when the pre and post increment should be done? These things are left unspecified in PHP, so the result could be anything. The possibilities are: 6*6 (decrement, take left value, take right value, increment) 6*7 (decrement, take left value, increment, take right value) 7*7 (take left value, take right value, decrement, increment) 7*8 (take left value, increment, take right value, decrement) All are quite valid way of interpreting the expression. Regarding the sorting, yes it does have logic behind it, it's just not the logic you might expect Here's the details (I made a journal post about it a while back) "2" < "10" - integer comparison "10" < "10a" - string comparison. Longer string wins. "10a" < "2" - string comparison. "2" wins because only the first characters are compared in this case.
-
Here's another one for you.. $i = 7; print ($i-- * $i++) . "\n"; $i = 7; print ($i++ * $i--) . "\n"; Here in php 4.3.10 and 5.1.4 I get this output: 42 56 And here's another oddity I found while wondering why my sorting wasn't working properly if ("2" < "10") print "2 < 10\n"; if ("10" < "10a") print "10 < 10a\n"; if ("10a" < "2") print "10a < 2\n"; This shows that a < b < c < a, which gives a loop in the ordering
-
I notice you use both $this->link and $this->linkid Apart from that, are you checking that pg_query() returned a resource before calling pg_fetch_array() with it? If pg_query() fails, it will return false.
-
Your code is quite long. If you can give us the output of var_dump(), then we can tell you what the problem is without having to look through all that code
-
Most languages leave such obscure questions undefined. Just don't do it Your code may work for this version of php, but there's no guarantees it will work next version. The same goes for function calls like f($b++, $b++);
-
Works fine on my server, but does not work on customers
btherl replied to cnl83's topic in PHP Coding Help
$file_name (used in the copy() destination) does not appear to be set anywhere. Is this passed in via form? If so, you can fix it by replacing $file_name with $_REQUEST['file_name'], in both locations it appears. And the cause will be that register_globals is switched off on your customer's server. -
Try a few settings with this: http://sg.php.net/manual/en/function.error-reporting.php You probably want this one: // Report all errors except E_NOTICE // This is the default value set in php.ini error_reporting(E_ALL ^ E_NOTICE);
-
Can you put the following code in: print "<pre>";var_dump($_SESSION['Uploaded_Files']);print"</pre>"; echo array_search( $_REQUEST['File'], $_SESSION['Uploaded_Files'] ); to verify that the array is what you think it should be? I'm assuming that's the call to array_search() you are interested in.
-
Would this query do it? $query = "UPDATE user_ids SET online = 0 WHERE timestamp < '$old_timestamp' AND online = 1"; Then to count the users online: $query = "SELECT count(*) FROM user_ids WHERE online = 1";
-
I think you might need a condition to join f and t in your inner subquery, like AND f.id = t.id To test it, run the inner subquery on its own and see what you get
-
It may be to do with when you increment the counter. As for the multiple APRs, I think it's whitespace. You can try ignoring any data that looks like an empty string (this will cause trouble if you really do have a blank APR of course So better is to append all APRs together until you reach the end tag) The approach I've used is to have the data handler keep on appending until the end tag is reached, and have the end tag handler store the data.
-
Yep, exactly. You missed it on the first line though.
-
The answer is no You can do echo "blah" . stripslashes($step1) . "blah", or you can put the output of stripslashes into variables and put those directly into the echoed string.
-
Date/Time Conversion from YYYY/MM/DD HH:MM:SS To Hour Am/Pm
btherl replied to sivanath.nagendran's topic in PHP Coding Help
date('ga', strtotime('2007/01/01 01:02:03')); -
If you're planning to feed the time into strtotime(), you should avoid m-d-Y format. Try Y-m-d format instead. It's much more computer friendly Make the change in your mysql query. You can reformat the date to m-d-Y later when you call date() in php.
-
[SOLVED] UPDATE querys mot working, but SELECT querys work
btherl replied to PC Nerd's topic in PHP Coding Help
There is a problem in the output you posted: INSERT INTO individual_messages (`User_ID` , `To` , `From` , `From_ID` , `Subject` , `Message_Body` ) VALUES ('1', '', 'Administration', '1, 'Quick Message' , 'thshadflusi gfolbay g' ) Notice the "'1". If that column is a string, it should be '1'. If it is an integer, it should be just 1, no quotes. The same for User_ID. If it's an integer, it should be just 1. If it's a string (varchar) it should stay as '1'. Try fixing that in your mysql query and see if it helps! If it doesn't, post the output you get here again, along with your modified query. -
Thankyou Timbo for a perfectly worded question That's how artacus was able to answer it so easily. It's a relief after reading so many "Please help, my file doesn't send the file into the database" type questions..
-
[SOLVED] Cleaning a string before inserting it into a php file
btherl replied to neoform's topic in PHP Coding Help
Enclose your string in single quotes, then you only need to escape single quotes and backslashes. Nothing else. You might want to consider heredoc syntax as well, which doesn't require escaping. http://www.php.net/manual/en/language.types.string.php#language.types.string.syntax.single -
The formatting there is quite weird. But the array looks normal. You should be able to access it like this: foreach ($locarray as $l) { print "-{$l[0]}- at offset {$l[1]}\n"; } $locarray[0][0] prints nothing out because the element there is an empty string. [0][0] is the first string, [0][1] the first offset, [1][0] the second string, [1][1] the second offset, etc etc
-
What do you want your code to do?
-
This can get you started http://sg.php.net/manual/en/ref.mcrypt.php "This is an interface to the mcrypt library, which supports a wide variety of block algorithms such as DES, TripleDES, Blowfish (default), 3-WAY, SAFER-SK64, SAFER-SK128, TWOFISH, TEA, RC2 and GOST in CBC, OFB, CFB and ECB cipher modes." Is your question "How do I implement 3DES in php", or are you asking more generally about how 3DES can be used?
-
Actually SimpleXML gives you an object.. if you want an array I think you will have to convert it yourself unfortunately. Or you could alter your code to use objects instead.
-
I wouldn't count on it. I can't find any mention in the documentation of such a use of limit with update.